For this project, I want to convey the feeling of the breeze with the moving waves made of pixels.
//jiaqiwa2; Jiaqi Wang; Section C
var portrait;
//I learned & referenced how to make a moving sin wave from this example:
//https://p5js.org/examples/math-sine-wave.html
let xspacing = 5; // Distance between each horizontal location
let w; // Width of entire wave
let theta = 0.0; // Start angle at 0
let amplitude = 20.0; // Height of wave
let period = 700.0; // How many pixels before the wave repeats
let dx; // Value for incrementing x
let yvalues; // Using an array to store height values for the wave
var num=8;
function preload(){
portrait=loadImage("https://i.imgur.com/J6Im42B.jpg");
}
function setup() {
createCanvas(460,400);
w = width + 16;
dx = (TWO_PI / period) * xspacing;
yvalues = new Array(floor(w / xspacing));
frameRate(10);
}
function draw() {
//image(portrait,0,0,460,400);
background(250);
//gradually "open" the portrait
if(num<40){
num+=0.5;
}
for(var i=0;i<num;i++){
var yUpper;
var yLower;
yUpper=height/2-i*5;
yLower=height/2+i*5;
amplitude+=1;
if(amplitude>40){
amplitude-=1;
}
calcWave();
renderWave(yUpper);
renderWave(yLower);
}
}
function calcWave() {
// Increment theta (try different values for
// 'angular velocity' here)
theta += 0.02;
// For every x value, calculate a y value with sine function
let x = theta;
for (let i = 0; i < yvalues.length; i++) {
yvalues[i] = sin(x) * amplitude;
x += dx;
}
}
function renderWave(Start) {
noStroke();
// draw the wave with an ellipse at each location
for (let x = 0; x < yvalues.length; x++) {
//y postition of the ellipse
var y=Start + yvalues[x];
//x position of the ellipse
var X=x * xspacing;
//find the corresponding pixel's location on my portriat
var Px=map(X,0,460,0,portrait.width);
var Py=map(y,0,400,0,portrait.height);
//check if this pixel is within the canvas.
if(y>0 & y<400){
let pix = portrait.get(Px, Py);
fill(pix);
ellipse(X, y, 7, 7);
}
}
}
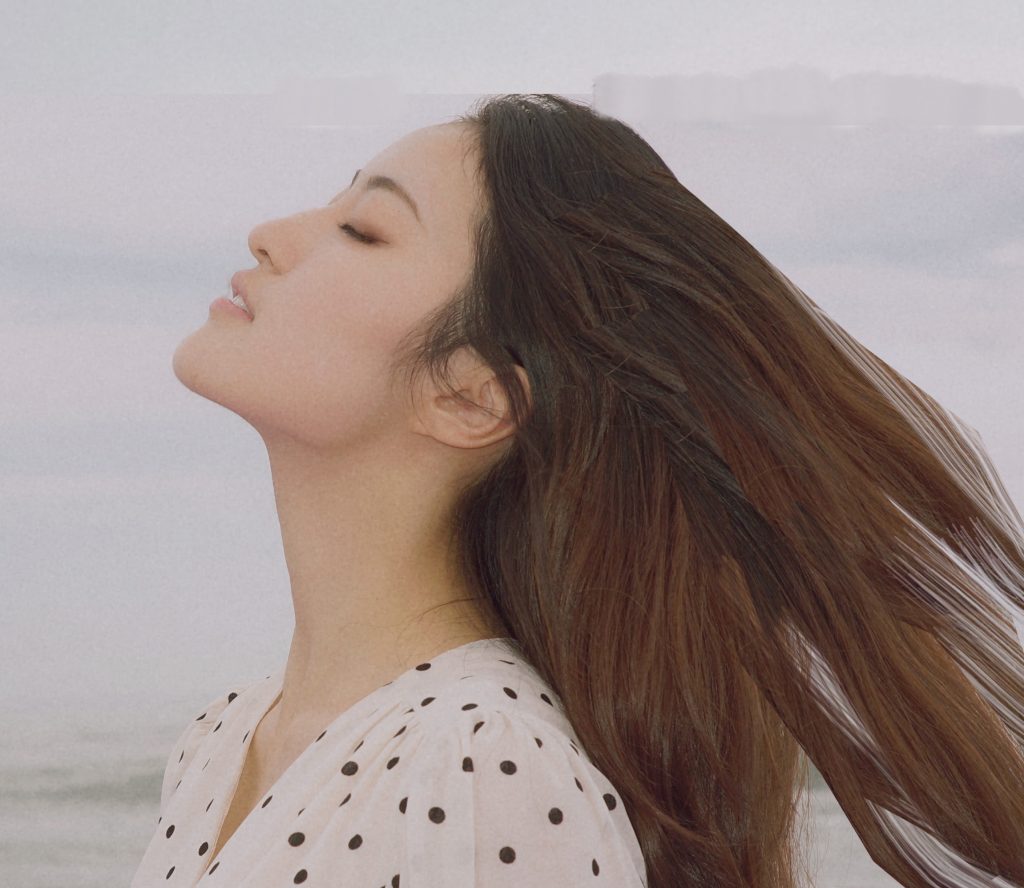
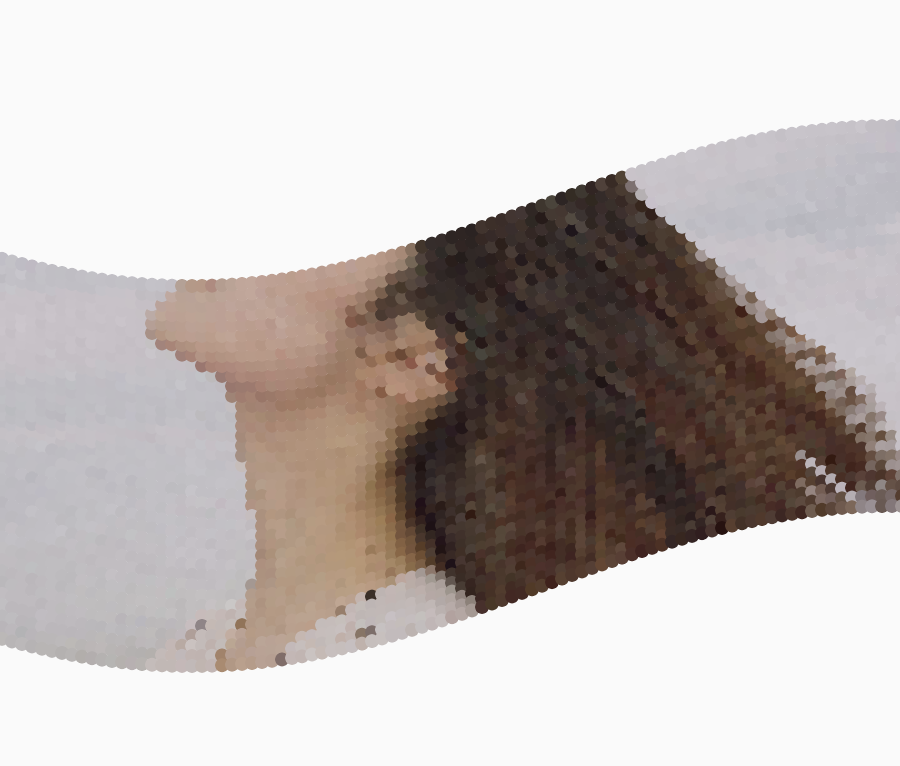
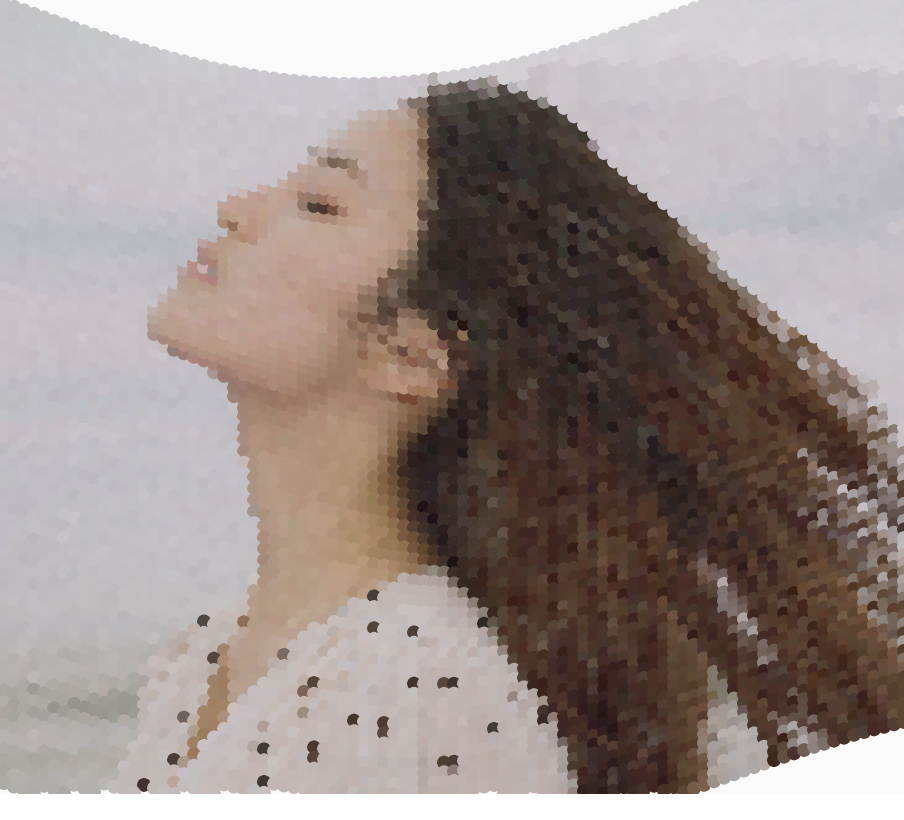