//Name: Hari Vardhan Sampath
//Section: E
//eMail address: harivars@andrew.cmu.edu
//Project-06
function setup() {
createCanvas(450, 300);
}
function draw() {
var s = map(second(), 0, 60, 0 , width); //runs from left to right
var m = map(minute(), 0, 60, 0 , width); //runs from left to right
var h = map(hour(), 0, 24, height , 0); //runs from bottom to top
var cR;
var cG;
var cB;
// color caliberation for the background w.r.t. hour
if (hour() > 0 & hour() < 6) {
cR = 0;
cG = 50;
cB = 100;
}
else if (hour() > 6 & hour() < 15) {
cR = 50;
cG = 150;
cB = 250;
}
else if (hour() > 15 & hour() < 19) {
cR = 50;
cG = 150;
cB = 250;
}
else if (hour() > 19 & hour() < 24) {
cR = 0;
cG = 25;
cB = 51;
}
background(cR, cG, cB); // cliberated background
// grid for second
for (var xS = 0; xS <= width; xS += width/60) {
stroke('rgba(10, 10, 10, 0.9)');
strokeWeight(1);
line(xS, 200, xS, height);
}
// grid for minute
for (var xM = 0; xM <= width; xM += width/60) {
stroke('rgba(10, 10, 10, 0.9)');
strokeWeight(4);
line(xM, 100, xM, 200);
}
// grid for hour
for (var xH = 0; xH <= width; xH += height/24) {
stroke('rgba(10, 10, 10, 0.9)');
strokeWeight(10);
line(width/2, xH, width - height/2, xH);
}
// second runs along the horizontal grid left to right
push();
stroke(cR, cG, cB);
strokeWeight(2);
line(s, 200, s, height);
pop();
// minuit runs along the horizontal grid from left to right
push();
stroke(cR, cG, cB);
strokeWeight(3);
line(m, 100, m, 200);
pop();
// hour runs along the vertical grid from bottom to top
push();
stroke(cR, cG, cB);
strokeWeight(9);
line(width/2, h, width - height/2, h);
pop();
}
Month: October 2022
Project 06
This is my duck clock
the amount of ducks corresponds to the 24 hours and they spin once a minute and blink once a second
//Evette LaComb
//elacomb@andrew.cmu.edu
// Section D
// Project 06 - Duck Clock
//Concept: grid of 5 x 5, 24 ducks, every hour a new duck swims onto screen.
//each duck rotates once a minute.
//need a function to draw the ducks
//need a loop for the ducks- where do they enter from, where do they go each hour
//need a transformation to roate each duck around its center axis- part of function
// b for bubbles in water:
var b = [];
//angle of roatation:
var angleR = 0;
var direction = 1;
//starting array for x position of ducks;
var stx = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0];
//starting array for y position of ducks;
var sty = [200, 280, 200, 120, 280, 200, 120, 120, 280, 200, 120, 360, 40, 280, 40, 200, 360, 40, 280, 360, 360, 120, 40, 360];
//end position array for position of ducks;
var endx = [200, 120, 280, 200, 200, 120, 280, 120, 280, 40, 360, 200, 280, 40, 360, 360, 120, 200, 360, 40, 280, 40, 120, 360];
var speed = 1;
function setup() {
createCanvas(400, 400);
// object and Array for water texture:
for (i = 0; i < 1000; i ++) {
b[i] = new Object();
b[i].c = random(255);
b[i].x = random(5, width-5);
b[i].y = random(5, height-5);
//speed that water moves:
b[i].dx = random(-0.1, 0.1);
b[i].dy = random(-0.1, 0.1);
}
frameRate(60);
}
function draw() {
background("lightblue");
var h = hour();
//loops to create water texture
for (i = 0; i <= 24; i ++) {
bubble(b[i]);
update_bubble(b[i]);
}
//loops t ocreate ducks corresponding to arrays
for (i = 0; i <= hour() - 1; i ++){
duck(stx[i], sty[i]);
stx[i] += speed;
if(stx[i] >= endx[i]){ stx[i] = endx[i]};
}
//beach details:
push();
rotate(radians(-45));
fill(255, 255, 255, 60);
ellipse(0, 0, 320, 150);
fill(200, 190, 160)
ellipse(0, 0, 300, 130)
pop();
stroke(0);
strokeWeight(2);
//text('Current hour:\n' + h, 5, 50);
}
function duck(stx, sty){
//duck stuff:
//draw duck at coordinate x, y
push();
translate(stx, sty);
//rotate the duck;
rotate(radians(angleR));
angleR += 0.007;
//cosmetics for duck:
noStroke();
fill("lightBlue");
ellipse(0, 0, 80, 70);
fill(230);
ellipse(0, 0, 70, 60);
fill(230);
triangle(10, 30, 10, -30, 45, 0)
fill("orange");
ellipse(-25, 0, 30);
fill("white");
//stuff to make ducks blink according to seconds
ellipse(-10, 0, 40);
var s = second();
if (s % 2 == 0){
fill("black");
}else{
fill("white");
}
ellipse(-15, 17, 5);
ellipse(-15, -17, 5);
pop();
}
function bubble(b){
//cosmetics of water texture:
noFill();
strokeWeight(10);
stroke(255, 255, 255, 20)
ellipse(b.x, b.y, 70, 70);
}
function update_bubble(b){
//tecnical stuff for water texture:
//water movement:
b.x += b.dx;
b.y += b.dy;
//if reach edge of screen change direction:
if ( b.x >= width || b.x < 0){
b.dx = -b.dx;
}
if (b.y >= height || b.y < 0){
b.dy = -b.dy;
}
}
Looking Outward – 06 – aarnavp
//Aarnav Patel
//aarnavp@andrew.cmu.edu
//Section D
//Looking Outwards
One random computational graphics project that really impressed me is the work of Anders Hoff in the piece A Tangle of Webs. While there is definitely the employment of certain algorithms, the start of the piece is created from scattering random vertices around the canvas. The preceding formula then uses a recursive method to track intersections of lines connecting these vertices, ultimately resulting in the organic web-like structure. The resulting form is also inspired by elastic bands, as they model certain interactions between adjacent nodes to create the elastic materiality effect.
What I especially appreciate about this piece is how natural the integration with technology and graphics was undertaken. The potential for this type of piece is even noted for how it can be applied in a 3D sphere. It really connects with some of the projects we do in class, as we can somehow create this generalized algorithms from a basis of random points, alluding to the mathematical concepts underlying randomness.
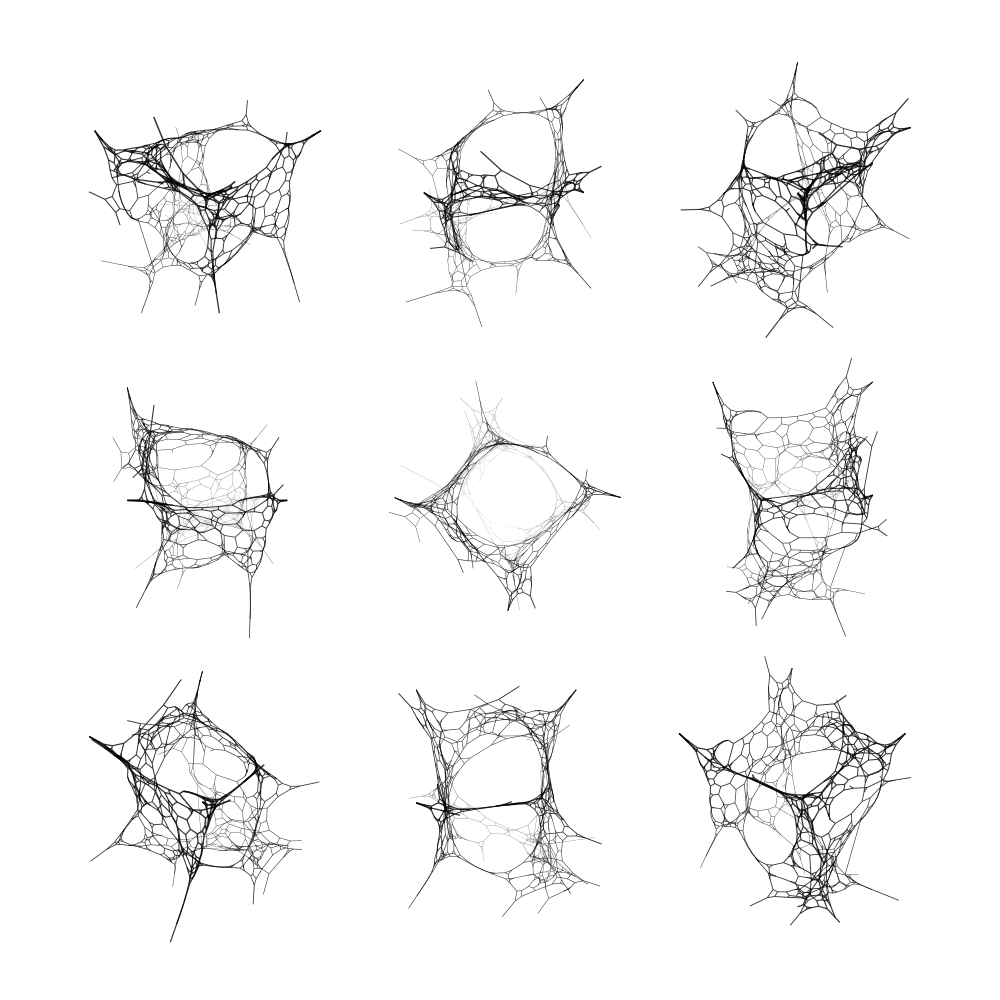
Project-06-aarnavp
For this project, I was inspired by the concept of dendrochronology, which is the study of time depiction through tree rings. Tree rings are indicative not only of the age of the tree, but also the conditions of the climate at the time. I thought this was really interesting, as the from a distance, the time can’t be easily counted, but it depicts more than just time, but place.
The tree ring is comprised of the layered brown/green ring (which denotes 24 layers for 24 hours), and then an underlying minutes and seconds red ring which grows gradually (and doesn’t layer).I also represented days from the moving ellipses in the center of the tree ring, to mimic the idea of celestial bodies.
//Aarnav Patel
//Section D
//aarnavp@andrew.cmu.edu
//Project 06
//Inspired by dendrochronology (how time is depicted through tree rings)
var radius;
var dayX = [];
var dayY = [];
var degree = [];
var dDegree = []
var diam;
function setup() {
createCanvas(480, 480);
let m = month();
//finding the days per month
if ( m == 4 || m == 6 || m == 9 || m == 11) {
diam = width / 30;
} else if (m == 1 || m ==3 || m == 5 || m == 7 || m == 8 || m == 10 || m == 12) {
diam = width / 31;
} else { //february
diam = width / 28;
}
radius = width / 60; //for seconds and minutes, they increment evenly 60 times until reach end of canvas
ringColor = color(random(150, 160), random(105, 155), random(90, 100), 255 / 24); //opacity is 255/24 so that the center of circle wil eventaully be 100% opacity
//random "magic paramters" is for random shade of brown (like tree barks)
for (var i = 0; i < day(); i++) {
append(dayX, diam * i)
append(dayY, 0);
append(degree, 0);
append(dDegree, radians(random(-1, 1)));
}
}
function draw() {
background(255);
translate(width / 2, height / 2); //set new origin at middle of canvas
fill(255, 0, 0, 20);
drawSecond();
fill(255, 0, 0, 20);
drawMinute();
fill(ringColor);
drawHour();
for (var i = 0; i < degree.length; i++) {
fill(255, 255, 255, 70);
ellipse(dayX[i], dayY[i], diam);
rotate(degree[i]);
degree[i] = degree[i] + dDegree[i]; //incrementing degree by specific dDegree
}
}
function drawHour() { //want the hours rings to be static military time (e.g 24:00) and show circles layered
for (var i = 0; i < hour(); i++) { //from 0 to 1 - current hour amount
noStroke();
ellipse(0, 0, width / i); //draws circle proportional to width
}
}
function drawMinute() {
ellipse(0, 0, minute() * radius); //minutes dynamic – increments evenly across canvas
}
function drawSecond() {
secondR = 8;
ellipse(0, 0, second() * radius); //second dynamic – increments evenly across canvas
}
anabelle’s project 06
we always think too much about how time passes by too quickly. don’t be obsessed with aging, and just think about the good that comes with your birthday!
the background indicates to minute (to some extent). at the start of the hour, the background will be pink. it will become increasingly bluer the greater the minute is.
the number of strawberries on the cake indicate the hour of the day in a 12-hour cycle. to distinguish am and pm, the fruit will turn into blueberries in the am and strawberries in the pm.
the number of candles indicates the month and the number of gifts indicates the day.
// kayla anabelle lee (kaylalee)
// kaylalee@andrew.cmu.edu
// section c
// project 6
// define variables
let redValue, blueValue, greenValue;
let strawberryFill;
let giftCounter;
let giftDraw;
function setup() {
createCanvas(480, 480);
let h = hour();
let m = minute();
let d = day();
let mon = month();
// initialize background color
redValue = 248;
blueValue = 226;
greenValue = 226;
giftCounter = 0;
}
function draw() {
// every minute, the background changes by this increment
let redMinute = 1.25;
let blueMinute = 0.633;
let greenMinute = 0.1167;
// final color will be (173, 188, 219)
background(redValue - (redMinute*minute()), blueValue - (blueMinute*minute()), greenValue - (greenMinute*minute())); // final color will be (173, 188, 219)
// set color variables for helper functions
let cakeColor = color(255, 214, 148);
let icingColor = color(246, 158, 178);
let tableColor = color(112, 77, 36);
let candleColor = color(247, 200, 238);
let outerFire = color(245, 154, 142);
let innerFire = color(255, 202, 150);
let giftColor = color(171, 156, 217);
let ribbonColor = color(248, 239, 233);
// draw table and cake
table(150, height - 105, 275, 190, tableColor); // 4/3 ratio
cakeBase(150, height - 160, 150, 75, cakeColor);
cakeBase(150, height - 230, 100, 50, cakeColor);
// the number of candles = month (12 candles total)
for (i = 1; i <= month()%12; i++) {
if (i <= 6) {
candle(i*15 + 96, 205, 8, 32, candleColor); // (top row)
} else {
candle(i*20 - 45, 255, 10, 40, candleColor); // 2/3 ratio (bottom row)
}
}
// the number of gifts = day (31 total)
giftDraw = true;
giftCounter = 0;
for (i = 0; i < 7; i++) {
for (j = 0; j < 5; j++) {
if (giftCounter >= day()) {
giftDraw = false;
} gift(280 + 30*i, 470 - 30*j, 25, 25, giftColor, ribbonColor, giftDraw);
giftCounter += 1;
}
}
print (giftCounter);
// now working on strawberries
// for PM, fill is BLUE. for AM, fill is PINK)
if (hour() <= 12) {
strawberryFill = color(73, 104, 152);
} if (hour() > 12) {
strawberryFill = color(231, 84, 128);
}
// the number of strawberries = hour of the day (12 strawberries total)
for (i = 1; i <= hour()%12; i ++) {
if (i <= 6) {
strawberry(i*16 + 92, 220, 15, 25, strawberryFill); // (top row)
} else {
strawberry(i*22 - 60, 270, 15, 25, strawberryFill); // 2/3 ratio (bottom row)
}
}
textSize(20);
textAlign(CENTER);
text('life is too short to worry about time. eat some cake!', 220, 180, 300, 200);
}
/*my functions*/
function cakeBase(x, y, w, h, color) { // draws the bread part of cake
var r = (w / 6);
noStroke();
fill(color);
// base rectangle
rectMode(CENTER);
rect(x, y, w, h);
// left arc
arc(x - w/2 + r/2, y - h/2, r, r, radians(180), radians(270));
// right arc
arc(x + w/2 - r/2, y - h/2, r, r, radians(270), radians(360));
// connect arcs
line(x - w/2 + r/2, y - 2*r, x + w/2 - r/2, y - 2*r);
rect(x, y - 7*r/4, w - r, r/2);
}
function table(x, y, w, h, color) { // draws table
fill(color);
rectMode(CENTER);
noStroke();
// vertical slabs
rect(x - 3*w/10, y + 3*h/10, w/12, h/2); // left
rect(x + 3*w/10, y + 3*h/10, w/12, h/2); // right
// horizontal slabs
rect(x, y, (3*w)/4, (h/6)); // bottom
rect(x, y - h/12, w, h/12); // top
}
function gift(x, y, w, h, boxColor, ribbonColor, draw) { // draws one present
if (!draw) {
return false;
}
rectMode(CENTER);
noStroke();
fill(boxColor) // use arrays to create different colors for each gift
// rectangles
rect(x, y, 4*w/5, 4*h/5); // bottom
rect(x, y - 2*h/5, w, h/10); // top
// bow
stroke(ribbonColor);
strokeWeight(w/10);
noFill();
circle(x - w/8, y - h/2, w/4);
circle(x + w/8, y - h/2, w/4);
// box ribbon
fill(ribbonColor);
noStroke();
rectMode(CENTER);
rect(x, y, w/5, 8*h/10);
}
function candle(x, y, w, h, color) { // the width to height should be a 1/4 ratio
rectMode(CENTER);
fill(color);
strokeWeight(1);
stroke(150);
rect(x, y, w, 4*h/5);
fill(255, 0, 0, 150); // outer flame
noStroke();
ellipse(x, y - 3*h/5, 4*w/5, 3*h/10);
fill(255, 165, 0, 150);
ellipse(x, y - 11*h/20, 3*w/5, h/5);
}
function strawberry(x, y, w, h, color) { // draws one strawberry
fill(color);
noStroke();
arc(x, y, w, h, radians(180), radians(0));
arc(x, y, w, h/10, radians(0), radians(180));
}
function icing(x, y, w, h, color) {
push();
translate(x, y);
fill(color);
stroke(color);
x += cos(radians(x));
y += sin(radians(y));
point(x, y);
pop();
}
anabelle’s blog 06
One work of random art that I enjoy is the infinite biome generation of Minecraft. Minecraft can create infinte, borderless worlds filled with a plethora of biomes, structures, and landforms. In my opinion, Minecraft’s unlimited ability to generate unique world after unique world is what keeps it at the top of the gaming market. From observation, I think Minecraft uses perlin noise/randomness to generate its biomes — none of the scenery changes feel jarring and the game has an overall organic, natural feeling to it. I just find it really cool that Minecraft is able to randomize SO many elements to bring together an organized, cohesive piece. For example, it doesn’t just randomize whether it’ll generate an ocean biome or desert biome; the biomes themselves have randomly generated elements so no two oceans are alike. Furthermore, Minecraft stores each generated “seed” or world so they can be reaccessed for whoever wants to explore a specific world. Minecraft never lets its audience become bored by constantly adding new, consistently creative randomized events that give the joy of open-world exploration with each play.
Some cool minecraft worlds: https://www.youtube.com/watch?v=gFHj4E_1o6E&ab_channel=Minecraft%26Chill
(Like, can you believe these seeds spawned naturally, rather than a player going in and building the biome themself? It’s so cool!)
Project 06 – Abstract Clock – srauch
Here is my abstract clock. It’s a beach with tides: high tide is at noon and low tide is at midnight. The grass rustles in the breeze, and the beach is strewn with starfish and shells. It’s dark from 8 pm to 5am. Sunrise is from 6am to 7am, and sunset is from 7pm to 8pm.
//Sam Rauch, section B, srauch@andrew.cmu.edu, project 06: abstract clock
//this code creates a beach with a tide. High tide is at noon, and low tide is at midnight.
//the grass rustles in the sea breeze. it gets darker from 7pm 8 pm and lighter from 6 to 7am.
var grasslength = []; //length of blades of grass
var sandspotX = []; //x position of specks in sand
var sandspotY = []; //y position of specks in sand
var waveY; //height of the tide
var waveShift; //amount the water's edge fluctuates by
var daytime; //the time of day in minutes
var darkness; //amount of darkness it is
function setup() {
createCanvas(400, 400);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
//initializing x position of specks in sand
for (var i = 0; i < 50; i++){
sandspotX[i] = random(0, width);
}
//initializing y position of specks in sand
for (var i = 0; i < 50; i++){
sandspotY[i] = random(200, 400);
}
frameRate(5);
//creating y position of tide. High tide is at noon, low tide is at midnight
daytime = hour()*60 + minute(); //time of day, in minutes
if (daytime < 690) { //if before noon
waveY = map(daytime, 0, 690, 400, 200); //before noon, wave gets higher with time
} else { //if after noon
waveY = map(daytime, 690, 1380, 200, 400); //after noon, wave gets lower with time
}
//making it get lighter and darker at sunrise and sunset
if (daytime >= 300 & daytime < 360) { //if from 5 to 6 am, it gets lighter
darkness = map(daytime, 300, 360, 120, 0);
} else if (daytime >= 1140 & daytime < 1200) { //from 7 to 8 pm, it gets darker
darkness = map(daytime, 1140, 1200, 0, 120);
} else if (daytime >= 360 & daytime < 1140) { //in the middle of the day, it's not dark
darkness = 0;
} else { //in the middle of the night, it's dark
darkness = 120;
}
}
function draw() {
noStroke();
background(155, 207, 207);
//row of grass bunches
for (var i = 0; i <2; i++){
for(var j = 0; j<=width; j += 20) {
grass(j, 220);
}
}
//sand
fill(196, 178, 122);
rect(0, 200, width, 200);
//little specks in sand
strokeWeight(3);
stroke(146, 128, 72)
for (var i = 0; i<50; i++){
point(sandspotX[i], sandspotY[i]);
}
noStroke();
//assorted shells and starfish
starfish(250, 250, color(120, 130, 120), 10);
starfish(50, 340, color(50, 74, 64), 50);
starfish(180, 370, color(120, 79, 97), 5);
shell(140, 260, color(60, 50, 70), color(100, 80, 107), 60);
shell(300, 300, color(80, 50, 50), color(120, 80, 97), -5);
//waves
waveShift = randomGaussian(1, 2);
fill(30, 100, 155, 240);
rect(0, waveY+waveShift, width, 300);
fill(30, 100, 155, 100);
rect(0, waveY-(random(1,3))+waveShift, width, 300);
//rectangle that makes darkness and lightness of environment using alpha channel
blendMode(MULTIPLY);
fill(35, 50, 70, darkness);
rect(0,0,width,height);
blendMode(BLEND);
}
//makes starfish
function starfish(x,y, color, spin){
push();
translate(x,y);
rotate(radians(spin));
strokeWeight(4);
stroke(color);
for (var i = 0; i<5; i++){ //draw five arms from 0,0, rotating after each one
line(0,0,0,-10);
rotate(radians(72));
}
noStroke();
pop();
}
//makes seashells
function shell(x,y, color1, color2, spin) {
push();
translate(x,y);
rotate(radians(spin));
strokeWeight(1);
stroke(color1);
fill(color2);
var radius = 7; //radius of seashell, ie distance from circles to center
var size = 12; //size of circles themselves
var angle = radians(0); //theta of circle location
for (var i = 0; i<20; i++) { //draw circles that get smaller as they get closer to the center
ellipse ( cos(angle)*radius, sin(angle)*radius, size);
angle-=radians(24);
size -= i/20; //decrease size of circles
radius -= i/30; //decrease radius of seashell
}
noStroke();
pop();
}
//makes a bunch of grass
function grass(x,y){
push();
translate(x,y);
stroke(65, 100, 52);
strokeWeight(2);
rotate(radians(-40));
for (var i = 0; i < 20; i++){ //creating each blade of grass in a bunch
for (var j = 0; j < 20; j++){ //creating random lengths for each blade of grass
grasslength[j] = random(35, 40);
}
line(0,0,0, -1*grasslength[i]);
rotate(radians(4));
}
pop();
}
Project-06-Abstract-Clock
Clock
//Brody Ploeger
//Section C
//jploeger@andrew.cmu.edu
//Assignment-06
function setup() {
createCanvas(450, 450);
background(220);
//text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
var s = second()
var m = minute()
var h = hour()
background(0)
//hours
for(var n = 0; n<h*height/24; n+=height/24){
noStroke();
fill('orange');
rect(0,0,width,n+20);
}
//hours
for(var n = 0; n<h*height/24; n+=height/24){
noStroke();
fill('red');
circle(width-20,0+n+10,20);
}
//minute counter
for(var j = 0; j<m*7.5; j+=7.5){
strokeWeight(2)
stroke(255)
line(0+j,0,0+j,height)
}
//move origin for second counter
push();
translate(width/2,height/2)
//secondcounter
for(var i = 0; i<s; i++){
rotate(radians(6));
secondcounter(0,0)
}
secondcounter(0,0)
pop();
}
//seconds function
function secondcounter(x,y){
stroke('green');
strokeWeight(10);
noFill();
arc(x,y,width/2,width/2,0,radians(6))
}
Looking Outwards-06
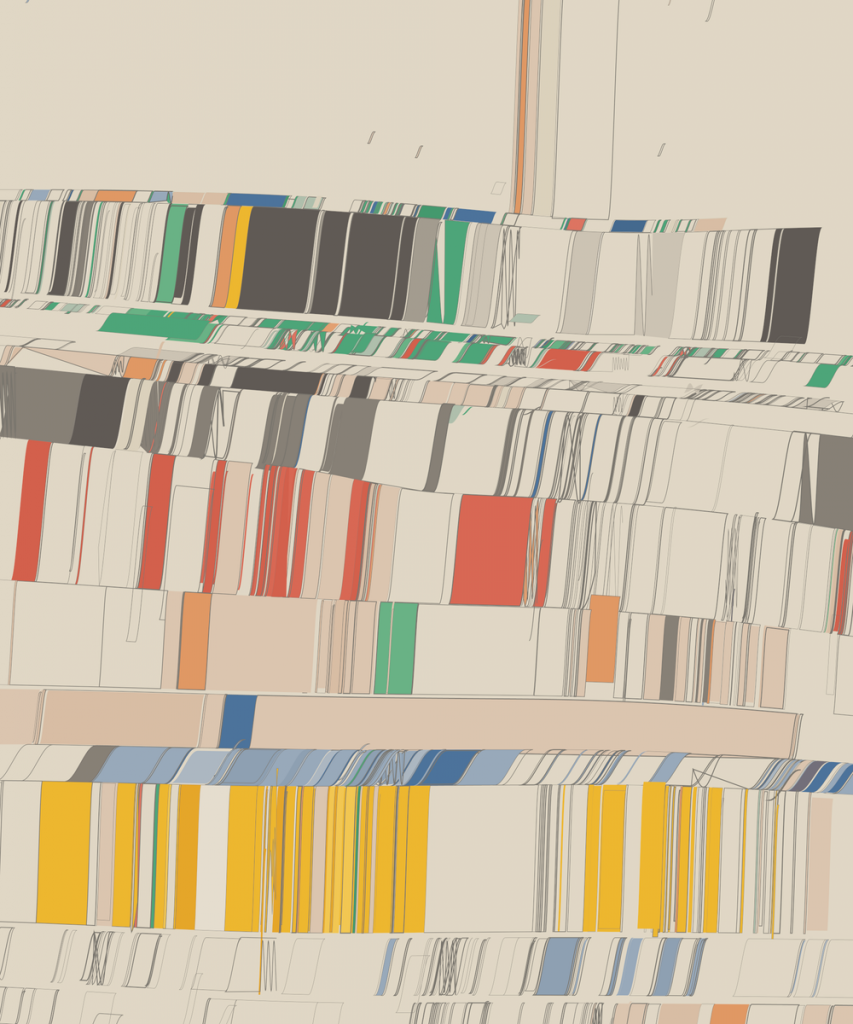
Tyler Hobbs is a visual artist who works with code to create digital drawings which he will also sometimes plot or paint. All of his works use computer algorithms he develops. Within these algorithms, Hobbs programs in an amount of randomness. What I find interesting about Hobbs’s work is the partnership he develops with the computer. When he begins his work, he does not have a final goal in mind, together with the randomness of the program he develops, the artwork becomes a journey. The randomness of the work is not pure randomness, but rather it is refined and becomes more refined as Hobbs works with it.
https://tylerxhobbs.com/process
https://tylerxhobbs.com/works/2022/careless-and-well-intentioned
Project 06 – Abstract Clock
For this project, I thought about the growth of plants as a representation of time. However, I decided to combine that concept by creating a method where by looking at the image, one can know in a more literal sense what time of day it is while still being an abstract representation.
The stem grows a little longer each minute and refreshes from the bottom once it reaches 59. This allows the viewer to know what minute of the hour it is. The number o pedals is representative of the hour and the shade change of the pedals represents the seconds. The background also changes from night to day depending on the typical times when the sun and moon are out.
// Sandy Youssef
// syoussef@andrew.cmu.edu
// section D
//Assignment 6
var x;
var y;
function setup() {
createCanvas(400, 480);
}
function draw() {
x = width/2;
y = 450;
// draw sun or moon depending on time of day where sun or moon are typically out
var h = hour(); // current hour of day 0-23
if ( h > 6 & h < 17) {
background(205,236,255);
//sun
fill("yellow");
ellipse(70, 70, 70);
} else {
background(32,58,131);
// draw moon
fill("white")
ellipse(330,70,70);
fill(32,58,131);
ellipse(310, 70,70);
}
//clouds
var xc = 100; // x cloud
var xy = 100; // y cloud
fill("white");
//left cloud
ellipse(xc + 25, xy +15, 50,25);
ellipse(xc+ 55, xy + 35, 50,33);
ellipse( xc, xy + 35, 60, 40);
ellipse(xc +35, xy + 37, 50, 50);
//right cloud
push();
translate(190,90);
ellipse(xc + 25, xy +15, 50,25);
ellipse(xc+ 55, xy + 35, 50,33);
ellipse( xc, xy + 35, 60, 40);
ellipse(xc +35, xy + 37, 50, 50);
pop();
//dirt
noStroke();
fill("brown");
rect(0, 460, width, height);
//stem
var m = minute(); // current minute in hour 0-59
fill(74,115,69);
rect(x-10,y-10- (m *5), 5, 300); // every minute the stem will grow and once it reaches 59 it will start over.
var s = second(); // current seconds 0-59
var h = hour(); // current hour of day 0-23
for (var i = 0; i < h; i ++) { // loop that creates a new pedal at each hour to count hour
push();
translate(x-10 +3, y-10- (m*5)); // translates pedals to the center of flower
rotate(radians(360/24)*i);
stroke("yellow")
strokeWeight(1);
// count seconds by changing shade of pedals at each second
if (s %2) { // if second is odd make light pink
fill(227,180,242);
} else {
fill(225,154,246); // if second is even make dark pink
}
ellipse(5 , (-15), 10, 25);
pop();
}
// center of flower
fill(243,255,131); // yellow
ellipse(x-10 + 3 , y-10- (m *5) , 20);
// grass
for (var x = 1; x < width; x = x+ 4) {
fill(0,240,0);
triangle(x,480, x+ 5 , 480, x +2.5, 440);
}
}
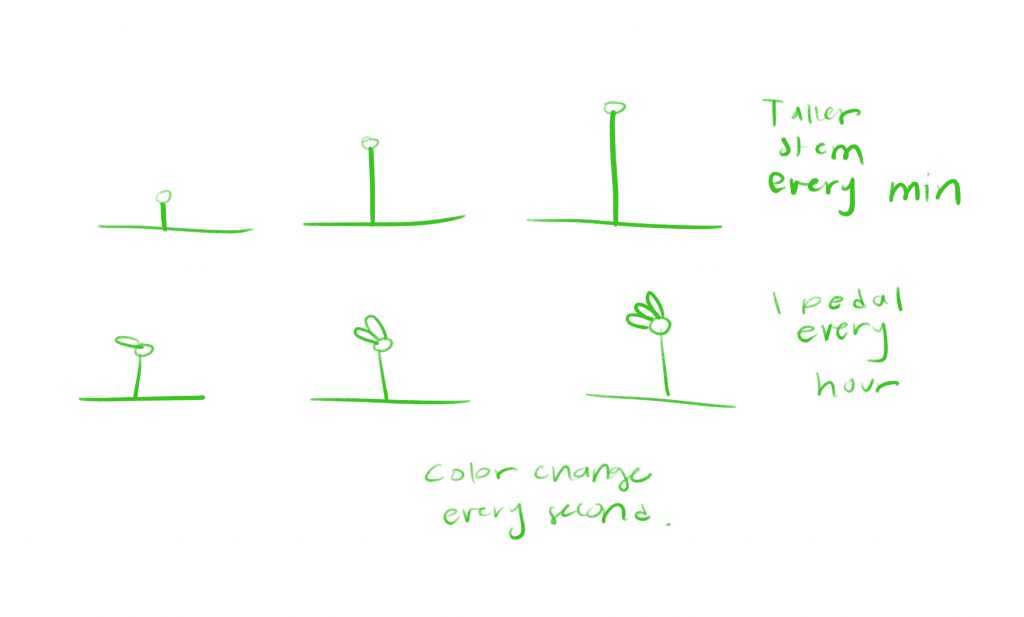
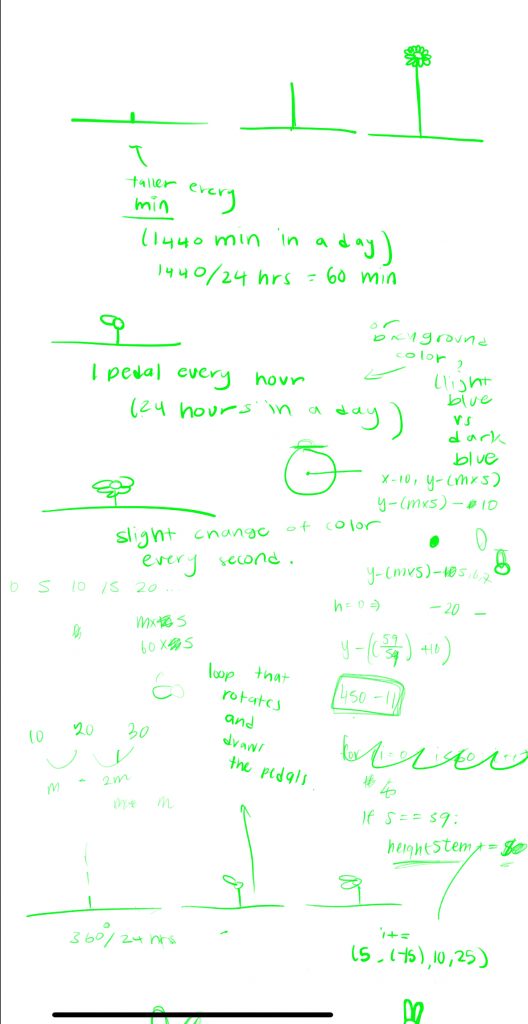