/*
Nicholas Wong
Section A
nwong1@andrew.cmu.edu
*/
var rockForeground = []; //Rocks in foreground
var rockMidground = []; //Rocks in midground
var mountains = []; //Mountains in background
//Rock variables
var foregroundRockChance = 0.03; //Probability of rock spawn per frame
var midgroundRockChance = 0.03; //Probability of rock spawn per frame
//Mountain variables
var noiseParam = 1; //Noise param for mountain generation
var noiseStep = 0.01; //Noise step for mountain generation
//Sky variables
var offset = 23; //Wave offset
//Floor grid variables
var lineStart = []; //Line at horizon
var lineEnd = []; //Line at bottom of canvas
function setup()
{
frameRate(30);
createCanvas(480, 300);
//Initialize mountains
for(let i = 0; i < width/4; i++)
{
var n = noise(noiseParam);
mountains[i];
var value = map(n, 0, 1, 0, height);
mountains.push(value);
noiseParam += noiseStep;
}
//Initialize Floor lines
for(let i = 0; i<49; i++)
{
lineStart[i] = 10*i;
lineEnd[i] = -1200 + (60*i);
}
}
function draw()
{
background(255, 166, 200);
//Draw sky wave
drawSkyPattern();
//Draw mountains
drawMountains();
//Draw floor
drawFloor();
//Draw floor lines
drawFloorLines();
//Color for rocks
var foregroundColor = color(115, 31, 77);
var midgroundColor = color(130, 57, 84);
//Create rocks based on probability
if(random(0,1) < foregroundRockChance)
{
rockForeground.push(createRock(width + 20,220 + random(0,20),20,random(20,30),foregroundColor)); //Rocks in foreground
}
else if (random(0,1) < midgroundRockChance)
{
rockMidground.push(createRock(width + 20, 190 + random(-20,20),10, 20, midgroundColor)); //Rocks in background
}
//Update positions
updateMidgroundRocks();
updateForegroundRocks();
}
//Draw wave
function drawSkyPattern()
{
push();
noFill();
for(let w = 0; w <= height; w += 10) //Number of waves
{
strokeWeight(1)
stroke(255)
//Create curvy lines
beginShape();
for(let x = 0; x < width; x++)
{
let angle = offset + x *0.01;
let y = map(sin(angle), -1, 1, 0, 50);
vertex(x,y + w);
}
vertex(width,height);
vertex(0,height);
endShape();
}
pop();
}
//Draw mountains
function drawMountains()
{
//Add and remove noise values to mountain list
mountains.shift();
var n = noise(noiseParam);
var value = map(n, 0, 1, 0, height);
noiseParam += noiseStep;
mountains.push(value);
//Draw mountain shapes based on noise values
beginShape();
for(var i = 0; i <= width/4; i++)
{
fill(224, 105, 149);
noStroke();
vertex((i * 6), mountains[i] - 50);
vertex((i + 1) * 6, mountains[i + 1] - 50);
vertex(300, 100000);
}
endShape(CLOSE);
//Draw mountain shapes based on noise values
beginShape();
for(var i = 0; i <= width/3; i++)
{
fill(191, 86, 125);
noStroke();
vertex((i * 10), mountains[i]- 10);
vertex((i + 1) * 10, mountains[i + 1] - 10);
vertex(300, 100000);
}
endShape(CLOSE);
}
//Update and cull rocks
function updateForegroundRocks()
{
for(let i = 0; i < rockForeground.length; i++)
{
rockForeground[i].move();
rockForeground[i].display();
//Check to see if rocks are off canvas
if(rockForeground[i].x + rockForeground[i].size < 0)
{
rockForeground.shift(); //Remove rock
}
}
}
//Update and cull rocks
function updateMidgroundRocks()
{
//Check to
for(let i = 0; i < rockMidground.length; i++)
{
rockMidground[i].move();
rockMidground[i].display();
//Check to see if rocks are off canvas
if(rockMidground[i].x + rockMidground[i].size < 0)
{
rockMidground.shift(); //Remove rock
}
}
}
//Draw floor lines
function drawFloorLines()
{
push();
for(let i = 0; i < 49; i++)
{
stroke(255, 148, 194);
strokeWeight(1);
//Draw line
line(lineStart[i],170,lineEnd[i],height);
//Move lines
lineStart[i] -= 5;
lineEnd[i] -= 30;
//Reset lines if off canvas
if(lineStart[i] == 0)
{
lineStart[i] = width;
}
if(lineEnd[i] == -1200)
{
lineEnd[i] = 1680;
}
}
pop();
}
//Draw floor rectangle
function drawFloor()
{
push();
noStroke();
fill(158, 70, 102);
rect(0,170,width,height);
pop();
}
//Create rock
function createRock(xPos, yPos,rockSpeed, rockSize, rockColor, )
{
var fRock = {x: xPos,
y: yPos,
speed: rockSpeed * -1,
sides: random(3,5),
size: rockSize,
color: rockColor,
variation: random(0.5,1),
move: rockMove,
display: rockDisplay}
return fRock;
}
function rockMove()
{
this.x += this.speed; //Move the rock
}
function rockDisplay()
{
//Change rock color
fill(this.color);
noStroke();
//Create rock based on size, variation, and number of sides
let angle = TWO_PI / this.sides;
beginShape();
for (let a = 0; a < TWO_PI; a += angle)
{
let sx = this.x + cos(a * this.variation) * this.size;
let sy = this.y + sin(a * this.variation) * this.size;
vertex(sx, sy);
}
endShape(CLOSE);
}
A stylized desert. Rocks are objects that are randomly generated, and are automatically culled when off the canvas. Rocks further away from the bottom of the canvas move slower than rocks closer to the bottom of the canvas. Mountains vary in speed to give the illusion of depth.
Inspiration:
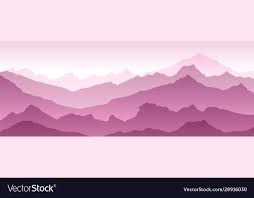