For this project I modeled the face from the aliens in Toy Story. I think they have relatively simple features that were easier to abstract. I found a cartoon collector toy version of them which served as a much better basis for the actual shapes.
sketchDownload
function setup() {
createCanvas(600, 600);
background(220);
noLoop();
}
function draw() {
var antennas = random(3);
var eyes = random(3);
var mouth = random(4);
var background = random(6);
if (background < 1) {
var backgroundColor = "#BF2D22"
} else if (background > 1 & background < 2) {
var backgroundColor = "#730B03"
} else if (background > 2 && background < 3) {
var backgroundColor = "#40211F"
} else if (background > 3 && background < 4) {
var backgroundColor = "#CA6861"
} else if (background > 4 && background < 5) {
var backgroundColor = "#400F0B"
} else {
var backgroundColor = "#8C2119"
}
//background
fill(backgroundColor);
noStroke();
rect(0,0,width,height);
//head
fill(179,209,25); //green
rect(75,175,450,350,90);
//ears
beginShape();
curveVertex(75,275);
curveVertex(75,275);
curveVertex(55,250);
curveVertex(35,230);
curveVertex(28,233);
curveVertex(25,240);
curveVertex(25,240);
curveVertex(30,350);
curveVertex(30,350);
curveVertex(28,360);
curveVertex(31,373);
curveVertex(38,380);
curveVertex(55,375);
curveVertex(65,370);
curveVertex(75,365);
curveVertex(75,365);
endShape();
beginShape();
curveVertex(600-75,275);
curveVertex(600-75,275);
curveVertex(600-55,250);
curveVertex(600-35,230);
curveVertex(600-28,233);
curveVertex(600-25,240);
curveVertex(600-25,240);
curveVertex(600-30,350);
curveVertex(600-30,350);
curveVertex(600-28,360);
curveVertex(600-31,373);
curveVertex(600-38,380);
curveVertex(600-55,375);
curveVertex(600-65,370);
curveVertex(600-75,365);
curveVertex(600-75,365);
endShape();
stroke(0,0,0,60); //transparent black
strokeWeight(5);
noFill();
beginShape();
curveVertex(75,285);
curveVertex(75,285);
curveVertex(35,240);
curveVertex(45,360);
curveVertex(45,360);
endShape();
beginShape();
curveVertex(600-75,285);
curveVertex(600-75,285);
curveVertex(600-35,240);
curveVertex(600-45,360);
curveVertex(600-45,360);
endShape();
//collar
stroke(126,77,152); //purple
strokeWeight(40);
line(155,530,455,530);
//shirt
noStroke();
fill(6,149,209); //blue
beginShape();
curveVertex(0,599);
curveVertex(0,599);
curveVertex(25,555);
curveVertex(155,530);
curveVertex(155,530);
curveVertex(455,530);
curveVertex(455,530);
curveVertex(574,555);
curveVertex(599,599);
curveVertex(599,599);
endShape();
//antennas
if (antennas < 1) {
fill(179,209,25);
beginShape();
curveVertex(270,175);
curveVertex(270,175);
curveVertex(285,125);
curveVertex(270,75);
curveVertex(270,75);
curveVertex(270,75);
curveVertex(330,75);
curveVertex(330,75);
curveVertex(330,75);
curveVertex(315,125);
curveVertex(330,175);
curveVertex(330,175);
endShape();
circle(300,75,60);
} else if (antennas > 1 & antennas < 2){
fill(179,209,25);
beginShape();
curveVertex(270-50,175);
curveVertex(270-50,175);
curveVertex(285-50,125);
curveVertex(270-50,75);
curveVertex(270-50,75);
curveVertex(270-50,75);
curveVertex(330-50,75);
curveVertex(330-50,75);
curveVertex(330-50,75);
curveVertex(315-50,125);
curveVertex(330-50,175);
curveVertex(330-50,175);
endShape();
beginShape();
curveVertex(270+50,175);
curveVertex(270+50,175);
curveVertex(285+50,125);
curveVertex(270+50,75);
curveVertex(270+50,75);
curveVertex(270+50,75);
curveVertex(330+50,75);
curveVertex(330+50,75);
curveVertex(330+50,75);
curveVertex(315+50,125);
curveVertex(330+50,175);
curveVertex(330+50,175);
endShape();
circle(300+50,75,60);
circle(300-50,75,60);
} else {
fill(179,209,25);
beginShape();
curveVertex(270,175);
curveVertex(270,175);
curveVertex(285,125);
curveVertex(270,75);
curveVertex(270,75);
curveVertex(270,75);
curveVertex(330,75);
curveVertex(330,75);
curveVertex(330,75);
curveVertex(315,125);
curveVertex(330,175);
curveVertex(330,175);
endShape();
beginShape();
curveVertex(270+80,175);
curveVertex(270+80,175);
curveVertex(285+80,125);
curveVertex(270+80,75);
curveVertex(270+80,75);
curveVertex(270+80,75);
curveVertex(330+80,75);
curveVertex(330+80,75);
curveVertex(330+80,75);
curveVertex(315+80,125);
curveVertex(330+80,175);
curveVertex(330+80,175);
endShape();
beginShape();
curveVertex(270-80,175);
curveVertex(270-80,175);
curveVertex(285-80,125);
curveVertex(270-80,75);
curveVertex(270-80,75);
curveVertex(270-80,75);
curveVertex(330-80,75);
curveVertex(330-80,75);
curveVertex(330-80,75);
curveVertex(315-80,125);
curveVertex(330-80,175);
curveVertex(330-80,175);
endShape();
circle(300,75,60);
circle(300+80,75,60);
circle(300-80,75,60);
}
//eyes
if (eyes < 1) {
fill(255,255,255);
stroke(0);
strokeWeight(3);
circle(300,275,90);
fill(0);
circle(300,275,40);
} else if (eyes > 1 & eyes < 2) {
fill(255,255,255);
stroke(0);
strokeWeight(3);
circle(300-60,275,90);
fill(0);
circle(300-60,275,40);
fill(255,255,255);
circle(300+60,275,90);
fill(0);
circle(300+60,275,40);
} else {
fill(255,255,255);
stroke(0);
strokeWeight(3);
circle(300,275,90);
fill(0);
circle(300,275,40);
fill(255,255,255);
circle(300+110,275,90);
fill(0);
circle(300+110,275,40);
fill(255,255,255);
circle(300-110,275,90);
fill(0);
circle(300-110,275,40);
}
//smile
var mouthH = random(400,450);
var mouthW = random(100,150);
noFill();
stroke(0);
strokeWeight(10);
beginShape();
curveVertex((width/2)+mouthW,400);
curveVertex((width/2)+mouthW,400);
curveVertex(300,mouthH);
curveVertex((width/2)-mouthW,400);
curveVertex((width/2)-mouthW,400);
endShape();
}
function mouseClicked() {
loop();
noLoop();
clear();
background(220);
//allowing user to click to loop another random face
}
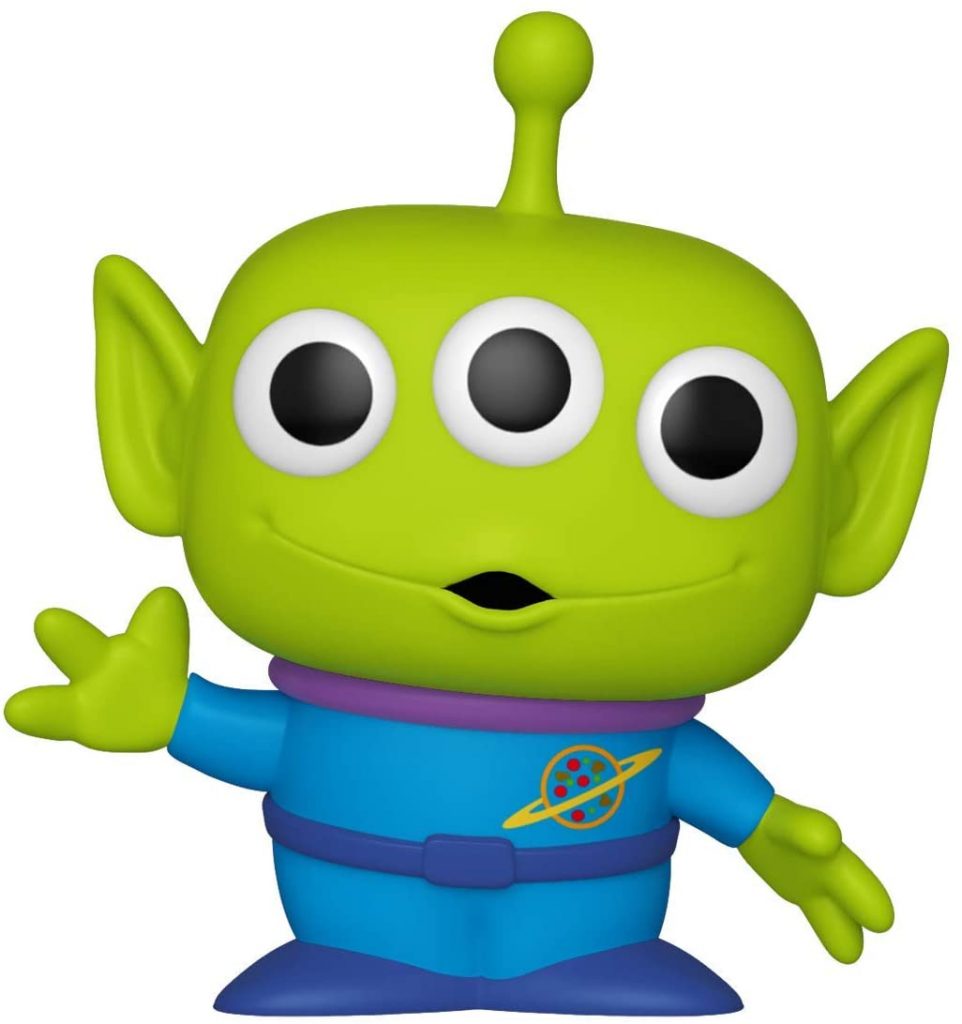