/* Hannah Cai
Section C
hycai@andrew.cmu.edu
Project-04-String Art
*/
var x1 = 100;
var y1 = 50;
var x2 = 300;
var y2 = 250;
var t = 25;
function setup() {
createCanvas(400,300);
background(0);
}
function draw() {
frameRate(40); //slowed down speed
background(0,5); //translucent background
stroke(255);
strokeWeight(.3);
//dotted square frame
for (i = 100; i <= 300; i += 5) {
point(i,50);
point(i,250);
}
for (i = 50; i <= 250; i += 5) {
point(100,i);
point(300,i);
}
// bottom left and top right corner curves
line(x1,250,100,y1);
line(300,height - y1,width - x1,50);
line(width - x1,250,100,height - y1);
line(300,y1,x1,50);
// top left and bottom right corner curves
line(100,y2,x1,50);
line(300,height - y2,width - x1,250);
//string animation
x1 += .5;
y1 += .5;
x2 -= .5;
y2 -= .5;
//constrain movement within square
x1 = constrain(x1,100,300);
y1 = constrain(y1,50,250);
x2 = constrain(x2,100,300);
y2 = constrain(y2,50,250);
//resets string animation when it hits the square's sides
if (x1 == 300 & y1 == 250 && x2 == 100 && y2 == 50) {
x1 = x2;
y1 = y2;
y2 = 250;
}
}
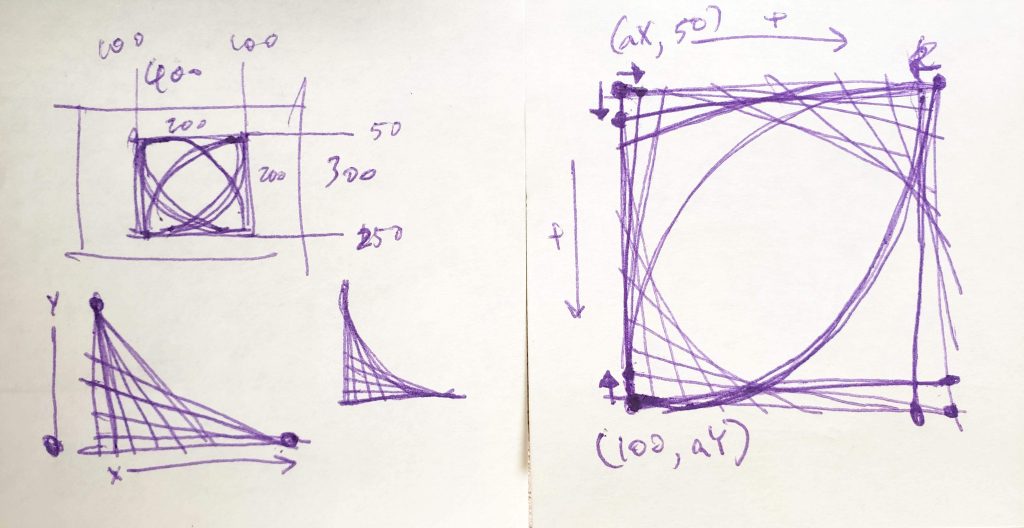
Since this project required four curves, I focused on drawing those curves, and this animation sort of evolved from that process. It took a while for me to figure out how to make curves, and for me to figure out the math for each curve. I know that we were supposed to use for() loops for our strings, but I found using if() made more sense for all the different curves, since there were quite a few different parameters. I’ll probably edit my code to use for() loops instead of if() for the string code as a challenge for myself sometime in the future.