A. 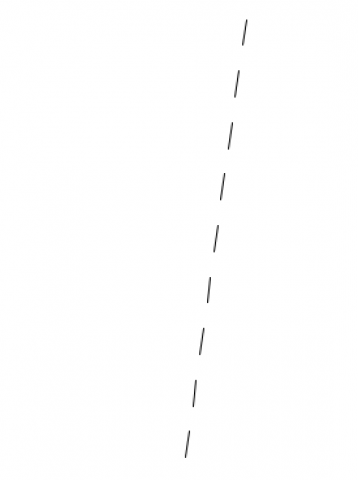
/* Basically I used the example code from the lerp() page on Processing
* but changed it so it would produce dotted lines for any random
* pair of vectors
* */
float dashed_length = 20;
void setup(){
size(400, 400);
background(100);
}
void draw(){
background(255);
int x1 = int(random(0,width));
int x2 = int(random(0,width));
int y1 = int(random(0,height));
int y2 = int(random(0,height));
float ydif = y2-y1;
float xdif = x2-x1;
float num_lines = sqrt(pow(ydif,2) + pow(xdif,2))/dashed_length;
print(num_lines);
for (int i = 0; i <= int(num_lines); i+=2) {
float dx1 = lerp(x1, x2, i/num_lines) + num_lines;
float dx2 = lerp(x1, x2, (i+1)/num_lines) + num_lines;
float dy1 = lerp(y1, y2, i/num_lines);
float dy2 = lerp(y1, y2, (i+1)/num_lines);
line(dx1, dy1, dx2, dy2);
}
noLoop();
}
B. 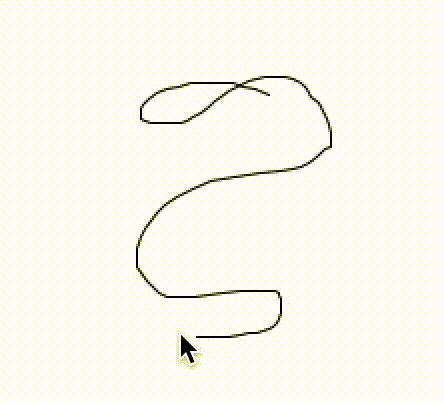
C.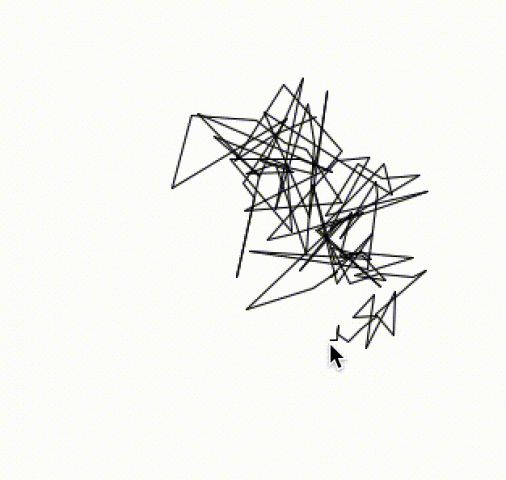
/**
* I basically altered the brownian motion tutorial from Processing
* to record mouseX, mouseY instead of random movement.
*/
int num = 100;
int range = 6;
float[] ax = new float[num];
float[] ay = new float[num];
float[] dx = new float[num];
float[] dy = new float[num];
// float[][] a = new float[num][2]; // LIVING LINE 2D ARRAY
// PVector[] a = new PVector[num]; // LIVING LINE PVECTORS
void setup()
{
size(640, 360);
for(int i = 0; i < num; i++) {
ax[i] = width/2;
ay[i] = height/2;
dx[i] = 0;
dy[i] = 0;
}
/* LIVING LINE PVECTORS
for(int i = 0; i < num; i++) {
a[i] = new PVector();
a[i].x = width/2;
a[i].y = height/2;
}
*/
/* LIVING LINE 2D ARRAY
for(int i = 0; i < num; i++){
for(int j = 0; j < 2; j++){
a[i][j] = width/2;
}
}
*/
frameRate(30);
}
void draw()
{
background(255);
// Shift all elements 1 place to the left
for(int i = 1; i < num; i++) {
dx[i-1] = dx[i];
dy[i-1] = dy[i];
ax[i-1] = ax[i];// + random(-range, range); SPICY LINE COMMENT
ay[i-1] = ay[i];// + random(-range, range); SPICY LINE COMMENT
}
/* LIVING LINE PVECTORS
for(int i = 1; i < num; i++) {
a[i-1].x = a[i].x;
a[i-1].y = a[i].y;
}
*/
/* // LIVING LINE 2D ARRAY
for(int i = 1; i < num; i++){
a[i-1][0] = a[i][0];
a[i-1][1] = a[i][1];
}
*/
// Put a new value at the end of the array
ax[num-1] = mouseX;
ay[num-1] = mouseY;
dx[num-1] = (ax[num-1] - ax[num-2]);
dy[num-1] = (ay[num-1] - ay[num-2]);
// a[num-1][0] = mouseX; // LIVING LINE 2D ARRAY
// a[num-1][1] = mouseY; // LIVING LINE 2D ARRAY
// a[num-1].x = mouseX; // LIVING LINE PVECTORS
// a[num-1].y = mouseY; // LIVING LINE PVECTORS
// Constrain all points to the screen
//ax[num-1] = constrain(ax[num-1], 0, width);
//ay[num-1] = constrain(ay[num-1], 0, height);
// Draw a line connecting the points
for(int i=1; i<num; i++) {
line(ax[i-1], ay[i-1], ax[i], ay[i]);
// line(a[i-1].x, a[i-1].y, a[i].x, a[i].y); // LIVING LINE PVECTORS
// line(a[i-1][0],a[i-1][1],a[i][0],a[i][1]); // LIVING LINE 2D ARRAY
}
}
D.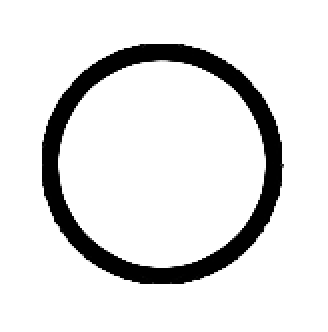
E. 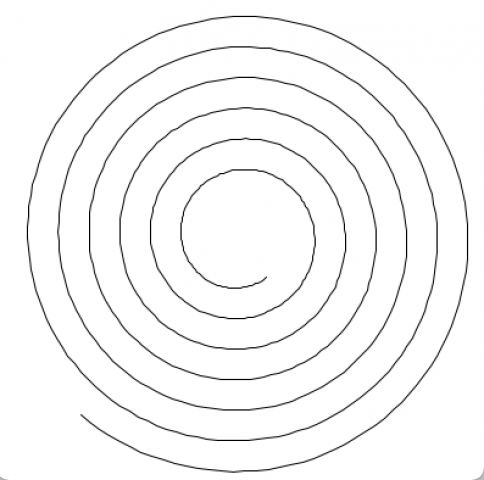
float r = 40;
void setup(){
size(400, 400);
background(100);
}
void draw(){
background(255);
translate(width/2, height/2);
//trig_circle();
trig_spiral();
noLoop();
}
void trig_spiral(){
beginShape();
for(float i = 1; i < 40; i+=0.05){
vertex(cos(i)*(r), sin(i)*(r));
r+=0.2;
}
endShape();
}
void trig_circle(){
beginShape();
for(float i = 1; i < 360; i+=1){
vertex(cos(i)*(r), sin(i)*(r));
}
endShape();
}
F. I got tired of math so I made a shitty offset curve – not parallel, just the normal vector of the slope
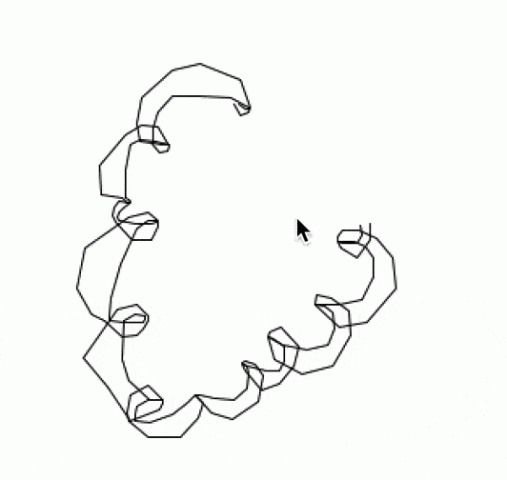
int num = 100;
int range = 6;
float[] ax = new float[num];
float[] ay = new float[num];
float[] dx = new float[num];
float[] dy = new float[num];
float[] ox = new float[num];
float[] oy = new float[num];
void setup()
{
size(640, 360);
for(int i = 0; i < num; i++) {
ax[i] = width/2;
ay[i] = height/2;
dx[i] = 0;
dy[i] = 0;
ox[i] = width/2;
oy[i] = height/2;
}
frameRate(30);
}
void draw()
{
background(255);
// Shift all elements 1 place to the left
for(int i = 1; i < num; i++) {
dx[i-1] = dx[i];
dy[i-1] = dy[i];
ax[i-1] = ax[i];
ay[i-1] = ay[i];
ox[i-1] = ox[i];
oy[i-1] = oy[i];
}
// Put a new value at the end of the array
ax[num-1] = mouseX;
ay[num-1] = mouseY;
dx[num-1] = (ax[num-1] - ax[num-2]);
dy[num-1] = (ay[num-1] - ay[num-2]);
//dksjfldkjslkdfjlsdj i hate this
//ox[num-1] = 25/(1+pow((ay[num-1] + dx[num-1])/(ax[num-1] - dy[num-1]),2));
//oy[num-1] = sqrt(25 - pow(ox[num-1],2));
ox[num-1] = ax[num-1] - dy[num-1];
oy[num-1] = ay[num-1] + dx[num-1];
// Draw a line connecting the points
for(int i=1; i<num; i++) {
//float val = float(i)/num * 204.0 + 51;
//stroke(val);
line(ax[i-1], ay[i-1], ax[i], ay[i]);
//float dx = 25/(1+pow(ay[num-1] + dx[num-1]/ax[num-1] - dy[num-1]),2));
//float dy = sqrt(25 - pow(dx,2));
line(ox[i-1], oy[i-1], ox[i], oy[i]);
}
}
G.
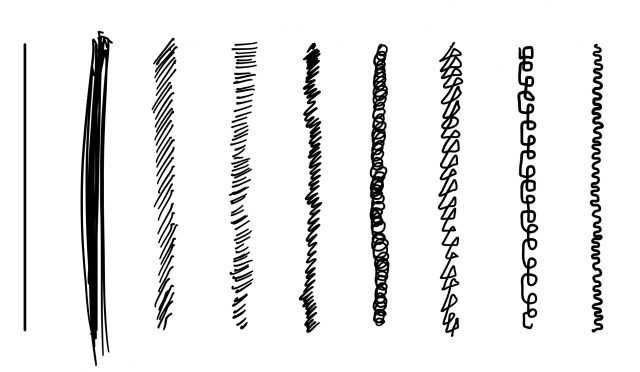

i was watching a lot of gordon ramsey
H.

“bears beets battlestar galactica”
import processing.svg.*;
int num = 1000;
int range = 6;
boolean record = false;
float[] ax = new float[num];
float[] ay = new float[num];
float[] dx = new float[num];
float[] dy = new float[num];
float[] ox = new float[num];
float[] oy = new float[num];
int num_lines = 5;
void setup()
{
size(640, 360);
for(int i = 0; i < num; i++) {
ax[i] = width/2;
ay[i] = height/2;
dx[i] = 0;
dy[i] = 0;
ox[i] = width/2;
oy[i] = height/2;
}
frameRate(30);
}
void draw()
{
background(255);
// Shift all elements 1 place to the left
for(int i = 1; i < num; i++) {
dx[i-1] = dx[i];
dy[i-1] = dy[i];
ax[i-1] = ax[i];
ay[i-1] = ay[i];
ox[i-1] = ox[i];
oy[i-1] = oy[i];
}
// Put a new value at the end of the array
ax[num-1] = mouseX;
ay[num-1] = mouseY;
dx[num-1] = (ax[num-1] - ax[num-2]);
dy[num-1] = (ay[num-1] - ay[num-2]);
// Draw a line connecting the points
if(record){
int now = millis();
randomSeed(now);
beginRecord(SVG, "foo_" + now + ".svg");
}
for(int i=1; i<num; i++) {
float mult = map(dx[i] + dy[i], 0, width+height, 1, 60);
for(int j = 0; j < num_lines; j++){
line(ax[i-1] - j*mult, ay[i-1], ax[i] - j*mult, ay[i]);
}
}
if(record){
endRecord();
record = false;
}
}
void keyPressed() {
record = true;
}
BUT, then I added some perpendicular lines instead:

import processing.svg.*;
int num = 1500;
int range = 6;
boolean record = false;
float[] ax = new float[num];
float[] ay = new float[num];
float[] dx = new float[num];
float[] dy = new float[num];
float[] ox = new float[num];
float[] oy = new float[num];
int num_lines = 15;
void setup()
{
size(640, 640);
for(int i = 0; i < num; i++) {
ax[i] = width/2;
ay[i] = height/2;
dx[i] = 0;
dy[i] = 0;
ox[i] = width/2;
oy[i] = height/2;
}
frameRate(30);
}
void draw()
{
background(255);
// Shift all elements 1 place to the left
for(int i = 1; i < num; i++) {
dx[i-1] = dx[i];
dy[i-1] = dy[i];
ax[i-1] = ax[i];
ay[i-1] = ay[i];
ox[i-1] = ox[i];
oy[i-1] = oy[i];
}
// Put a new value at the end of the array
ax[num-1] = mouseX;
ay[num-1] = mouseY;
dx[num-1] = (ax[num-1] - ax[num-2]);
dy[num-1] = (ay[num-1] - ay[num-2]);
// Draw a line connecting the points
if(record){
int now = millis();
randomSeed(now);
beginRecord(SVG, "foo_" + now + ".svg");
}
for(int i=1; i<num; i++) {
float d_lines = int(map(dx[i] + dy[i],-200, 200, 0,num_lines));
for(float j = 0; j < d_lines; j++){
float multx1 = lerp(ax[i], ax[i-1], j/d_lines);
float multx2 = lerp(ax[i]-dy[i], ax[i-1] - dy[i-1], j/d_lines);
float multy1 = lerp(ay[i], ay[i-1], j/d_lines);
float multy2 = lerp(ay[i] + dx[i], ay[i-1] + dx[i-1], j/d_lines);
line(multx1, multy1, multx2, multy2);
}
}
if(record){
endRecord();
record = false;
}
}
void keyPressed() {
record = true;
}