/*
Veronica Wang
Section B
yiruiw@andrew.cmu.edu
Project 06
*/
var prevSec;
var millisRolloverTime;
function setup() {
createCanvas(600, 600);
millisRolloverTime = 0;
}
function draw() {
background(30);
// Fetch the current time
var H = hour();
var M = minute();
var S = second();
// Reckon the current millisecond,
// particularly if the second has rolled over.
if (prevSec != S) {
millisRolloverTime = millis();
}
prevSec = S;
var mils = floor(millis() - millisRolloverTime);
//text label of time
noStroke();
fill('white');
text("Hour: " + H, 10, 22);
text("Minute: " + M, 10, 42);
text("Second: " + S, 10, 62);
text("Millis: " + mils, 10, 82);
//hour ellipse and arc
stroke('gold');
var hourR = 150;
var color = 'gold';
fill(30);
ellipse(width / 3, height / 3, hourR * 2, hourR * 2);
timefiller(24, H, width/3, height/3, hourR, color);
//minute ellipse and arc
stroke('skyblue');
var minR = 100;
color = 'skyblue';
noFill();
ellipse(width * 0.45, height * 0.5, minR * 2, minR * 2);
timefiller(60, M, width * 0.45, height * 0.5, minR, color);
//second ellipse and arc
stroke('lightblue');
var secR = 75;
color = 'lightblue';
noFill();
ellipse(width * 0.6, height * 0.6, secR * 2, secR * 2);
timefiller(60, S, width * 0.6, height * 0.6, secR, color);
//milli second ellipse and arc
stroke('white');
var milR = 50;
color = 'white';
noFill();
ellipse(width * 0.75, height * 0.6, 100, 100);
timefiller(1000, mils, width * 0.75, height * 0.6, milR, color);
//function to draw arc based on division of circle
function timefiller(divNum, currNum, x, y, r, color){
var incr = 2 * PI / (divNum * 2); //divide circle into increments based on time unit
var pt2 = currNum * incr + PI / 2; //time increment point on circle
var pt1 = PI / 2 - currNum * incr; //time increment point on circle
fill(color);
arc(x, y, 2 * r, 2 * r, pt1, pt2, OPEN); //draw open arc based on current time increment points
}
}
In this project I decided to represent the abstract clock with a series of circles being filled up. I challenged myself to write a new function for drawing those open arcs and it turned out just the way I intended. I had trouble figuring out the increments and correct elements to make the arc with, but it reminded me of the string art project which helped me a lot with this.
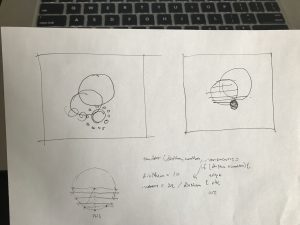