sketch
// Jisoo Geum
// Section B
// jgeum@andrew.cmu.edu
// Project-10
//global variables
var thethings = [];
var boomSpeed = 0.005;
var boomDetail = 0.05;
function setup() {
createCanvas(480,250);
// create an initial collection of thethings
for (var i = 0; i < 10; i++){
var rx = random(width);
thethings[i] = makeThethings(rx);
}
frameRate(10);
}
function draw() {
//background(70,223,191);
background(0);
makeBooms();
//display the things (ellipses)
updateAndDisplayThethings();
removeThethingsThatHaveSlippedOutOfView();
addNewThethingsWithSomeRandomProbability();
//display speaker
makeSpeaker();
}
function makeBooms(){
noStroke();
/*
//1st boom
//fill(51,194,248);
//fill(0);
noFill();
strokeWeight(1);
//stroke(160,240,19);
stroke(247,223,90);
beginShape();
vertex(-10,height);
for (var x = 0; x < width; x++) {
var t = (x * boomDetail*3) + (millis() * boomSpeed*20);
var y = map(noise(t), 0, 1 , 200, height);
//4th num ++=more straight, --= more abrubt
//last num ++=lower, --= higher
vertex(x ,y/6); // y++ = lower, y -- = higher
}
vertex(width+10,height);
endShape();
//2nd boom
fill(0);
//noFill();
strokeWeight(1.2);
//stroke(160,240,19);
stroke(70,223,191);
beginShape();
vertex(-10,height);
for (var x = 0; x < width; x++) {
var t = (x * boomDetail) + (millis() * boomSpeed*20);
var y = map(noise(t), 0, 1 , 200, height);
//4th num ++=more straight, --= more abrubt
//last num ++=lower, --= higher
vertex(x ,y/4); // y++ = lower, y -- = higher
}
vertex(width+10,height+10);
endShape();
*/
// 3rd boom
fill(160,240,19);
//noFill();
strokeWeight(5);
//stroke(160,240,19); // green
stroke(70,223,191);
beginShape();
vertex(-10,height);
for (var x = 0; x < width; x++) {
var t = (x * boomDetail) + (millis() * boomSpeed*20);
var y = map(noise(t), 0, 1 , 100 , height);
//4th num ++=more straight, --= more abrubt
//last num ++=lower, --= higher
vertex(x ,y/2); // y++ = lower, y -- = higher
}
vertex(width+10,height);
endShape();
// 4th boom
//fill(70,223,191); turqoise
fill(0);
//noFill();
strokeWeight(3);
stroke(160,240,19); green
//stroke(254,112,99); // orange
//stroke(255);
beginShape();
vertex(-10,height);
for (var x = 0; x < width; x++) {
var t = (x * boomDetail+10) + (millis() * boomSpeed*20);
var y = map(noise(t), 0, 1 , 0 , height-90);
//4th num ++=more straight, --= more abrubt
//last num ++=lower, --= higher
vertex(x ,y); // y++ = lower, y -- = higher
}
vertex(width+10,height*2);
endShape();
}
function updateAndDisplayThethings(){
// Update thething's positions, and display them.
for (var i = 0; i < thethings.length; i++){
thethings[i].move();
thethings[i].display();
}
}
function removeThethingsThatHaveSlippedOutOfView(){
var thethingsToKeep = [];
for (var i = 0; i < thethings.length; i++){
if (thethings[i].x + thethings[i].breadth > 0) {
thethingsToKeep.push(thethings[i]);
}
}
thethings = thethingsToKeep; // remember the surviving things
}
function addNewThethingsWithSomeRandomProbability() {
// With a very tiny probability, add a new thing to the end.
var newThethingsLikelihood = 0.007;
if (random(0,1) < newThethingsLikelihood) {
thethings.push(makeThethings(width));
}
}
// update position of thethings every frame
function thethingsMove() {
this.x += this.speed;
}
// draw the thethings
function thethingsDisplay() {
var floorHeight = 10;
var thethingsX = random(0,300);
var size1 = random(5,25);
var size2 = random(2,20);
var size3 = random(15,30);
var bHeight = this.nFloors * floorHeight;
var transheight = random(20,height)
// making the things
push();
translate(this.x, height - 50);
noFill();
stroke(160,240,19);
strokeWeight(1.5);
ellipse(thethingsX, -bHeight/4, size1 , size1);
stroke(70,223,191);
ellipse(thethingsX, -bHeight/4, size2 , size2);
//stroke(80,93,255);
//ellipse(thethingsX, -bHeight+95, size3 , size3);
//line(thethingsX, -bHeight+90,thethingsX+20, -bHeight+90,)
pop();
}
function makeThethings(birthLocationX) {
var tt = {x: birthLocationX,
breadth: .8,
speed: -1,
nFloors: round(random(10,12)),
move: thethingsMove,
display: thethingsDisplay}
return tt;
}
function makeSpeaker (){
// the ellipses
/* strokeWeight(0.5);
stroke(160,240,19);
noFill();
ellipse(width/2, height-50,250,40);
ellipse(width/2, height-50,270,47);
ellipse(width/2, height-50,290,55);
ellipse(width/2, height-50,310,62);
ellipse(width/2, height-50,330,68);
ellipse(width/2, height-50,350,73);
ellipse(width/2, height-50,370,77);
*/
noStroke();
fill(244,93,255); // pink pill shape
rectMode(CENTER);
rect(width/2,height-80,235,60,30);
fill(80,93,255); // blue
rect(width/2,height-80, 40, 60 ); // blue middle part
noFill();
strokeWeight(6);
stroke(244,93,255); // pink 'b'
ellipse(width/2,height-75,15,15);
line(232,height-95,232,height-75);
stroke(80,93,255); // the O s
ellipse(150,height-80,25,25);
ellipse(190,height-80,25,25);
ellipse(285,height-80,25,25);
ellipse(325,height-80,25,25);
strokeWeight(5);
line(160,220,180,220); // -
line(290,220,310,220); //+
line(300,210,300,230); //+
}
This project was very fun but also challenging for me since the concept was open-ended. The terrain reminded me of the waves of sound so I decided to create a ‘landscape of sound’. The result did not come out like my initial idea, but I enjoyed the process of choosing vibrant colors. I didn’t delete some of the codes I previously wrote so that I (or anyone) can try different variations of the landscape in the future.
These are some of the different styles I tried.
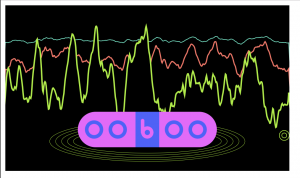
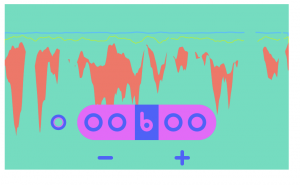
My initial sketch in Adobe Illustrator.
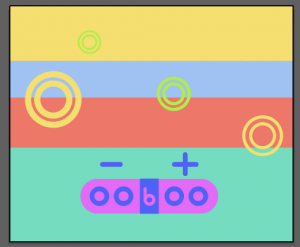