sketch
/*
Jihoon Park
Section A
jihoonp@andrew.cmu.edu
inClass 10.25
*/
var clouds = [];
var balloons = [];
var stork = [];
function setup() {
createCanvas(400,600);
for (var i=0; i<15; i++) { //initial placement of clouds
var cloudsX = random(width);
var cloudsY = random(height);
clouds[i] = makeClouds(cloudsX, cloudsY);
}
for (var i=0; i<5; i++) { //initial placement of storks
var storkX = random(width);
var storkY = random(100, 500);
stork[i] = makeStork(storkX, storkY);
}
for (var i=0; i<3; i++) { //initial placement of balloons
var balloonX = random(width);
var balloonY = random(height);
balloons[i] = makeBalloons(balloonX, balloonY);
}
frameRate(10);
}
function draw() {
background(132, 181, 204); //sky blue background
showClouds();
deleteClouds();
makeNewClouds();
showBalloons();
deleteBalloons();
makeNewBalloons();
showStork();
deleteStork();
makeNewStork();
myBalloon();
}
//---------------------------FUNCTIONS THAT MAKE CLOUDS--------------------------------------------------
function showClouds() { //this makes the clouds move down
for(var i=0; i<clouds.length; i++) {
clouds[i].move();
clouds[i].display();
}
}
function deleteClouds() { //deletes clouds that disappear from sight
var cloudsToKeep = [];
for (var i=0; i< clouds.length; i++) {
if(clouds[i].y >600) {
cloudsToKeep.push(clouds[i]);
}
}
}
function makeNewClouds() { //creates more clouds coming down
var newCloudLiklihood =0.05
if (random(0,1) < newCloudLiklihood) {
clouds.push(makeClouds(random(width), 0));
}
}
function cloudMove() { //sets the move property of clouds
this.y += this.speed;
}
function cloudDisplay() { //what clouds look like
var cloudSize=this.cloudSize;
fill(223, 255, 233);
noStroke();
push(); //moves the center point to center of cloud
translate(this.x, this.y);
ellipse(0, 0, cloudSize, cloudSize); //graphic elements of clouds
ellipse(0, cloudSize, 3*cloudSize, cloudSize);
ellipse(-cloudSize/2, cloudSize/2, cloudSize, cloudSize);
ellipse(cloudSize/2, cloudSize/2, cloudSize, cloudSize);
pop();
}
function makeClouds(birthLocationX, birthLocationY) { //function that makes the clouds
var clouds = {x : birthLocationX, //clouds are born in random places
y : birthLocationY,
speed : random(1, 5), //sets random speed of clouds
cloudSize : random(10, 25), //sets random size of clouds
move : cloudMove,
display : cloudDisplay}
return clouds;
}
//-------------------------------------------------------------------------------------------------------
//---------------------------FUNCTIONS THAT MAKE STORK---------------------------------------------------
function showStork() { //this makes the stork move left
for(var i=0; i<stork.length; i++) {
stork[i].fly();
stork[i].display();
}
}
function deleteStork() { //deletes stork that disappear from sight
var storkToKeep = [];
for (var i=0; i< stork.length; i++) {
if(stork[i].x <0) {
storkToKeep.push(stork[i]);
}
}
}
function makeNewStork() { //creates more stork coming from left
var newStorkLiklihood =0.03
if (random(0,1) < newStorkLiklihood) {
stork.push(makeStork(650, random(100, 500)));
}
}
function storkFly() {
this.x += this.speed;
}
function storkDisplay() { //makes graphic elements of the Stork
push();
translate(this.x, this.y);
stroke(140);
strokeWeight(1);
fill(255, 220, 98);
triangle(-50, 1, -4, -3, -4, 3); //makes beak
line(-50, 0, -4,0);
strokeWeight(2); //makes legs
line(70, 7, 110, 15);
line(110, 15, 117, 10);
line(110, 15, 120, 15);
line(110, 15, 117, 20);
noStroke();
fill(243, 239, 244);
ellipse(0, 0, 20, 20); //makes head
ellipse(50, 0, 50, 20); //makes body
beginShape(); //makes wings
curveVertex(40, 0);
curveVertex(40, 0);
curveVertex(60, -40);
curveVertex(80, -40);
curveVertex(60, 0);
curveVertex(60, 0);
endShape();
strokeWeight(6);
stroke(243, 239, 244);
line(5,0, 25, 0); //makes neck
fill(0);
noStroke();
stroke(0);
ellipse(0, -2, .2, .2); //makes eyes
pop();
}
function makeStork(birthLocationX, birthLocationY) {
var stork = {x : birthLocationX,
y : birthLocationY,
speed : -random(2, 6),
fly : storkFly,
display : storkDisplay}
return stork;
}
//-------------------------------------------------------------------------------------------------------
function showBalloons() { //this makes the balloons move up
for(var i=0; i<balloons.length; i++) {
balloons[i].move();
balloons[i].display();
}
}
function deleteBalloons() { //deletes Balloons that disappear from sight
var BalloonsToKeep = [];
for (var i=0; i< balloons.length; i++) {
if(balloons[i].y >600) {
balloonsToKeep.push(balloons[i]);
}
}
}
function makeNewBalloons() { //creates more Balloons coming down
var newBalloonLiklihood =0.05
if (random(0,1) < newBalloonLiklihood) {
balloons.push(makeBalloons(random(width), 600));
}
}
function balloonMove() { //sets the move property of Ballons
this.y += this.speed;
}
function balloonDisplay() {
push();
translate(this.x, this.y);
fill(173, 140, 82);
stroke(105, 84, 49);
strokeWeight(3);
beginShape(); //basket
vertex(-7, -7);
vertex(7, -7);
vertex(5, 5);
vertex(-5, 5);
endShape(CLOSE);
stroke(30, 40, 20);
strokeWeight(2);
line(-7, -7, -10, -20);
line(7, -7, 10, -20);
strokeWeight(.5);
fill(255, 97, 118);
ellipse(0, -30, 30, 30);
pop();
}
function makeBalloons(birthLocationX, birthLocationY) {
var balloon = {x : birthLocationX,
y : birthLocationY,
speed : -random(1, 6),
move : balloonMove,
display : balloonDisplay}
return balloon;
}
//---------------------------FUNCTION THAT MAKES MY BALLOON----------------------------------------------
function myBalloon() { //function makes the balloon im in in perspective
//basket
fill(105, 84, 49);
strokeWeight(10);
stroke(173, 140, 82);
beginShape();
vertex(0, 610);
vertex(50, 520);
vertex(350, 520);
vertex(400,610);
endShape(CLOSE);
//rope
stroke(30, 39, 20);
strokeWeight(5);
line(50,515, 20,70);
line(350,515, 380, 70);
//balloon
fill(255, 224, 151);
strokeWeight(1);
stroke(140);
beginShape();
curveVertex(200,-100);
curveVertex(50, -50);
curveVertex(-30, 100);
curveVertex(200, 50);
curveVertex(430, 100);
curveVertex(350, -50);
curveVertex(200, -100);
endShape(CLOSE);
fill(255, 161, 193);
beginShape();
curveVertex(-30, 100)
curveVertex(0, 100);
curveVertex(20,70);
curveVertex(50, -50);
curveVertex(-30, 0);
endShape(CLOSE);
fill(204, 125, 178);
beginShape();
curveVertex(430, 100)
curveVertex(400, 100);
curveVertex(380, 70);
curveVertex(350, -50);
curveVertex(430, 0);
endShape(CLOSE);
}
I created a perspective view from a hot balloon going up into the air. clouds’s downward movement makes an illusion that the viewer is going up while size variation gives the sky a sense of depth. To add more fun, a flock of storks appear as well as other balloons.
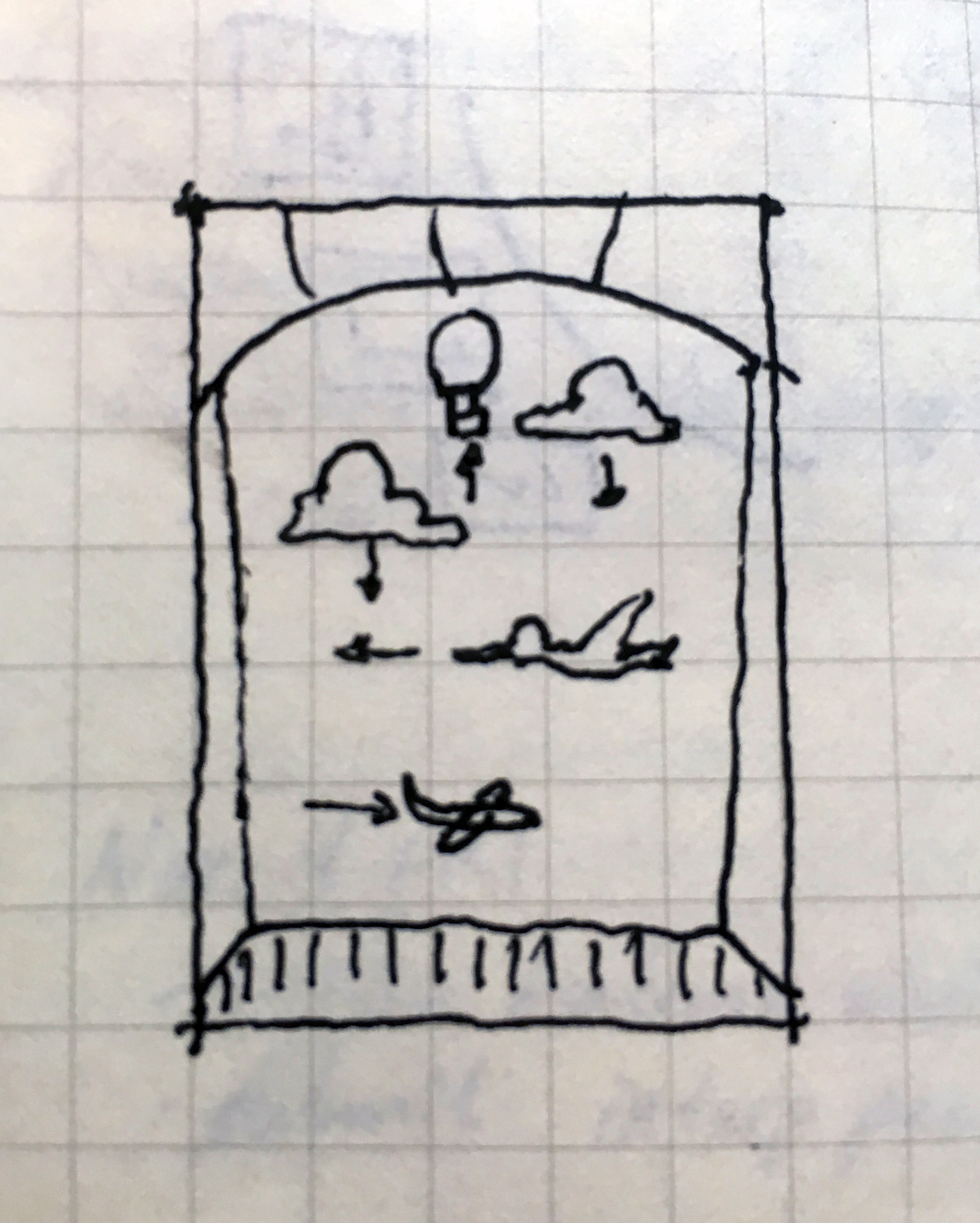