For this week’s project, I decided to make a pattern off of 2 animals and the things that they eat! In this case, I chose a rabbit and a bear, and alternated the pattern in between the bigger animal face and smaller food item to create a rhythm both vertically and horizontally that alternates between big and small.
// Susie Kim, Section A
// susiek@andrew.cmu.edu
// Assignment-05-A
function setup() {
createCanvas(600, 600);
background(163, 185, 216);
noLoop();
}
function draw() {
background(163, 185, 216);
// bunnies
for(var x = 25; x <= 600; x+= 150) {
for(var y = 0; y <= 600; y+= 200){
push();
translate(x, y);
bunny(x,y);
pop();
}
}
// carrots
for(var x = -50; x <= 600; x+= 150) {
for(var y = 0; y <= 600; y+= 200){
push();
translate(x, y);
carrot(x,y);
pop();
}
}
// bears
for(var x = -50; x <= 600; x+= 150) {
for(var y = 100; y <= 600; y+= 200){
push();
translate(x, y);
bear(x,y);
pop();
}
}
//fishes
for(var x = 25; x <= 600; x+= 150) {
for(var y = 100; y <= 600; y+= 200){
push();
translate(x, y);
fish(x,y);
pop();
}
}
}
function bunny(x,y) {
// head
noStroke();
fill(255);
ellipse(50, 65, 69, 50);
// ears
ellipse(30, 37, 25, 55);
ellipse(70, 33, 23, 55);
// eyes
fill(51, 51, 51);
ellipse(38, 60, 5, 5);
ellipse(62, 60, 5, 5);
// nose
ellipse(50, 70, 6, 6)
// cheeks
fill(246, 202, 201);
ellipse(30, 69, 13, 8);
ellipse(70, 69, 13, 8);
// mouth
strokeWeight(2);
noFill();
stroke(51, 51, 51);
curve(50, 30, 50, 70, 40, 75, 40, 60);
curve(50, 30, 50, 70, 60, 75, 60, 60);
}
function bear(x,y) {
// head
noStroke();
fill(225, 189, 152);
ellipse(50, 60, 68, 58);
// ears
ellipse(25, 35, 24, 24);
ellipse(75, 35, 26, 29);
// cheeks
fill(239, 163, 163);
ellipse(30, 65, 13, 8);
ellipse(70, 65, 13, 8);
// eyes
fill(51, 51, 51);
ellipse(37, 55, 5, 5);
ellipse(63, 55, 5, 5);
// nose
ellipse(50, 65, 6, 6);
// mouth
strokeWeight(2);
noFill();
stroke(51, 51, 51);
line(50, 65, 50, 72);
curve(45, 60, 45, 71, 55, 71, 55, 60);
}
function carrot(x,y) {
//leaf1
noStroke();
fill(165, 209, 117);
ellipse(50, 38, 9, 20);
// leaf2
push();
translate(57, 38);
rotate(radians(30));
ellipse(0, 0, 9, 23);
pop();
//leaf3
push();
translate(43, 38);
rotate(radians(-30));
ellipse(0, 0, 9, 23);
pop();
// carrot body
fill(253, 195, 117);
ellipse(50, 60, 17, 35);
}
function fish(x,y) {
// body
noStroke();
fill(74, 98, 168);
ellipse(46, 50, 38, 18);
push();
// back fin 1
translate(63, 54);
rotate(radians(17));
ellipse(0, 0, 23, 9);
pop();
// back fin 2
push();
translate(63, 46);
rotate(radians(-17));
ellipse(0, 0, 23, 9);
pop();
// eye
fill(0);
ellipse(37, 47, 3, 3);
// side fin
fill(58, 78, 155);
ellipse(48, 53, 15, 7)
}
To start, I “sketched” out each animal, as well as the pattern, in illustrator, and coded from there:

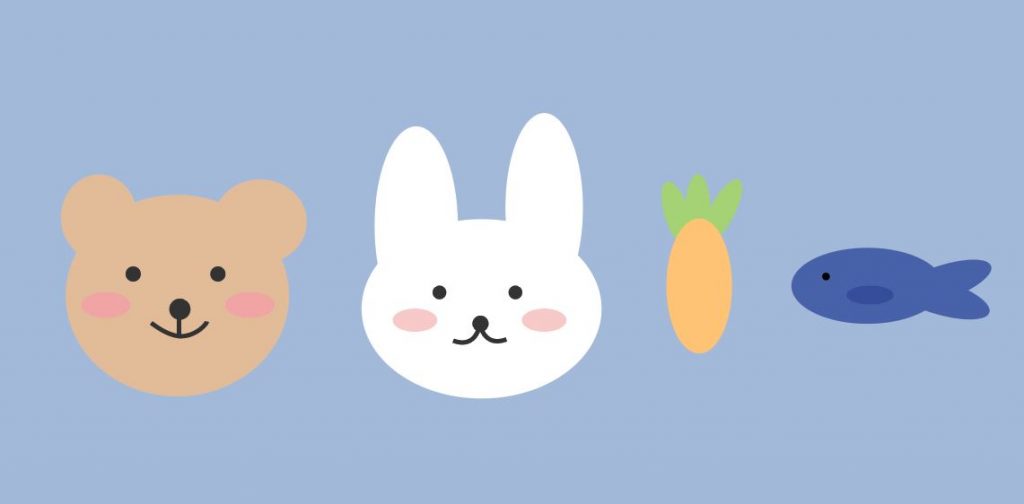