For this project, I was really inspired by some of the curves and the fact that the hypocycloid resembled the outline of a flower! I wanted to create something that was a cross between something resembling Tyler the Creator’s ‘golf wang’ line and a bed of moving flowers in the wind.
I therefore came up with my final product, which has flowers with centers that move and rotate depending on the user’s mouse position:
sketchDownload
// Susie Kim
// susiek@andrew.cmu.edu
// Section A, 15-104
// Assignment 7
// set global variables
var angle = 0;
function setup() {
createCanvas(480, 480);
frameRate(15);
}
function draw() {
background(247, 202, 201); // sets background to pink tone
// create grid of flowers, starting at 40, 40. results in a 5x5 grid of flowers.
for(var x = 40; x <= 480; x+= 100) {
for(var y = 40; y <= 480; y+= 100) {
push();
translate(x, y);
// have flowers rotate depending on mouse X pos
rotate(radians(angle));
angle += mouseX;
// call upon flower functions to draw flower outer and center
drawHypocycloid();
drawAstroid();
pop();
}
}
}
// draw blue flower exterior
function drawHypocycloid() {
// set local variables
var a = int(map(mouseX, 0, width, 15, 40));
var b = int(map(mouseY, 0, height, 8, 13));
// set colors and strokes
fill(145, 168, 209);
strokeWeight(2);
stroke(255);
beginShape();
// draw hypocycloids, with size increasing for mouse X and number of petals increasing with mouseY
for (var i = 0; i < 250; i++) {
var subAngle = map(i, 0, 110, 0, TWO_PI);
var x = a*((b - 1)*cos(subAngle) + cos(subAngle) + cos((b - 1)*subAngle)) / b;
var y = a*((b - 1)*sin(subAngle) + sin(subAngle) + sin((b - 1)*subAngle)) / b;
vertex(x,y);
}
endShape();
}
// draw yellow center of the flowers
function drawAstroid() {
// set colors and strokes
fill(255, 255, 0);
strokeWeight(.1);
stroke(255);
beginShape();
// draw for each astroid, with size increasing with mouse X position
for (var i = 0; i < 300; i++) {
var subAngle = map(mouseX, 0, width, 7, 15); // changes size with X pos
var x2 = subAngle*pow(cos(i), 5);
var y2 = subAngle*pow(sin(i), 5);
vertex(x2, y2);
}
endShape();
}
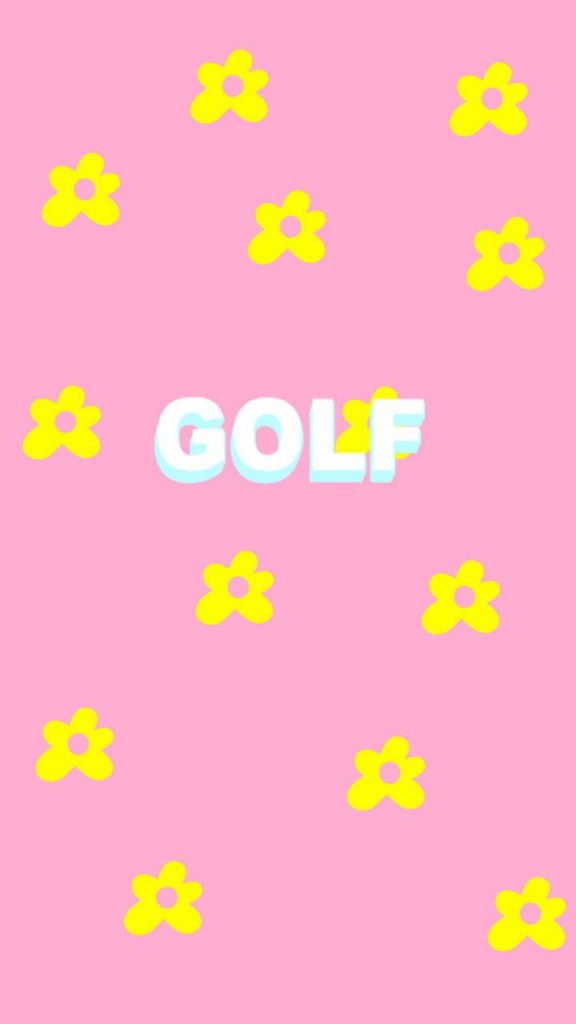
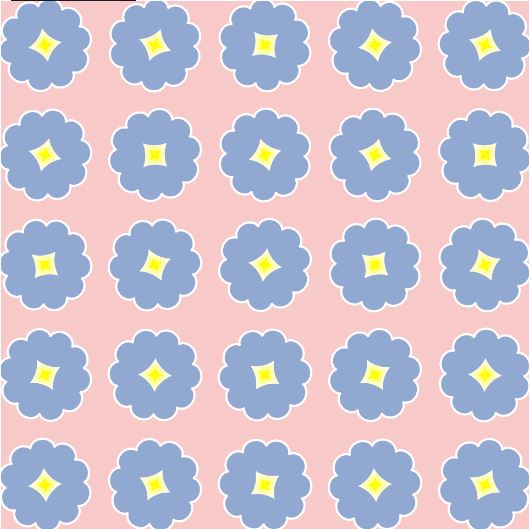
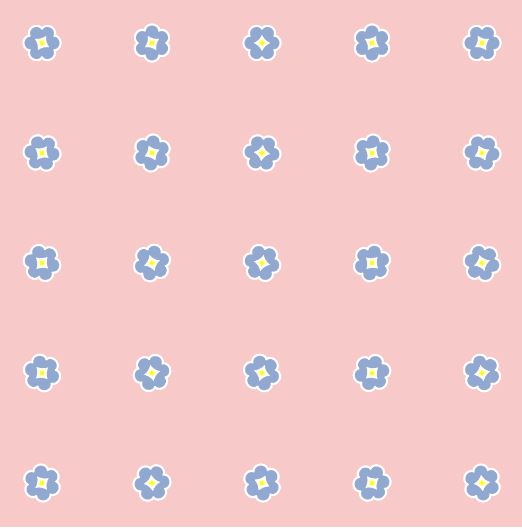