//Keng Pu (Paul) Li
//section A
//9/27/22
var numLines = 50;
var dx3;
var dy3;
var dx4;
var dy4;
function setup() {
createCanvas(500,500);
}
function draw() {
var backG = random(100,200);
background(250,0,20,random(50,backG));
for(var w = 0; w<7; w++){
for(var i = 0; i<18; i++){
push();
scale(0.15);
translate(390+w*525,-350+i*510);
stringArt();
pop();
}
for(var i = 0; i<18; i++){
push();
scale(0.15);
translate(290+w*525,350+i*510);
stringArtFlipped();
pop();
}
}
noLoop();
}
function stringArt(){
//string art like the ones from week 4 but tranformed
//most left
dx3 = 5;
dy3 = 1;
dx4 = 5;
dy4 = 5;
//most left
x3 = 0;
y3 = width/2+100;
x4 = width/2;
y4 = width/2+100;
for(var i = 0; i<40; i++){
strokeWeight(4);
stroke(255,90,180);
line(x3,y3,x4,y4);
x3 += dx3;
y3 += dy3;
x4 -= dx4;
y4 += dy4;
}
push();
translate(0,200);
for(var i = 0; i<40; i++){
strokeWeight(2);
stroke(25,255,100);
line(x3,y3,x4/4,y4/5);
x3 -= dx3;
y3 += dy3;
x4 -= dx4;
y4 += dy4;
}
pop();
}
function stringArtFlipped(){
//string art like the ones from week 4 but tranformed
//most left
dx3 = 5;
dy3 = 1;
dx4 = 5;
dy4 = 5;
//most left
x3 = 0;
y3 = width/2+100;
x4 = width/2;
y4 = width/2+100;
push();
rotate(radians(180));
for(var i = 0; i<40; i++){
strokeWeight(2);
stroke(0,90,180);
line(x3,y3,x4,y4);
x3 += dx3;
y3 += dy3;
x4 -= dx4;
y4 += dy4;
}
push();
translate(0,200);
for(var i = 0; i<40; i++){
strokeWeight(1);
stroke(255,20,10);
line(x3,y3,x4/4,y4/5);
x3 -= dx3;
y3 += dy3;
x4 -= dx4;
y4 += dy4;
}
pop();
pop();
}
Category: SectionA
Project 05
this is my wallpaper:
var x
var y
function setup() {
createCanvas(600, 600);
background(236, 233, 216);
}
function draw() {
//background
backgroundLine();
//blue triangle
for (var y = 50; y < height-20; y += 100) {
for (var x = 50; x < width-20; x += 50) {
BlineTriangle(x, y)
}
}
//pink triangle
for (var y = 50; y < height-20; y += 100) {
for (var x = 50; x < width-20; x += 50) {
PlineTriangle(x, y)
}
}
//brown circles
noStroke();
for (var b = 50; b < height-20; b += 100) {
for (var a = 50; a < width-20; a += 50) {
circle(a, b, 7);
fill(167, 105, 54, 170)
}
}
}
function BlineTriangle(x, y) {
var dist = 4
for (var i = 0; i<=20; i += 2) {
stroke(90, 135, 145);
strokeWeight(0.75)
dist += 4*sqrt(3)/2;
line((x-i), (y+dist), (x+i), (y+dist))
}
}
function PlineTriangle(x, y) {
var dist = 4
for (var i = 0; i<=20; i += 2) {
stroke(232, 163, 147);
strokeWeight(0.75)
dist += 4*sqrt(3)/2;
line((x+i), (y-dist), (x-i), (y-dist))
}
}
function backgroundLine() {
noStroke();
fill(240, 70);
for(var i = 0; i <= 750; i += 50) {
rect(0, i, 750, 10);
}
}
Looking Outwards-05
The project I choose this time is a video on YouTube uploaded by verklagekasper, and it’s part of his Jelly Trip Experience. This video artwork is created by 3D computer art, showing various shape, color, and sound in perpetual jelly motion. While this video is uploaded about 10 years ago, the content is still amazing when I watch it. The colors are futuristic and change with a fluid feeling, which makes people see a glimpse of the color of the universe. And this variety of color changing makes the audience completely attracted by the video’s visual impact. Besides the color, the render makes the surface has transparency and refraction.
Here is a link to the video: https://www.youtube.com/watch?v=btVGCqpIoyk
Project 5: Wallpaper
A wall paper of metacircles!!!
/*
* Andrew J Wang
* ajw2@andrew.cmu.edu
* Section A
* Project-05
*
* This program draws wallpapaer
*/
//set circle sizes
var sizeCircle=50;
function setup() {
createCanvas(600,600);
background(255);
}
function draw() {
//array for the metacircles (both directions)
for (var x=0; x<=width; x+=sizeCircle*2)
{
for (var y=0; y<=height; y+=sizeCircle*2)
{
metaCircle(x,y,sizeCircle);
metaCircle2(x+sizeCircle,y+sizeCircle,sizeCircle);
}
}
//filling cirlces that cover the gap between shapes
for (var x=sizeCircle; x<=width+sizeCircle; x+=sizeCircle*2)
{
for (var y=0; y<=height; y+=sizeCircle*2)
{
//change color of strokes and fills
fill(0);
stroke(0);
strokeWeight(sizeCircle/8);
circle(x,y,sizeCircle/3);
circle(x-sizeCircle,y+sizeCircle,sizeCircle/3);
}
}
//noLoop() to prevent refreshing as the patterns is all ramdom
noLoop();
}
// first type of metacircle
function metaCircle(x,y,size,c) {
//set random values from 0 to 2 to get 3 different colors for the shape
var guess = Math.floor(Math.random()*3);
if (guess==0)
{
fill (255,125,125,255);
}
else if (guess==1)
{
fill (125,255,125,255);
}
else if (guess==2)
{
fill (125,125,255,255);
}
//two circles as base
strokeWeight(0);
circle(x+size/2,y-size/2,size);
circle(x-size/2,y+size/2,size);
//one rotated square fill the middle part
rectMode(CENTER);
push();
translate(x,y);
rotate(1/4*Math.PI)
rect(0,0,size/2,size/2);
pop();
//drawing the outline with 4 arcs
stroke(0);
strokeWeight(size/8);
noFill();
arc(x-size/2, y-size/2, size, size, 0, HALF_PI);
arc(x+size/2, y+size/2, size, size, PI, PI+HALF_PI);
arc(x+size/2, y-size/2, size, size, -PI, HALF_PI);
arc(x-size/2, y+size/2, size, size, 0, PI+HALF_PI);
//patterns
stroke(25);
strokeWeight(size/16);
arc(x-size/2, y+size/2, size/3*2, size/3*2, 0, PI+HALF_PI);
arc(x+size/2, y-size/2, size/3*2, size/3*2, -PI, HALF_PI);
arc(x-size/2, y+size/2, size/3, size/3, 0, PI+HALF_PI);
arc(x+size/2, y-size/2, size/3, size/3, -PI, HALF_PI);
}
//second type of metacircle same as above (no further explanations)
function metaCircle2 (x,y,size,c) {
var guess = Math.floor(Math.random()*3);
if (guess==0)
{
fill (255,125,125,255);
}
else if (guess==1)
{
fill (125,255,125,255);
}
else if (guess==2)
{
fill (125,125,255,255);
}
strokeWeight(0);
circle(x+size/2,y+size/2,size);
circle(x-size/2,y-size/2,size);
rectMode(CENTER);
push();
translate(x,y);
rotate(1/4*Math.PI)
rect(0,0,size/2,size/2);
pop();
stroke(0);
strokeWeight(size/8);
noFill();
arc(x-size/2, y+size/2, size, size, -HALF_PI, 0);
arc(x+size/2, y-size/2, size, size, HALF_PI, PI);
arc(x+size/2, y+size/2, size, size, -HALF_PI, PI);
arc(x-size/2, y-size/2, size, size, HALF_PI, 2*PI);
stroke(220);
strokeWeight(size/16);
arc(x+size/2, y+size/2, size/3*2, size/3*2, -HALF_PI, PI);
arc(x-size/2, y-size/2, size/3*2, size/3*2, HALF_PI, 2*PI);
arc(x+size/2, y+size/2, size/3, size/3, 0, -HALF_PI, PI);
arc(x-size/2, y-size/2, size/3, size/3, HALF_PI, 2*PI);
}
Looking Outwards – 05: 3D Computer Graphics
I love how Santi Zoraidez is able to use 3D computer graphics to render materials of multiple different objects with different textures, creating a surreal visual effect. Also, the collision of the hyper-realistic object, perfectly smooth texture, and the unrealistic physics of the items create a scene that viewers might believe is too real that it is not real at all. The ability to change textures at will and render them with perfect real-life physics (light and shadows), creates such a new type of art style, a style in between realism and surrealism, a purely imaginative discussion of space (artists goal) formed by realistic materials, textures and shapes (3D computer graphics).
Link:
https://www.instagram.com/p/CX_tYgmNwn3/
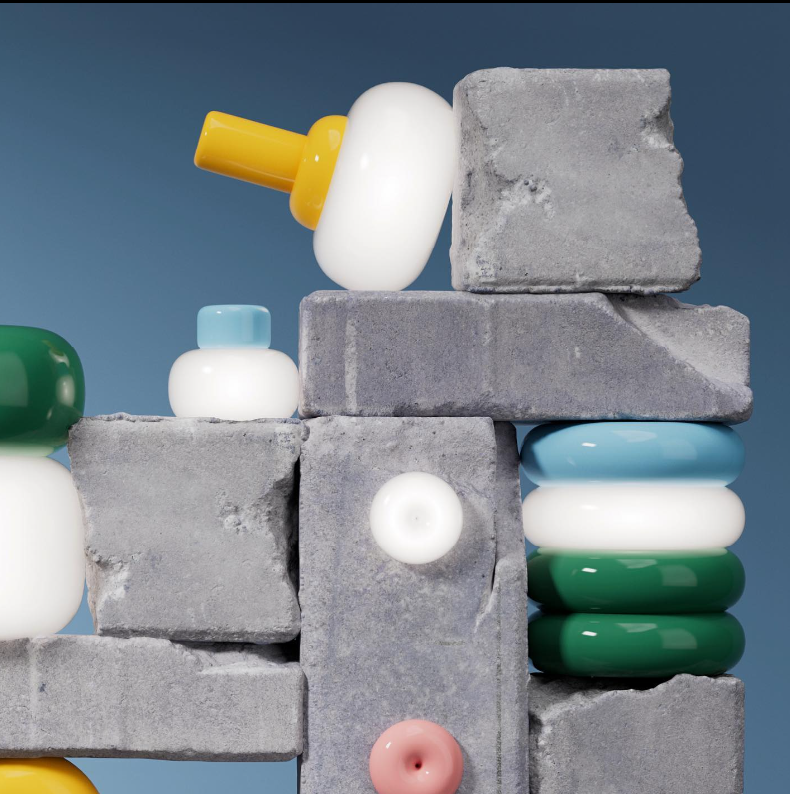
Landscape by Afanassy Pud
Alexia Forsyth
Pud is a Russian contemporary artist. His work has been displayed in over twenty exhibits since 1970. His piece “Landscape” is a three-dimensional tapestry showing a colorful collection of trees and hills. I really admire the traditional aspects of the landscape that are stylized with more modern ideas. Afanasy Pud transforms the common image of trees and hills into a psychedelic visual experience. The artist considers himself a scientist and programmer. He used Corel and Photostyler software to create his pieces. More recently, he favors CorelPhotopaint and FractalDesignPainter as his main source of composition. His artistic sensibilities are demonstrated through the overlaying of colors and odd patterns onto a plain image.
Link: https://digitalartarchive.siggraph.org/artwork/afanassy-pud-landscape/
LookingOutwards-05
In the “House of Gods” by Adam Martinakis, he explores the supernatural balance between heaven and earth through 3D art. I admire the solid shapes and colors, light in juxtaposition with darkness, and Greek mythology influences. Each statue, depicting ancient gods, is crafted very delicately with the emotional intensity of a real marble figure. Adam Martinakis’ work revolves around the intersection of humanity and the unknown- through 3D image rendering, digital sculpture/video, and computer-generated visual media. I appreciate the ambiguity of his art, which produces a wide variety of interpretations. As a member of the Greek Chamber of Fine Arts, and trained in ceramics/architecture in Athens, Martinakis utilizes this expertise in order to craft a new technological perspective on history. In the “House of Gods”, the art combines themes of realism and industrialism (through ladders, stairs), and mythical (unique textures, glowing artifacts, gods.)
Hannah Wyatt
Project 4 – String Art
For this project, I wanted to test the variability of how string art can generate, so I made a random string generator that creates what eventually looks like TV static. Press down the mouse to generate more and more strings until the screen is completely covered!
//Cole Savitz-Vogel
//csavitzv
//Section A
var x1;
var y1;
var x2;
var y2;
var x3;
var y3;
var x4;
var y4;
var x5;
var y5;
var x6;
var y6;
function setup() {
createCanvas(500, 500);
background(0);
}
function draw() {
if (mouseIsPressed){
strokeWeight(.5);
web();
push();
translate(random(0,500),random(0,500));
web();
pop();
push();
translate(random(0,500),random(0,500));
web();
pop();
push();
}
}
function web() {
x3 = random(1, 500);
y3 = random(1, 500);
x4 = random(1, 500);
y4 = random(1, 500);
x5 = random(1, 500);
y5 = random(1, 500);
x6 = random(1, 500);
y6 = random(1, 500);
x1 = x3;
y1 = y3;
x2 = x5;
y2 = y5;
line(x3, y3, x4, y4);
line(x5, y5, x6, y6);
strokeWeight(0.5);
for (var i = 0; i < 51; i+=1) {
var xdelta1 = (x4 - x3);
var ydelta1 = (y4 - y3);
var xdelta2 = (x5 - x6);
var ydelta2 = (y5 - y6);
noFill();
stroke(random(i,200), random(i,200), random(i,200));
arc(x1, y1, x2, y2, random(0,3), random(0,3));
x1 += xdelta1;
y1 += ydelta1;
x2 += xdelta2;
y2 += ydelta2;
}
}
Project 04-String Art
These are my abstract butterflies.
// Natalie Koch
// nataliek
// Section A
// Abstract Butterflies
var numLines = 400;
var c1 = ['pink', 'blue' , 'purple'] //color 1
var c2 = ['blue', 'red', 'yellow'] //color 2
var c3 = ['pink', 'green' , 'orange'] //color 3
var dx1 = 10*(150-50)/numLines;
var dy1 = 10*(300-50)/numLines;
var dx2 = 10*(350-300)/numLines;
var dy2 = 10*(100-300)/numLines;
function setup() {
createCanvas(400, 400);
background(0);
}
function draw() {
function a (x1, y1, x2, y2,dx1, dy1, dx2, dy2, colorList) {
for (var i = 0; i <= numLines; i += 1) {
stroke(random(colorList))
line(x1, y1, x2, y2);
x1 += cos(i)*dx1*15;
y1 += sin(i)*dy1*15;
x2 += cos(i)*dx2*20;
y2 += sin(i)*dy2*20;
}
}
a(50,50,300,300,dx1,dy1,dx2,dy2, c1)
a(50,200,200,375,dx1,dy1,dx2,dy2, c2)
a(150,25,300,200,dx1,dy1,dx2,dy2, c3)
noLoop()
}
Looking Outwards-04
For this project, a work that I found to be very interesting was “Purform – White Box, Audiovisual Performance” from 2010. I was mesmerized by the abstract visualization of sound on the giant screens, the animations smooth and flowing along to the music playing. This is something that I admire because I can recognize how difficult something like this must be to achieve. I personally very much love music, and to see it visualized through waves and other abstract shapes with this technology was very exciting. I’m not quite sure about the algorithms needed, but I would assume that they used trigonometric functions in order to make these kinds of shapes. The artist needed to be able to visualize how they wanted the sound to be portrayed on these screens, because this kind of project could have been interpreted in many different ways. The artists’ vision needed to be specific and they needed to use coding and other technology to make their vision a reality.
Watch the video here.