// Nawon Choi
// nawonc@andrew.cmu.edu
// Section C
// Project 06 Abstract Clock
var prevSec;
var millisRolloverTime;
var ons = ['zero', 'one', 'two', 'three', 'four', 'five', 'six', 'seven', 'eight', 'nine'];
var tns = ['ten', 'eleven', 'twelve', 'thirteen', 'fourteen', 'fifteen', 'sixteen', 'seventeen', 'eighteen', 'nineteen'];
var tnspls = ['twenty', 'thirty', 'forty', 'fifty', 'sixty', 'seventy', 'eighty', 'ninety'];
var milli;
var sec;
var mins;
var hr;
function setup() {
createCanvas(400, 400);
millisRolloverTime = 0;
}
function draw() {
background("black");
// Fetch the current time
var H = hour();
var M = minute();
var S = second();
if (prevSec != S) {
millisRolloverTime = millis();
}
prevSec = S;
var mils = floor(millis() - millisRolloverTime);
textFont("Helvetica");
textAlign(CENTER, CENTER);
// increase in size based on time
fill(50);
textSize(mils);
textStyle(NORMAL);
var mm = int(mils / 10);
milli = toWords(mm);
text(milli, width / 2, height / 2);
fill(75);
textSize(S * 5);
textStyle(NORMAL);
sec = toWords(S);
text(sec, width / 2, height / 2);
fill(150);
textSize(M * 2);
textStyle(NORMAL);
mins = toWords(M);
text(mins, width / 2, height / 2);
fill(230);
textSize(H);
textStyle(NORMAL);
hr = toWords(H);
text(hr, width / 2, height / 2);
}
function toWords(x) {
if (x < 10) {
return ons[x];
} else if (x >= 10 & x < 20) {
var i = x - 10;
return tns[i];
} else {
if (x % 10 == 0) {
var i = (x / 10) - 2;
return tnspls[i];
} else {
var i = x % 10;
var o = ons[i];
var ii = int(x / 10) - 2;
var t = tnspls[ii];
var arr = [t, o];
return join(arr, "");
}
}
}
When thinking of ideas for this assignment, I initially brainstormed a variety of different pictorial representations of the passage of time. I eventually decided to go with a text-based clock after seeing this image online. I thought it was interesting to see time represented textually rather than in an analog or digital form.
The most challenging part of this assignment was converting the number of the time into text. I could only convert numbers up to 99, so for milliseconds, the number converted is divided by 10. I figured it didn’t matter as much because the milliseconds are illegible. I also had the size of the text increase based on the time.
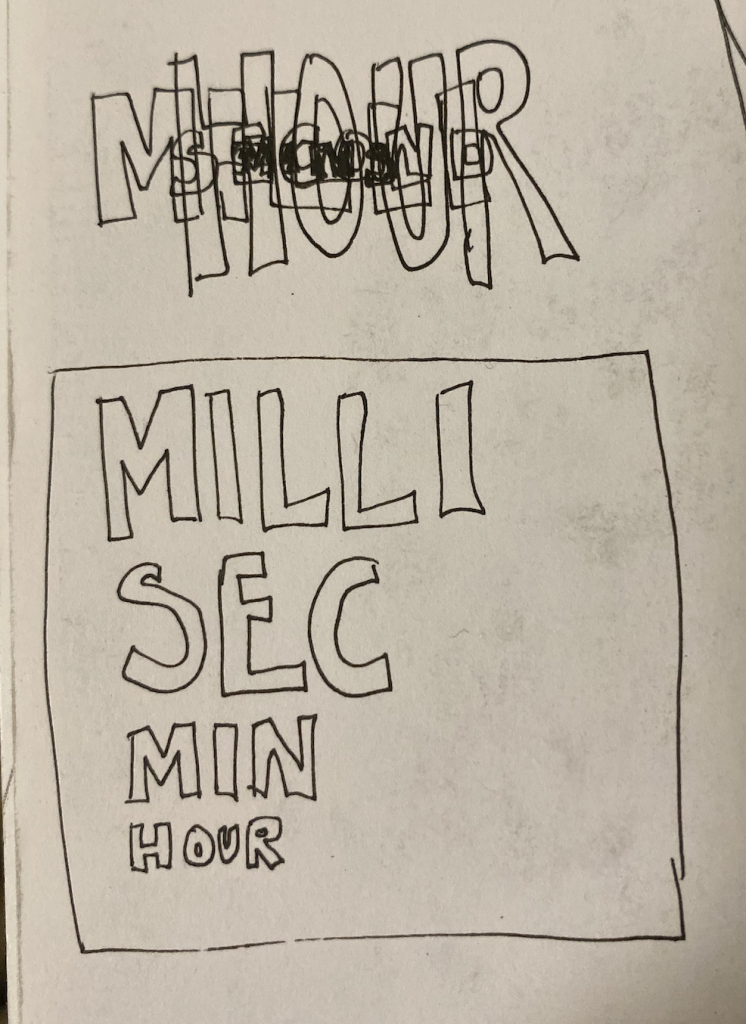