For this project I wanted to make a fun character that dances every time you click it!
Month: September 2022
Looking Outwards 02
The piece of work I selected is Inhotim by the computational architect Michael Hansmeyer, https://www.michael-hansmeyer.com/inhotim. I selected this piece because I found the way the artist created a dialogue between the sculptures to be quite beautiful and compelling. I also have had some experience with computational design in architecture so I really enjoy getting to see its application in the world outside of class. From what I know it seems like the artist could have used Python in the Rhino plug-in Grasshopper to create the work since it seems like he manipulated the mesh surface in rhino to create the push pull illusion. It seems like the artist’s sensibility manifested in their algorithm in the way that grasshopper is really about manipulating the earth and a given terrain, and that’s what Inhotim does. It uses nature as a base, but its intervention could not exist without an algorithm.
Project 02 Self Portrait Hannah Wyatt
This is my project
Variable Faces
This project was extremely difficult for me because I kept making tiny mistakes which absolutely broke my code. This was definitely a learning experience, and towards the end I had more fun creating.
// Kathy Lee
//Section D
// Variable Setup
var faceHeight = 200;
var faceWidth = 200;
var shirt = 200;
var eye = 50;
var mouth = 50;
// Color Variables
var r = 50;
var g = 50;
var b = 50;
// mouthsize, hair length, eyesize
function setup() {
createCanvas(640, 480);
text("Smiling Alien", 10, 15);
}
function draw() {
background (220);
noStroke();
// Shirt
fill(200 - r, 200 - g, 200 - b);
ellipse((width / 2), (height / 2) + 200, shirt + 100, shirt * 1.5);
// Face
fill(r + 50, g + 50, b + 50);
ellipse(width / 2, (height / 2) + 50, faceWidth, faceHeight);
// Eyes
fill(r + 150, g + 150, b + 150);
ellipse((width / 2.5) + 25, height / 2, faceWidth - 150, eye); // left eye
fill(r + 150, g + 150, b + 150);
ellipse((width / 2) + 40, height / 2, faceWidth - 150, eye); // right eye
fill("black");
ellipse((width / 2.5) + 20, height / 2, faceWidth - 200, eye - 30); // left pupil
fill("black")
ellipse((width / 2.5) + 110, height / 2, faceWidth - 200, eye - 30); // right pupil
// Mouth
fill(r + 175, g + 175, b + 175);
ellipse(width / 2, (height / 2) + 75, mouth + 50, mouth / 2);
fill(r + 50, g + 50, b + 50);
ellipse(width / 2, (height / 2) + 70, mouth + 50, mouth / 2); // top ellipse to make smiling mouth
}
function mousePressed() {
faceWidth = random(150, 300);
faceHeight = random(150, 300);
shirt = random(200, 300);
eye = random(50, 60);
mouth = random(50, 150);
r = random(0, 255);
g = random(0, 255);
b = random(0, 255);
}
LookingOutwards-02 (Section A)
- https://www.mrkism.com/quantum.htm
- Markos R. Kay (neé Christodoulou)
- Quantum Fluctuations (2016),
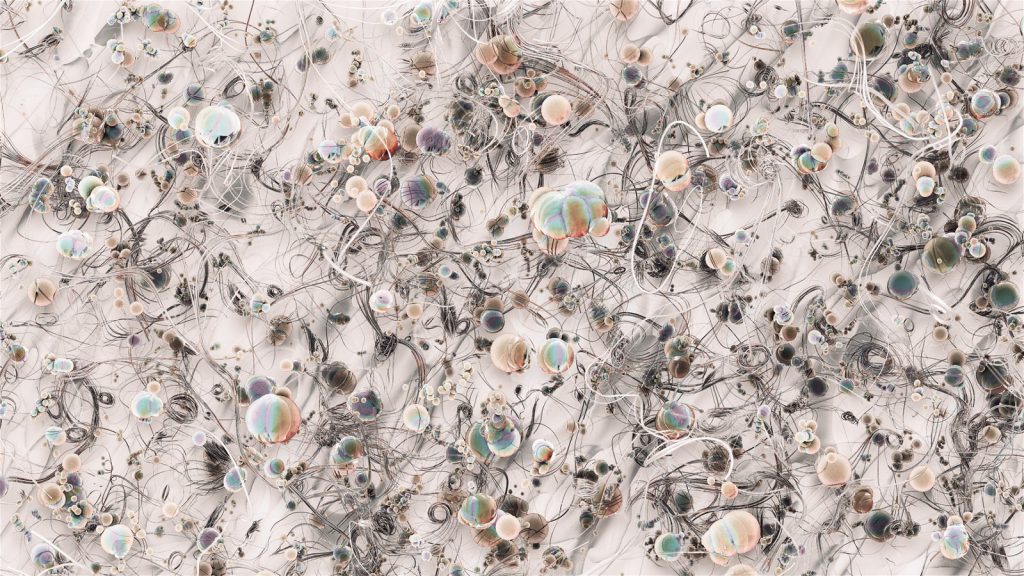
Project 2: Variable Faces
For this self Portrait, I tried to learn more about static tools like the arc and Curve Vertex, as well as varying tools that change color, shape, positioning depending on a number of conditions. Over the process of creating the piece, I began to like the limits created with horizontal/vertical movement of the mouse.
//Graham Murtha Section A
// MAKE SURE TO MOVE THE MOUSE DOWN TO THE BOTTOM :)
var eyeSize = 40;
var earSize = 50
var skinR = 160
var skinG = 20
var skinB = 160
var shirtR = 150
var shirtG = 150
var shirtB = 0
function setup() {
createCanvas(350, 500);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
if (mouseX < width/2) {
if (mouseY < height/2) {
background(140,20,140); //purple 1
} else {
background(100,10,150); // purple 2
}
} else {
if (mouseY < height/2) {
background(170,50,170); // purple 3
} else {
background (200,20,140); //purple 4
}
} // BACKGROUND
fill(skinR,skinG,skinB);
strokeWeight(2);
stroke(1);
ellipse(250, 216, earSize, earSize*1.66) //ear left
ellipse(97,216,earSize,earSize*1.66) //ear Right
fill(shirtR,shirtG,shirtB)
triangle(175,400,350,500,0,500) // SHIRT
fill(skinR,skinG,skinB);
beginShape();
curveVertex(175, 175);
curveVertex(175, 75);
curveVertex(300, 375);
curveVertex(175,470);
curveVertex(50, 375);
curveVertex(175, 75);
curveVertex(175, 175);
endShape(); // HEAD SHAPE
fill(255,141,176)
rect(110.17,60.72,129,133.3);
strokeWeight(4)
arc(175,191,160,30,0,3.14159265); // hat
fill(255,255,240);
noStroke()
ellipse(125,270,eyeSize,eyeSize*.75); // eye 1
ellipse(230,270,eyeSize,eyeSize*.75); // eye 2
fill(255,0,255);
noStroke()
if(mouseX < width/3){
ellipse(125,270,10,10); // pupil 1a
ellipse(230,270,10,10); // pupil 1b
}
if(mouseX >= width/3 & mouseX < (2*width)/3){
rect(120,265,10,10); //pupil 2a
rect(225,265,10,10); //pupil 2b
}
if(mouseX >= (2*width)/3){
triangle(120,265,130,265,125,275); //pupil 3a
triangle(225,265,235,265,230,275); //pupil 3b
}
if (mouseY < height/1.2) {
fill(0,0,0);
ellipse(175,400,(mouseX/6),(mouseY/9)); // open, moving mouth
} else {
stroke(1)
strokeWeight(4);
line(125,400,225,400); // closed mouth
}
if (mouseY < height/1.2) {
arc(125,210,30,10,3.14159265,0) //normal brow 1
arc(230,210,30,10,3.14159265,0) //normal brow 2
} else {
fill(skinR,skinG,skinB)
arc(125,265,50,30,3.14159265,0) // angry brow 1
arc(230,265,50,30,3.14159265,0) // angry brow 2
}
if (mouseY > height/1.2) {
arc(125,278,50,30,0,3.14159265) // eyebag 1
arc(230,278,50,30,0,3.14159265) //eyebag 2
}
}
function mousePressed() {
eyeSize = random(20, 60);
earSize = random(40,80);
skinR = random(150,240);
skinG = random(10,60);
skinB = random(150,240);
shirtR = random(140,255);
shirtG = random(140,255);
shirtB = random(0,10);
}
LookingOutwards-02
Section E, Clare Katyal
I researched the Nonsense Laboratory created by Allison Parrish in 2021. This project uses a program created by Parrish called Pincelate. This program breaks down English words into their phonetics. The idea of the program is to play with the way words are spelled the same way you play with clay or with notes on an instrument. The program inputs words, translates them into how they sound in the mouth, and then ejects a word for the program’s user to try to say. I admire how versatile the program is because it is able to work with any word, and even words that aren’t really words, like made up sounds or jumbles of letters. The front-end implementation was created by Allison Parrish, while the visuals of the program and instructions were created by Jenny Goldstick and Tim Szetela. Allison Parrish creates many different programs playing on scrambled words, which is interesting because it makes one think about how every word we know is just random letters put together. It is cool to think about how people create the words that have so much meaning to people.
https://www.youtube.com/watch?v=cPAbx5kgCJo
Created by Allison Parrish
https://artsexperiments.withgoogle.com/nonsense-laboratory/
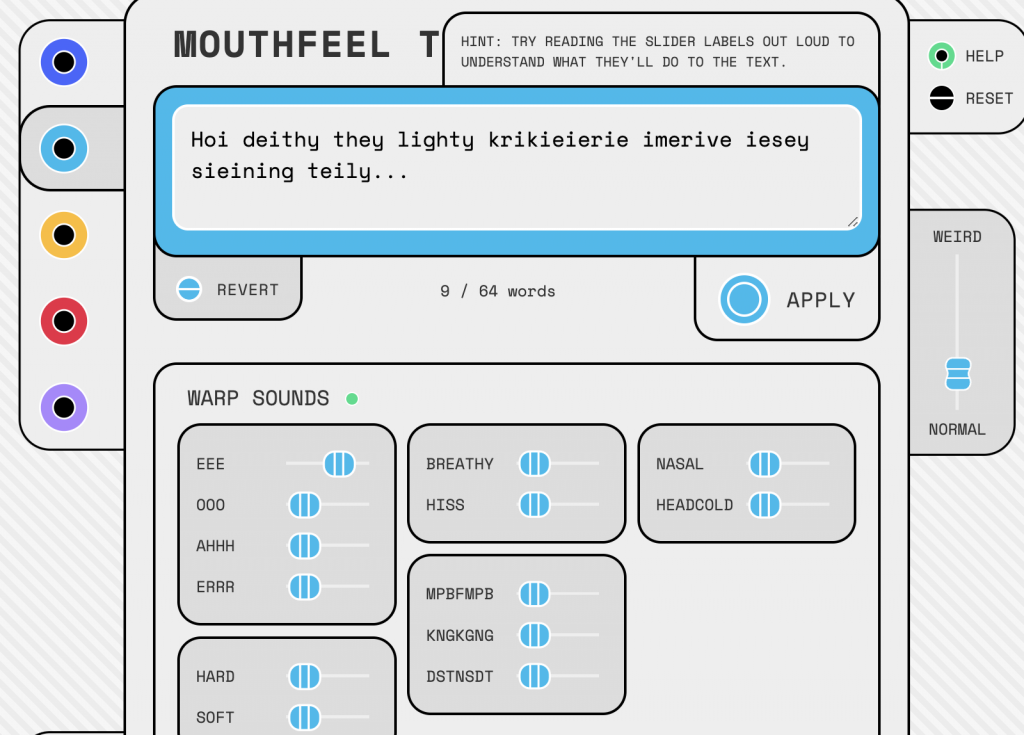
Project-02-Variable-face
Section E
I tried to play with adding makeup to the face when it was clicked, in order to add more color on the face. I also tried to play with beauty standards, and had the lips and eyebrows get fuller, as this is what many celebrities look like nowadays.
var boxWidth = 6
function setup() {
createCanvas(640, 480);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(247,233,106,[255]);
fill(75,36,36,[255]); //hair
if (mouseY < width/2) { //hair grows
ellipse(width/2,height/2+50,230,400);
} else {
ellipse(width/2,height/2+170,260,700);
}
fill(212,179,135,[255]);
ellipse(width/2,height/2,180,250); //face
if (mouseIsPressed) //lipstick
fill(219,58,107,[100]);
if (mouseIsPressed) {
ellipse((width/2),(height/2+50),70,30); //lips
} else {
ellipse((width/2),(height/2+50),40,15);
}
if (mouseIsPressed) //blush
fill(255,204,204,[255]);
circle((width/2+40),(height/2),30);
circle((width/2-40),(height/2),30);
fill(0)
strokeWeight(3);
line((width/2-40),(height/2+50),(width/2+40),(height/2+50)); //mouth
fill(255); //eye whites
ellipse((width/2-30),(height/2-40),30,15);
ellipse((width/2+30),(height/2-40),30,15);
fill(75,32,32,[255]);
if (mouseX > width/2) { //pupils dilate
boxWidth=12;
} else {
boxWidth=6;
}
ellipse((width/2-30),(height/2-40),boxWidth,boxWidth); //eye right
ellipse((width/2+30),(height/2-40),boxWidth, boxWidth); //eye left
arc((width/2-30),(height/2-60),30,mouseY,PI,radians(360)); //eyebrow right arches
arc((width/2+30),(height/2-60),30,mouseY,PI,radians(360)); //eyebrow left arches
fill(164,135,106,[255]); //nose
strokeWeight(1);
triangle((width/2-15),(height/2+10),(width/2+15),(height/2+10),(width/2),(height/2-30));
fill(223,193,163,[255]);
strokeWeight(1);
rect((width/2-9),(height/2-30),18,35);
fill(0);
ellipse((width/2-5),(height/2+5),8,5); //nostrails
ellipse((width/2+5),(height/2+5),8,5); //nostrails
}
Kaitlyn Project 2- Section A
While I was creating this, I just thought of the things that could change and added as I went. The first things that came to mind were doing things with the eyes because unfilled they looked a bit creepy. But then I added hair and thought it would be fun to have other things pop up.
Looking Outward-02
I was inspired by Frederik Vanhoutte’s generative art. I’m someone who really loves color and lights so I love how his art incorporates in an illusion of “light” through the use of colors. I think I’m drawn to it because it is interesting how light is really just the use of contrasting colors and also certain shades or colors. He also makes the circle look spherical through the use of perspective even though the shape is really 2D on my computer.
I specifically looked at piece 653 from this archive:
https://winterbloed.be/instagram-archive-2021-part-ii/#&gid=1&pid=653
I don’t know the algorithm that generated the work. However, I suppose he used many thin arcs to create that spherical effect. He also knew how to color the different lines to give it that effect of light. His artistic knowledge is shown in this through that use of persepecitve and the colors he chooses.
This is what the piece looks like
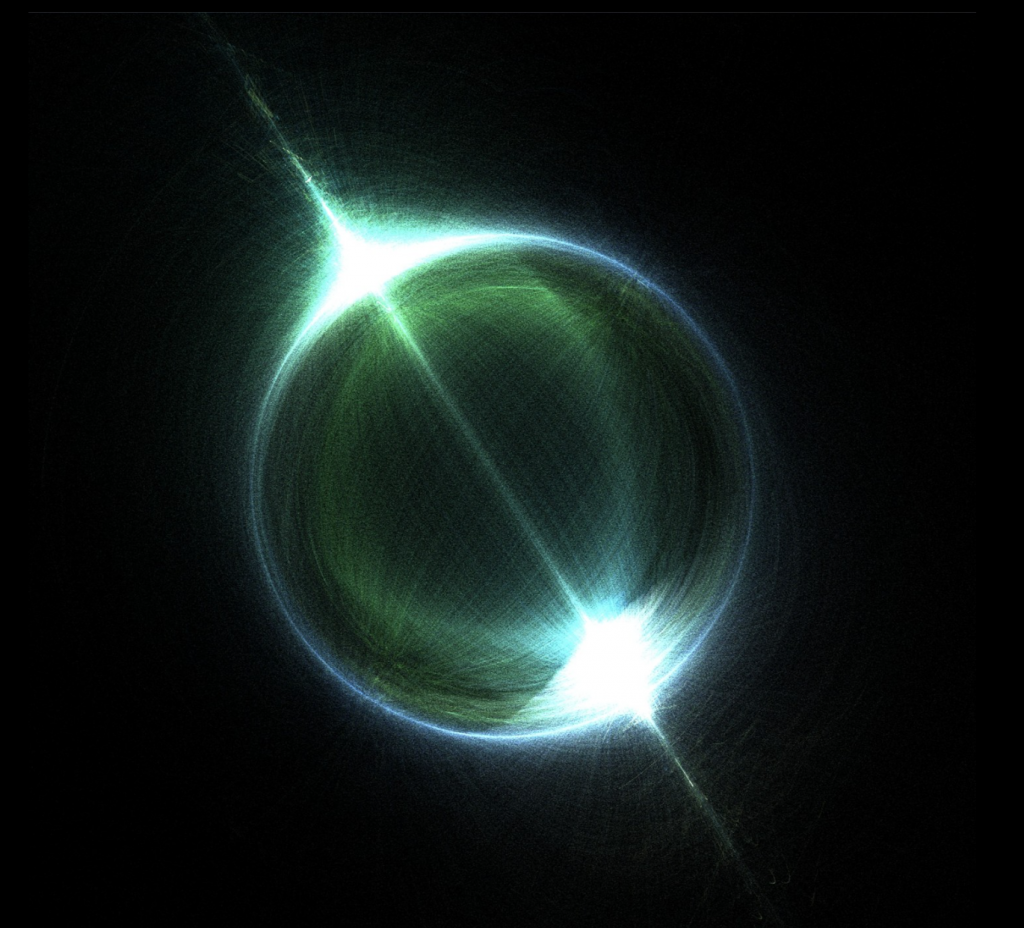
Frederik Vanhoutte, 2021