For this Looking Outwards, I’ve decided to look into Lauren. I first got interested in her because I saw this description saying, creator/lead-developer of p5.js. One of her works that caught my eye was the LAUREN, in which she attempted to become a human version of Amazon Alexa. It is an installation of a series of custom-designed networked smart devices like camera, door locks, electronic functions, switches, and etc. She claimed that her performance was better than an AI because she can understand the user as a person and anticipate their needs. It was interesting for me because recently there’s a lot of tryout going on to create AI devices to make people’s life easier. LAUREN was an improved version of current AIs except for empathy, that only humans can do.
Month: November 2020
Project 11: Generative Landscape
var clouds = [];
var bikeImage = [];
var bike = [];
var terrainCurve = 0.01;
var terrainSpeed = 0.001;
function preload(){
var filenames =[];
filenames[0] = "https://i.imgur.com/DSPjyoM.png";
filenames[1] = "https://i.imgur.com/kGGBLDh.png";
filenames[2] = "https://i.imgur.com/v9RMtPT.png";
filenames[3] = "https://i.imgur.com/ZUC5xZ2.png";
for(var i = 0; i < filenames.length; i++){
bikeImage.push(loadImage(filenames[i]));
}
}
function setup() {
createCanvas(480,480);
//clouds
for (var i = 0; i < 9; i++){
var ccloud = random(width);
clouds[i] = drawCloud(ccloud);
}
frameRate(11);
imageMode(CENTER);
//bike
var b = makeCharacter(240, 350);
bike.push(b);
}
function draw() {
sky(0, 0, width, height);
DisplayClouds();
removeClouds();
newClouds();
ground();
for (var i = 0; i < bike.length; i++) {
var bb = bike[i];
bb.stepFunction();
bb.drawFunction();
}
}
function sky(x, y, w, h) {
//sky
var Blue;
var Pink;
Blue = color(114, 193,215);
Pink = color(253, 209, 164);
for (var i = y; i <= y + h; i++) {
var ssky = map(i, y, y + h, 0, 1.1);
var cco = lerpColor(Blue, Pink, ssky);
stroke(cco);
line(x, i, x + w, i);
}
//mountains
beginShape();
stroke(1, 33,92);
strokeWeight(200);
for (var x = 0; x < width; x++){
var tt = (x * terrainCurve) + (millis() * terrainSpeed);
var mm = map(noise(tt), 0,1, 110, 255);
vertex(x, mm + 180);
}
endShape();
}
this.display = function() {
strokeWeight(this.border);
stroke(240);
fill(240);
ellipse(this.x, this.y, this.display, this.display);
}
function ground(){
beginShape();
noStroke();
fill(18,12,46);
rect(0,400,width,80);
endShape();
}
function cloudDisplay(){
noStroke();
var floorH = 21;
var cloudH = this.nFloors * floorH;
fill(250,50);
push();
translate(this.x, height - 95);
ellipse(95, -cloudH, this.breadth, cloudH / 2);
pop();
push();
fill(250,80)
translate(this.x, height - 220);
ellipse(95, - cloudH, this.breadth/1.5, cloudH);
pop();
}
function DisplayClouds(){
for (var i = 0; i < clouds.length; i++){
clouds[i].move();
clouds[i].display();
}
}
function cloudMove(){
this.x += this.speed;
}
function removeClouds(){
var cKeep = [];
for (var i = 0; i < clouds.length; i++){
if (clouds[i].x + clouds[i].breadth > 0) {
cKeep.push(clouds[i]);
}
}
clouds = cKeep;
}
function newClouds(){
//random probability
var newP = 0.01;
if (random(0,0.4) < newP) {
clouds.push(drawCloud(width));
}
}
function drawCloud(xx) {
var cha = {x: xx,
breadth: random(95, height),
speed: -random(1,4),
nFloors: round(random(4,8)),
move: cloudMove,
display: cloudDisplay}
return cha;
}
function makeCharacter(cx, cy) {
ccha = {x: cx, y: cy,
imageNumber: 0,
stepFunction: characterStep,
drawFunction: characterDraw
}
return ccha;
}
function characterStep() {
this.imageNumber++;
if (this.imageNumber == 4) {
this.imageNumber = 0;
}
}
function characterDraw() {
image(bikeImage[this.imageNumber], this.x, this.y,120,120);
}
For this Deliverable 11 project, I tried to collaborate on what I’ve learned from deliverable 9, using preloads for calling image animations and generating landscapes from deliverable 11. I also tried to show the difference in the speed by giving a certain frameRate for the rider and background mountains. Clouds are displayed with low opacity and different speed so that it shows how it has a different relationship with elements on the ground(mountains and humans). It was hard for me to figure out having two functions of makeCharacter because I had two figures to move: character, and the elements in the sky.
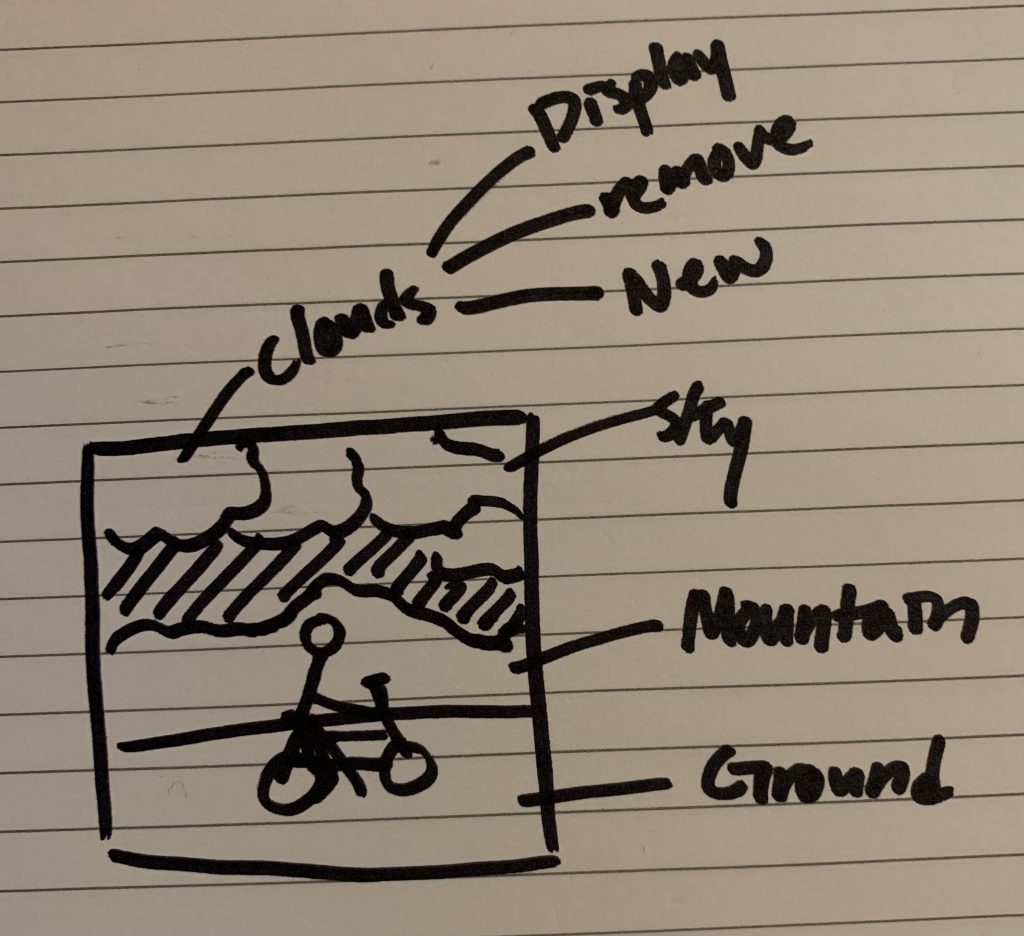
Project 11
var x = 0;
var backgroundValue = [];
var noiseParam = 0;
var noiseStep = 0.1;
function setup() {
createCanvas(480, 400);
frameRate(15);
var ilength = (width/5) + 1
for (i = 0; i < ilength; i++) {
var n = noise(noiseParam);
var value = map(n, 0, 1, 0, height);
backgroundValue.push(value);
noiseParam += noiseStep
}
}
function draw() {
var size = random(50, 150);
background(166, 238, 255);
stroke(160, 160, 255);
strokeWeight(10);
backgroundValue.shift();
var x2 = 0;
var ilength = (width/5) + 1
var n = noise(noiseParam);
var value = map(n, 0, 1, 0, height);
backgroundValue.push(value);
noiseParam += noiseStep
for (i = 0; i < ilength; i++) {
beginShape();
curveVertex(x2, backgroundValue[i]);
curveVertex(x2, backgroundValue[i]);
curveVertex(x2, height);
curveVertex(x2 + 5, backgroundValue[i + 1]);
curveVertex(x2 + 5, backgroundValue[i + 1]);
endShape(CLOSE);
x2 += 5
}
noStroke();
fill(color(random(255), random(255), random(255)));
coral(x, size);
x += 1
if (x > 480) {
x = 0;
}
}
function coral(x, size) {
beginShape();
curveVertex(x, 400);
curveVertex(x, 400);
curveVertex(x + 5, size*3);
curveVertex(x - 20, size*2);
curveVertex(x + 5, size*2 - 5);
curveVertex(x - 10, size);
curveVertex(x, size + 5);
curveVertex(x + 10, size*2 - 20);
curveVertex(x + 20, size*3);
curveVertex(x + 20, size*4);
curveVertex(x + 70, size);
curveVertex(x + 80, size*2);
curveVertex(x + 50, 400);
curveVertex(x + 50, 400);
endShape(CLOSE);
}
Project 11: Landscape
For this week’s project, I really wanted to get away from the craziness of the semester and portray the calming view from the beach house my cousins and I used to go to. I have really vivid memories of sitting on the porch, eating fruit while watching the fireflies and water wade in and out at nighttime: something I wish I could be doing right now.
// Susie Kim
// susiek@andrew.cmu.edu
// Section A
// Project 11
// set global variables
var landSpeed = 0.001;
var landFlow = 0.005;
var firefly = [];
var fWidth = 50;
var fLeft = 27 - fWidth;
// load in images for background
function preload() {
feet = loadImage('https://i.imgur.com/VLK5brn.png');
fruit = loadImage('https://i.imgur.com/NNwEjtA.png');
cloud = loadImage('https://i.imgur.com/m6zIO0u.png');
}
function setup() {
createCanvas(400, 480);
background(29, 51, 81);
imageMode(CENTER);
// create fireflies at random locations on canvas
for (var i = 0; i < 7; i++) {
var xLocation = random(20, 380);
var yLocation = random(0, 360);
firefly[i] = makeFireflies(xLocation, yLocation);
}
}
function draw() {
background(29, 51, 81);
image(cloud, width/2, height/2-65, width-20, height-20); // background cloud image
// blue water
fill(147, 169, 209);
noStroke();
rect(0, 200, 400, 200);
// call upon functions to draw land, foreground, and update fireflies
land();
createForeground();
updateFirefly();
}
// draws front foreground porch, feet, and fruit dish
function createForeground() {
fill(188, 158, 130);
strokeWeight(1);
stroke(124, 87, 61);
// create porch base
rect(0, 360, 400, 120);
rect(0, 350, 400, 10);
rect(0, 0, 30, 355);
rect(370, 0, 30, 355);
// insert self-drawn images of feet laying on porch and fruit
image(feet, 100, 405, 150, 150);
image(fruit, 300, 410, 125, 125);
}
// draws undulating sand landscape
function land() {
fill(249, 228, 183);
stroke(255);
strokeWeight(8);
beginShape();
vertex(0, height);
// undulating line of sandscape
for (var x = 0; x < width; x++) {
var a = (x*landFlow) + (millis()*landSpeed);
var y = map(noise(a), 0, 1, height*12/20, height*11/20);
vertex (x, y);
}
vertex(width, height);
endShape();
}
// draws fireflies
function drawFirefly() {
fill(255, 245, 0, 100);
noStroke();
push();
translate(this.x1, this.y1); // allows fireflies to move depending on function
scale(this.fireflySize); // allows fireflies to be scalable depending on make function
ellipse(50, 50, 10, 10);
ellipse(50, 50, 5, 5);
pop();
}
// summons fireflies at given location with variables
function makeFireflies(xlocation, ylocation) {
var makeFirefly = {x1: xlocation,
y1: ylocation,
fireflySize: random(0.25, 2),
speed: -1,
move: moveFirefly,
draw: drawFirefly}
return makeFirefly;
}
// makes fireflies move across the page
function moveFirefly() {
this.x1 += this.speed;
if (this.x1 <= fLeft) {
this.x1 += width - fLeft;
}
}
// updates the array of fireflies
function updateFirefly() {
for (i = 0; i < firefly.length; i++) {
firefly[i].move();
firefly[i].draw();
}
}
Here is the main sketch that I did for this project:
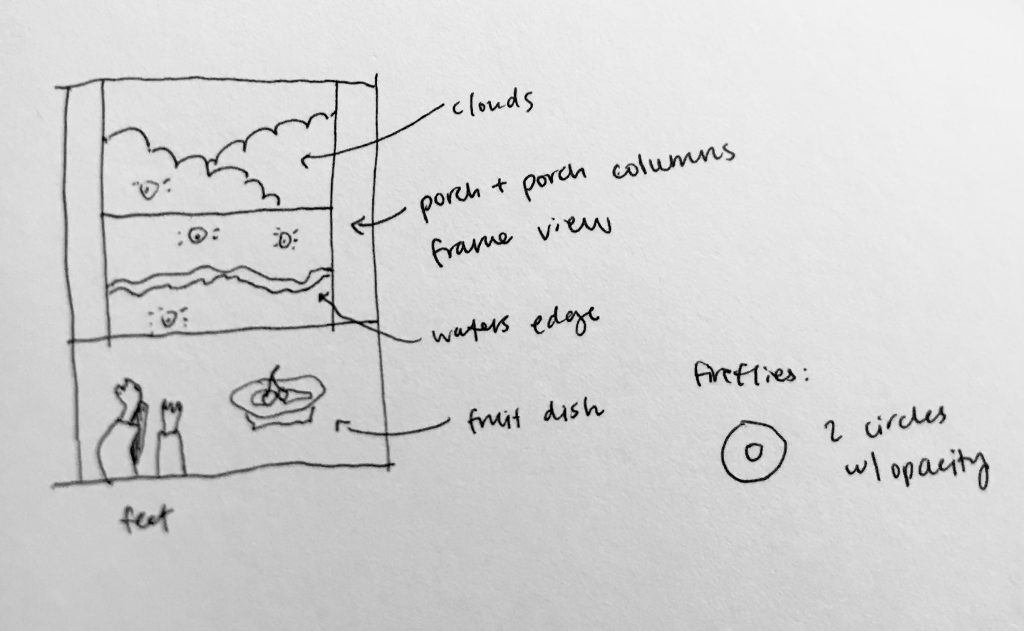
Looking Outwards 11
The artist I am focusing on today is Jessica Rosenkrantz from the Nervous System art studio. Her work takes scientific theory on pattern formation in nature to create algorithms for design. What I admire about her work is that I can recognize what these generative patterns remind me of in nature. For instance, her project Porifera (2018) resembles a sea sponge. And her project Growing Objects (2014) resembles a crystallized snowflake. I don’t if these were patterns she was drawing from, but having that reference for me grounds these highly computerized projects into a context I understand. Jessica graduated from MIT in 2005 with degrees in biology and architecture, then from Harvard’s Graduate School of Design (for architecture) in 2008.
Project 11
For this project I decided to create a series of clouds and planes which would move across the blue sky. With the amount of time spent indoors the past few months, it’s helpful to remember what it’s like to look up.
var clouds = [];
var xcNoise = [];
var ycNoise = [];
var scNoise = [];
var planes = [];
function setup() {
createCanvas(480, 480);
//creating initial number of objects
for (var i = 0; i < 4; i++){
var rx = random(width);
clouds[i] = makeClouds(rx);
}
for (var i = 0; i < 2; i++){
var rx = random(width);
planes[i] = makePlanes(rx);
}
for (var i = 0; i < 20; i++) {
xcNoise[i] = random(-40,40);
ycNoise[i] = random(-40,40);
scNoise[i] = random(60,70)
}
frameRate(10);
}
function draw() {
background(200,215,255);
updateAndDisplayPlanes();
updateAndDisplayClouds();
offScreenPlanes();
offScreenClouds();
newPlanes();
newClouds();
}
//making sure objects move across the screen
function updateAndDisplayClouds(){
for (var i = 0; i < clouds.length; i++){
clouds[i].move();
clouds[i].display();
}
}
function updateAndDisplayPlanes(){
for (var i = 0; i < planes.length; i++){
planes[i].move();
planes[i].display();
}
}
//removing objects once they're no longer needed
function offScreenClouds(){
var cloudsToKeep = [];
for (var i = 0; i < clouds.length; i++){
if (clouds[i].x + clouds[i].breadth > 0) {
cloudsToKeep.push(clouds[i]);
}
}
clouds = cloudsToKeep;
}
function offScreenPlanes(){
var planesToKeep = [];
for (var i = 0; i < planes.length; i++){
if (planes[i].x + planes[i].breadth > 0) {
planesToKeep.push(planes[i]);
}
}
planes = planesToKeep;
}
//different probabilities for adding new objects
function newClouds() {
var newCloud = 0.0095;
if (random(0,1) < newCloud) {
clouds.push(makeClouds(width+100));
}
}
function newPlanes() {
var newPlane = 0.007;
if (random(0,1) < newPlane) {
planes.push(makePlanes(width+100));
}
}
//moving the objects across the screen at different speeds
function cloudMove() {
this.x += this.speed;
}
function planeMove() {
this.x += this.speed;
}
//creating the actual objects for clouds and planes
function cloudDisplay() {
fill(255);
stroke(0);
push();
translate(this.x, this.placement);
stroke(200);
noStroke();
fill(255);
for (let i = 0; i < this.nFloors; i ++) {
circle(xcNoise[i], ycNoise[i], scNoise[i]);
}
pop();
}
function planeDisplay() {
push();
stroke(0);
fill(0);
translate(this.x, this.placement);
ellipse(0,0,40,8);
triangle(2,0,5,-25,-10,0);
triangle(2,0,5,25,-10,0);
triangle(15,0,15,8,10,0);
triangle(15,0,15,-8,10,0);
pop();
}
//creating the objects for clouds and planes, and their parameters
function makeClouds(birthLocationX) {
var cld = {x: birthLocationX,
breadth: 50,
speed: random(-2,-.8),
nFloors: round(random(4,10)),
move: cloudMove,
display: cloudDisplay,
placement: random(0,height)}
return cld;
}
function makePlanes(birthLocationX) {
var pln = {x: birthLocationX,
breadth: 20,
speed: random(-4,-2),
move: planeMove,
display: planeDisplay,
placement: random(0,height)}
return pln;
}
Looking Outwards 11
For this looking outwards, I took a closer look at a project and architect that I knew of, but not about, Jenny Sabin. She began her career with two arts degrees from the University of Washington, and went back to school a few years later and received a Masters in Architecture from the University of Pennsylvania. She has her own studio based in Philadelphia, and is now also a part of the Department of Architecture at Cornell University. Her work focuses on computational design, as well as digital fabrication. The project below is titled Lumen, and was on display in the summer of 2017 at MoMA PS1. The project is a great example of how digital fabrication can create precise spaces without giving way to corners or flat walls. Lumen is a knitted structure which serves to create private and shaded spaces in the summer heat, while lighting up to create dynamic and exciting spaces at night. The project was successful enough that it became one of a series of similar works from her and her studio.
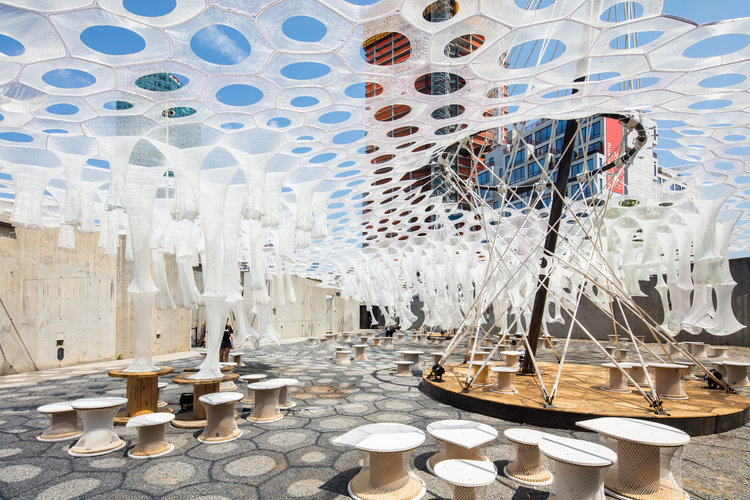
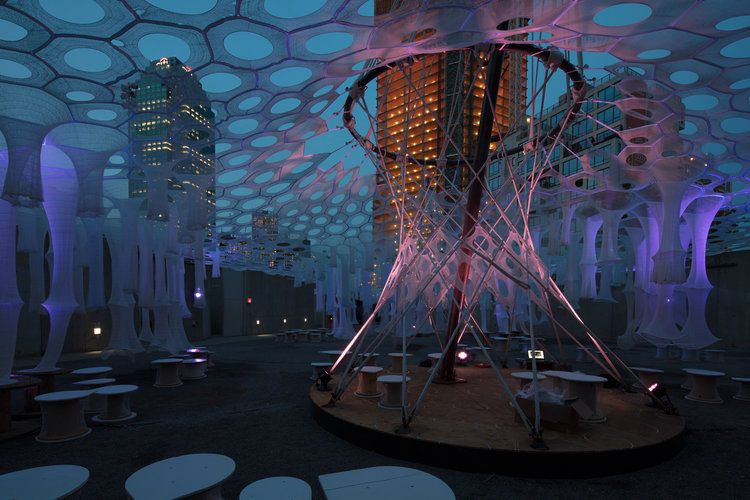
Looking Outwards 11
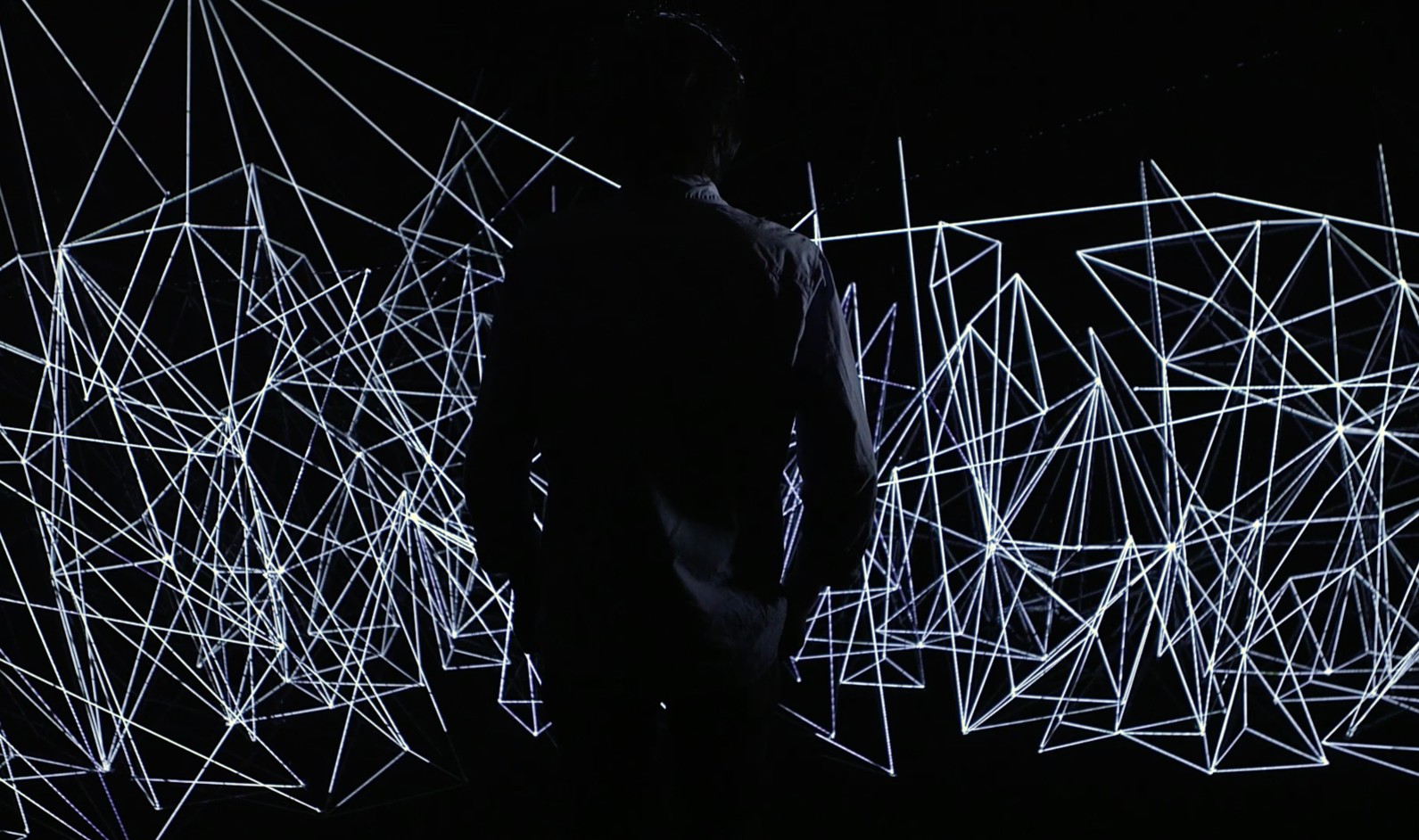
The female new media artist that I chose to highlight is Mimi Son. She studied interactive design at the Copenhagen Institute of Interaction Design before starting a design firm alongside Elliot Woods named Kimchi and Chips. Mimi’s fascination with geometry and Buddhist philosophy play a large role in inspiring her work and the elements of time and space.
Line Segments Space is a work by Mimi Son displayed in nylon string and digital emulsion. This display is shown in the Vimeo above where you can see the interaction between the light display and sound. I found this to be such a captivating form of line art. Each of lines represents a transformed 2 dimensional canvas which is also represented in different dimension, sound.
Project 11 Generative Landscape
Planetscape?
//tjchen
// project landscape
var stars=[]; // array for circles
var streaks=[]; // array for streaks
var speed = 3; // change for how zoom
// color initialization
var r;
var g;
var b;
// rate of change fo color
var dr;
var dg;
var db;
function setup() {
createCanvas(480, 480);
//initalize objects
for(i=0; i<=500; i++ ){
var s =makeStar();
var st=makeStreak();
stars.push(s);
streaks.push(st);
}
//background color setup
r = (random(255));
g = (random(255));
b = (random(255));
dr = (random(-5,5));
db = (random(-5,5));
dg = (random(-5,5));
}
function draw() {
background(r,g,b);
//set it up from the middle
translate(width/2,height/2);
//draw the stars and streaks
for(i=0; i<500; i++){
var s = stars[i];
s.drawstar();
s.updatestar()
var st = streaks[i];
st.drawstreak();
st.updatestreak();
}
// color update
if (r>255 || r<0){
dr= -dr;
}
if (g>255 || g<0){
dg= -dg;
}
if (b>255 || b<0){
db= -db;
}
r+=dr;
g+=dg;
b+=db;
}
//star construction
function makeStar(){
var star={
x:random(-width,width),
y:random(-height,height),
z:random(width), // constant ratio for zoom
c:color(random(255),random(255),random(255)),
drawstar: stardraw,
updatestar: starupdate,
}
return star;
}
function stardraw(){
noStroke();
fill(this.c);
// draw stars but mapped to z
var dx = map(this.x/this.z,0,1,0,width);
var dy = map(this.y/this.z,0,1,0,width);
// map it for sizze increase
var r = map(this.z,0,width,20,0);
ellipse(dx,dy,r);
}
function starupdate(){
this.z-=speed;
// reset
if (this.z<1){
this.z = width;
this.x = (random(-width,width));
this.y = (random(-height,height));
}
}
// streak construction
function makeStreak(){
var streak={
x:random(-width,width),
y:random(-height,height),
z:random(width),
c:color(random(255),random(255),random(255),50),
pz: this.z, // previous location of z
drawstreak: streakdraw,
updatestreak: streakupdate,
}
return streak;
}
function streakdraw(){
strokeWeight(5);
stroke(this.c);
var dx = map(this.x/this.z,0,1,0,width);
var dy = map(this.y/this.z,0,1,0,width);
var px = map(this.x/this.pz,0,1,0,width);
var py = map(this.y/this.pz,0,1,0,width);
//draw line
line(dx,dy,px,py);
}
function streakupdate(){
this.z-=speed;
// reset
if (this.z<1){
this.z = width;
this.x = (random(-width,width));
this.y = (random(-height,height));
this.pz = this.z;
}
}
LO 11 Women in Computational Deisgn
Jenny Wu is a co-founder at the architectural design firm Oyler Wu collaborative and Her own jewelry design company Lace by Jenny Wu. The firm is a highly experimental architectural practice that engages architecture and design with a hyper critical lens that focuses on research and fabrication. Their work crosses a variety of scales, from furniture detailing to large scale institutional buildings. Jenny Wu a classically trained architect received her alma mater from Columbia University and then Harvard graduate school of design, is currently a faculty member at the Southern California Institute of Architecture. What’s interesting about her work is that there’s a strong sense of connection between the jewelry she designs to the architectural work the firm produces, that engages the power of digital fabrication in relations to formal studies that relate back to the human in a variety of scales from the intimate jewelry to the inhabitable space of a building.
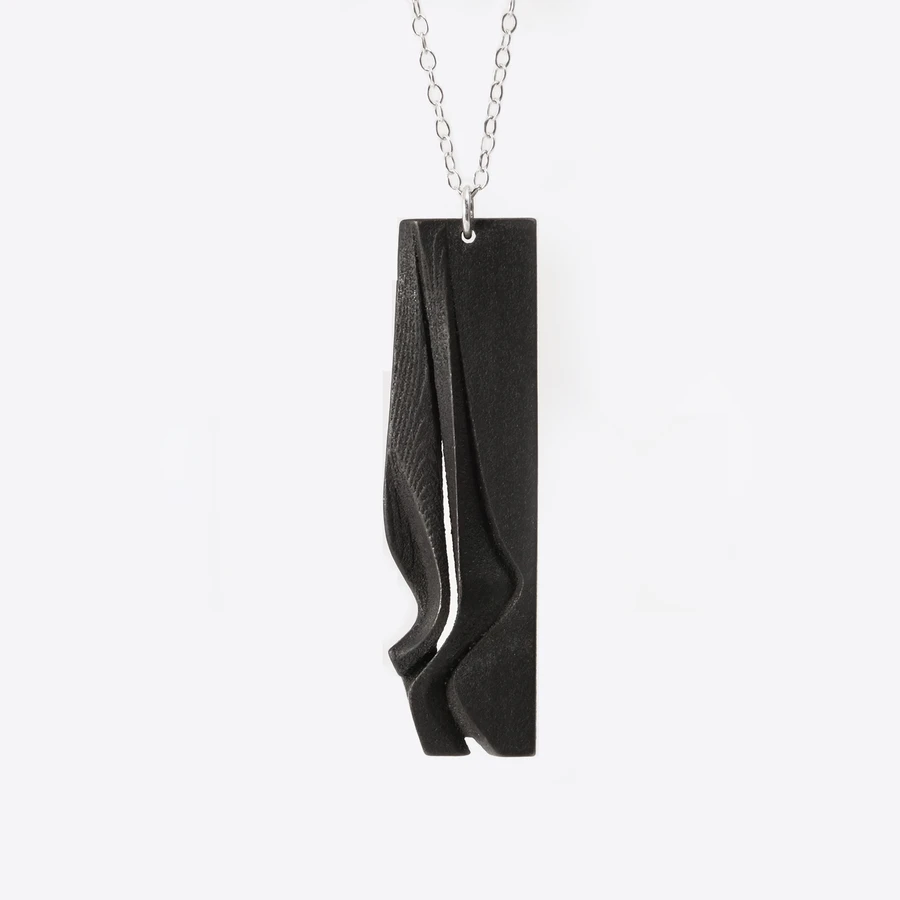