// Han Yu
// Section D
// hyu1@andrew.cmu.edu
// Project 10 Generative Landscape
var terrainSpeed = 0.0005;
var terrainDetail = 0.01;
var terrainD1 = 0.0005;
var terrainS1 = 0.0002;
var fish1 = "https://i.imgur.com/pw06Fwc.png";
var fish = [];
var fishy1;
function preload() {
fishy1 = loadImage(fish1);
}
function setup() {
createCanvas(480, 300);
//create an initial collection of fish
for (var i=0; i<10; i++) {
var rx = random(width*5);
fish[i] = makeFish(rx);
}
frameRate(10);
}
function draw() {
background(255);
//sun
noStroke();
fill(252,163,41);
ellipse(80, 60, 70, 70);
//mountain
noFill(0);
stroke(135,120,108)
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t),0,1,20,height)-height/4;
vertex(x, height);
vertex(x, y);
}
endShape();
fill(135,120,108,100);
noStroke();
//water-top
beginShape();
stroke(119,209,249,150);
for (var x1 = 0; x1 < width; x1++) {
var t1 = (x1 * terrainD1) + (millis() * terrainS1);
var y1 = map(noise(t1),0,1,20,height);
vertex(x1, height);
vertex(x1,y1);
}
endShape();
//water-bottom
beginShape();
stroke(119,209,259);
for (var x1 = 0; x1 < width; x1++) {
var t1 = (x1 * terrainD1) + (millis() * terrainS1);
var y1 = map(noise(t1),0,1,28,height);
vertex(x1, height);
vertex(x1,y1);
}
endShape();
//fish
push();
translate(0.1 * width, 0.1 * height);
scale(0.8);
scale(0.2,0.2);
updateAndDisplayFish();
addNewFishWithProbability();
}
function updateAndDisplayFish() {
for (var i = 0; i < fish.length; i++) {
fish[i].move();
fish[i].display();
}
}
function addNewFishWithProbability() {
var newFishLikelihood = 0.05;
if (random(1) < newFishLikelihood) {
fish.push(makeFish(-300));
}
}
function fishMove() {
this.x += this.speed*(1/this.size);
}
function fishDisplay() {
push();
scale(this.size, this.size);
translate(this.x*(1/this.size), this.y*(1/this.size));
image(fishy1,0,0);
pop();
}
function makeFish(birthLocationX) {
var fishy = {x: birthLocationX,
size: random(0.3, 0.6),
y: random(0.8*height*(1/0.2), height*(1/0.2)),
speed: random(1,5),
move: fishMove,
display: fishDisplay};
return fishy;
}
I started out thinking to do a project on natural landscape with mountain, water and grassland. But as I dived into the project, I found the overall scene rather dull and lifeless so I decided to focus on the underwater scene with fish instead.
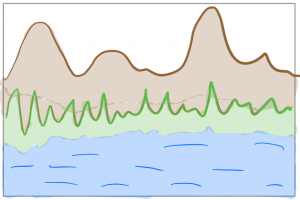