Heying Wang
heyingw@andrew.cmu.edu
var nPoints=100;
function setup() {
createCanvas(480, 480);
background(220);
frameRate(5);
}
function draw() {
var x;
var y;
//constrain mouseX and mouseY
constrain(mouseX,0,width);
constrain(mouseX,0,height);
//limite the size of the astroid with map method
//sets up a constantly chaging background
var a = map(mouseX,0,width,20,100);
var b= map(mouseY,0,height,20,100);
background(100+a,150-b,100+b-a);
for(i=0;i<=200;i++){
fill('yellow');
circle(random(width),random(height),1,1)
}
//draw one large astroid at the center
fill(a,b,50);
translate(width/2+random(-2,2),height/2+random(-2,2));
astroid(a,b);
fill(random(250),random(250),random(250));
heart(a);
push();
//the one on the bottom right
translate(width/4+random(-2,2),height/4+random(-2,2));
rotate(radians(mouseX));
fill(a,b,160);
astroid(a,b);
fill(random(250),random(250),random(250));
heart(a);
//the one on the top left
pop();
push();
translate(-width/4+random(-2,2),-height/4)+random(-2,2);
rotate(radians(mouseX));
fill(a,b,160);
astroid(a,b);
fill(random(250),random(250),random(250));
heart(a);
//the one on the top right
pop();
pop();
push();
translate(width/4+random(-2,2),-height/4)+random(-2,2);
rotate(radians(mouseX));
fill(a,b,160);
astroid(a,b);
fill(random(250),random(250),random(250));
heart(a);
//the one on the bottom left
pop();
pop();
translate(-width/4+random(-2,2),height/4)+random(-2,2);
rotate(radians(mouseX));
fill(a,b,160);
astroid(a,b);
fill(random(250),random(250),random(250));
heart(a);
}
//draw astroid and the lines
function astroid(a,b){
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = a*pow(cos(t),3);
y = b*pow(sin(t),3);
vertex(x, y);
}
endShape(CLOSE);
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = a*pow(cos(t),3);
y = b*pow(sin(t),3);
strokeWeight(0.2);
stroke('wight');
line(x,y,0,0);
}
}
//draw heart
function heart(a){
k=map(a,0,100,0.5,1.2);
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x =k*16*pow(sin(t),3);
y = k*13/16*13*cos(t)-5*cos(2*t)-2*cos(3*t)-cos(4*t);
vertex(x, y);
}
endShape(CLOSE);
}
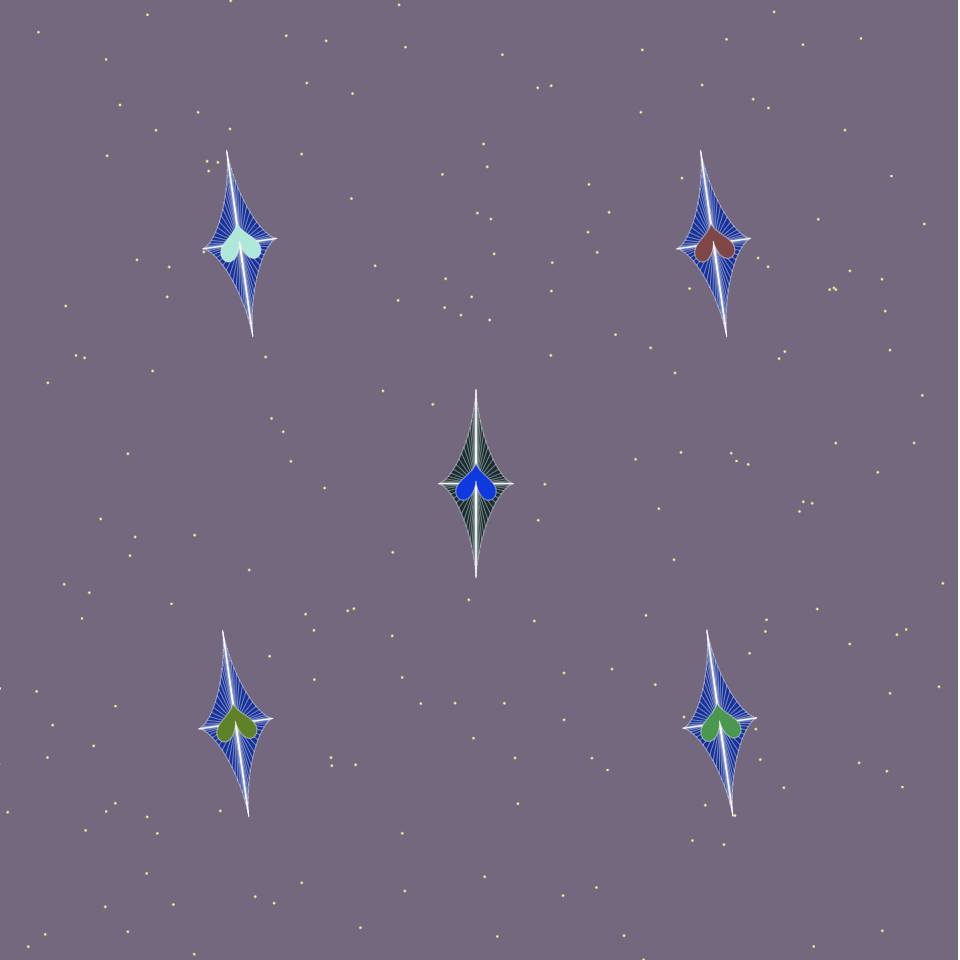
I made this constantly changing pattern with hearts curve and astroid curve. The background color corresponds to the position of mouse x and mouse y.
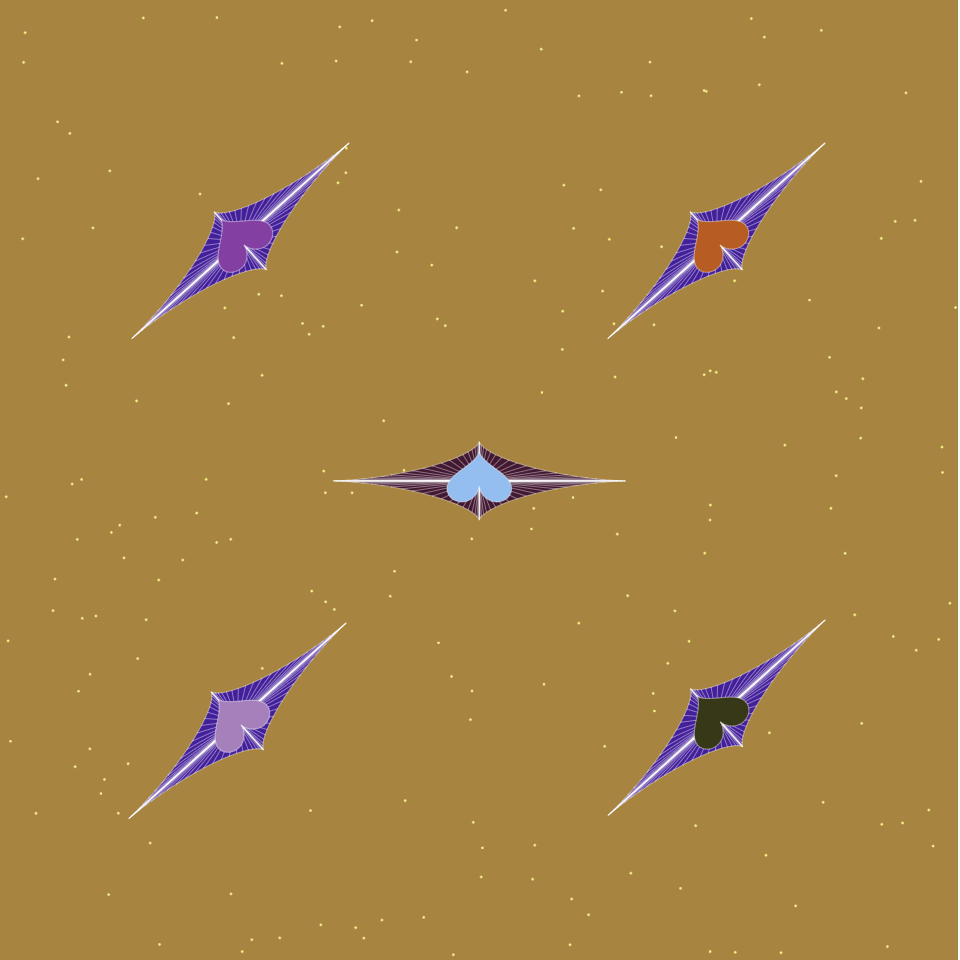
The size of the astroid curve and the heart curve also depends on where the user places he mouse. They can become as narrow as a pointer or as wide as a square. They will also be rotating around the center as the mouse move clockwise or anti-clockwise.
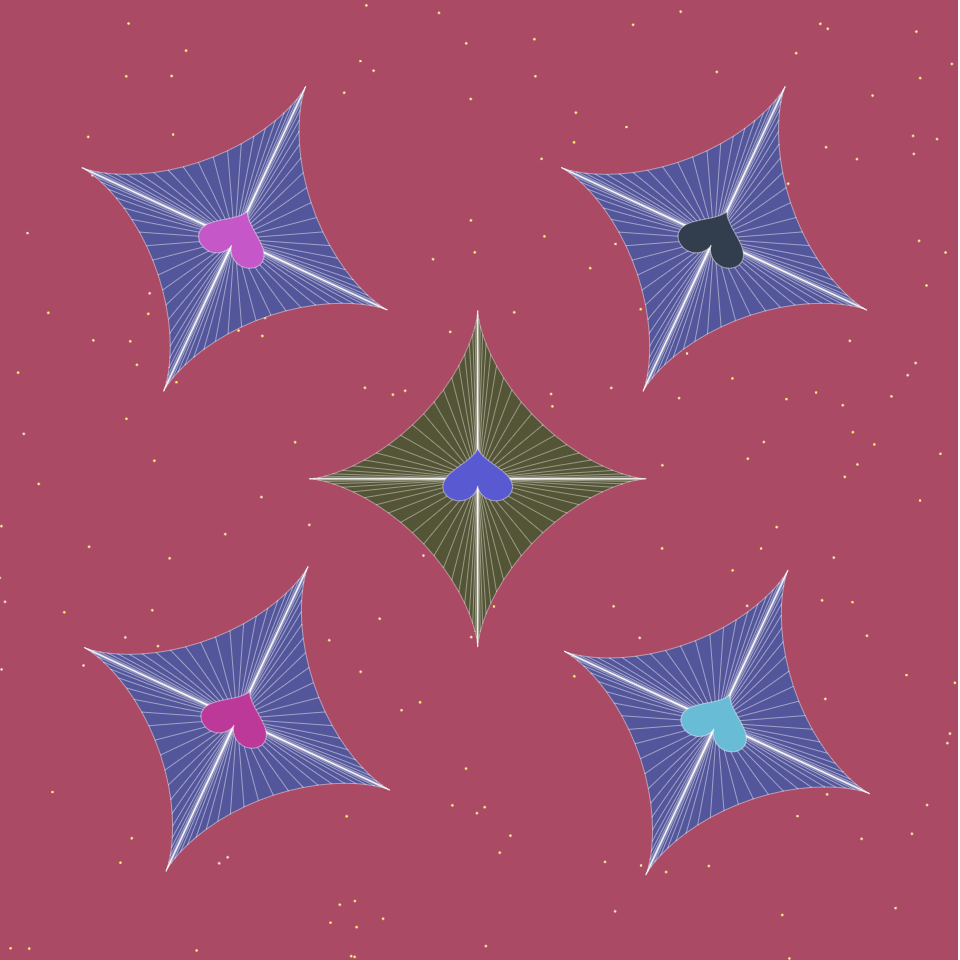
Thus, the user can interact with the page by moving their mouse around to create their unique, desired pattern.
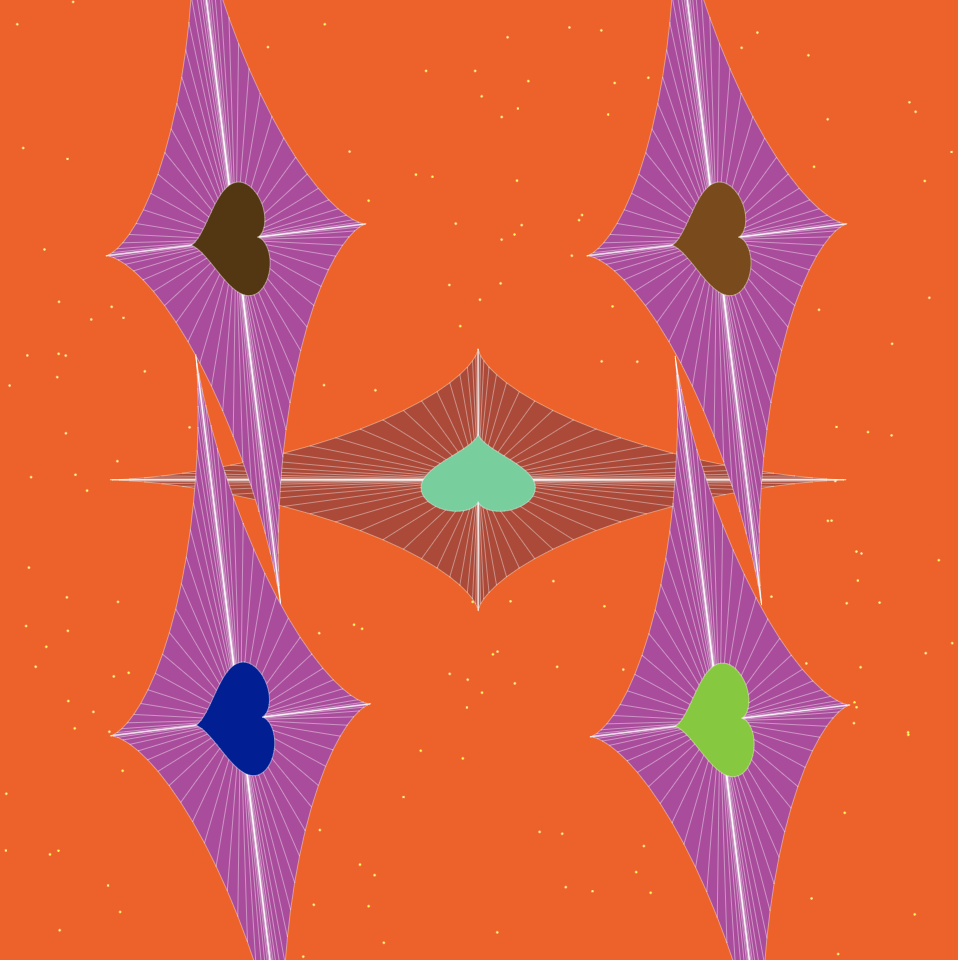