//simple string art
//hollyl
//section d
var dx1;
var dy1;
var dx2;
var dy2;
var numLines = 25;
function setup(){
createCanvas(400, 300);
background(200);
line(200, 25, 200, 125);
line(217.67, 132.33, 288.38, 61.17);
line(225, 150, 325, 150);
line(217.67, 167.67, 288.38, 238.38);
line(200, 175, 200, 275);
line(182.33, 167.67, 111.62, 238.38);
line(175, 150, 75, 150);
line(182.33, 132.33, 111.62, 61.17);
dx1 = (200 - 200)/numLines;
dy1 = (125 - 25)/numLines;
dx2 = (288.38 - 217.67)/numLines;
dy2 = (61 - 132.33)/numLines;
dx3 = (325 - 225)/numLines;
dy3 = (150 - 150)/numLines;
dx4 = (288.38 - 217.67)/numLines;
dy4 = (238.38 - 167.67)/numLines;
dx5 = (200 - 200)/numLines;
dy5 = (275 - 175)/numLines;
dx6 = (182.33 - 111.62)/numLines;
dy6 = (238.38 - 167.67)/numLines;
dx7 = (175 - 75)/numLines;
dy7 = (150 - 150)/numLines;
dx8 = (182.33 - 111.62)/numLines;
dy8 = (132.33 - 61.17)/numLines;
}
function draw(){
var x1 = 200; //north-north-east
var y1 = 25;
var x2a = 217.67;
var y2a = 132.33;
for(var i = 0; i <= numLines; i += 1){
line(x1, y1, x2a, y2a);
x1 += dx1;
y1 += dy1;
x2a += dx2;
y2a += dy2;
}
var x2b = 288.38; //north-east-east
var y2b = 61.17;
var x3a = 225;
var y3a = 150;
for(var i = 0; i <= numLines; i += 1){
line(x2b, y2b, x3a, y3a);
x2b -= dx2;
y2b -= dy2;
x3a += dx3;
y3a -= dy3;
}
var x3b = 325; //south-east-east
var y3b = 150;
var x4a = 217.67;
var y4a = 167.67;
for (var i = 0; i <= numLines; i += 1){
line(x3b, y3b, x4a, y4a);
x3b -= dx3;
y3b += dy3;
x4a += dx4;
y4a += dy4;
}
var x4b = 288.38; //south-south-east
var y4b = 238.38;
var x5a = 200;
var y5a = 175;
for (var i = 0; i <= numLines; i += 1){
line(x4b, y4b, x5a, y5a);
x4b -= dx4;
y4b -= dy4;
x5a += dx5;
y5a += dy5;
}
var x5b = 200; //south-south-west
var y5b = 275;
var x6a = 182.33;
var y6a = 167.67;
for (var i = 0; i <=numLines; i += 1){
line(x5b, y5b, x6a, y6a);
x5b += dx5;
y5b -= dy5;
x6a -= dx6;
y6a += dy6;
}
var x6b = 111.62; //south-west-west
var y6b = 238.38;
var x7a = 175;
var y7a = 150;
for (var i = 0; i <= numLines; i += 1){
line(x6b, y6b, x7a, y7a);
x6b += dx6;
y6b -= dy6;
x7a -= dx7;
y7a += dy7;
}
var x7b = 75; //north-west-west
var y7b = 150;
var x8a = 182.33;
var y8a = 132.33;
for (var i = 0; i <= numLines; i += 1){
line(x7b, y7b, x8a, y8a);
x7b += dx7;
y7b += dy7;
x8a -= dx8;
y8a -= dy8;
}
var x8b = 111.62; //north-north-west
var y8b = 61.17;
var x1b = 200;
var y1b = 125;
for (var i = 0; i <= numLines; i += 1){
line(x8b, y8b, x1b, y1b);
x8b += dx8;
y8b += dy8;
x1b += dx1;
y1b -= dy1;
}
noLoop();
}
notes:
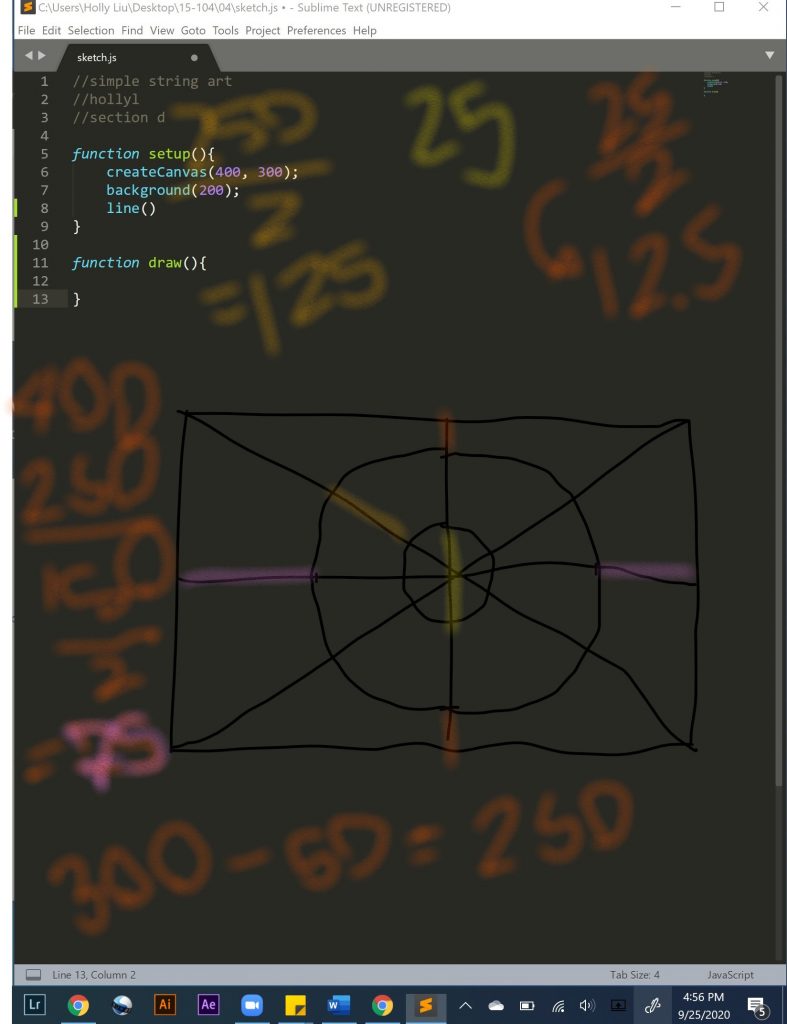