var highMountain = [];
var lowMountain = [];
var cabin = [];
var trees = [];
var dogImage = [];
var dog = [];
var noiseParam = 0;
var noiseStep = 0.01;
function preload(){
var filenames = [];
filenames[0] = "https://i.imgur.com/KGHR8lv.png";
filenames[1] = "https://i.imgur.com/KGHR8lv.png";
filenames[2] = "https://i.imgur.com/unhcm3R.png";
filenames[3] = "https://i.imgur.com/unhcm3R.png";
filenames[4] = "https://i.imgur.com/J2pZbow.png";
filenames[5] = "https://i.imgur.com/J2pZbow.png";
filenames[6] = "https://i.imgur.com/unhcm3R.png";
filenames[7] = "https://i.imgur.com/unhcm3R.png";
for (var i = 0; i<filenames.length; i++) {
dogImage[i] = loadImage(filenames[i]);
}
}
function setup() {
createCanvas(480, 240);
frameRate(24);
imageMode(CENTER);
//mountains
for (var i = 0; i <= width/4; i++) {
var value = map(noise(noiseParam), 0, 1, 0, height*1.1);
highMountain.push(value);
noiseParam += noiseStep;
}
for (var i = 0; i <= width/5; i++) {
var value = map(noise(noiseParam), 0, 1, 0, height*1.9);
lowMountain.push(value);
noiseParam += noiseStep;
}
// create an initial collection of cabins
for (var i = 0; i < 10; i++){
var rx = random(width);
cabin[i] = makeCabin(rx);
var r = random(width);
trees[i] = makeTree(r);
}
//create three walking characters
var d = makeCharacter(400, 200);
dog.push(d);
}
function draw() {
background(200);
//sky as a gradient
var gradPurple = color(134, 123, 198);
var gradPink = color(240, 198, 209);
for (var i = 0; i < height; i++){
var gradient = map (i, 0, (height/3)*1.5, 0, 1);
var bgColor = lerpColor(gradPurple, gradPink, gradient);
stroke(bgColor);
line(0, i, width, i);
}
//snow
stroke(239, 241, 253);
strokeWeight(2);
for (var i = 0; i < 750; i++) {
point(random(0,width), random(-0, height));
}
//sun
noStroke();
fill(242, 226, 233, 90);
circle(width/2, height/2-40, 60);
fill(242, 226, 233, 75);
circle(width/2, height/2-40, 50);
fill(242, 226, 233, 60);
circle(width/2, height/2-40, 40);
fill(242, 226, 233);
circle(width/2, height/2-40, 30);
//clouds
cloud(45, 80);
cloud(180, 130);
cloud(360, 160);
cloud(445, 70);
//mountains
drawHighMountain();
drawLowMountain();
//snow on the ground
fill(239, 241, 253);
rect(0, 180, width, 60);
//trees
updateAndDisplayTrees();
removeTreesThatHaveSlippedOutOfView();
addNewTreesWithSomeRandomProbability();
//cabins
updateAndDisplayCabins();
removeCabinsThatHaveSlippedOutOfView();
addNewCabinsWithSomeRandomProbability();
//walking dog
for (var i = 0; i < dog.length; i++) {
var d = dog[i];
d.stepFunction();
d.drawFunction();
}
//fence
for (var x = 10; x <= width-10; x+=10) {
stroke(198, 173, 203);
strokeWeight(5);
line(x, height, x, height-25);
}
line(0, height-15, width, height-15);
}
function drawHighMountain() {
highMountain.shift();
var value = map(noise(noiseParam), 0, 1, 0, height*1.1);
highMountain.push(value);
noiseParam += noiseStep;
push();
fill(170, 190, 250);
stroke(170, 190, 250);
beginShape();
vertex(0, height);
for (var i = 0; i < width/4; i++) {
vertex(i*5, highMountain[i]);
vertex((i+1)*5, highMountain[i+1]);
}
vertex(width, height);
endShape();
pop();
}
function drawLowMountain() {
lowMountain.shift();
var value = map(noise(noiseParam), 0, 1, 0, height*1.9);
lowMountain.push(value);
noiseParam += noiseStep;
push();
fill(193, 205, 246);
stroke(193, 205, 246);
beginShape();
vertex(0, height);
for (var i = 0; i < width/5; i++) {
vertex(i*5, lowMountain[i]);
vertex((i+1)*5, lowMountain[i+1]);
}
vertex(width, height);
endShape();
pop();
}
//cabins
function makeCabin(birthLocationX) {
var bldg = {x: birthLocationX,
breadth: 70,
speed: -5.0,
nFloors: round(random(2,8)),
move: cabinMove,
display: cabinDisplay}
return bldg;
}
// method to update position of cabin every frame
function cabinMove() {
this.x += this.speed;
}
// draw the cabin and some windows
function cabinDisplay() {
var floorHeight = 8;
var cHeight = this.nFloors * floorHeight;
noStroke();
fill(95, 124, 213);
push();
translate(this.x, height - 40);
//chimmney
rect(this.breadth-10, -cHeight - 20, 10, 15);
//roof
stroke(239, 241, 253);
triangle(-7,-cHeight,this.breadth/2,-cHeight-20,this.breadth+7,-cHeight);
//home
stroke(95, 124, 213);
rect(0, -cHeight, this.breadth, cHeight);
fill(239, 241, 253);
noStroke();
circle(this.breadth/2, -cHeight - 10, 10);
rect(this.breadth-10, -cHeight - 20, 10, 5);
//windows
fill(148, 178, 249);
stroke(95, 124, 213);
for (var i = 0; i < this.nFloors-1; i++) {
rect(5, -15 - (i * floorHeight), this.breadth - 10, 10);
}
pop();
}
function updateAndDisplayCabins(){
// Update the cabin's positions, and display them.
for (var i = 0; i < cabin.length; i++){
cabin[i].move();
cabin[i].display();
}
}
function removeCabinsThatHaveSlippedOutOfView(){
var cabinToKeep = [];
for (var i = 0; i < cabin.length; i++){
if (cabin[i].x + cabin[i].breadth > 0) {
cabinToKeep.push(cabin[i]);
}
}
cabin = cabinToKeep; // remember the surviving cabin
}
function addNewCabinsWithSomeRandomProbability() {
// With a very tiny probability, add a new cabin to the end.
var newCabinLikelihood = 0.01;
if (random(0,1) < newCabinLikelihood) {
cabin.push(makeCabin(width));
}
}
// dog walking character
function makeCharacter(cx, cy) {
c = {x: cx, y: cy,
imageNumber: 0,
stepFunction: characterStep,
drawFunction: characterDraw
}
return c;
}
function characterStep() {
this.imageNumber++;
if (this.imageNumber == 8) {
this.imageNumber = 0;
}
}
function characterDraw() {
image(dogImage[this.imageNumber], this.x, this.y);
}
// trees
function makeTree(birthLocationX) {
var t = {x: birthLocationX,
breadth: round(random(20,30)),
speed: -5.0,
treeHeight: round(random(30,70)),
move: treeMove,
display: treeDisplay}
return t;
}
function treeMove() {
this.x += this.speed;
}
function treeDisplay() {
push();
translate(this.x, height - 60);
noStroke();
fill(153, 139, 196);
rect(-2, 0, 6, 8);
fill(242, 198, 210);
triangle(0, 0, 0, -this.treeHeight, -this.breadth/2, 0);
fill(223, 186, 209);
triangle(0, 0, 0, -this.treeHeight, this.breadth/2, 0);
stroke(225);
line(0, 0, 0, -this.treeHeight);
pop();
}
function updateAndDisplayTrees(){
for (var i = 0; i < trees.length; i++){
trees[i].move();
trees[i].display();
}
}
function removeTreesThatHaveSlippedOutOfView(){
var treesToKeep = [];
for (var i = 0; i < trees.length; i++){
if (trees[i].x + trees[i].breadth > 0) {
treesToKeep.push(trees[i]);
}
}
trees = treesToKeep;
}
function addNewTreesWithSomeRandomProbability() {
var newTreeLikelihood = 0.07;
if (random(0,1) < newTreeLikelihood) {
trees.push(makeTree(width));
}
}
function cloud(x, y) {
fill(239, 241, 253, 50);
arc(x, y, 60, 40, PI + TWO_PI, TWO_PI);
arc(x + 30, y, 90, 90, PI + TWO_PI, TWO_PI);
arc(x + 25, y, 40, 70, PI + TWO_PI, TWO_PI);
arc(x + 50, y, 70, 40, PI + TWO_PI, TWO_PI);
}
For this project, I wanted to created a snowy landscape. I started by drawing two layers of mountains and then added the cabins, the trees, and the running dog. I feel like the cool tone color palette contrasts really well with the dog. The sun and clouds looked pretty plain so I played with the opacity of the shapes to create some visual interest. The hardest part was figuring out how to do the gradient, but I’m happy with the way that it turned out and the way it looks with the snow! I didn’t want to overcrowd the piece too much so the last thing I added was a fence.
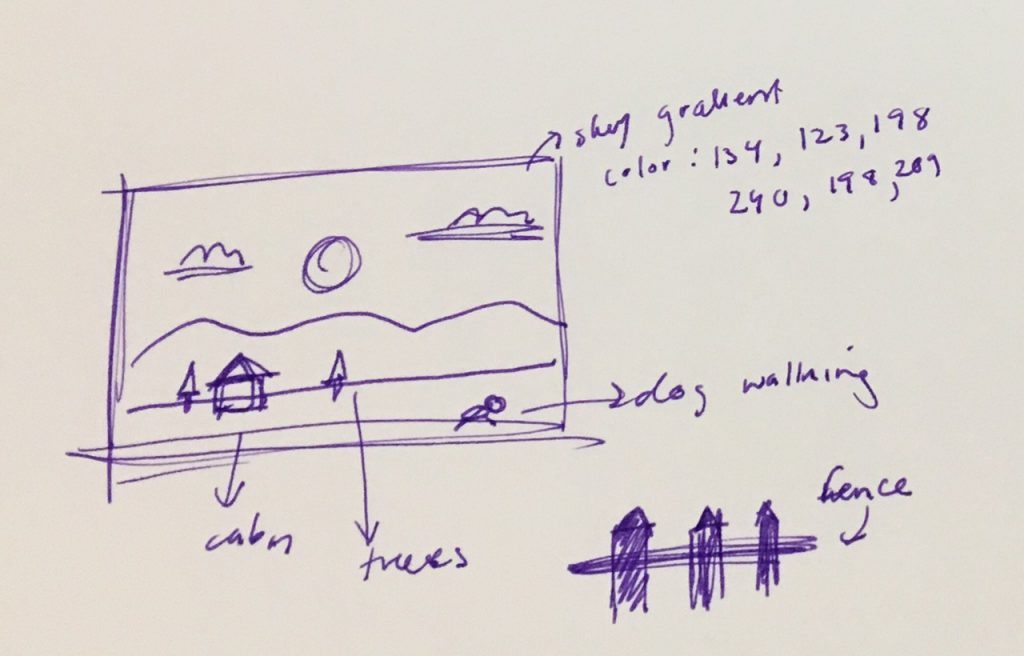