var clouds = [];
var bikeImage = [];
var bike = [];
var terrainCurve = 0.01;
var terrainSpeed = 0.001;
function preload(){
var filenames =[];
filenames[0] = "https://i.imgur.com/DSPjyoM.png";
filenames[1] = "https://i.imgur.com/kGGBLDh.png";
filenames[2] = "https://i.imgur.com/v9RMtPT.png";
filenames[3] = "https://i.imgur.com/ZUC5xZ2.png";
for(var i = 0; i < filenames.length; i++){
bikeImage.push(loadImage(filenames[i]));
}
}
function setup() {
createCanvas(480,480);
//clouds
for (var i = 0; i < 9; i++){
var ccloud = random(width);
clouds[i] = drawCloud(ccloud);
}
frameRate(11);
imageMode(CENTER);
//bike
var b = makeCharacter(240, 350);
bike.push(b);
}
function draw() {
sky(0, 0, width, height);
DisplayClouds();
removeClouds();
newClouds();
ground();
for (var i = 0; i < bike.length; i++) {
var bb = bike[i];
bb.stepFunction();
bb.drawFunction();
}
}
function sky(x, y, w, h) {
//sky
var Blue;
var Pink;
Blue = color(114, 193,215);
Pink = color(253, 209, 164);
for (var i = y; i <= y + h; i++) {
var ssky = map(i, y, y + h, 0, 1.1);
var cco = lerpColor(Blue, Pink, ssky);
stroke(cco);
line(x, i, x + w, i);
}
//mountains
beginShape();
stroke(1, 33,92);
strokeWeight(200);
for (var x = 0; x < width; x++){
var tt = (x * terrainCurve) + (millis() * terrainSpeed);
var mm = map(noise(tt), 0,1, 110, 255);
vertex(x, mm + 180);
}
endShape();
}
this.display = function() {
strokeWeight(this.border);
stroke(240);
fill(240);
ellipse(this.x, this.y, this.display, this.display);
}
function ground(){
beginShape();
noStroke();
fill(18,12,46);
rect(0,400,width,80);
endShape();
}
function cloudDisplay(){
noStroke();
var floorH = 21;
var cloudH = this.nFloors * floorH;
fill(250,50);
push();
translate(this.x, height - 95);
ellipse(95, -cloudH, this.breadth, cloudH / 2);
pop();
push();
fill(250,80)
translate(this.x, height - 220);
ellipse(95, - cloudH, this.breadth/1.5, cloudH);
pop();
}
function DisplayClouds(){
for (var i = 0; i < clouds.length; i++){
clouds[i].move();
clouds[i].display();
}
}
function cloudMove(){
this.x += this.speed;
}
function removeClouds(){
var cKeep = [];
for (var i = 0; i < clouds.length; i++){
if (clouds[i].x + clouds[i].breadth > 0) {
cKeep.push(clouds[i]);
}
}
clouds = cKeep;
}
function newClouds(){
//random probability
var newP = 0.01;
if (random(0,0.4) < newP) {
clouds.push(drawCloud(width));
}
}
function drawCloud(xx) {
var cha = {x: xx,
breadth: random(95, height),
speed: -random(1,4),
nFloors: round(random(4,8)),
move: cloudMove,
display: cloudDisplay}
return cha;
}
function makeCharacter(cx, cy) {
ccha = {x: cx, y: cy,
imageNumber: 0,
stepFunction: characterStep,
drawFunction: characterDraw
}
return ccha;
}
function characterStep() {
this.imageNumber++;
if (this.imageNumber == 4) {
this.imageNumber = 0;
}
}
function characterDraw() {
image(bikeImage[this.imageNumber], this.x, this.y,120,120);
}
For this Deliverable 11 project, I tried to collaborate on what I’ve learned from deliverable 9, using preloads for calling image animations and generating landscapes from deliverable 11. I also tried to show the difference in the speed by giving a certain frameRate for the rider and background mountains. Clouds are displayed with low opacity and different speed so that it shows how it has a different relationship with elements on the ground(mountains and humans). It was hard for me to figure out having two functions of makeCharacter because I had two figures to move: character, and the elements in the sky.
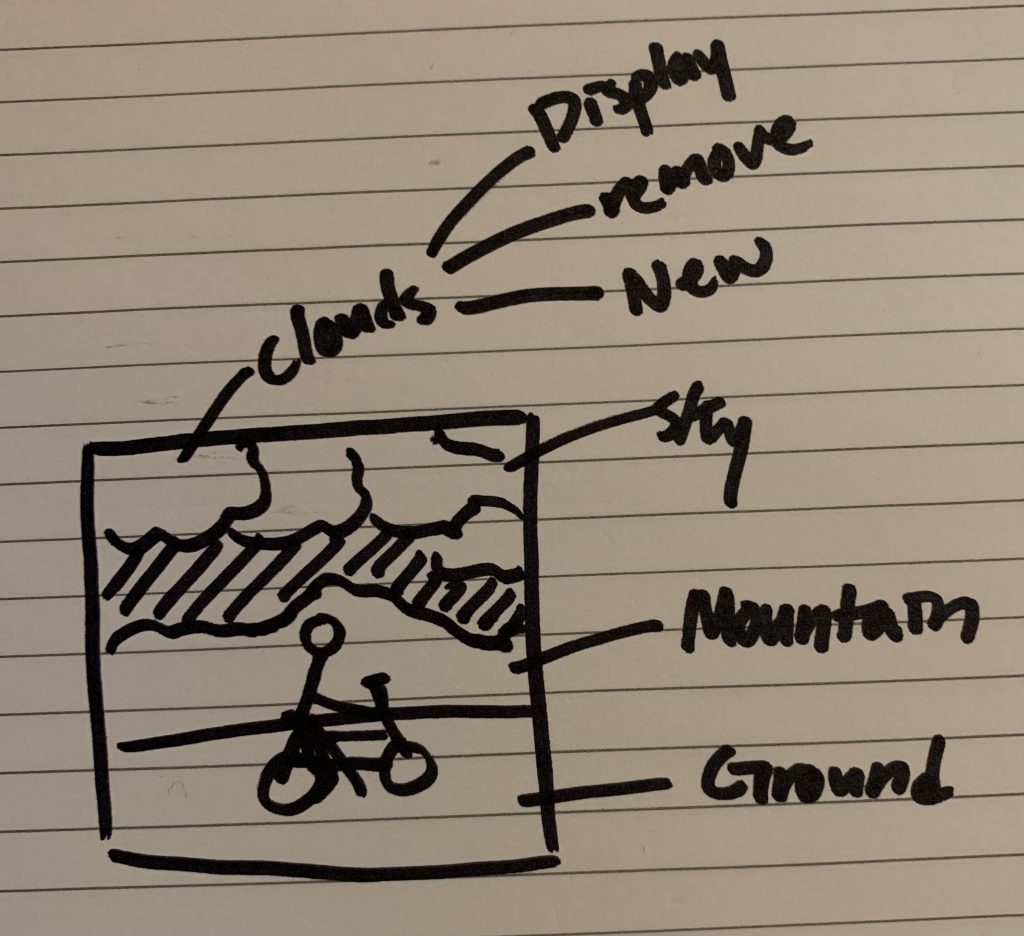