I recently saw a tv show that talked about fish. It reminded me of my goldfish I used to take care of for 7 years, but died just a few months before I graduated high school. In memories of my goldfish, I wanted to create an abstract clock of a fish tank in space on an unknown planet. Each tank represents hours, minutes, and seconds as the water fills up the tank.
//Stefanie Suk
//ssuk@andrew.cmu.edu
//15-104 Section D
var fishx = 15; //width of fish
var fishy = 10; //height of fish
function setup() {
createCanvas(600, 400);
}
function draw() {
var h = hour();
var m = minute();
var s = second();
var starx = random(0, 600); //random x position of stars
var stary = random(0, 250); //randolm y position of stars
background(50); //background color
//moon and stars
fill("yellow");
ellipse(70, 70, 100, 100); //moon at top left
ellipse(starx, stary, 5, 5); //stars randomly placed
//light brown base of planet
fill(184, 165, 162)
rect(0, 250, width, 200);
//darker brown oval holes on planet, left to right
fill(158, 142, 140);
strokeWeight(6);
stroke(0);
ellipse(30, 300, 100, 40);
ellipse(100, 380, 100, 40);
ellipse(300, 290, 100, 40);
ellipse(400, 350, 100, 40);
ellipse(600, 280, 100, 40);
//mapping time to fit in the fish tank
mS = map(s, 0, 70, 0, 110);
mM = map(m, 0, 70, 0, 110);
mH = map(h, 0, 30, 0, 110);
//hour tank on left, dark blue
strokeWeight(5);
stroke(255);
fill(31, 56, 240);
rect(120, 150, 100, 100);
//minute tank in middle, dark blue
stroke(255);
fill(31, 56, 240);
rect(260, 150, 100, 100);
//second tank on right, dark blue
stroke(255);
fill(31, 56, 240);
rect(400, 150, 100, 100);
//three fish in each tank right to left
strokeWeight(1);
stroke(0);
fill(255, 169, 157);
ellipse(420, 230, fishx, fishy);
triangle(427, 230, 435, 225, 435, 235);
ellipse(320, 200, fishx, fishy);
triangle(313, 200, 305, 195, 305, 205);
ellipse(190, 215, fishx, fishy);
triangle(197, 215, 205, 210, 205, 220);
//time rect in tank changing upwards, light blue
noStroke();
fill(157, 185, 255);
rect(122.5, 152, 95, 95 - mH); //hour
fill(157, 185, 255);
rect(262.5, 152, 95, 95 - mM); //minute
fill(157, 185, 255);
rect(402.5, 152, 95, 95 - mS); //second
}
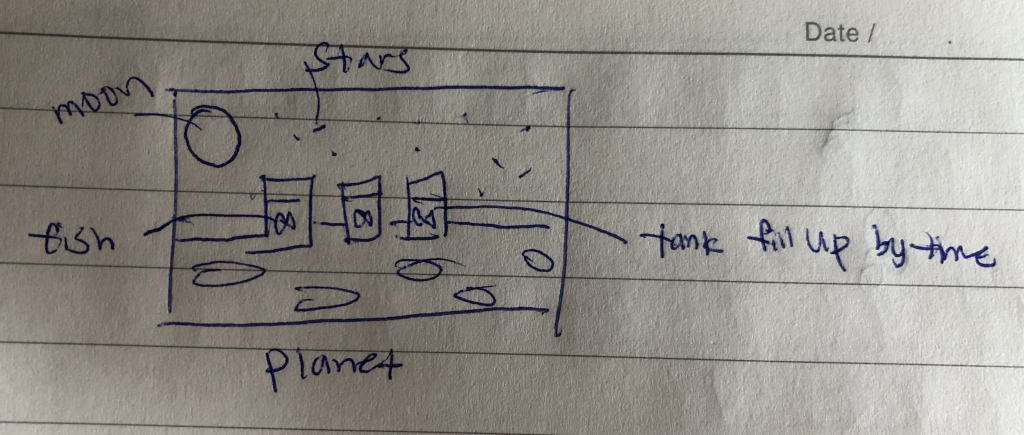