sketchDownload
//Nicholas Wong
//Section A
//nwong1@andrew.cmu.edu
//Assignment 09
var size = 10 //Default size of stroke
function preload()
{
//A profile picture
img = loadImage("https://i.imgur.com/1XXSpjL.png")
}
function setup()
{
createCanvas(480,480);
img.loadPixels(); //Load pixels
//Set modes
imageMode(CENTER);
rectMode(CENTER);
textAlign(CENTER);
angleMode(RADIANS);
//Set custom framerate
frameRate(120)
}
function draw()
{
//Offset canvas to fit my face in the middle
translate(-100,10)
//Get random x and y coordinates
let x = floor(random(width));
let y = floor(random(height));
//Get color of coordinates
let pix = img.get(x,y);
//Create strokes
drawStrokes(x,y,pix);
drawInstructions();
}
function drawStrokes(x,y,pix)
{
push();
translate(100,0) //Recenter canvas
translate(x,y);
rotate(revolveMouse(x,y));//Rotate strokes around mouse
//Draw stroke
stroke(pix, 128);
strokeWeight(0.25*size + random(0,2)); //Stroke size influenced by mouse scroll
line(0,-size + random(0,2),0,size+ random(0,2)); //Stroke length based on mouse scroll
pop();
}
function revolveMouse(x,y)
{
//Generates angle from mouse position and (x,y) coordinate
let dx = mouseX - x;
let dy = mouseY - y;
let angle = atan2(dy,dx)
return angle; //Returns angle
}
function mouseWheel(event)
{
//Check if scrolling up
if (event.deltaY < 0)
{
size++; //Increase size
}
else //Scrolling down
{
size -= 1; //Decrease size
}
}
function drawInstructions()
{
//Text box at bottom of canvas
push();
translate(100,10);
noStroke();
fill(0)
rect(width/2,height-50,285,35)
fill(255)
textSize(10)
text('SCROLL MOUSEWHEEL TO CHANGE STROKE SIZE',width/2,height-47)
pop();
}
Strokes radiate and rotate around mouse pointer, mouse scrolling changes the stroke size; scroll up to increase size, scroll down to decrease size. Here are some examples with varying mouse positions, stroke sizes, and durations.
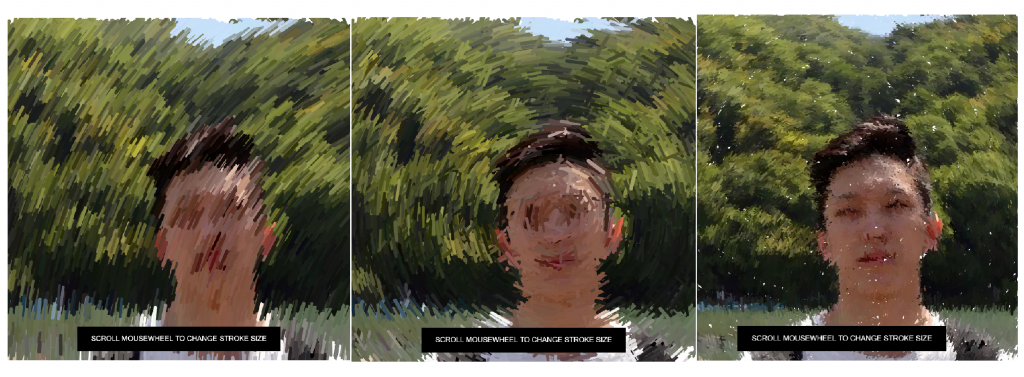