I personally have a preference for pastel colors because I think when you use patterns/repeating patterns, it is visually “easier on the eyes.” I wanted to use a light green background, and it inspired me to make a project about nature and animals. I wanted to challenge myself in terms of producing the image that would get repeated, so I chose a koala. I made the tree trunk pretty long because I wanted the overall wallpaper to appear as if there were koalas sitting on every branch up and down the tree. It was challenging to layer shapes to create the koala itself because its legs were in a specific position which forced me to use a lot of curveVertex in order to get the shape just right.
//Annie Kim
//andrewID:anniekim@andrew.cmu.edu
//anniekim-05-Project
//SectionB
function setup() {
createCanvas(525, 500);
background(204, 242, 207); //light green background
noLoop();
}
function draw() {
background(204, 242, 207); //light green background
noStroke();
//for loop to repeat the koalas everywhere
for (var x = 0; x < width; x += 88.3) {
for (var y = 0; y < height; y += 96) {
push();
translate(x, y);
drawKoala();
pop();
}
}
} //function draw end
function drawKoala() {
//branch that koala is sitting on
fill(80, 55, 41); //brown color
stroke(80, 55, 41);
beginShape();
curveVertex(.6, 83);
curveVertex(.6, 83);
curveVertex(25, 84);
curveVertex(60, 85); //right endpoint of branch
curveVertex(65, 88);
curveVertex(58, 88);
curveVertex(23, 94);
curveVertex(.6, 96);
curveVertex(.6, 96);
endShape();
fill(137, 189, 161); //leaf on branch
stroke(67, 109, 91); //green color
beginShape();
curveVertex(65, 88);
curveVertex(65, 88);
curveVertex(71, 89);
curveVertex(73, 90);
curveVertex(77, 96);
curveVertex(73, 95);
curveVertex(71, 94);
curveVertex(69, 93);
curveVertex(68, 92);
curveVertex(67, 91);
curveVertex(65, 88);
curveVertex(65, 88);
endShape();
stroke(57, 99, 71); //lines on leaf, dark green color
line(65, 88, 77, 96);
line(68, 92, 67, 90);
line(67, 90, 71, 89);
line(70, 91, 73, 91);
line(70, 91, 72, 94.5);
line(72, 92, 75, 93);
fill(74, 49, 31); //vertical base of tree on left
stroke(74, 49, 31);
rect(1, 0, 10, 90); //left side of function
rect(85, 0, 5, 95); //right side of function
stroke(44, 19, 11); //lines of tree base
line(3, 2, 3, 15);
line(7, 4, 7, 31);
line(1.5, 38, 1.5, 68);
line(0, 75, 0, 115);
//koala base body
fill(138);
stroke(0);
circle(6, 80, 10); //tail
fill(138);
stroke(0);
beginShape(); //connecting body points
curveVertex(20, 47);
curveVertex(20, 47);
curveVertex(18, 48);
curveVertex(15, 50);
curveVertex(10, 53);
curveVertex(14, 56);
curveVertex(12, 59);
curveVertex(9, 63);
curveVertex(7, 68);
curveVertex(5.5, 72);
curveVertex(5.3, 77);
curveVertex(5.4, 81);
curveVertex(5.8, 83);
curveVertex(6.2, 85);
curveVertex(6.5, 86);
curveVertex(6.5, 86);
curveVertex(10, 89);
curveVertex(14, 90);
curveVertex(17, 90);
curveVertex(20, 89);
curveVertex(24, 90);
curveVertex(27, 89);
curveVertex(31, 90);
curveVertex(33, 90);
curveVertex(36, 89);
curveVertex(38, 90);
curveVertex(40, 90);
curveVertex(43, 89);
curveVertex(43, 86);
curveVertex(41, 83);
curveVertex(43, 70);
curveVertex(43, 60);
curveVertex(43, 48);
curveVertex(43, 48);
endShape();
noFill(); //hind leg outline
beginShape();
curveVertex(13, 75);
curveVertex(13, 75);
curveVertex(17, 74);
curveVertex(21, 76);
curveVertex(22, 79);
curveVertex(22, 82);
curveVertex(21, 84);
curveVertex(25, 86);
curveVertex(25, 87);
curveVertex(26, 89);
curveVertex(26, 89);
endShape();
beginShape(); //part of the right front leg outline
curveVertex(22, 75);
curveVertex(22, 75);
curveVertex(23, 79);
curveVertex(25, 83);
curveVertex(26, 85);
curveVertex(26, 87);
curveVertex(26, 87);
endShape();
beginShape(); //line separating front two legs
curveVertex(31, 70);
curveVertex(31, 70);
curveVertex(33, 78);
curveVertex(33, 81);
curveVertex(33, 83);
curveVertex(33, 85);
curveVertex(35, 87);
curveVertex(36, 89);
curveVertex(36, 89);
endShape();
//light grey stomach patch of fur
fill(210);
noStroke();
triangle(30, 65, 33, 76, 43, 60);
stroke(0);
beginShape();
curveVertex(33, 75);
curveVertex(33, 75);
curveVertex(35, 74);
curveVertex(37, 72);
curveVertex(39, 75);
curveVertex(40, 71);
curveVertex(42, 74);
curveVertex(42, 70);
curveVertex(45, 70);
curveVertex(42, 66);
curveVertex(44, 67);
curveVertex(43, 60);
curveVertex(43, 60);
endShape();
// koala base head black outline
stroke(0);
strokeWeight(1);
ellipse(28, 51, 40, 26);
ellipse(28, 45, 41, 36);
circle(10, 36, 19);
circle(48, 36, 19);
noStroke();
// koala base head no outline
fill(140);
ellipse(28, 51, 39, 25);
ellipse(28, 45, 40, 35);
circle(10, 36, 18); //left ear
circle(48, 36, 18); //right ear
//hair patch at top of forehead
stroke(0);
strokeWeight(0.5);
beginShape();
curveVertex(23, 37);
curveVertex(23, 37);
curveVertex(24, 33);
curveVertex(26, 36);
curveVertex(28, 31);
curveVertex(30, 36);
curveVertex(32, 33);
curveVertex(33, 37);
curveVertex(33, 37);
endShape();
//koala face parts below
//light color patch under nose and mouth
noStroke();
fill(210);
ellipse(28, 56, 10, 12);
//light color part of ears
circle(10, 37, 10);
circle(48, 37, 10);
//covering part of the ears to make arc shape
fill(140);
circle(15, 40, 11);
circle(43, 40, 11);
//nose
fill(30);
ellipse(28, 54, 9, 9.5);
stroke(30);
strokeWeight(1);
arc(28, 59, 6, 3, 0, PI, CHORD);
//eyes
fill(0);
circle(20, 47, 4);
circle(36, 47, 4);
fill(255); // white shine of eyes
noStroke();
circle(20.5, 46, 1.5);
circle(36.5, 46, 1.5);
//leaf in mouth
//stem
stroke(43, 76, 45);
noFill();
arc(34, 61, 30, 5, PI, 0, OPEN);
//petals of flower
fill(239, 217, 221);
stroke(255, 180, 190);
circle(48, 56, 8);
circle(45, 60, 8);
circle(48, 63, 8);
circle(53, 61, 8);
circle(52, 57, 8);
fill(255); //white center part of flower
circle(49, 59, 7);
}
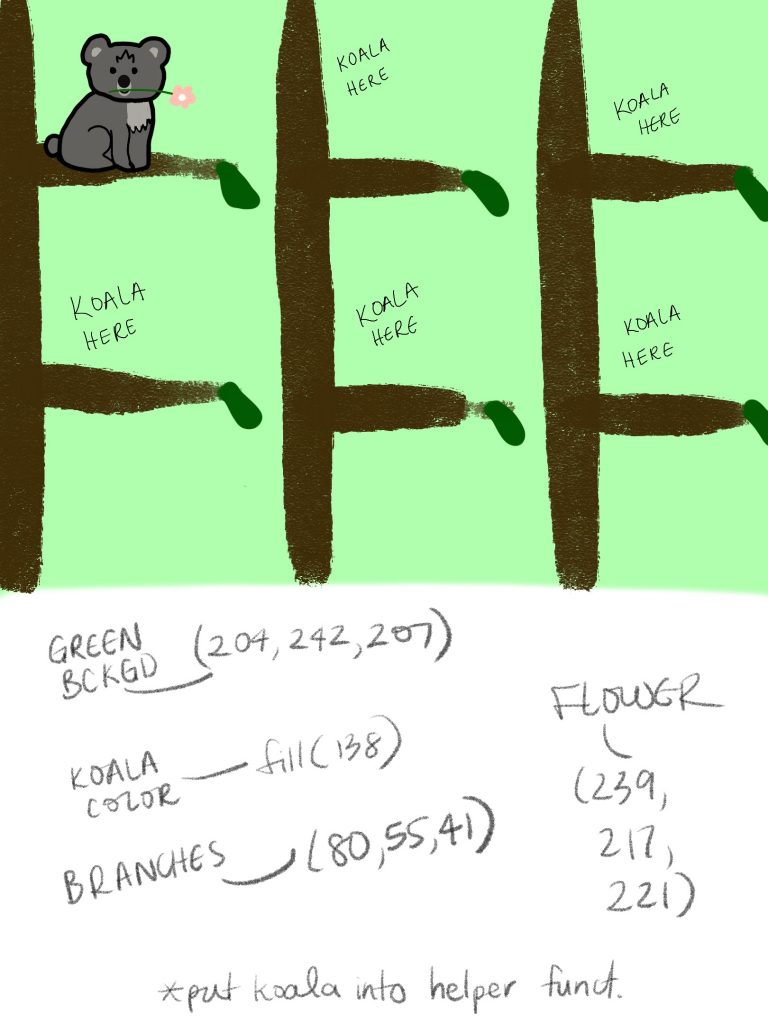