My process was starting with a sketch and then systematically testing and working out the individual controls for each circle.
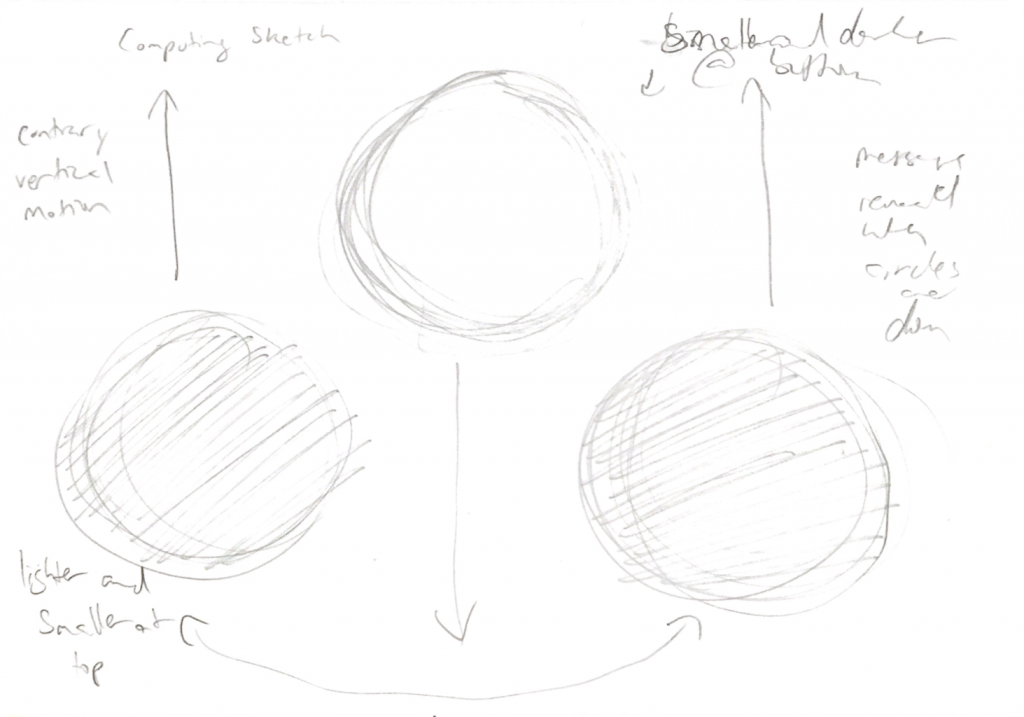
//4 parameters: shade of circles/text, size of circles, position of circles, which message appears
function setup() {
createCanvas(600, 450);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(255,0,0);
var grayscale = max(min(mouseX,255),0); //as mouse moves right circles become lighter
var size1 = max(min(mouseY,227),70); //increases size as mouse moves down. Min diameter is 70, max diameter is 227
var size2 = 297 - size1; //opposite of size 1, makes the middle circle have the opposite diameter of the other two
var yShift = constrain(mouseY,0,height/3); //as mouse moves down circles move down
var yShift1 = -yShift; //two circles move opposite direction of center circle
//mouse makes different messages appear
if(yShift == height/3){ //when the middle circle is at its lowest position the message "Et hoco modo nihil" appears
textSize(45);
fill(abs(255-grayscale));
textAlign(LEFT);
text('Et hoc',50,400);
textAlign(CENTER);
text('modo',width/2,50);
textAlign(RIGHT);
text('nihil',550,400);
}
if(yShift == 0){ //when the middle circle is at its highest position the message "In Quarta Parametri" appears
textSize(45);
fill(abs(255-grayscale));
textAlign(LEFT);
text('In',50,50);
textAlign(CENTER);
text('Quarta', width/2, 400);
textAlign(RIGHT);
text('Parametri',550,50);
}
if(height/3 + yShift + 30 == height/2){ //When all three circles are aligned the message "Quid est amor? Infantem et non cocuerunt mihi" appears
textSize(45);
textAlign(LEFT);
fill(grayscale);
text('Quid est amor?',50,50);
textSize(30);
textAlign(RIGHT);
fill(abs(255-grayscale));
text('Infantem et non nocuerunt mihi.',550,400);
}
noStroke();
fill(grayscale);
circle(width/5,(height*2)/3 + yShift1 + 10,size1);
circle((width*4)/5,(height*2)/3 + yShift1 + 10,size1);
fill(abs(255-grayscale)); //this circle experiences the opposite color of the other two
circle(width/2,height/3 + yShift + 30,size2);
}