For my project this week, I wanted to make something that wouldn’t have the most common approach among the class. I figured that a lot of people would look to do a horizontal landscape, where there is a lot of movement with the X position. Therefore, I decided to do the house from Up but moving vertically!
I referred to images of the actual house from the Up animation to try to recreate it with shapes. This was not too difficult because I have gotten used to recreating specific images through layering shapes in p5js. However, the most difficulty I had with this assignment was with making the clouds reappear after they disappear off of the canvas. (I actually had to go to OH for a while for this.. thanks Peter for the help.)
One thing I wish I could have done differently though, is thinking through the concept more. Once I had basically established all of the objects I made, and had movement, I realized that by making the house go “into the sky” there wasn’t much more detail I could add other than birds. I guess with doing things horizontally, there are a lot more realistic features you could add that would make sense (such as mountains or trees).
//Annie Kim
//anniekim@andrew.cmu.edu
//Section B
//anniekim-11-project
var balloon = [];
var cloud = [];
var bird = [];
var a = 430; //height at which ground is started
function setup() {
createCanvas(340, 480);
//create an initial collection of clouds:
for (var j = 0; j < 15; j ++) {
cloud[j] = makeCloud();
}
//create initial collection of balloons:
for (var i = 0; i < 100; i ++) {
balloon[i] = makeBalloon();
}
//create initial collection of birds:
for (var k = 0; k < 5; k ++) {
bird[k] = new Object();
//set its properties
bird[k].x = random(-10, 340);
bird[k].y = random(0, 250);
bird[k].dx = random(-2, 2);
bird[k].dy = random(-2, 2);
}
frameRate(6);
}
function draw() {
background(143, 214, 236); //light blue sky color
drawGround();
updateAndDisplayClouds();
for (var i = 0; i < 4; i ++) {
drawBirds(bird[i]);
bird[i].x += bird[i].dx;
}
updateAndDisplayBalloons();
removeCloudsThatHaveSlippedOutOfView();
addNewCloudsWithSomeRandomProbability();
drawHouse();
}
function updateAndDisplayBalloons() {
//will update and place the balloons:
for (var i = 0; i < 100; i ++) {
balloon[i].move();
balloon[i].display();
}
}
function updateAndDisplayClouds() {
//update the cloud's positions and display them:
for (var i = 0; i < 15; i ++) {
cloud[i].move();
cloud[i].display();
cloud[i].y += cloud[i].dy;
}
}
function removeCloudsThatHaveSlippedOutOfView() {
//will remove clouds that are off the canvas and readd in other helper function
var cloudsToKeep = [];
for (var i = 0; i < cloud.length; i ++) {
if (cloud[i].y < height) {
cloudsToKeep.push(cloud[i]);
}
}
cloud = cloudsToKeep;
}
function addNewCloudsWithSomeRandomProbability() {
//with a very tiny probability, add a new cloud to the end:
var newCloudLikelihood = 0.35;
if (random(0,1) < newCloudLikelihood) {
cloud.push(makeCloud());
}
}
function cloudMove() {
this.y += this.dy;
this.x += this.dx;
}
function cloudDisplay() {
fill(255);
noStroke();
circle(this.x, this.y, 40);
circle(this.x - 25, this.y - 2, 13);
ellipse(this.x - 35, this.y + 10, 30, 20);
circle(this.x - 20, this.y + 12, 25);
ellipse(this.x + 20, this.y + 10, 30, 20);
}
function balloonDisplay() {
fill(this.color);
stroke(this.color);
ellipse(this.x, this.y, 14, 19);
stroke(255, 100);
line(this.x, this.y + 9.5, 170, 315);
}
function balloonMove() {
this.x += this.dx;
if (this.x > 250 || this.x < 100) {
this.dx = -this.dx;
}
}
function makeCloud() {
var cld =
{x : random(-50, 370),
y : random(-100, 240),
dy : 2,
dx : 1,
move : cloudMove,
display : cloudDisplay}
return cld;
}
function makeBalloon() {
var bln =
{x : random(100, 250),
y : random(100, 230),
dx : random(-3, 3),
color : color(random(130, 255), random(130, 255), random(130, 255)),
move : balloonMove,
display : balloonDisplay}
return bln;
}
function drawHouse() {
noStroke();
//ROOF OF HOUSE
fill(111, 95, 137); //purple color
quad(120, 315, 220, 315, 240, 360, 100, 360);
//PART OF HOUSE
fill(114, 159, 215); //blue color
rect(120, 360, 100, 20);
//PART OF HOUSE
fill(247, 214, 215); //pink color
rect(120, 380, 100, 50);
//POINTY PART OF ROOF AND WINDOWS
fill(241, 234, 150); //yellow color
rect(130, 310, 20, 20);
triangle(130, 310, 150, 310, 140, 300); //left window
triangle(160, 360, 210, 360, 185, 270);
rect(160, 360, 50, 10);
//BAY WINDOW OF HOUSE
fill(141, 196, 91); //green color
rect(160, 370, 50, 60);
triangle(160, 430, 176, 430, 176, 433);
triangle(192, 433, 192, 430, 210, 430);
fill(161, 216, 111);
rect(176, 370, 17, 63);
//FRONT DOOR
fill(118, 100, 88); //brown color
rect(135, 395, 20, 35);
stroke(50, 40, 20);
noFill();
rect(137.5, 398, 15, 30);
line(145, 398, 145, 428);
line(137.5, 413, 152.5, 413);
//WINDOWS
//top left window
fill(200, 222, 233); //light blue color
stroke(114, 159, 215); //darker light blue color
square(135, 315, 10);
line(140, 315, 140, 325);
line(135, 320, 145, 320);
//right window
rect(175, 325, 20, 30);
line(175, 345, 195, 345);
//bay window 1
rect(162.5, 390, 10, 20);
rect(179.5, 392, 10, 20);
rect(196.5, 390, 10, 20);
}
function drawGround() {
fill(126, 182, 128);
noStroke();
rect(0, a, 340, 50);
//ground "take-off"
a += 1;
}
function drawBirds(b) {
stroke(0);
strokeWeight(2);
noFill();
arc(b.x, b.y, 25, 20, PI + QUARTER_PI, 0, OPEN);
arc(b.x + 24, b.y, 25, 15, PI, QUARTER_PI - HALF_PI, OPEN);
b.x += b.dx;
b.y += b.dy;
//if bird goes off canvas, it'll come back opposite
if (b.x > width + 25 || b.x < -25) {
b.dx = -b.dx;
b.dy = -b.dy;
}
}
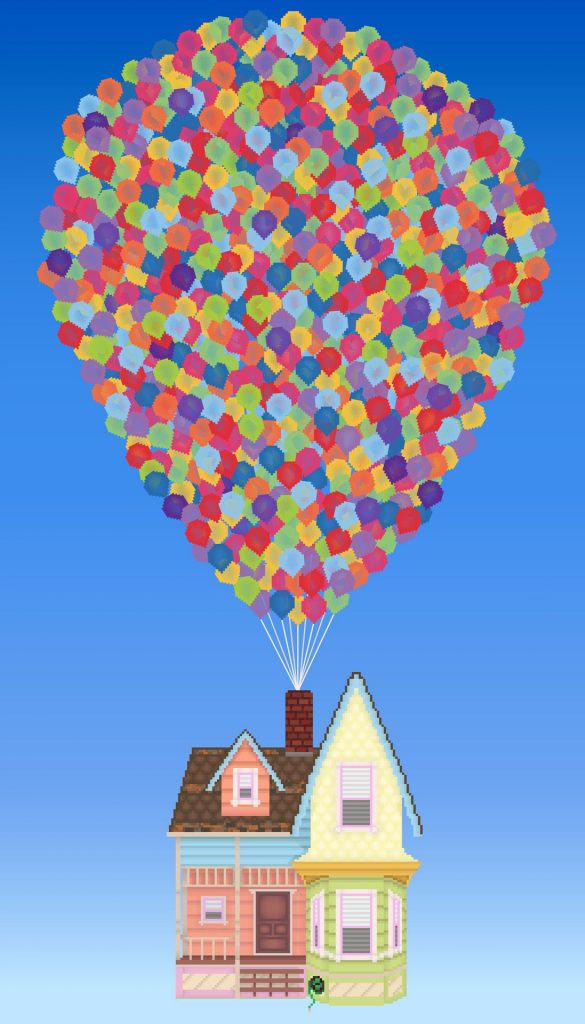
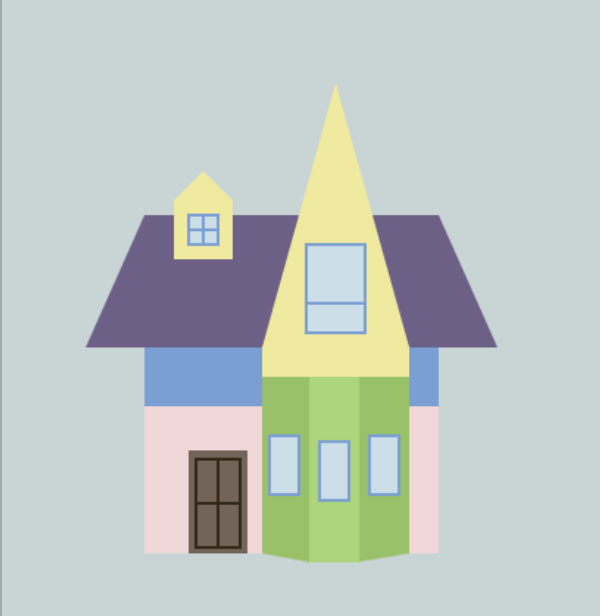
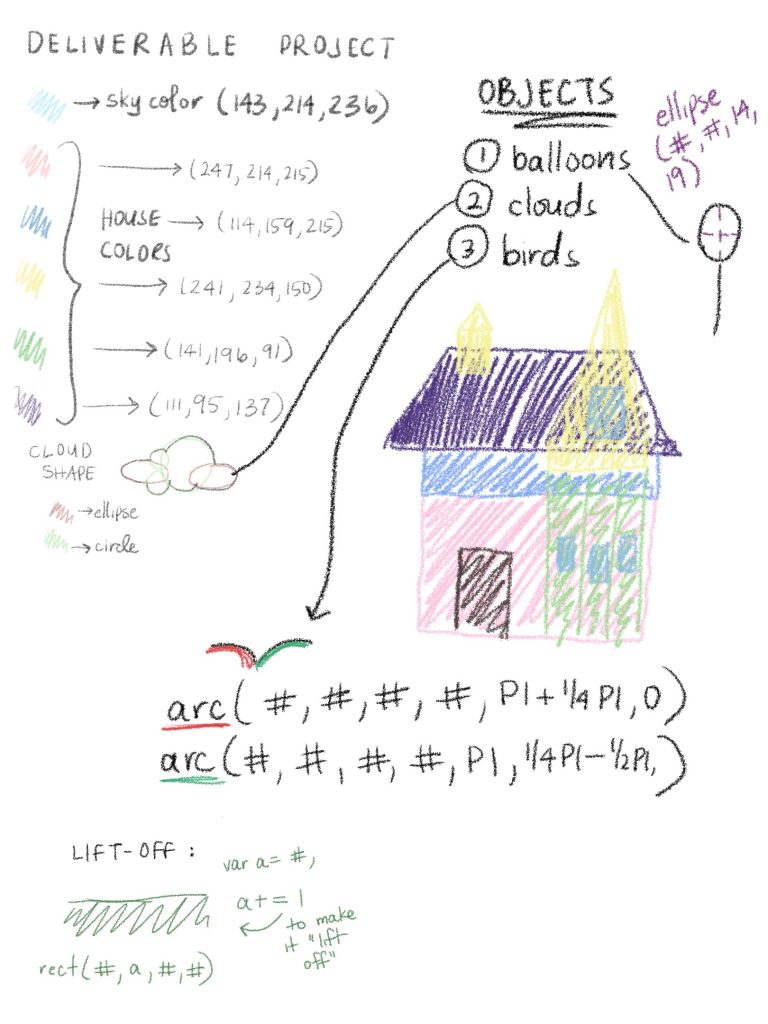