//Kade Stewart
//Section B
//kades
//Project-10
var planes = [];
//a variable that holds each of the colors that a plane could be
var pastels = [230, 245, 245, 255, 250, 205, 180, 236, 180];
var terrain1 = [];
var terrain2 = [];
var terrain3 = [];
function setup() {
createCanvas(480, 480);
//initialize a plane
planes[(planes.length)] = new Plane();
//add terrain that has random but connected altitudes
var y = height/4;
for (var i = 0; i < width; i++) {
terrain1.push(y += random(-1,1));
}
y = height/2;
for (var i = 0; i < width; i++) {
terrain2.push(y += random(-1,1));
}
y = height * (3/4);
for (var i = 0; i < width; i++) {
terrain3.push(y += random(-1,1));
}
}
function Plane() {
this.y = random(0, height);
this.x = width + 50;
this.speed = floor(random(-.01, -3)); //this is the speed that the plane actually goes back
this.path = []; //this is where the path is stored for the plane
this.pathspeed = this.speed * 3; //this is the speed that the path is made
this.c = floor(random(1,4)); //this is where the color is determined
//this is function that draws both the plane and the path
this.draw = function() {
stroke(0);
strokeWeight(1);
fill(pastels[this.c], pastels[this.c + 1], pastels[this.c + 2]);
triangle(this.x - (6 * -this.pathspeed/2), this.y - 9 * -this.pathspeed/2,
this.x + 18 * -this.pathspeed/2, this.y,
this.x, this.y);
//draw the path
if (this.path.length > 2) {
for (var i = 1; i < this.path.length; i++) {
stroke(125);
strokeWeight(1);
noFill();
line(this.x + (this.pathspeed * (i-1)), this.path[this.path.length - i - 1],
this.x + (this.pathspeed * (i)), this.path[this.path.length - i]);
}
}
}
}
function updatePlanes(index) {
planes[index].x += planes[index].speed;
planes[index].y += random(-1, 1);
//if the plane is past the window, delete it
if (planes[index].x - (18 * planes[index].pathspeed/2) < 0) {
planes.splice(index, 1);
}
}
function updatePath(index) {
var p = planes[index];
//add the planes current position to the path to trace where it's been
p.path.push(p.y);
//if the path is longer than the window, delete what's outside of the window
if (p.path.length * p.pathspeed > width) {
p.path.splice(0, 1);
}
}
function randomlyMakePlane(odds) {
//this is the randomizer that decided whether the plane is made or not
var newPlaneMaybe = random(-odds + 1, 1);
if (newPlaneMaybe > 0) {
planes[(planes.length)] = new Plane();
}
}
//draw 3 different tiers of background, to give a sense of depth
function drawTerrain() {
for (var j = 0; j < width; j++) {
stroke(255, 241, 245);
strokeWeight(5);
noFill();
line(j, terrain1[j], j, height);
}
for (var j = 0; j < width; j++) {
stroke(255, 235, 239);
strokeWeight(5);
noFill();
line(j, terrain2[j], j, height);
}
for (var j = 0; j < width; j++) {
stroke(255, 209, 220);
strokeWeight(5);
noFill();
line(j, terrain3[j], j, height);
}
}
function updateTerrain() {
terrain1.splice(0, 1);
terrain1.push(terrain1[terrain1.length-1] + random(-1, 1));
terrain2.splice(0, 1);
terrain2.push(terrain2[terrain2.length-1] + random(-1, 1));
terrain3.splice(0, 1);
terrain3.push(terrain3[terrain3.length-1] + random(-1, 1));
}
function draw() {
background(255, 250, 253);
//this is where the terrain is drawn and then generated
drawTerrain();
updateTerrain();
for (var i = 0; i < planes.length; i++) {
var p = planes[i];
//draw the planes
planes[i].draw();
//update its path
updatePath(i);
//update its x and y position, and delete it if it's too far off the screen
updatePlanes(i);
}
//**60** to 1 odds that there is a new plane made (about 1 every second)
randomlyMakePlane(60);
}
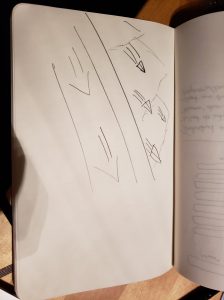
I wanted to make a generative landscape that wasn’t just actual land, or even anything that was extremely natural, but something more artificial. I came up with paper airplanes, which ended up being an interesting challenge. I randomly generated airplanes that were large and fast to look closer to the viewer (who’s reference frame was moving forward) or small and slow to seem farther away. The background, varying pink levels, also moves to increase the viewer’s sense that they’re moving as well. Overall, I think I achieved my goal of giving the viewer a sense of wonderment.