function setup() {
createCanvas(480, 640);
background(255);
text("p5.js vers 0.9.0 test.", 10, 15);
}
//humans in current form
function draw() {
background(99, 197, 218);
strokeWeight(3)
fill(78, 53, 36, [255])
ellipse(360, 240, 60, 80) //ear right
fill(78, 53, 36, [255])
ellipse(100, 240, 60, 80) //ear left
fill(121, 92, 52, [255])
ellipse(230, 270, 280, 320) //head
fill(255, 255, 255, [255])
ellipse(180, 220, 80, 64) //eye left
fill(255, 255, 255, [255])
ellipse(280, 220, 80, 64) //eye right
fill(78, 53, 36, [255])
ellipse(180, 200, 20, 20) //pupil left
fill(78, 53, 36, [255])
ellipse(280, 200, 20, 20) //pupil right
fill(131, 92, 52, [255])
triangle(230, 250, 200, 320, 260, 320) //nose
fill(184, 63, 63, [255])
arc(220, 260, 400, 200, 1, 2, 2) //mouth
fill(0, 0, 0, [255])
triangle(230, 60, 127, 160, 333, 160) //mohawk
fill(255, 255, 255, [255])
ellipse(378, 259, 7, 7) //pupil right
//monkey (humans pre-evolutionary form)
if (mouseIsPressed) {
earValue1 = random(40,90)
earValue2 = random(50,100)
eyeValue1 = random(20,100)
eyeValue2 = random(40,80)
pupilValue1= random(10,20)
pupulValue2 = random(5,30)
toungeValue = random(40,120)
background(150, 255, 150);
strokeWeight(0)
fill(78, 53, 36, [255])
ellipse(360, 260, earValue1, 80) //ear right
fill(78, 53, 36, [255])
ellipse(100, 200, 60, earValue2) //ear left
fill(121, 92, 52, [255])
ellipse(230, 270, 280, 320) //head
fill(255, 255, 255, [255])
ellipse(180, 200, 100, eyeValue1) //eye left
fill(255, 255, 255, [255])
ellipse(280, 200, eyeValue2, 38) //eye right
fill(78, 53, 36, [255])
ellipse(180, 200, pupilValue1, pupulValue2) //pupil left
fill(78, 53, 36, [255])
ellipse(280, 200, pupulValue2, pupilValue1) //pupil right
fill(131, 92, 52, [255])
triangle(240, 230, 200, 300, 260, 310) //nose
fill(184, 63, 63, [255])
ellipse(194, 402, 64, 100) // tounge
fill(184, 63, 63, [255])
arc(230, 320, 200, 100, 1, 3, 4) //mouth
}
}
Category: Uncategorized
Project 02-Variable-Face
//Sofia Rolla
//Section D
var eyeSize = 20;
var pupilSize= 5;
var faceWidth = 150;
var faceHeight = 200;
var noseWidth = 20;
var noseHeight = 30;
var mouthSize = 40;
var faceR = 199
var faceG = 177
var faceB = 140
var pupilR = 112
var pupilG = 166
var pupilB = 233
var noseR = 200
var noseG = 190
var noseB = 170
var mouthR = 0
var mouthG = 0
var mouthB = 0
function setup() {
createCanvas(640, 480);
}
function draw() {
background(170,10,90);
//head
fill(faceR, faceG, faceB)
ellipse(width / 2, height / 2, faceWidth, faceHeight);
//eyes
fill(255)
var eyeLX = width / 2 - faceWidth * 0.25;
var eyeRX = width / 2 + faceWidth * 0.25;
ellipse(eyeLX, height / 2, eyeSize, eyeSize);
ellipse(eyeRX, height / 2, eyeSize, eyeSize);
//pupils
fill(pupilR, pupilG, pupilB)
ellipse(eyeLX, height / 2, pupilSize, pupilSize);
ellipse(eyeRX, height / 2, pupilSize, pupilSize);
//nose
var noseY = height / 2 + faceHeight* 0.15;
fill(noseR, noseG, noseB)
ellipse(width/2, noseY, noseWidth, noseHeight);
//mouth
fill(mouthR, mouthG, mouthB)
ellipse(width / 2, height / 2 + .4 * faceHeight, mouthSize, faceHeight/10)
//hair
arc(width / 2, height / 2-35, faceWidth+10, faceHeight-10, PI,2*PI, PI);
}
function mousePressed() {
faceWidth = random(75, 150);
faceHeight = random(100, 200);
eyeSize = random(20, 40);
pupilSize = random(5,15);
noseWidth = random(5,20)
noseHeight= random (10,30);
mouthX = random (20, 50);
faceR = random (170,230)
faceG = random (50,200)
faceB = random (50,200)
mouthR = random (50,200)
mouthB = random (50,200)
mouthG = random (50,200)
pupilR = random (50,200)
pupilG = random (50,200)
pupilB = random (50,200)
noseR = random (50,200)
noseG = random (50,200)
noseB = random (50,200)
}
This was a difficult process for me. I struggled with finding the right variables and with setting proper variables for positions of certain facial features. I hope that this process will get easier as I keep learning.
Project 02: Faces and Variables
Within my project, what I wanted to do was to examine what we call faces. What exactly are the processes by which we determine what is a face or not? In such, I attempted to use the most chaotic combinations by randomizing virtually every color combination on screen possible.
var eyeSize = 20;
var faceWidth = 100;
var faceHeight = 150;
var x = 1;
var y = 255;
var z = 2;
var mouth = 1
var hair = 1
function setup() {
createCanvas(300, 300);
}
function draw() {
strokeWeight(3);
stroke(y,x,z);
fill(x,y,z);
background(y - 100, x + 30, z + 5);
ellipse(width / 2, height / 2, faceWidth, faceHeight);
var eyeLX = width / 2 - faceWidth * 0.25;
var eyeRX = width / 2 + faceWidth * 0.25;
fill(x - 80, y - 80, z - 80);
ellipse(eyeLX, height / 2, eyeSize, eyeSize);
ellipse(eyeRX, height / 2, eyeSize, eyeSize);
if (mouth == 1) {
//happy mouth
arc(width / 2, (height / 2) + 25, faceWidth - 40, faceHeight / 2, 0, radians(180));
}
if (mouth == 2) {
//sad face
noFill();
arc(width / 2, (height / 2) + 50, faceWidth - 40, faceHeight / 2, radians(180), 0);
}
if (mouth == 3) {
//shocked mouth
fill(255,102,102);
ellipse(width / 2, (height / 2) + 50, faceHeight / 2, faceWidth / 2);
}
if (hair == 1) {
//party hat
fill(z,x,y);
triangle((width / 2) - (faceWidth / 2), (height / 2) - (faceHeight / 2), width / 2, (height / 4) - 70, (width / 2) + (faceWidth / 2), (height / 2) - (faceHeight / 2));
}
if (hair == 2) {
//beret
fill(0);
ellipse(width / 2, (height / 2) - 70, faceWidth + 20, faceHeight / 2);
}
if (hair == 3) {
//bowl cut
fill(z,y,x + 10);
arc(width / 2, (height / 2) - (faceHeight / 2) + 30, faceHeight - 15,(faceWidth / 2) + 30, radians(180), 0);
rect((width / 2) - (faceWidth / 1.5), (height / 2) - (faceHeight / 2) + 30, faceWidth / 3, faceHeight / 2);
rect((width / 2) + (faceWidth / 3), (height / 2) - (faceHeight / 2) + 30, faceWidth / 3, faceHeight / 2);
}
}
function mousePressed() {
if (mouseIsPressed == true) {
x = random(0,255);
y = random(0,255);
z = random(0,255);
fill(x,y,z);
}
faceWidth = random(75, 150);
faceHeight = random(100, 200);
eyeSize = random(10, 30);
faceColor = random(0,255);
hair += 1;
if (hair > 3) {
hair = 1;
}
mouth += 1;
if (mouth > 3) {
mouth = 1;
}
}
LookingOutwards-02
Climate change is a topic that is seriously important, yet often overlooked despite its devastating impact on every single aspect of living, especially for lower income communities who often contribute the least to the destruction of the planet. My Climate 2050, created by Mitchell Whitelaw in 2018 demonstrates the drastic impact of climate change in a way that is both personal and easy to understand. Dr. Whitelaw utilized a unique program that took in all the data about predicted temperature trends in the near future and used color coordination to match a temperature to a color, creating a glance at a year in Brisbane impacted by climate change. Dr. Whitelaw’s understanding of simplicity and color theory allowed him to create a diagram that was very easy to understand, as he used reds to signify hotter temperatures and greens to signify cooler temperatures. He also utilized the impact of the red circle to represent the cycle by which it will only get worse. This allows the viewer to truly understand just how horrendous the impacts of climate change will be.
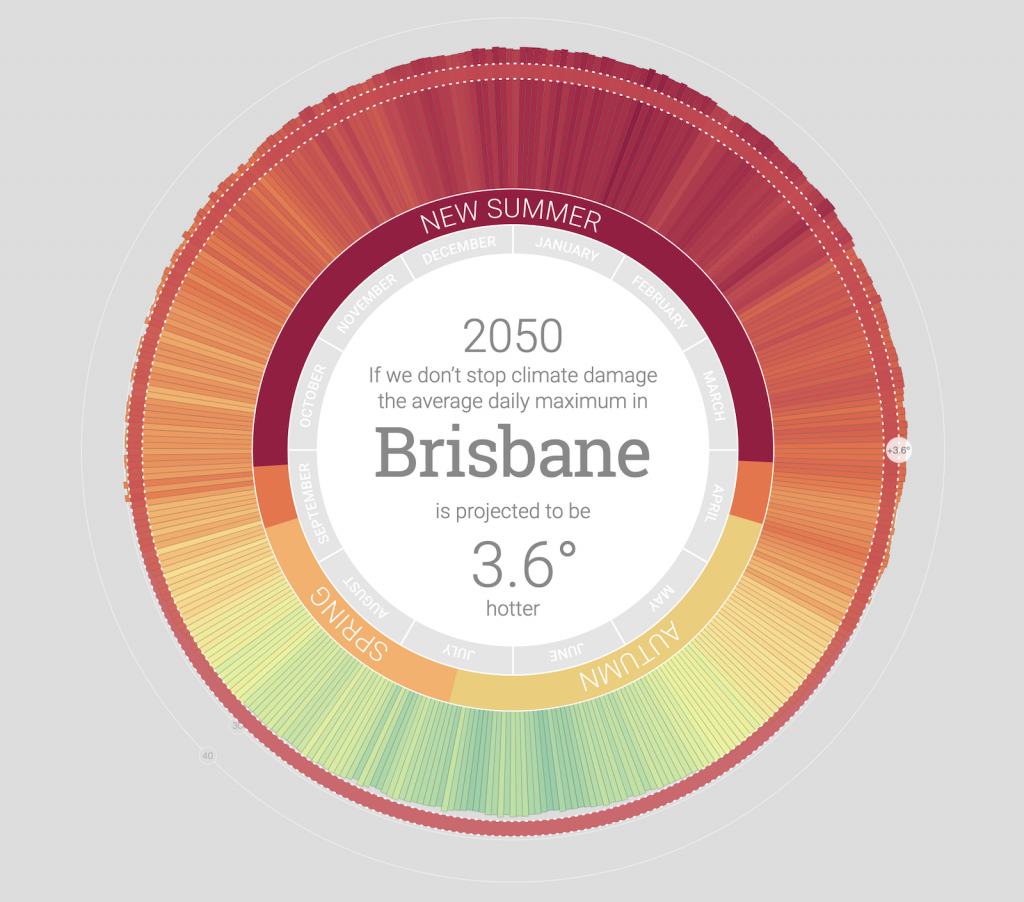
^^My Climate 2050, Mitchell Whitelaw (2018)
Srishty’s Project 2: Variable Face

//Srishty Bhavsar
//Section C
//15-104
// GLOBAL VARIABLES
var headsize = 100;
var eye = 1;
var mouth = 1;
var earring = 1;
var shirtcol = 1;
var hair = 1;
function setup() {
createCanvas(500, 300);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
createCanvas(500,300);
if (mouseY < (width/2)) { // If mouse is in top half of canvas, color is yellow. If in lower half, color is blue
background(252,239,145);
} else {
background(204, 204, 255);
}
strokeWeight(0);
triangle(240,180,260,180,250,195); //mouth
fill(164,116,73); // TAN
rect(240,208,20,40); //neck
//HEAD CHANGE, HAIR CHANGE, EAR CHANGE
if (headsize <= 88 ) {
fill(0);
ellipse(250,280,180,400); // hair
fill(164,116,73); // TAN
rect(240,208,20,40); //neck
ellipse(200,160,15,25); //ear
ellipse(300,160,15,25); //ear 2
//EARRING CHANGE WITHIN IF
if ( earring == 1) {
fill(255); // white
ellipse(300,170,3,10); //earrings
ellipse(200,170,3,10); //earrings
} else if (earring == 2) {
fill(255); //pink
ellipse(300,170,5,5) //earrings
ellipse(200,170,5,5); //earrings
} else {
fill(207,255,229); //mint
rect(297, 170, 3, 8); // rect earrings
rect(200, 170, 3, 8); // rect earrings
}
fill(189,154,122); // skin color
ellipse(250,150,100,120); // head + ears
fill(0);
arc(280, 105, 55, 70, 1, PI + QUARTER_PI, CHORD); //front bang
rect(270,93,10,23); //bangs
rect(260,93,10,23); //bangs
rect(280,101,10,23); //bangs
rect(289,110,9,23); //bangs
triangle(200,140,210,103,260,82); // strand
ellipse(225,133,17,10); // one eye
fill(189,154,122); // SKIN
ellipse(225,135,17,10); // skin carve
} else if (headsize >= 88 & headsize <= 100) {
fill(132, 34, 34); // AUBURN HAIR
ellipse(250,150,130,150); // TIED UP
fill(164,116,73); // TAN
rect(240,208,20,40); //neck
ellipse(290,160,15,25); //ear adjusted slim
ellipse(210,160,15,25); //ear 2 adjusted slim
//EARRING CHANGE WITHIN IF
if ( earring >= 10 & earring <= 20) {
fill(255); // white
ellipse(290,170,3,10); //earrings
ellipse(210,170,3,10); //earrings
} else if (earring >= 20 & earring <= 30) {
fill('pink'); // pink
ellipse(290,170,5,5); // circle earrings
ellipse(210,170,5,5); // circle earrings
} else {
fill(207,255,229); //mint
rect(290, 170, 3, 8); // rect earrings
rect(210, 170, 3, 8); // rect earrings
}
fill(189,154,122); // skin color
ellipse(250,150,75,120); // slim head + ears
fill(132, 34, 34);
arc(280, 105, 55, 70, 1, PI + QUARTER_PI, CHORD); //front bang
rect(270,93,10,23); //bangs
rect(260,93,10,23); //bangs
rect(280,101,10,23); //bangs
rect(289,110,9,23); //bangs
fill(132, 34, 34); // AUBURN EYEBROWS
ellipse(225,133,17,10); // one eyebrow
fill(189,154,122); // SKIN
ellipse(225,135,17,10); // skin carve
} else {
fill(108,25,96); // MAGENTA HAIR
ellipse(250,280,200,400); // hair
fill(164,116,73); // TAN
rect(240,208,20,40); //neck
ellipse(310,160,15,25); //ear adjusted wide
ellipse(190,160,15,25); //ear 2 adjusted wide
fill(63, 20, 20);
ellipse(310,170,3,10); //earrings
ellipse(190,170,3,10); //earrings
fill(189,154,122);
ellipse(250,150,120,120); // widest head + wider hair + ears
fill(108,25,96); // MAGENTA eyebrow
ellipse(225,133,19,10); // one eyebrow
ellipse(275,133,19,10); //second eyebrow
fill(189,154,122); // SKIN
ellipse(225,135,19,10); // skin carve
ellipse(275,135,19,10); //skin carve
fill(108,25,96);
triangle(180,140,210,103,255,80); // hair strand
}
if (eye >= 10 & eye <= 20){
fill(72,60,50); // BROWN
ellipse(225,145,10,10); // one eye
ellipse(275,145,10,10); //second eye
} else if (eye >= 20 & eye <= 30) {
fill(72,60,50); // BROWN
ellipse(225,145,10,10); // one eye
ellipse(275,145,10,10); //second eye
fill(189,154,122); // SKIN
ellipse(225,147,10,10); // one eye SKIN COLOR
ellipse(275,147,10,10); //second eye SKIN COLOR
} else if (eye >= 30 & eye <= 40) {
fill(72,60,50); // BROWN
ellipse(225,145,10,10); // one eye
ellipse(275,146,10,10); //second eye
fill(189,154,122); // SKIN
ellipse(275,148,10,10); //second eye
} else {
fill(72,60,50); // BROWN
ellipse(225,145,10,10); // one eye
ellipse(275,145,10,10); //second eye
}
strokeWeight(0);
fill(0);
stroke(72,60,50); // BLUSH PINK
fill(242,212,215);
ellipse(220,170,10,5); //blush1
ellipse(280,170,10,5); //blush2
strokeWeight(.5);
stroke(101,67,33); // DARK BROWN
line(255,140,255,160); // nose long
line(245,160,255,160); // nose short
strokeWeight(0);
// SHIRT COLOR CHANGE
if (shirtcol >= 10 & shirtcol <= 20) {
fill(79, 121, 66); //DARK GREEN
ellipse(250,330,140,200); //arms
fill(134,169,111);
ellipse(250,360,115,280); // body
} else if (shirtcol >= 20 & shirtcol <= 30) {
fill(172, 112, 136); //DARK GREEN
ellipse(250,330,140,200); //arms
fill(222, 182, 171);
ellipse(250,360,115,280); // body
} else if (shirtcol >= 30 & shirtcol <= 40) {
fill(241, 166, 97); //DARK GREEN
ellipse(250,330,140,200); //arms
fill(255, 216, 169);
ellipse(250,360,115,280); // body
} else if (shirtcol >= 40 & shirtcol <= 50) {
fill(134, 88, 88); //DARK GREEN
ellipse(250,330,140,200); //arms
fill(142, 127, 127);
ellipse(250,360,115,280); // body
} else if (shirtcol >= 50 & shirtcol <= 60) {
fill(110, 133, 183); //DARK GREEN
ellipse(250,330,140,200); //arms
fill(196, 215, 224);
ellipse(250,360,115,280); // body
} else {
fill(79, 121, 66); //DARK GREEN
ellipse(250,330,140,200); //arms
fill(134,169,111);
ellipse(250,360,115,280); // body
}
// MOUTH CHANGE
if (mouth >= 10 & mouth <= 20) {
fill(255,182,193); // pink
triangle(240,180,260,180,250,195); // MOUTH HAPPY
} else if (mouth >= 20 & mouth <= 30) {
fill(203, 76, 78); // light red
ellipse(250,180,10,20); // MOUTH SHOCKED
} else if (mouth >= 30 & mouth <= 40) {
strokeWeight(1);
stroke(0); //BLAXK
line(240,180,260,180) //PLAIN MOUTH
} else {
strokeWeight(1);
stroke(0);
line(240,180,260,180);
}
}
function mousePressed() {
//when the user clicks, the variables of the following features are reassigned to random values within their respective ranges
headsize = random(75,120); // head witdth
eye = random(10,40); // 4 eye types
mouth = random(10,40); // 4 mouth types
earring = random (10,30); // 3 earring types
shirtcol = random(10,60); // 6 color shirts
}
Project 2
// Bridget Doherty
// Section C
var eyeSize = 20;
var faceWidth = 200;
var faceHeight = 300;
var noseWidth = 20
var on = true;
var color1 = 50;
var color2 = 50;
var color3 = 50;
function setup() {
createCanvas(640, 480);
angleMode(DEGREES);
}
function draw() {
let backgroundColor = color(color3, color2, color1);
background(backgroundColor);
let eyeColor = (color('#7d754f'));
let skinColor = (color(255, 220, 177));
let hairColor = (color('#80643d'));
var centerX = width / 2;
var centerY = height / 2;
//neck
fill(skinColor);
rect(centerX-(faceWidth/3), centerY+30, faceWidth/1.5, 200);
// main face
ellipse(centerX, centerY, faceWidth, faceHeight);
// nose
triangle(centerX, centerY+40, centerX, centerY, centerX+noseWidth, centerY+40);
//shirt
let shirtColor = color(color1, color2, color3)
fill(shirtColor);
rect(centerX-(faceWidth/2), centerY+170, faceWidth, 70)
let sleeveColor = color(color1-50, color2-50, color3-50)
fill(sleeveColor);
triangle(centerX-(faceWidth/2), centerY+170, centerX-(faceWidth/2)-30, 480, centerX-(faceWidth/2), 480);
triangle(centerX+(faceWidth/2), centerY+170, centerX+(faceWidth/2)+30, 480, centerX+(faceWidth/2), 480)
// eyes
var eyeLX = width / 2 - faceWidth * 0.25;
var eyeRX = width / 2 + faceWidth * 0.25;
fill('white'); // left eye
ellipse(eyeLX, centerY, eyeSize+20, eyeSize);
fill(eyeColor);
ellipse(eyeLX, centerY, eyeSize, eyeSize);
fill('black')
ellipse(eyeLX, centerY, eyeSize/2, eyeSize/2);
fill('white'); // right eye
ellipse(eyeRX, centerY, eyeSize+20, eyeSize);
fill(eyeColor);
ellipse(eyeRX, centerY, eyeSize, eyeSize);
fill('black')
ellipse(eyeRX, centerY, eyeSize/2, eyeSize/2);
// bowl cut hair
var hairY = (height / 2) - faceHeight/8
fill(hairColor);
arc(centerX, hairY, faceWidth, faceHeight, 180, 0);
// happy or sad mouth
var mouthY = (height / 2) + faceHeight/3.5
stroke(10);
noFill();
if (on == true) {
arc(centerX, mouthY, 80, 60, 0, 180);
} else if (on == false) {
arc(centerX, mouthY, 80, 60, 180, 0);
}
}
function mousePressed() {
faceWidth = random(150, 300);
faceHeight = random(150, 350);
eyeSize = random(10, 30);
noseWidth = random(-40, 40);
if (on == true) {
on = false;
} else {
on = true; }
getRandomColor();
}
function getRandomColor(){
// function generates a random number for color variables
color1 = random(40, 255);
color2 = random(40, 255);
color3 = random(40, 255);
}
Looking Outwards-02
I wrote about a similar piece of art last week, but seeing that this week’s prompt was generative art, I knew I had to find another piece from this form/coding language. Max/MSP is a graphical language-slash-program primarily meant for creation with signal flow, whether that be control signals, audio, or video/image signals. It’s often used in conjunction with Ableton for live music generation as well. The piece that I found on youtube (linked below) is attempting to emulate a generative ambient patch on a Eurorack synth setup. Something that I find really interesting about a lot of computer-generated music or audio pieces is that so much of the technique is trying to emulate analog systems and pieces of equipment. Humans have been used to analog audio since broadcasting began, and even though digital audio techniques are much more efficient and often cheaper, we keep striving towards that analog quality and technique. Even the physical layout of the Max patch is reminiscent of a modular synth setup, and the program itself is designed with ‘patch cables’ as the method of connection between objects, or actions.
Project 2
project 2
var eyeSize = 20;
var faceWidth = 300;
var faceHeight = 250;
var counter = 1;
var hatH = faceHeight/1.5;
var bodyR = 90;
var bodyG = 100;
var bodyB = 90;
var eyeY = 3;
var eyeX = 1;
var faceR = 90;
var faceG = 100;
var faceB = 90;
var hatR = 140;
var normFaceR = 255;
var normFaceG = 220;
function setup() {
createCanvas(480,640);
}
function draw() {
strokeWeight(1);
stroke(0);
background(20,160,190);
if(counter >= 1 & counter < 2){
//body
fill(bodyR,bodyG,bodyB);
circle(width/2,height-50,500);
}
//face
fill(normFaceR, normFaceG, 177);
ellipse(width / 2, height / 2, faceWidth, faceHeight);
var eyeLX = width / 2 - faceWidth * 0.3;
var eyeRX = width / 2 + faceWidth * 0.3;
strokeWeight(3);
fill(250,250,250);
//eye
ellipse(eyeLX, height / 2-10, eyeSize*2, eyeSize-5);
ellipse(eyeRX, height / 2-10, eyeSize*2, eyeSize-5);
fill(70,30,20);
circle(eyeLX, height / 2-10, eyeSize/2, eyeSize/3);
circle(eyeRX, height / 2-10, eyeSize/2, eyeSize/3);
//cowboy
if (counter >= 1 & counter < 2){
noStroke();
fill(hatR,75,0);
rect(width/2-60,height/2-faceHeight/2-hatH,120,hatH)
ellipse(width/2,height/2-faceHeight/2,faceWidth-50,40);
//facial hair
strokeWeight(5);
stroke(140,110,100);
noFill();
curve( width/2-faceWidth/2 + 30, height/2 -100,
width/2-faceWidth/2 + 50, height/2 -50,
width/2-faceWidth/2 + 80, height/2 -50,
width/2-faceWidth/2 + 110, height/2 -30);
curve( width/2+faceWidth/2 + 30, height/2 -100,
width/2+faceWidth/2 - 50, height/2 -50,
width/2+faceWidth/2 - 80, height/2 -50,
width/2+faceWidth/2 - 110, height/2 -30);
stroke(200,20,30);
fill(200,20,30);
//mouth
curve(width/2-faceWidth/2 + 110,height/2-70
,width/2-50,height/2+30
,width/2+50,height/2+30,
width/2+faceWidth/2 ,height/2-30);
//arms
fill(20);
stroke(10);
strokeWeight(5);
curve(width/2 - 150, height/2+100,
width/2-200,height/2+150,
width/2-100,height-20,
width/2-40, height-50);
curve(width/2 + 150, height/2+100,
width/2+200,height/2+150,
width/2+100,height-20,
width/2+40, height-50);
}
//alien
if (counter >= 2 & counter < 3){
strokeWeight(8);
stroke(50,210,150);
line(width/2,height/2-faceHeight/2, width/2, faceHeight/10+30);
fill(mouseX,mouseY,mouseX);
ellipse(width/2, faceHeight/10+30, faceWidth/5, faceHeight/3);
fill(faceR,faceG,faceB);
ellipse(width / 2, height / 2, faceWidth, faceHeight);
//eye
fill(0,50,10);
noStroke();
ellipse(eyeLX+30, height / 2-10, eyeSize*eyeX, eyeSize*eyeY);
ellipse(eyeRX-30, height / 2-10, eyeSize*eyeY, eyeSize*eyeX);
fill(0);
ellipse(eyeLX+30, height / 2-10, eyeSize*eyeX-5, eyeSize*eyeY-5);
ellipse(eyeRX-30, height / 2-10, eyeSize*eyeY-10, eyeSize*eyeX-10);
//body
stroke(0);
strokeWeight(1);
fill(0,faceG-80,faceB+50);
triangle(150,height/2+150,width-150,height/2+150,width/2,height-20);
//arms
fill(0,200,0);
stroke(0,200,0);
strokeWeight(1);
curve(width/2 - 60, height/2+100,
width/2-200,height/2+150,
width/2-30,height-80,
width/2-40, height-50);
line(width/2-30,height-80,width/2-50,height-60);
curve(width/2 + 60, height/2+100,
width/2+200,height/2+150,
width/2+30,height-80,
width/2+40, height-50);
line(width/2+30,height-80,width/2+50,height-60);
//mouth
fill(20,200,200);
strokeWeight(2);
stroke(30,100,500);
rect(width/2-faceWidth/6,height/2+faceHeight/6,faceWidth/3,faceHeight/20);
}
//crazy
if(counter < 1){
background(200,50,50);
fill(200,50,90);
ellipse(width / 2, height / 2, faceWidth+random(20,50), faceHeight+random(20,50));
fill(255);
//body
stroke(0);
strokeWeight(1);
fill(0,faceG-80,faceB+50);
//
fill(255);
//eye
ellipse(width / 2, height / 2, faceWidth, faceHeight);
ellipse(eyeLX+30, height / 2-10, random(30,50),random(40,80));
ellipse(eyeRX-30, height / 2-10, random(30,50),random(20,80));
fill(random(10,80),random(100,255),random(150,255));
ellipse(eyeLX+30, height / 2-10, random(30,50)-10,random(40,80)-10);
fill(random(150,255),random(10,80),random(150,205));
ellipse(eyeRX-30, height / 2-10, random(30,50)-10,random(20,80)-10);
fill(random(30));
ellipse(eyeLX+30, height / 2-10, 10,10);
ellipse(eyeRX-30, height / 2-10, 10,10);
//brows
line(width/2-80,height/2-random(60,100),width/2-random(20,40),height/2-20);
line(width/2-80,height/2-random(60,100),width/2-random(20,40),height/2-20);
line(width/2+80,height/2-random(60,100),width/2+random(20,40),height/2-20);
line(width/2+80,height/2-random(60,90),width/2+random(10,50),height/2-20);
//nose
fill(random(100,255),50,50);
ellipse(width/2-10,height/2+30,10,20);
ellipse(width/2+10,height/2+30,10,20);
//mouth
curve(width/2-faceWidth/2 + random(90,120),height/2+100
,width/2-50,height/2+random(60,80)
,width/2+50,height/2+random(60,70),
width/2+faceWidth/2 ,height/2+random(90,120));
//body
fill(20,20,20);
triangle(width/2,height/2+130, random(50,100),random(height-50,height),width-random(50,100),random(height-50,height));
//arms
curve(width/2 - 150, height/2-100,
width/2-random(190,210),height/2-150,
width/2-100,height-30,
width/2-40, height-50);
curve(width/2 + 150, height/2-100,
width/2+200,height/2-150,
width/2+random(90,110),height-30,
width/2+40, height-50);
}
}
function mousePressed() {
// when the user clicks, these variables are reassigned
// to random values within specified ranges. For example,
// 'faceWidth' gets a random value between 75 and 150.
faceWidth = random(300, 400);
faceHeight = random(190,270);
eyeSize = random(15, 30);
counter = random(0,3);
bodyR = random(100,110);
bodyG = random(100,160);
bodyB = random(30,150);
faceR = random(20,80);
faceG = random(100,250);
faceB = random(100,110);
eyeY = random(1,6);
eyeX = random(0.5,2);
hatR = random(100,255);
normFaceR = random(210,255);
normFaceG = random(190,220);
}
Project – 02 Variable Face
Project 2 – Variable Face
Inspired by the popular Sumikk Gurashi characters from Japanese company San-X, this project explores the variable faces of cute 2D characters in the context of a simple text-based meme format. The occurrence of each feature (ie. body color, lack/ type of ear, mouth, whiskers, arm position, text) are determined by their own unique variables, allowing for a wide scope of character generation.
// Tsz Wing Clover Chau
// Section E
function setup() {
createCanvas(640, 480);
background(220);
}
var counter = 0;
var ears = false;
var earsUp = false;
var r = 0;
var rArms = 0;
var rEars = 0;
var rMouth = 0;
var rWhiskers = 0;
var rText = 0;
let c = [255, 255, 255];
let t = ["Is for me?", "A positive weed", "Am i even\na penguin?", "Made of 1% meat, \n99% fat"];
function mouseClicked(){
r = random([0,1,2,3]);
rEars = random([0,1,2,3]);
rArms = random([0,1,2,3]);
rMouth = random([0,1,2,3]);
rWhiskers = random([0,1,2,3]);
rText = random([0,1,2,3]);
print("%s", r);
counter += 1;
if (counter > 3){ // wrapping bounds
counter = 0;
}
if (r%2 == 0){
ears = true;
} else {
ears = false;
}
if (r == 2) {
whiskers = true;
} else {
whiskers = false;
}
}
function draw() {
background(220);
scale(1.45 *0.93);
ellipseMode(CENTER);
rectMode(CENTER);
var cX = width/2;
var cY = height/2;
var sizeX = width/3;
var sizeY = width/3.75;
if (r == 0){
c = [255, 255, 255]; // shirokuma
} else if (r == 1){
c = [202, 240, 248]; // tokage
} else if (r == 2){
c = [254, 250, 224]; // neko
} else {
c = [217, 224, 163]; // penguin
}
var offsetX = width/35;
var offsetY = height/10;
//BODY
fill(c);
rect(cX + offsetX, cY + (offsetY*2), sizeX - offsetX, height - (cY + offsetY), 70);
ellipse(cX, cY, sizeX, sizeY);
noStroke();
rect(cX + offsetX, cY + (offsetY*2)-2, sizeX - offsetX-2, height - (cY + offsetY)-5, 70);
stroke(0);
strokeWeight(3);
beginShape();
curveVertex(cX - sizeX/1.75, cY - sizeY*0.9); //1st control point
curveVertex(cX + sizeX/2.5, cY - offsetY*1.07);
curveVertex(cX + sizeX/1.5, cY + (offsetY*2));
curveVertex(cX + sizeX/3.75, height - offsetY*1.02);
curveVertex(cX - sizeX/1.75, cY + sizeY*0.7); //2nd control point
endShape();
//ARMS
if (rArms == 0){
arc(cX, cY + offsetY*1.5, offsetY, offsetX*1.2, PI / 2, 3 * PI / 2, OPEN);
arc(cX - offsetY, cY + offsetY*1.5, offsetY, offsetX*1.2, 3*PI / 2, PI / 2, OPEN);
} else if (rArms %3 == 0){
arc(cX - offsetY, cY + offsetY*1.5, offsetY, offsetX*1.2, 3*PI / 2, PI / 2, OPEN);
} else if (rArms == 1){
arc(cX, cY + offsetY*1.5, offsetY, offsetX*1.2, PI / 2, 3 * PI / 2, OPEN);
} else {
arc(cX, cY + offsetY*1.5, offsetY, offsetX*1.2, PI / 2, 3*PI / 2, OPEN);
arc(cX - offsetX*4.25, cY + offsetY*1.5, offsetY, offsetX*1.2, PI / 2, 3*PI / 2, OPEN);
}
//EYES
push();
fill(0,0,0);
ellipse(cX - offsetY*1.2, cY - offsetY/6, offsetX/2, offsetX/3);
ellipse(cX + offsetY*0.2, cY - offsetY/6, offsetX/2, offsetX/3);
pop();
//NOSE / MOUTH
push();
if (rMouth %3 == 0){ // beak
fill(255, 220, 116);
ellipse(cX - offsetY/2, cY+offsetX - offsetY/6, offsetY/1.5, offsetX);
line(cX - offsetY/1.25, cY + offsetY/5, cX - offsetY/5, cY + offsetY/5);
} else if (rMouth %2 == 0) { // snout
fill(255);
strokeWeight(2);
rect(cX - offsetY/2, cY+offsetX*1.15, offsetY*0.85, offsetX*1.4, 120);
fill(0);
ellipse(cX - offsetY/2, cY+offsetX - offsetY/6, offsetY/3, offsetX/1.75);
} else {
fill(0);
ellipse(cX - offsetY/2, cY+offsetX - offsetY/6, offsetY/3, offsetX/1.75);
}
pop();
//EARS
fill(c);
if (rEars %2 == 0){ // bear
push();
rotate(radians(15));
arc(cX + offsetY*1.8, cY - sizeY, offsetY*1.1, offsetY, -PI*0.95, PI*0.05, OPEN);
fill(252, 213, 206);
ellipse(cX + offsetY*1.8, cY - sizeY*1.02, offsetY*0.45, offsetY/4);
pop();
push();
rotate(radians(-20));
arc(cX - sizeX/1.5, cY + offsetX/1.75, offsetY*1.1, offsetY, -PI*1.1, -PI*0.03, OPEN);
fill(252, 213, 206);
ellipse(cX - sizeX/1.5, cY + offsetX/2.2, offsetY*0.45, offsetY/4);
pop();
} else if (rEars%3 == 0){ // cat
push();
rotate(radians(-20));
arc(cX - sizeX*0.85, cY + offsetX*1.5, offsetY*1.35, offsetY*1.15, -PI*0.7, -PI*0.1, PIE);
noStroke();
arc(cX - sizeX*0.837, cY + offsetX*1.6, offsetY*1.25, offsetY*1.05, -PI*0.7, -PI*0.1, PIE);
stroke(0);
arc(cX - sizeX*0.25, cY + offsetX*3.85, offsetY*1.35, offsetY*1.15, -PI*0.7, -PI*0.1, PIE);
noStroke();
arc(cX - sizeX*0.235, cY + offsetX*3.95, offsetY*1.25, offsetY*1.05, -PI*0.7, -PI*0.1, PIE);
pop();
}
//WHISKERS
if (rWhiskers %3 == 0 ){
line(cX - offsetY*2.4, cY, cX - offsetX*5.5, cY + offsetY*0.05);
line(cX - offsetY*2.4, cY + offsetX/2, cX - offsetX*5.5, cY + offsetX/2 - offsetY*0.05);
line(cX + offsetY, cY, cX + offsetX*2, cY + offsetY*0.05);
line(cX + offsetY, cY + offsetX/2, cX + offsetX*2, cY + offsetX/2 - offsetY*0.05);
}
push();
textSize(18);
noStroke();
fill(255,255, 255);
text(t[rText], width/14, height/2);
pop();
}