/*
Nicholas Wong
Section A
*/
var spacing = 6; //Spacing between waves
var offset; //Wave offset
function setup() {
createCanvas(600,400);
offset = 23;
}
function draw()
{
background(20,10,15)
//Star grid
for (let x = 0; x <= width; x += 15) //Cols
{
for (let y = 0; y <= height / 2; y += 15) //Rows
{
push();
noStroke();
fill(255)
circle(x+(random(0,20)),y+(random(0,20)),random(0.5,1.5)) //Offsets each star X and Y by a random amount
pop();
}
}
//Wave generation
for(let w = 0; w <= height; w += spacing) //Number of waves
{
fill(0.75*w,0.15*w,0.25*w) //Color darkens with number of loops
strokeWeight(0.25)
stroke(w)
wave(w, offset*w); //Offset of waves and wave pattern determined by number of loops
}
noLoop();
}
// Wave function
function wave(yOffset, offset)
{
beginShape();
for(let x = 0; x < width; x++)
{
let angle = offset + x *0.01;
let y = map(sin(angle), -1, 1, 100, 200);
vertex(x,y + yOffset);
}
vertex(width,height);
vertex(0,height);
endShape();
}
Category: Uncategorized
Project 5: Wallpaper
var s = 100
var x = 50
var y = 50
function setup() {
createCanvas(600, 600);
background(60, 0, 33); //mauve 4, background color
strokeWeight(0);
rectMode(CENTER);
}
function draw() {
for (let r = 0; r <=5; r += 1) {
for (let c = 0; c <= 5; c += 1) {
tile(); //tile pattern
y += 100;
//s += 100;
}
y = 50;
tile();
x += 100;
}
}
function tile() {
push();
translate(x, y);
//random dots
fill(235, 181, 211);
circle(random(-50, 0), random(-50, 0), random(2,7));
circle(random(0, 50), random(0, 50), random(2,7));
fill(193, 99, 151);
circle(random(-50, 0), random(0, 50), random(2,7));
circle(random(0, 50), random(-50, 0), random(2,7));
fill(120, 26, 78);
circle(random(-50, 0), random(-50, 0), random(2,7));
circle(random(0, 50), random(0, 50), random(2,7));
//lines
fill(120, 26, 78); // mauve 3
push();
rotate(radians(45));
rect(0, 0, s/2 + 10, s/2 + 10);
pop();
push();
fill(60, 0, 33); //mauve 4
rotate(radians(45));
rect(0, 0, s/2, s/2);
pop();
//circles
fill(120, 26, 78); // mauve 3
circle(-25, -25, 30);
circle(25, -25, 30);
circle(25, 25, 30);
circle(-25, 25, 30);
fill(60, 0, 33); //mauve 4
circle(-25, -25, 20);
circle(25, -25, 20);
circle(25, 25, 20);
circle(-25, 25, 20);
//flower
fill(193, 99, 151); //mauve 2
push();
for(let x=0; x < 8; x += 1) {
ellipse(10, 10, s/2, 10);
rotate(radians(45));
}
pop();
//inner flower
push();
fill(235, 181, 222); //mauve 1
for(let x=0; x < 8; x += 1) {
ellipse(5, 2, s/2, 10);
rotate(radians(45));
}
pop();
//flower center
fill(193, 99, 151); //mauve 2
circle(0, 0, 15);
pop();
}
I drew inspiration from my sketch. However, I decided to add more flower patterns in my code than the flower petals in my original sketch.
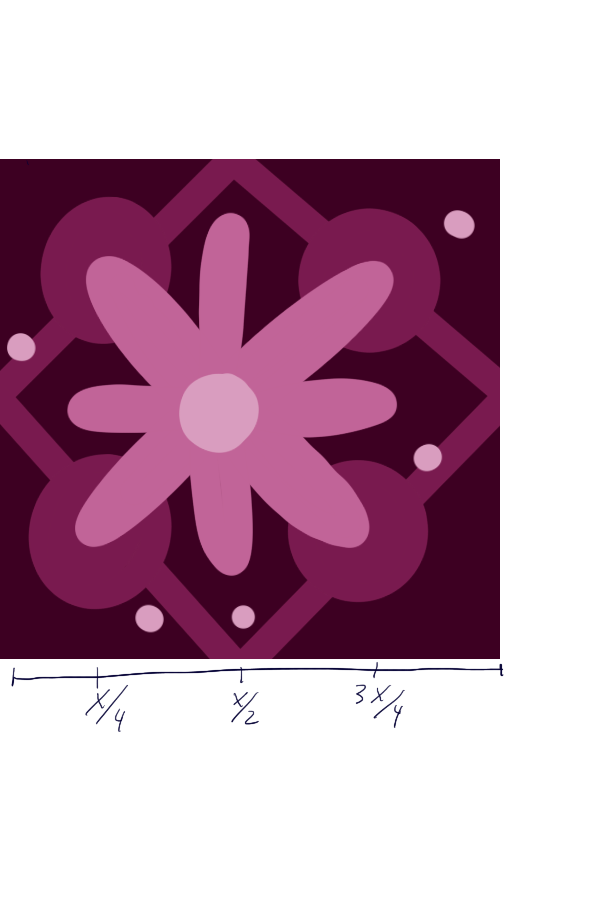
Project 05 – Wallpaper
Too much Among Us was played in the making of this wallpaper.
Cyan sus.
/*
*Eric Zhao
*ezhao2@andrew.cmu.edu
*
*Draws a wallpaper consisting of a "3D lattice"
*and Among Us sprites.
*/
var w = 50; //pillar length and height
var s = 10; //pillar width
var pillarHue = 101;
var pillarLight = 75;
var pillarDark = 25;
function setup() {
colorMode(HSB);
createCanvas(600, 600);
background(16, 34, 93);
}
function draw() {
//draws the lattice of pillars
for(let i = 0; i < 12; i++){
for(let j = 0; j < 12; j++){
if((i+j) % 2 == 0){
pillar2(i*w, j*w, s, w, pillarHue, pillarLight, pillarDark);
} else{
pillar(i*w, j*w, s, w, pillarHue, pillarLight, pillarDark);
}
}
}
//draws the Among Us sprites on rows with odd numbers of spaces
for(let i = 0; i < height; i += 2*w){
push();
translate(0, i);
for(let j = 0; j < width-w; j += 2*w){
push();
translate(j + w/2, w/2);
scale(0.45);
amongUs();
pop();
}
pop();
}
translate(w, w);
//draws the Among Us sprites on rows with even numbers of spaces
for(let i = 0; i < height-2*w; i += 2*w){
push();
translate(0, i);
for(let j = 0; j < width-2*w; j += 2*w){
push();
translate(j + w/2, w/2);
scale(0.45);
amongUs();
pop();
}
pop();
}
//shh, here's the impostor!
translate(width/3+15, height/3-2);
scale(0.2);
knife();
noLoop();
}
function pillar(x, y, s, w, hue, light, shadow){
//pillar function drawing a lattice element top left to bottom right
fill(hue, 40, shadow);
quad(x, y, x+s, y, x+w, y+w-s, w+x, w+y);
fill(hue, 40, light);
quad(x, y, w+x, w+y, w-s+x, w+y, x, s+y);
}
function pillar2(x, y, s, w, hue, light, shadow){
//pillar function drawing a lattice element bot left to top right
fill(hue, 40, shadow);
quad(x, y+w, x+w, y, x+w, y+s, x+s, y+w);
fill(hue, 40, light);
quad(x, y+w, x, y+w-s, x+w-s, y, x+w, y);
}
function amongUs(){
//creates an Among Us sprite lookalike
push();
noStroke();
fill(50);
ellipse(53, 94, 70, 15); //shadow
backpack();
body();
visor();
pop();
}
function body(){
//Among Us sprite body
//body base color
noStroke();
fill(187, 100, 76);
beginShape();
curveVertex(59, 76);
curveVertex(59, 76);
curveVertex(62, 88);
curveVertex(75, 88);
curveVertex(78, 74);
curveVertex(81, 55);
curveVertex(79, 38);
curveVertex(69, 7);
curveVertex(38, 7);
curveVertex(28, 48);
curveVertex(32, 91);
curveVertex(50, 91);
curveVertex(52, 76);
curveVertex(59, 76);
curveVertex(59, 76);
endShape();
//body highlight color
fill(172, 56, 80);
beginShape();
curveVertex(45, 62);
curveVertex(45, 62);
curveVertex(70, 63);
curveVertex(79, 38);
curveVertex(69, 7);
curveVertex(45, 4);
curveVertex(36, 32);
curveVertex(39, 54);
curveVertex(45, 62);
curveVertex(45, 62);
endShape();
//outline
stroke(0);
strokeWeight(6);
noFill();
beginShape();
curveVertex(59, 76);
curveVertex(59, 76);
curveVertex(62, 88);
curveVertex(75, 88);
curveVertex(78, 74);
curveVertex(81, 55);
curveVertex(79, 38);
curveVertex(69, 7);
curveVertex(38, 7);
curveVertex(28, 48);
curveVertex(32, 91);
curveVertex(50, 91);
curveVertex(52, 76);
curveVertex(59, 76);
curveVertex(59, 76);
endShape();
}
function backpack(){
//Among Us backpack (body colored)
noStroke();
//backpack base color
fill(187, 100, 76);
beginShape();
curveVertex(33, 27);
curveVertex(33, 27);
curveVertex(19, 31);
curveVertex(17, 69);
curveVertex(30, 72);
curveVertex(30, 72);
endShape();
//backpack highlight
fill(172, 56, 80);
beginShape();
curveVertex(33, 27);
curveVertex(33, 27);
curveVertex(19, 28);
curveVertex(18, 39);
curveVertex(33, 36);
curveVertex(33, 36);
endShape();
strokeWeight(6);
noFill();
//outline
stroke(0);
strokeWeight(6);
noFill();
beginShape();
curveVertex(33, 27);
curveVertex(33, 27);
curveVertex(19, 31);
curveVertex(17, 69);
curveVertex(30, 72);
curveVertex(30, 72);
endShape();
}
function visor(){
//Among Us visor section
strokeWeight(6);
noStroke();
fill(193, 38, 43);
ellipse(64, 28, 35, 25);
fill(196, 36, 87);
ellipse(67, 24, 25, 18);
fill(0, 0, 100);
ellipse(68, 24, 15, 5);
//outline
stroke(0);
noFill();
ellipse(64, 28, 35, 25);
}
function knife() {
//for the killer only...
strokeWeight(2);
fill(0, 0, 37);
rect(0, 0, 15, 30);
fill(11, 67, 51);
rect(-10, 30, 35, 15);
fill(0, 0, 50);
triangle(-5, 45, 7.5, 100, 20, 45);
noStroke();
fill(0, 0, 85);
triangle(7.5, 45, 7.5, 100, -5, 45);
noFill();
stroke(0);
triangle(-5, 45, 7.5, 100, 20, 45);
noLoop();
}
Looking Outwards-05
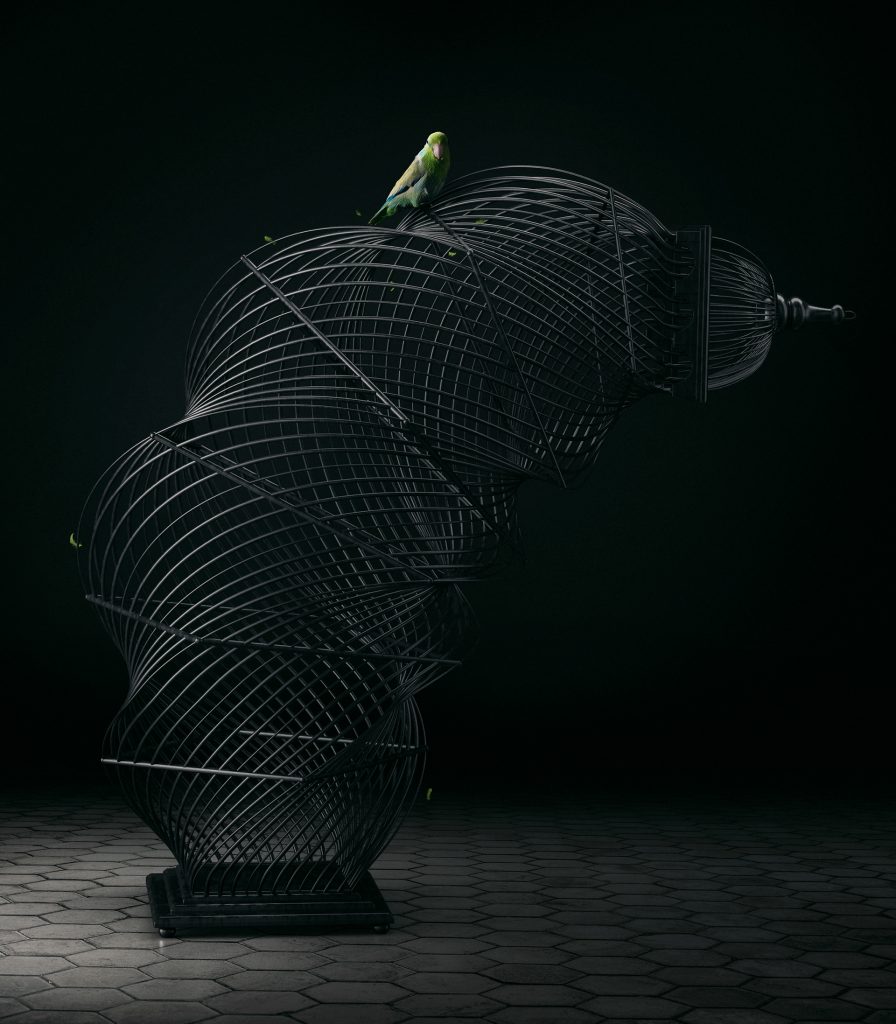
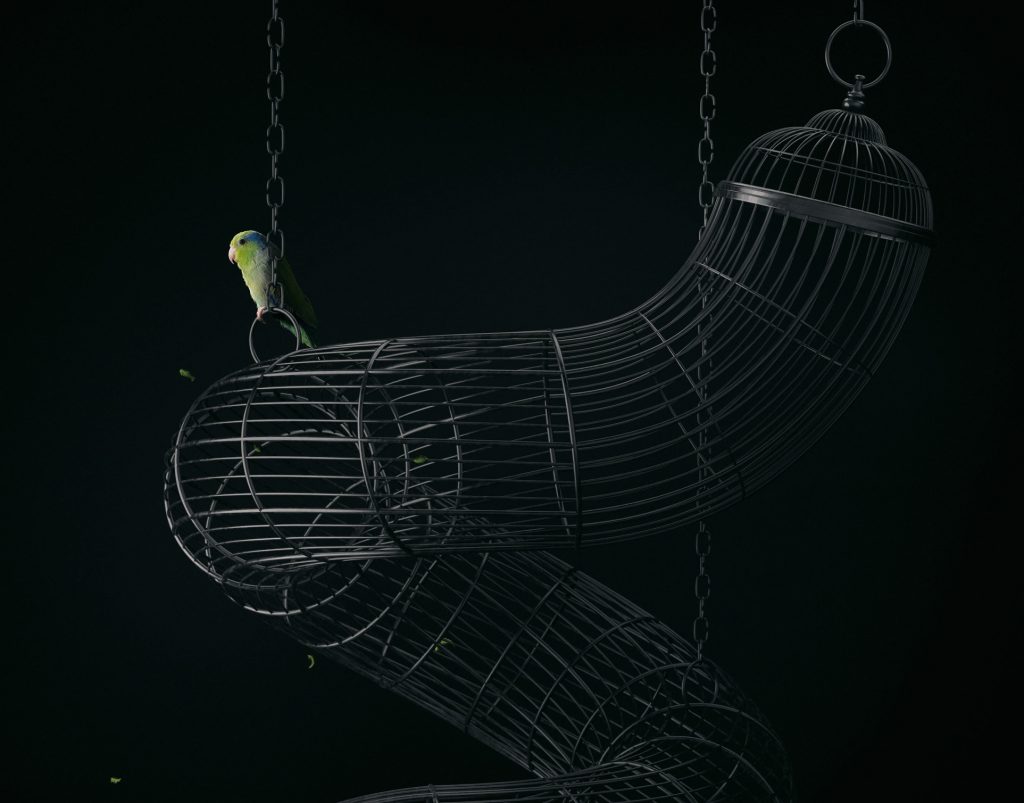
https://www.behance.net/gallery/40600473/FREE-BIRD
This collection is named “Free Bird” and is by Mike Campau. He’s a photographer who uses CGI to distort components of reality. Normally, he makes commercial art/graphics for companies like Beats by Dre, Under Armour, Nike, etc., but he also does personal projects like the one shown. In this collection, I like the way that he distorted that he used CGI to distort the bird cages. The statement included with it is “an escape from the odd and dark world we live in… and sometimes there are no doors to get out.” I think that the distortion of the cages helps convey the complexity of the problems people try to escape and creates a very somber and imposing mood. I don’t understand where the doors come in to play though. I’m guessing we are supposed to question how the bird managed to get out of the cage in the first place and wonder why the bird is still sitting on the cage if it managed to get out which I find interesting. He says that he made this in Photoshop to make this. I know that you can make 3D models and texture them in Photoshop, but I’ve never done it before so I couldn’t say exactly how he did it. Also, the bird a real bird and not a 3D model.
Project 05- Wallpaper
I created a wallpaper with illustrations of birds, rainbows, and clouds. Recently, I saw two parrots outside my window (it was odd because I live in the middle of an urban city). I was worried they might not survive the cold weather, but they flew away as my family opened the window to bring them in. I wanted to create visuals of the scene I saw that day in my wallpaper.
//Stefanie Suk
//15-104 Section D
var positionbx = 10; //starting position x for blue bird
var positionby = 10; //starting position y for blue bird
var offsetbx = 120; //spacing x for blue bird
var offsetby = 140; //spacing y for blue bird
var positionyx = 75; //starting position x for yellow bird
var positionyy = 75; //starting position y for yellow bird
var offsetyx = 120; //spacing x for yellow bird
var offsetyy = 140; //spacing y for yellow bird
var positionrx = 10; //starting position x for rainbow
var positionry = 95; //starting position y for rainbow
var offsetrx = 120; //spacing x for rainbow
var offsetry = 140; //spacing y for rainbow
var positioncx = 70; //starting position x for cloud
var positioncy = 20; //starting position y for cloud
var offsetcx = 120; //spacing x for cloud
var offsetcy = 140; //spacing y for cloud
var positiondx = 0; //starting position x for dots
var positiondy = 0; //starting position y for dots
var offsetdx = 20; //spacing x for dots
var offsetdy = 20; //spacing y for dots
function setup() {
createCanvas(500, 600);
background(195, 213, 195);
noLoop();
}
function draw() {
for(var a=0; a<50; a++){ //column of grid
for(var b = 0; b<50; b++){ //row of grid
push();
translate(positiondx + offsetdx*b, positiondy + offsetdy*a); //scale down
dots();
pop();
}
}
// blue bird grid
for(var a=0; a<5; a++){ //column of grid
for(var b = 0; b<5; b++){ //row of grid
push();
translate(positionbx + offsetbx*b, positionby + offsetby*a);
scale(0.5); //scale down
bluebird();
pop();
}
}
// yellow bird grid
for(var a=0; a<5; a++){ //column of grid
for(var b = 0; b<5; b++){ //row of grid
push();
translate(positionyx + offsetyx*b, positionyy + offsetyy*a);
scale(0.4); //scale down
yellowbird();
pop();
}
}
//rainbow grid
for(var a=0; a<5; a++){ //column of grid
for(var b = 0; b<5; b++){ //row of grid
push();
translate(positionrx + offsetrx*b, positionry + offsetry*a);
scale(0.5); //scale down
rainbow();
pop();
}
}
//cloud grid
for(var a=0; a<5; a++){ //column of grid
for(var b = 0; b<5; b++){ //row of grid
push();
translate(positioncx + offsetcx*b, positioncy + offsetcy*a);
cloud();
pop();
}
}
}
function bluebird() {
//body
noStroke();
fill(178, 232, 245);
ellipse(0, 0, 50, 50); //head
ellipse(0, 40, 60, 80);
fill(178, 232, 245); //body
push()
rotate(radians(30));
ellipse(48, 30, 10, 60) //tail
// white section of head
fill(250);
rotate(radians(220));
arc(0, 0, 45, 45, 0, QUARTER_PI);
pop();
//beak
fill(187, 201, 205);
ellipse(4, 0, 10, 10); //right grey circle
ellipse(-4, 0, 10, 10); //left grey circle
fill(157, 170, 173);
triangle(-6, 2, 6, 2, 0, 18); //dark grey beak
//eye
fill(10);
ellipse(10, -2, 7, 7); //right eye
ellipse(-10, -2, 7, 7); //left eye
//wings
fill(132, 163, 170);
ellipse(20, 40, 15, 40); //right dark blue wing
ellipse(-20, 40, 15, 40); //left dark blue wing
}
function yellowbird() {
//body
noStroke();
fill(245, 242, 125);
ellipse(0, 0, 50, 50); //head
ellipse(0, 40, 60, 80);
fill(245, 242, 125); //body
push()
rotate(radians(30));
ellipse(48, 30, 10, 60) //tail
// orange section of head
fill(255, 176, 117);
rotate(radians(220));
arc(0, 0, 45, 45, 0, QUARTER_PI);
pop();
//beak
fill(212, 208, 150);
ellipse(4, 0, 10, 10); //right greyish yellow circle
ellipse(-4, 0, 10, 10); //left greyish yellow circle
fill(151, 150, 131);
triangle(-6, 2, 6, 2, 0, 18); //dark greyish yellow beak
//eye
fill(10);
ellipse(10, -2, 7, 7); //right eye
ellipse(-10, -2, 7, 7); //left eye
//wings
fill(160, 212, 150);
ellipse(20, 40, 15, 40); //right green wing
ellipse(-20, 40, 15, 40); //left green wing
}
function rainbow() {
push();
noStroke();
fill(255, 92, 92);
rotate(radians(158));
arc(0, 0, 35, 35, 0, PI+QUARTER_PI, OPEN); //red layer
fill(255, 156, 92);
arc(0, 0, 30, 30, 0, PI+QUARTER_PI, OPEN); //orange layer
fill(255, 245, 92);
arc(0, 0, 25, 25, 0, PI+QUARTER_PI, OPEN); //yellow layer
fill(160, 240, 125);
arc(0, 0, 20, 20, 0, PI+QUARTER_PI, OPEN); //green layer
fill(125, 193, 240);
arc(0, 0, 15, 15, 0, PI+QUARTER_PI, OPEN); //blue layer
fill(175, 125, 240);
arc(0, 0, 10, 10, 0, PI+QUARTER_PI, OPEN); //purple layer
pop();
}
function cloud() {
noStroke();
fill(255);
ellipse(0, 0, 20, 20);
ellipse(-10, 5, 15, 15);
ellipse(12, 4, 17, 17);
ellipse(22, 5, 10, 10); //white ellipses left to right
}
function dots() {
noStroke();
fill(255, 255, 255, 6);
ellipse(0, 0, 5, 5); //dots for background
}
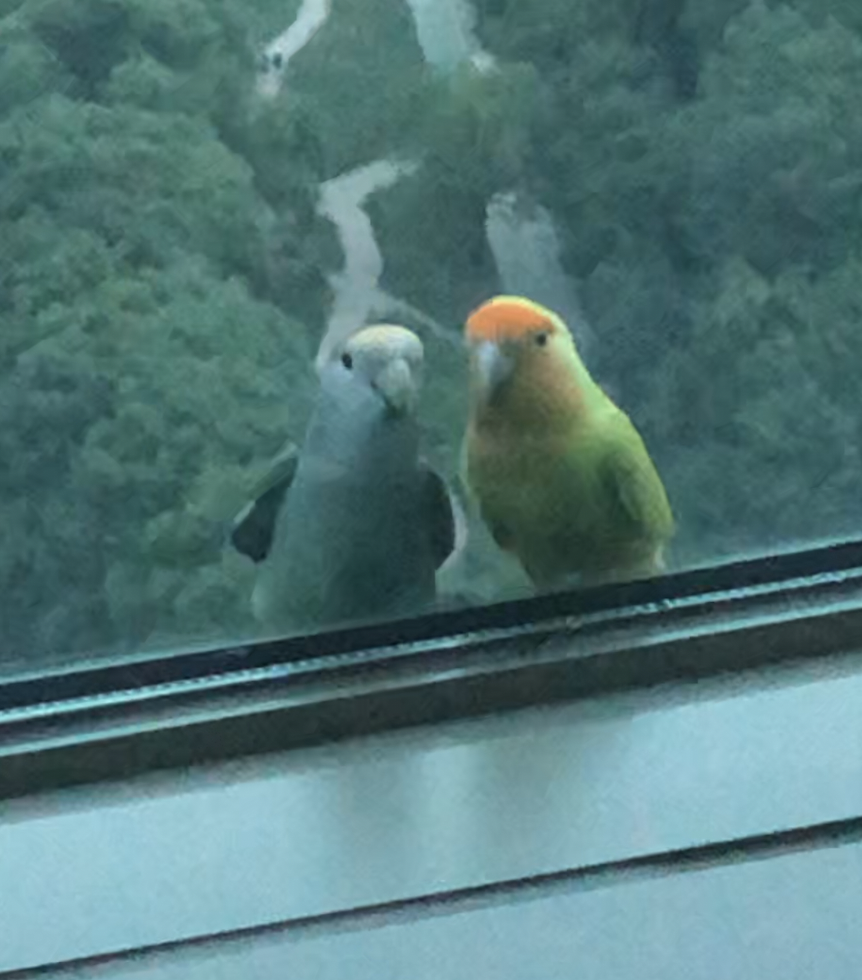
Project 05: Wallpaper
The biggest challenge in this project was dealing with drawing the spines on the cactus. It turns out P5.js doesn’t really like nested loops in functions and it crashed the program when I tried to use it, so I had to do the inefficient solution of manually drawing the points.
function setup() {
createCanvas(600, 600);
strokeWeight(3)
}
function draw() {
for (x = 120; x <= width - 120; x += 120) {
for (y = 120; y <= height - 120; y += 120) {
createCactus(x, y, 60, 80)
}
}
}
function createCactus(cactusx, cactusy, cactusw, cactush) {
push()
rotate(radians(random(-PI * 2, PI * 2)))
translate(cactusx,cactusy)
fill(35, 117, 67)
ellipse(0, 0, cactusw, cactush)
body()
arms(45)
flower()
pop()
noLoop()
}
function body() {
point((random(-20, 20)), random(-30, 30))
point((random(-20, 20)), random(-30, 30))
point((random(-20, 20)), random(-30, 30))
point((random(-20, 20)), random(-30, 30))
point((random(-20, 20)), random(-30, 30))
point((random(-20, 20)), random(-30, 30))
point((random(-20, 20)), random(-30, 30))
point((random(-20, 20)), random(-30, 30))
point((random(-20, 20)), random(-30, 30))
point((random(-20, 20)), random(-30, 30))
noLoop()
}
function arms (x) {
push()
translate(x,0)
rotate(degrees(90))
ellipse(0, 0, 20, 40)
pop()
push()
translate(-x,0)
rotate(degrees(230))
ellipse(0, 0, 20, 40)
pop()
}
function flower() {
noStroke()
push()
translate(0, -40)
for (r = 90; r <= 270; r += 180) {
rotate(degrees(r))
fill(245, 110, 191)
ellipse(0, 0, 10, 20)
}
pop()
}
//// for (x = 0; x <= cactusw/2.75; x += 10) {
//for (y = 0; y <= cactush/2.25; y += 10) {
//point(x,y)
//}
//}
///for (x = -(cactusw/3); x <= 0; x += 10) {
///for (y = 0; y <= cactush/2.25; y += 10) {
//point(x,y)
//}
//}
//for (x = -(cactusw/3); x <= 0; x += 10) {
//for (y = (-cactush/2.75); y <= 0; y += 10) {
//point(x,y)
//}
//}
//for (x = 0; x <= cactusw/2.75; x += 10) {
//for (y = (-cactush/2.75); y <= 0; y += 10) {
//point(x,y)
//}
//}
Project 5 Wallpaper
Continuing my exploration of abstracting puritst paintings of the architect Le Corbusier/ Charles Edouard Jeanneret. This week I decided to attempt to re-interpret some of the common motifs that occur in his paintings into the wall paper assignment. Purists paintings and cubist paintings alike require often require subtle differences in their repetitions of geometry to achieve their ambiguous depth and transparency, so reinterpreting them into a wall paper which inherently a tiled seamless graphic proved to be a difficult task.
//tjchen
//section A
function setup() {
createCanvas(600, 600);
background(220);
}
function draw() {
scale(1);
for ( var row = 0; row <= height; row += 80){
for(var col = 0; col <= width; col += 80){
tilebigger1(col,row);
}
}
for ( var row = 40; row <= height; row += 80){
for(var col = 0; col <= width; col += 80){
tilebigger2(col,row);
}
}
}
function tilebigger1(x,y){
tile(x,y);
push()
translate(20,20);
rotate(radians(180));
//translate(x+40,y);
tile(-x-60,-y-20);
pop();
}
//second row type
function tilebigger2(x,y){
push()
translate(20,20);
rotate(radians(180));
//translate(x+40,y);
tile(-x-20,-y-20);
pop();
tile(x+40,y);
}
function tile(x,y){
noStroke();
push();
translate(x,y);
//background
fill(146, 195, 234);
square(0,0,40);
//midground
fill(88, 96, 105);
rect(0,0,40,15);
//women/bull/violin
strokeWeight(1);
stroke(0);
fill(253, 223, 186);
beginShape();
curveVertex(40,0);
curveVertex(40,0);
curveVertex(23.9,4.56);
curveVertex(21.62,10.8);
curveVertex(24.7,16.55);
curveVertex(32.69,16.29);
curveVertex(35.72,23.64);
curveVertex(31.37,27.76);
curveVertex(23.89,29.92);
curveVertex(22.41,36.07);
curveVertex(29.58,39.75);
curveVertex(40,40);
curveVertex(40,40);
endShape();
//eyes
noFill();
strokeWeight(1);
stroke(0);
rectMode(CENTER);
rect(26.18,9.83,3,5,2);
rect(26.64,33.83,3,5,2);
//nose bottle
rectMode(CORNER);
noStroke();
fill(86, 122, 183);
rect(3.5,18.5,15,22);
fill(255);
rectMode(CENTER);
strokeWeight(1);
stroke(0);
rect(11,20,15,8,4);
fill(183, 52, 9);
noStroke();
circle(8.5,20,3);
circle(13.5,20,3);
//guitar middle
noFill();
strokeWeight(1);
stroke(0);
arc(40,20,15,15,HALF_PI,PI+HALF_PI,OPEN);
pop();
}
Project – 05 – Wallpaper
function setup() {
createCanvas(600,600);
}
function draw() {
background(255,203,134);
// background(0,24,91);
// Repeat Confetti
for (var x = 20; x < width; x += 80){
for (var y = 20; y < height; y += 185){
push();
translate(x,y);
confetti(x,y);
pop();
}
}
// Repeat Pumpkin
for (var x = 0; x < width - 100; x += 200){
for (var y = 0; y < height - 100; y += 200){
push();
translate(x,y);
pumpkin(x,y);
pop();
}
}
// Repeat Ghost
for (var x = 0; x < width - 200; x += 200){
for (var y = 0; y < height - 200; y += 200){
push();
translate(x,y);
ghost(x,y);
pop();
}
}
}
function pumpkin(a,b){
// Pumpkin Stem
noStroke();
fill(48,26,0);
triangle(95,25,105,25,100,60);
// Shadow Under Pumpkin
fill(239,177,115);
ellipse(100,145,130,35);
// Pumpkin
fill(243,132,4);
ellipse(66.5,100,50,100);
ellipse(100,100,55,100);
ellipse(133,100,50,100);
// Eyes
fill(0);
ellipse(73,90,12);
ellipse(126,90,12);
// Mouth
fill(0);
ellipse(100,120,20,30);
fill(243,132,4);
ellipse(100,110,20,15);
fill(243,132,4);
rect(95,110,8,10);
// Nose
fill(0);
triangle(93,105,100,92,107,105);
}
function confetti(a,b){
noStroke();
// Yellow Circles
fill(255,251,203);
ellipse(0,0,25);
}
function ghost(a,b){
fill(255,255,255)
noStroke();
// Body
ellipse(200,210,65,90);
ellipse(200,235,23,45);
ellipse(180,235,23,45);
ellipse(220,235,23,45);
// Eyes
fill(0);
ellipse(185,205,5);
ellipse(215,205,5);
// Mouth
ellipse(200,210,7,4);
// Cheeks
fill(255,193,221);
ellipse(183,210,7,4);
ellipse(217,210,7,4);
}
I was inspired by the fall season and Halloween festivities for this project.
Floral Wallpaper
For my wallpaper assignment, I created a floral wallpaper pattern, using geometric shapes.
https://editor.p5js.org/ssahasra/sketches/9Td-E7s4z
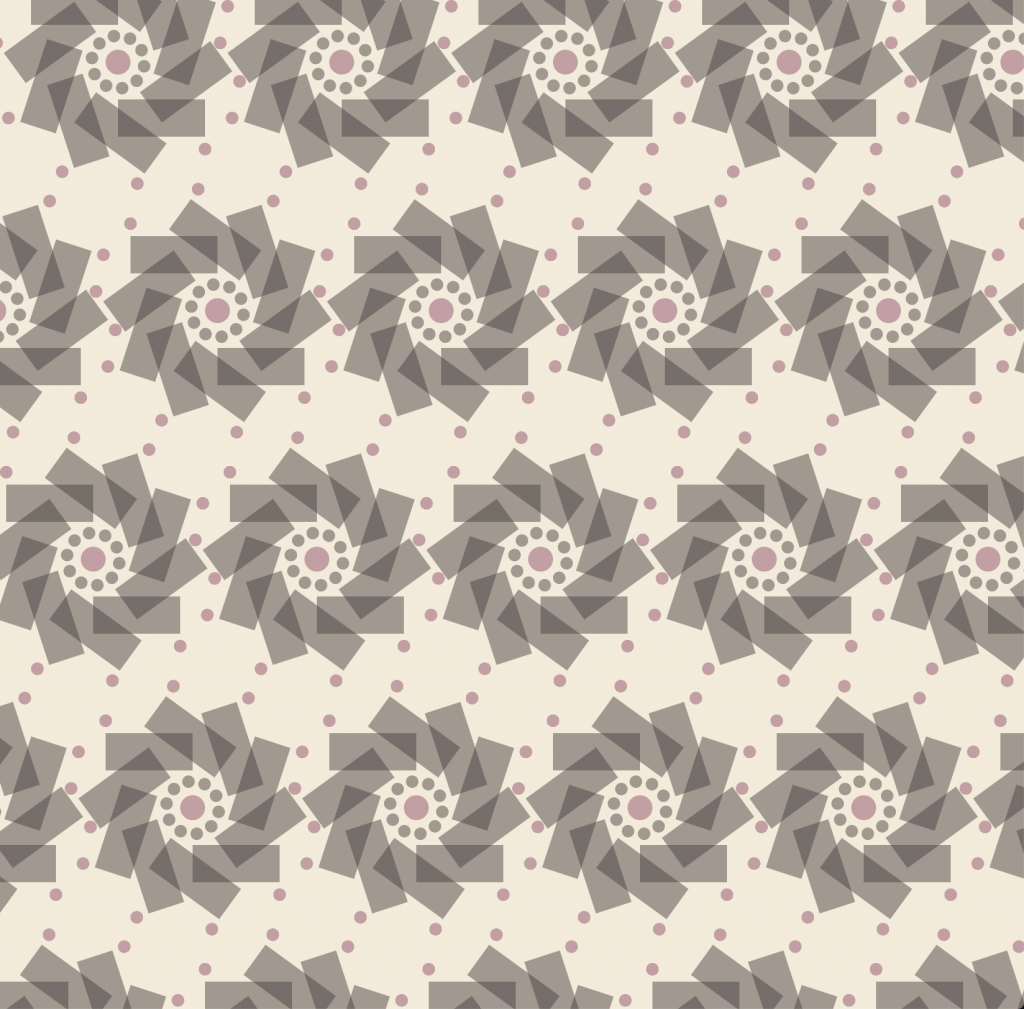
// Create the canvas
createCanvas(1200, 1200);
background(244,235,217);
}
function draw() {
background(244,235,217);
for (var y = 50; y < height; y += 200) {
for (var x = (y/2)-y; x < width; x += 180) {
flower(x+(width/10),y);
}}}
function flower(x,y) {
push();
translate(x,y);
noStroke();
fill(201,157,163);
ellipse(0,0,20,20);
for (let i = 0; i < 20; i ++) {
fill(72,61,63,70);
rect(0, 30, 70, 30);
fill(201,157,163);
ellipse(70, 70, 10, 10);
fill(72,61,63,70);
ellipse(15, 15, 10, 10);
rotate(PI/5);
}
pop();
href=”https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/10/sketch-wallpaper-sanika.js”>sketch-wallpaper-sanikaDownload
Project-05: Hexagrams
The creation of optical illusions has always intrigued me. Hexagrams have always been a favorite geometric pattern of my and most importantly, the ability to create depth by underlaying and overlaying different portions. This ended up being more difficult than I had hoped as with p5js, drawings always layer based upon where in the function each element is created. For this reason, I had to separate one side of the hexagram into two pieces to actually create the illusion I wanted which resulted in a lot of calculations and math.
//side length of the hexagon that a hexagram is based upon
var s = 50
function setup() {
createCanvas(3.5*3*s, 12*s*sqrt(3)/2);
background(150);
noLoop();
}
function draw() {
//translates the center of the canvas to the center of the first hexagram
translate(3/2*s, 2*s*sqrt(3)/2);
//loop creates the gradient effect of the background
for (var i = 0; i<width/10; i++) {
for (var j = 0; j<height/10; j++) {
push();
//translates to start at the top left corner of the canvas
translate(-3/2*s, -2*s*sqrt(3)/2);
//varies the color based on the variables of the loop
fill(255/height*j*10+50, 255/height*j,255/height*j*10+50, 255);
noStroke();
//draws squares based upon the variables of the loop
rect(i*10, j*10, 10, 10);
pop();
}
}
//loops creates the repeating pattern of hexagrams
for(var i = 0; i<width/(3*s); i++) {
for (var j = 0; j<height/(4*s*sqrt(3)/2); j++) {
push();
//translates based upon variables to draw the grid of hexagrams
translate(150*i, 4*s*sqrt(3)/2*j);
//rotates the hexagrams to create the hourglass patterns
rotate(radians(60*i));
rotate(radians(60*j));
//draws the hexagram
opticHexagram();
pop();
}
}
}
//I acknowledge code that is unused should be deleted, however the following
//block comment is necessary to show the math done to create the visual.
//Each function is a building block to the final opticHexagram
/*function hexagon() {
line(-s/2, -s*sqrt(3)/2, s/2, -s*sqrt(3)/2)
line(s/2, -s*sqrt(3)/2, s, 0)
line(s, 0, s/2, s*sqrt(3)/2)
line(s/2, s*sqrt(3)/2, -s/2, s*sqrt(3)/2)
line(-s/2, s*sqrt(3)/2, -s, 0)
line(-s, 0, -s/2, -s*sqrt(3)/2)
}
function spikes() {
triangle(-s/2, -s*sqrt(3)/2, s/2, -s*sqrt(3)/2, 0, -2*s*sqrt(3)/2);
triangle(s/2, -s*sqrt(3)/2, s, 0, 3/2 * s, -s*sqrt(3)/2);
triangle(s, 0, s/2, s*sqrt(3)/2, 3/2 * s, s*sqrt(3)/2);
triangle(s/2, s*sqrt(3)/2, -s/2, s*sqrt(3)/2, 0, 2*s*sqrt(3)/2);
triangle(-s/2, s*sqrt(3)/2, -s, 0, -3/2 * s, s*sqrt(3)/2);
triangle(-s, 0, -s/2, -s*sqrt(3)/2, -3/2 * s, -s*sqrt(3)/2);
}
function hexagram() {
noFill();
triangle(0, -2*s*sqrt(3)/2, 3/2 * s, s*sqrt(3)/2, -3/2 * s, s*sqrt(3)/2);
triangle(3/2 * s, -s*sqrt(3)/2, 0, 2*s*sqrt(3)/2, -3/2 * s, -s*sqrt(3)/2)
line(0, -2*s*sqrt(3)/2, 3/2 *s, s*sqrt(3)/2);
line(3/2 * s, s*sqrt(3)/2, -3/2 * s, s*sqrt(3)/2);
line(-3/2 * s, s*sqrt(3)/2, 0, -2*s*sqrt(3)/2);
line(3/2 * s, -s*sqrt(3)/2, 0, 2*s*sqrt(3)/2);
line(0, 2*s*sqrt(3)/2, -3/2 * s, -s*sqrt(3)/2);
line(-3/2 * s, -s*sqrt(3)/2, 3/2 * s, -s*sqrt(3)/2);
}
*/
//creates a hexagram with interlacing sides to create an optical illusion
function opticHexagram() {
push();
noStroke();
//half of the white side of the lighter triangle
//this needed to be broken into two pieces in order for the optical illusion
//to work
fill(255);
quad(0, 2*s*sqrt(3)/2,0, .8*2*s*sqrt(3)/2, .8*-3/4 * s, .8*s*sqrt(3)/4,
-3/4*s, s*sqrt(3)/4);
//black side of the dark triangle
fill(0);
quad(.8*-3/2*s, .8*s*sqrt(3)/2 , -3/2*s, s*sqrt(3)/2, 3/2*s, s*sqrt(3)/2,
.8*3/2*s, .8*s*sqrt(3)/2)
//medium side of the lighter triangle
fill(255-50);
quad(.8*3/2 * s, .8*-s*sqrt(3)/2, 3/2 * s, -s*sqrt(3)/2, 0, 2*s*sqrt(3)/2,0,
.8*2*s*sqrt(3)/2)
//medium side of the darker triangle
fill(0+50);
quad(0, -2*s*sqrt(3)/2, 0, .8*-2*s*sqrt(3)/2,.8*3/2*s, .8*s*sqrt(3)/2 ,
3/2*s, s*sqrt(3)/2);
//darkest side of the lighter triangle
fill(255-100);
quad(-3/2 * s, -s*sqrt(3)/2, .8*-3/2 * s, .8*-s*sqrt(3)/2,.8*3/2 * s,
.8*-s*sqrt(3)/2, 3/2 * s, -s*sqrt(3)/2);
//lightest side of the darker triangle
fill(0+100);
quad(0, -2*s*sqrt(3)/2, 0, .8*-2*s*sqrt(3)/2,.8*-3/2*s, .8*s*sqrt(3)/2 ,
-3/2*s, s*sqrt(3)/2);
//other half of the white side of the lighter triangle
//completes the illusion
fill(255);
quad(.8*-3/2 * s, .8*-s*sqrt(3)/2, -3/2 * s, -s*sqrt(3)/2, -3/4 * s,
s*sqrt(3)/4, .8*-3/4*s, .8*s*sqrt(3)/4)
pop();
}