For this project, I was inspired by the week’s lab. At first, I thought it would be simple to convert the fish code to a clock but this was not the case. I had to play around with the arrays and indexes to make everything work. The number of fish represent the minutes as well as the level of water. The color of the water represents the hour of the day to show the tank getting dirtier until it is finally cleaned. Lastly, the clam opening and closing represents seconds.
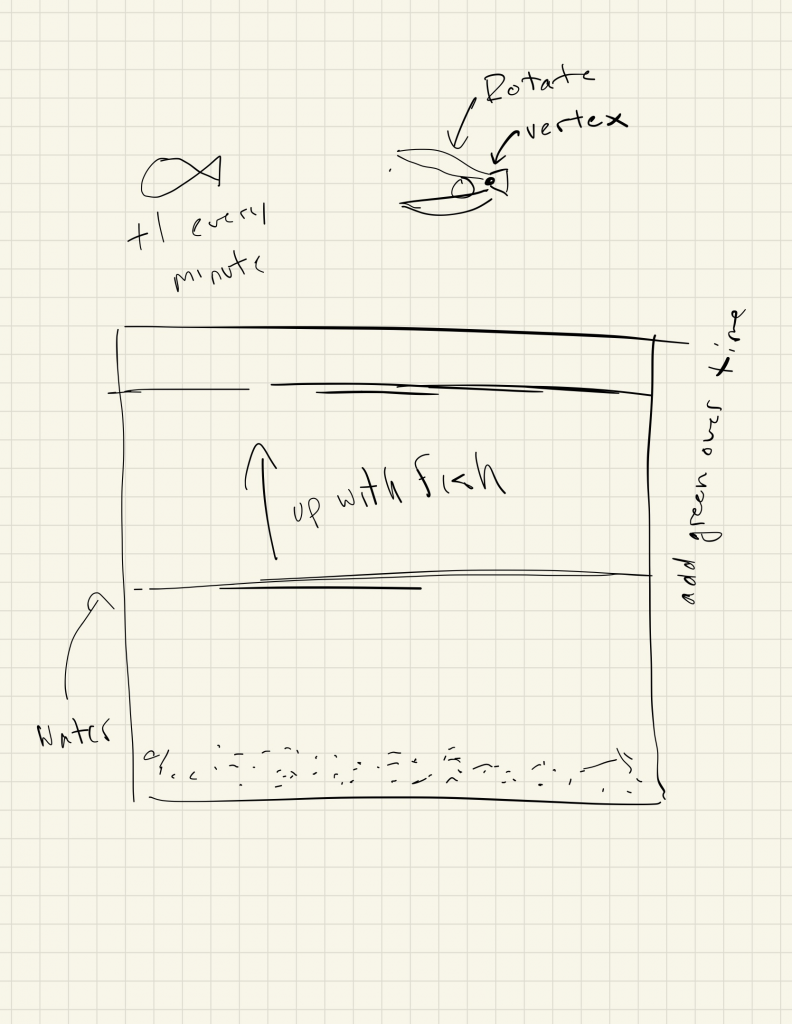
var x = [];
var y = [];
var dx = [];
var c = [];
//var numFish = minute()
function setup() {
createCanvas(480, 480);
background(220);
//stores values for 59 fish in an array
for (var i=0; i<59; i++) {
//random x position for fish array
x[i] = random(50, width-50);
//y position so fish will only be spawned within the water
y[i] = 3/4*height-i*((5/12/60)*height)
//random speed of the fish
dx[i] = random(-5, 5);
//random color of the fish
c[i] = color(random(255), random(255), random(255));
}
}
function draw() {
//variables to keep track of time
var s = second();
var h = hour();
var m = minute();
background(220);
stroke(0);
//fish tank with water level
//creates background rectangle of water based upon the hour
fill(0, 255, 255-(75/23)*h);
rect(10, 1/4*height, width - 20, 3/4*height)
//matches the background color and shrinks to reveal more water
fill(220);
rect(10, 1/4*height, width - 20, 1/3*height-(1/4*height/60)*m);
//creates fish
//creates the number of fish based upon minutes (+1 fish each minute)
//resets to zero fish every hour
for (i=0; i<m; i++) {
fish(x[i], y[i], dx[i], c[i]);
x[i] += dx[i];
//makes the fish turn within the aquarium
if(x[i] >= width - 25) {
dx[i] = -dx[i]
} else if(x[i] <= 25) {
dx[i] = -dx[i]
}
}
//sand
fill(237, 201, 175);
rect(10, 410, width-20, height-405);
//open and closes the clam every second (logic is based upon even/odd
//seconds)
if(s%2 == 1) {
openClam();
} else {
closedClam();
}
}
//used two functions to open and close clam
function closedClam() {
fill(199, 176, 255);
//top half of clam
arc(385, 400, 70, 30, PI, 0, CHORD)
//bottom half of clam
arc(385, 400, 70, 30, 0, PI, CHORD);
noStroke();
//back of clam
quad(430, 410, 430, 390, 410, 400, 410, 400);
}
function openClam() {
fill(255);
//creates the pearl
circle(390, 400, 20);
fill(199, 176, 255);
push();
//allows for rotation around back of clam
translate(420,400)
//rotates the top half of the clam
rotate(radians(30));
//top half of clam
arc(385-420, 400-400, 70, 30, PI, 0, CHORD)
pop();
//bottom half of clam
arc(385, 400, 70, 30, 0, PI, CHORD);
noStroke();
//back of clam
quad(430, 410, 430, 390, 410, 400, 410, 400);
}
//creates the fish based on the following parameters
function fish(x, y, dx, c){
//fills fish with the color from the array
fill(c);
//initial position of the fish based on the array
ellipse(x, y, 20, 10);
//logic for whether the fish tail is on the right or left side of fish
if(dx >= 0) {
triangle(x - 10, y, x - 15, y - 5, x - 15, y + 5);
}
else if(dx < 0) {
triangle(x + 10, y, x + 15, y - 5, x + 15, y + 5);
}
}