sketchDownload
//Jessie Chen
//D
//Project 11
//Generative Landscape
var kiki = [];
var stars = [];
var clouds = [];
var animation = 0;
function preload() {
//loads kiki animation frames
var kikiFrames = [];
kikiFrames[0] = "https://i.imgur.com/LxKITCM.png";
kikiFrames[1] = "https://i.imgur.com/535FqDb.png";
for (var i = 0; i < kikiFrames.length; i++) {
kiki[i] = loadImage(kikiFrames[i]);
}
}
function setup() {
createCanvas(400, 300);
imageMode(CENTER);
frameRate(5);
noStroke();
//create an initial collection of stars
for (var i = 0; i < 10; i++) {
var rx = random(width);
var ry = random(height)
stars[i] = makeStars(rx, ry);
}
//creates an initial collection of clouds
for (var i = 0; i < 10; i++) {
var rx = random(width);
var ry = random(height);
clouds[i] = makeClouds(rx, ry);
}
}
function draw() {
background(39, 36, 55);
//draws the moon
fill(233, 220, 152);
ellipse(200, 150, 200, 200);
updateStars();
removeStars();
addNewStars();
updateClouds();
removeClouds();
addNewClouds();
//plays kiki animation
image(kiki[animation%kiki.length], 200, 150, 270, 200);
animation ++;
}
//update positions
function updateStars() {
for (var i = 0; i < stars.length; i++) {
stars[i].move();
stars[i].display();
}
}
//remove stars that have gone off canvas
function removeStars() {
var starsToKeep = [];
for (var i = 0; i < stars.length; i++) {
if (stars[i].x < width) {
starsToKeep.push(stars[i]);
}
}
stars = starsToKeep;
}
//add new stars from the left
function addNewStars() {
var newStarLikelihood = 0.05;
if (random(0,1) < newStarLikelihood) {
stars.push(makeStars(-10, random(0, height)));
}
}
//stars move to the right
function starsMove() {
this.x += this.speed;
}
//draws stars
function starsDisplay() {
fill(233, 220, 152, 100);
ellipse(this.x, this.y, this.size + 10);
fill(233, 220, 152)
ellipse(this.x, this.y, this.size);
}
//stars object
function makeStars(startX, startY) {
var star = {x: startX,
y: startY,
speed: 0.5,
size: random(2, 5),
move: starsMove,
display: starsDisplay}
return star;
}
//update positions
function updateClouds() {
for (var i = 0; i < clouds.length; i++) {
clouds[i].move();
clouds[i].display();
}
}
//remove clouds that have gone off canvas
function removeClouds() {
var cloudToKeep = [];
for (var i = 0; i < clouds.length; i++) {
if (clouds[i].x < width + 100) {
cloudToKeep.push(clouds[i]);
}
}
clouds = cloudToKeep;
}
//add new clouds from the left
function addNewClouds() {
var newCloudLikelihood = 0.05;
if (random(0,1) < newCloudLikelihood) {
clouds.push(makeClouds(-100, random(0, height)));
}
}
//clouds move to the right
function cloudsMove() {
this.x += this.speed;
}
//draws clouds
function cloudsDisplay() {
fill(102, 94, 110, 60);
noStroke();
arc(this.x, this.y, 25 * this.size, 20 * this.size, PI + TWO_PI, TWO_PI);
arc(this.x + 10, this.y, 25 * this.size, 45 * this.size, PI + TWO_PI, TWO_PI);
arc(this.x + 25, this.y, 25 * this.size, 35 * this.size, PI + TWO_PI, TWO_PI);
arc(this.x + 40, this.y, 30 * this.size, 20 * this.size, PI + TWO_PI, TWO_PI);
}
//clouds object
function makeClouds(startX, startY) {
var cloud = {x: startX,
y: startY,
speed: 2,
size: random(0, 8),
move: cloudsMove,
display: cloudsDisplay}
return cloud;
}
This was inspired by Kiki’s Delivery Service by Studio Ghibli. I used Procreate to draw out the animation frames of Kiki and imported them to my code to create a little animation. I was reminded of this scene where she flew on her broom at night, so I decided to base the project on that. As she flies through the sky, accompanied by her cat, Jiji, she moves through clouds and passes stars.
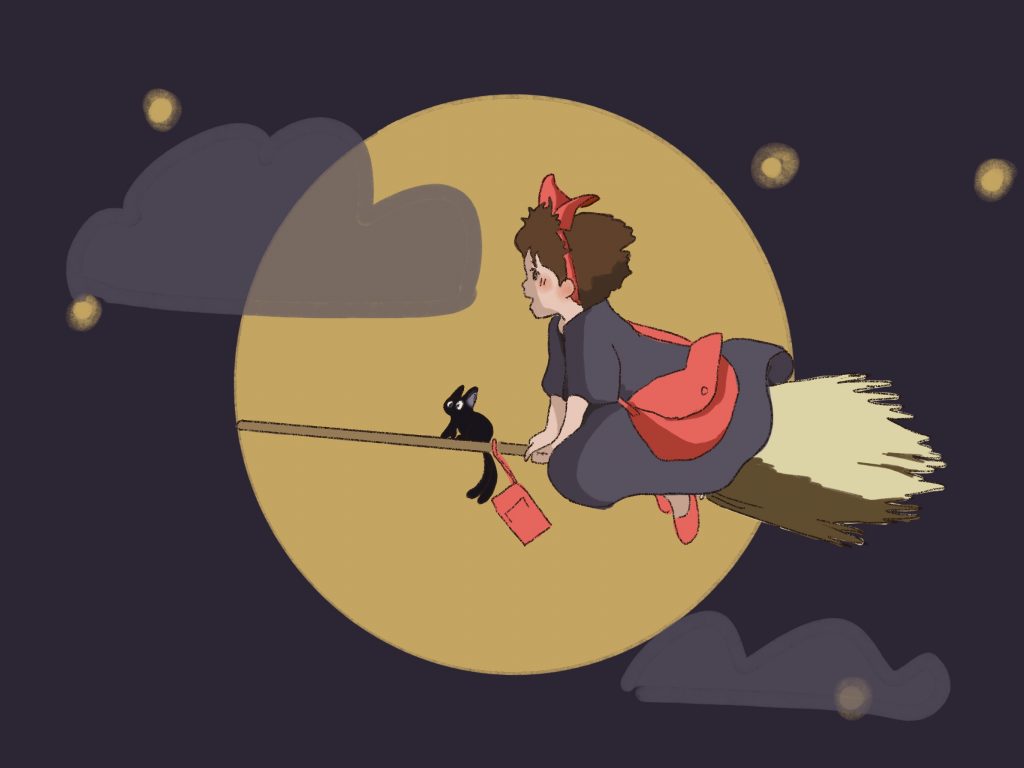