lingfanj-project03
var position = -30; // set a standard position for the rectangles
var ssize = 10; // set a standard size for the rectangles
var rectcolor; //call a variable for the color of rectangles
function setup(){
createCanvas(640,480);
}
function draw(){
background(0);
var m = max(min(mouseX, 640),0); //create a new variable "m" that give mouseX a range between 0 to 640
var angle = m * 360 / 640; //remap the domain from 0-640 to 0-360 for angle
var rectcolor = m * 255 / 640; // remap the domain from 0-640
var p = position * (1 + m / 500); //make the positions bigger over time
var rectsize = ssize * (1 + m / 1000); //make the sizes of the rectangles bigger
stroke(255, rectcolor, rectcolor); //set stroke color for all the squares
strokeWeight(0.5);
translate(width/2, height/2); //translate the origin to the center of the canvas
rotate(radians(angle));
fill(255, rectcolor, rectcolor); //fill the special square
rect(p * 0.5,p,rectsize,rectsize);
//create other white squares to form a bigger square ring
fill("white");
rect(p,p,rectsize,rectsize);
rect(0,p,rectsize,rectsize);
rect(-p * 0.5,p,rectsize,rectsize);
rect(-p,p,rectsize,rectsize);
rect(p,p * 0.5,rectsize,rectsize);
rect(p,0,rectsize,rectsize);
rect(p,-p * 0.5,rectsize,rectsize);
rect(p,-p,rectsize,rectsize);
rect(p * 0.5,-p,rectsize,rectsize);
rect(0,-p,rectsize,rectsize);
rect(-p * 0.5,-p,rectsize,rectsize);
rect(-p,-p,rectsize,rectsize);
rect(-p,p * 0.5,rectsize,rectsize);
rect(-p,0,rectsize,rectsize);
rect(-p,-p * 0.5,rectsize,rectsize);
//use the scale and rotate syntax to offset a smaller ring and rotate in a different direction
push();
scale(0.5);
rotate(radians(-2 * angle));
fill(255, rectcolor, rectcolor);
rect(p * 0.5,p,rectsize,rectsize);
fill("white");
rect(p,p,rectsize,rectsize);
rect(0,p,rectsize,rectsize);
rect(-p * 0.5,p,rectsize,rectsize);
rect(-p,p,rectsize,rectsize);
rect(p,p * 0.5,rectsize,rectsize);
rect(p,0,rectsize,rectsize);
rect(p,-p * 0.5,rectsize,rectsize);
rect(p,-p,rectsize,rectsize);
rect(p * 0.5,-p,rectsize,rectsize);
rect(0,-p,rectsize,rectsize);
rect(-p * 0.5,-p,rectsize,rectsize);
rect(-p,-p,rectsize,rectsize);
rect(-p,p * 0.5,rectsize,rectsize);
rect(-p,0,rectsize,rectsize);
rect(-p,-p * 0.5,rectsize,rectsize);
pop();
//use the scale and rotate syntax to offset a bigger ring and rotate in different direction
push();
scale(2.2);
rotate(radians(-2 * angle));
fill(255, rectcolor, rectcolor);
rect(p * 0.5,p,rectsize,rectsize);
fill("white");
rect(p,p,rectsize,rectsize);
rect(0,p,rectsize,rectsize);
rect(-p * 0.5,p,rectsize,rectsize);
rect(-p,p,rectsize,rectsize);
rect(p,p * 0.5,rectsize,rectsize);
rect(p,0,rectsize,rectsize);
rect(p,-p * 0.5,rectsize,rectsize);
rect(p,-p,rectsize,rectsize);
rect(p * 0.5,-p,rectsize,rectsize);
rect(0,-p,rectsize,rectsize);
rect(-p * 0.5,-p,rectsize,rectsize);
rect(-p,-p,rectsize,rectsize);
rect(-p,p * 0.5,rectsize,rectsize);
rect(-p,0,rectsize,rectsize);
rect(-p,-p * 0.5,rectsize,rectsize);
pop();
//use the scale and rotate syntax to offset a bigger ring
push();
scale(5);
fill(255, rectcolor, rectcolor);
rect(p * 0.5,p,rectsize,rectsize);
fill("white");
rect(p,p,rectsize,rectsize);
rect(0,p,rectsize,rectsize);
rect(-p * 0.5,p,rectsize,rectsize);
rect(-p,p,rectsize,rectsize);
rect(p,p * 0.5,rectsize,rectsize);
rect(p,0,rectsize,rectsize);
rect(p,-p * 0.5,rectsize,rectsize);
rect(p,-p,rectsize,rectsize);
rect(p * 0.5,-p,rectsize,rectsize);
rect(0,-p,rectsize,rectsize);
rect(-p * 0.5,-p,rectsize,rectsize);
rect(-p,-p,rectsize,rectsize);
rect(-p,p * 0.5,rectsize,rectsize);
rect(-p,0,rectsize,rectsize);
rect(-p,-p * 0.5,rectsize,rectsize);
pop();
//use the scale and rotate syntax to offset the biggest ring rotate in different direction
push();
rotate(radians(-2 * angle));
scale(12);
fill(255, rectcolor, rectcolor);
rect(p * 0.5,p,rectsize,rectsize);
fill("white");
rect(p,p,rectsize,rectsize);
rect(0,p,rectsize,rectsize);
rect(-p * 0.5,p,rectsize,rectsize);
rect(-p,p,rectsize,rectsize);
rect(p,p * 0.5,rectsize,rectsize);
rect(p,0,rectsize,rectsize);
rect(p,-p * 0.5,rectsize,rectsize);
rect(p,-p,rectsize,rectsize);
rect(p * 0.5,-p,rectsize,rectsize);
rect(0,-p,rectsize,rectsize);
rect(-p * 0.5,-p,rectsize,rectsize);
rect(-p,-p,rectsize,rectsize);
rect(-p,p * 0.5,rectsize,rectsize);
rect(-p,0,rectsize,rectsize);
rect(-p,-p * 0.5,rectsize,rectsize);
pop();
}
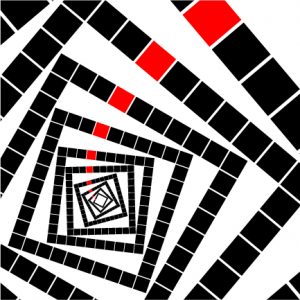
I got the composition idea from another elective I am taking called communication design. The assignment was to just use squares and to create different kinds of compositions. Since the composition has a strong motion in it, I thought it would be a good idea to do it in this project as well.