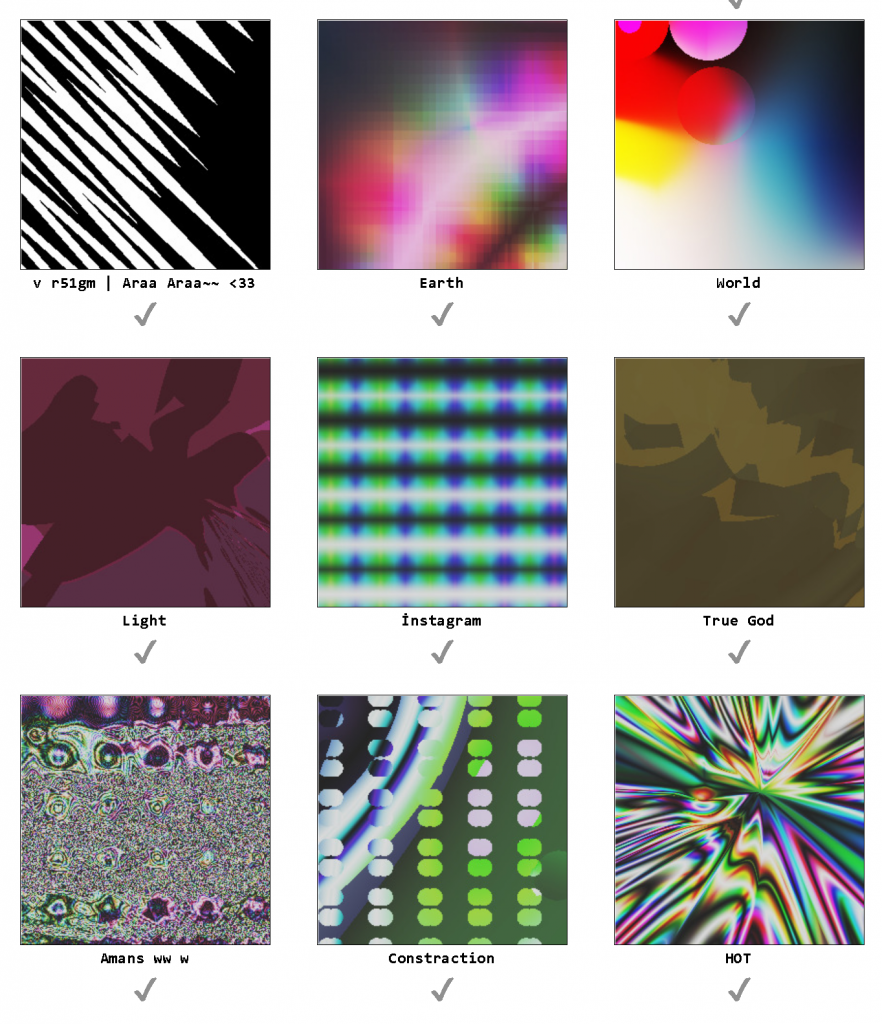
This project uses random numbers to generate randomized art based on a user-input title string to seed the generation. It produces completely random psychedelic works that are featured in a constantly updated publicly available gallery. Based on the creator’s description, it seems that the process for image generation is based on the seed from the title and then random number generators use that as input to create different but categorically similar works. Some of them share similar overall compositions, materials, or color combinations. The creator’s sensibilities are manifested through how the code displays the randomness, or how the actual visual display reflects the random numbers. In some sense though, the actual art is created by the user’s name input, because this is what seeds the visual generation. The creator just created the method or tool for the translation of that text to a randomized visual output.
random-art.org
Coded by Andrej Bauer, works ‘created’ by the public