// Lingfan Jiang
// Section B
// lingfanj@andrew.cmu.edu
// Project-06
var y = 100;
var up = 0;
function setup(){
createCanvas (480, 200);
}
function draw(){
background("pink");
//turn down the frame rate to limit the movement
frameRate(1);
angleMode(DEGREES);
var H = hour();
var M = minute();
var S = second();
//remap second according to the width of the canvas
var x = map(S, 0, 60, 0, 480);
//fill the base with dark grey
fill(45);
strokeWeight(0);
rect(0, 145, 48, 100);
rect(48, 140, 40, 100);
rect(88, 135, 40, 100);
rect(128, 130, 40, 100);
rect(168, 125, 40, 100);
rect(208, 120, 72, 100);
rect(280, 125, 40, 100);
rect(320, 130, 40, 100);
rect(360, 135, 40, 100);
rect(400, 140, 40, 100);
rect(440, 145, 40, 100);
//fill the platforms with different shades of grey
fill(240);
rect(0, 110, 48, 35);
fill(200);
rect(48, 105, 40, 35);
fill(160);
rect(88, 100, 40, 35);
fill(120);
rect(128, 95, 40, 35);
fill(100);
rect(168, 90, 40, 35);
fill(80);
rect(208, 85, 72, 35);
fill(100);
rect(280, 90, 40, 35);
fill(120);
rect(320, 95, 40, 35);
fill(160);
rect(360, 100, 40, 35);
fill(200);
rect(400, 105, 40, 35);
fill(240);
rect(440, 110, 40, 35);
textSize(10);
text('Time Survived:', 140, 170);
textSize(13);
text(nf(H + ' hours', 8, 0), 220, 170);
text(nf(M + ' minutes', 10, 0), 279, 170);
//set a strokeweight for the man
strokeWeight(0.7);
//body
line (x, y, x, y + 8);
//front leg
line (x, y + 8, x + 5, y + 10);
line (x + 5, y + 10, x + 5, y + 15);
//back leg
line (x, y + 8, x, y + 11);
line (x, y + 11, x - 5, y + 15);
//arms
line (x, y + 4, x + 6, y + 4);
line (x, y + 6, x + 6, y + 6);
//head
ellipse(x, y, 5, 5);
//pac-man at the back
strokeWeight(0.5);
fill("yellow");
arc(x - 30, y + 8, 20, 20, 40, 320, PIE);
//eye of pac-man
fill(0);
ellipse(x - 30, y + 3, 2.5, 2.5);
//set conditions so that the running man moves up 5 pixels every 5 seconds
if (S % 5 == 0) {
up = 5;
}
else {
up = 0;
};
//set the man back to the left of the screen
if (S > 60) {
x = 0;
};
//make the man goes up before he reaches the middle
if (S > 0 & S < 30){
y -= up;
}
//make the man goes down after he reaches the middle
if (S > 30 & S < 60) {
y += up;
};
}
The way to read the clock is that the minute and the hour are clearly stated in the text, and the second is related to the position of the stickman. The stickman would climb up 5 pixels every 5 seconds before it reaches to the middle, and it would climb down afterward.
The biggest struggle I had while doing this assignment is that I do not know how to make the man only step up once every 5 seconds and not to go straight up unstopping. Also, every time I refresh the page, the stickman would go back to its pre-set “y” location which causes a problem visually. Although there are a few hard codes in it, the final result still turns out good to me.
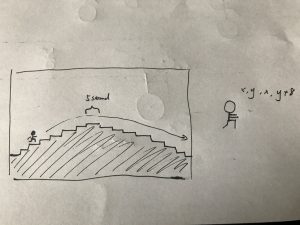