// CJ Walsh
// Section D
// cjwalsh@andrew.cmu.edu
// Project-06
var cx, cy;
var sunDim, hourDim, minDim, secDim;
var hx, hy;
var mx, my;
var sx, sy;
var tx = [];
var ty = [];
var starNum;
var colors = ['white', '#D8B495', '#DBD8A2'];
function setup() {
createCanvas(480, 480);
cx = width/2;
cy = height/2;
sunDim = 0.2*480; // establishing variables for the sun and orbit paths
hourDim = 0.55*480;
minDim = 0.85*480;
secDim = 70;
starNum = 150;
// loop created for generation of stars
for (i = 0; i < starNum; i++) {
tx[i] = random(0, width);
ty[i] = random(0, height);
}
}
function draw() {
background('black');
// drawing background of stars
for (i = 0; i < starNum; i++) {
fill(colors[i%3]);
ellipse(tx[i], ty[i], 5, 5);
}
var s = second(); // establishing variables for sec, min, hr
var h = hour() % 12; // hours set to 12 hr day rather than 24hr
var m = minute();
var sAngle = s/60*2*PI - PI/2; // establishing variables for angle of hands
var mAngle = m/60*2*PI - PI/2 + s/60/60*2*PI;
var hAngle = h/12*2*PI - PI/2 + m/60/12*2*PI;
// drawing paths of orbit
noFill();
stroke('#919191');
strokeWeight(2);
ellipse(cx, cy, hourDim, hourDim);
ellipse(cx, cy, minDim, minDim);
// drawing sun and radiating lines
noStroke();
fill('#DB5858');
ellipse(cx, cy, sunDim, sunDim);
noFill();
stroke('#FFC269');
for (s = sunDim; s <= sunDim+4*12; s+=12) {
ellipse(cx, cy, s, s);
}
// Hour Planet
hx = cx + hourDim/2*cos(hAngle);
hy = cy + hourDim/2*sin(hAngle);
drawHour();
// Minute Planet
mx = cx + minDim/2*cos(mAngle);
my = cy + minDim/2*sin(mAngle);
drawMin();
// Second Moon
sx = mx + secDim/2*cos(sAngle);
sy = my + secDim/2*sin(sAngle);
drawSec();
}
function drawHour() {
noStroke();
fill('#DD6C9F');
ellipse(hx, hy, 50, 50);
fill('#844364');
ellipse(hx+14, hy+3, 15, 15);
ellipse(hx-6, hy-5, 5, 5);
ellipse(hx-10, hy+10, 10, 10);
}
function drawMin() {
noStroke();
fill('#4A74A5');
ellipse(mx, my, 35, 35);
fill('#303C6D');
ellipse(mx-3, my-6, 17, 13);
ellipse(mx+4, my+6, 6, 6);
}
function drawSec() {
noStroke();
fill('#73B3FF');
ellipse(sx, sy, 12, 12);
}
For this project I wanted to create a clock that looked like planets orbiting a sun. Although the design is still centered around circular themes, I think its still breaking outside the idea of a clock, and definitely couldnt be identified as a clock by first glance. My process began with sketching my ideas on paper, and then translating that into a drawing using Adobe Illustrator. After that I was able to translate my sketches into code, creating both fixed and animated objects on my canvas. I used the knowledge I gained from Assignment C to help me create my new clock. I added in the ability of the planets to move slightly in the transition from minute to minute or hour to hour. As a final touch, I drew some randomly generated stars to fill the background. This was definitely a fun project to make, though one that challenged my knowledge.
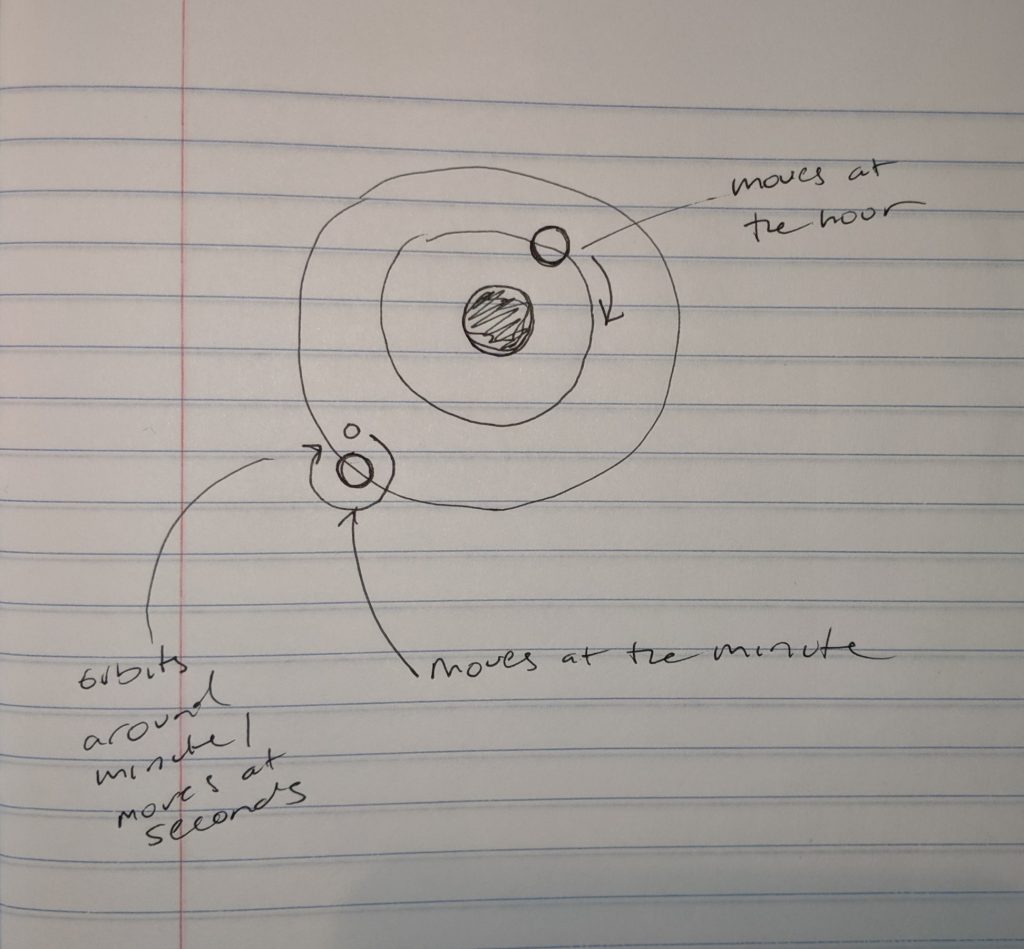
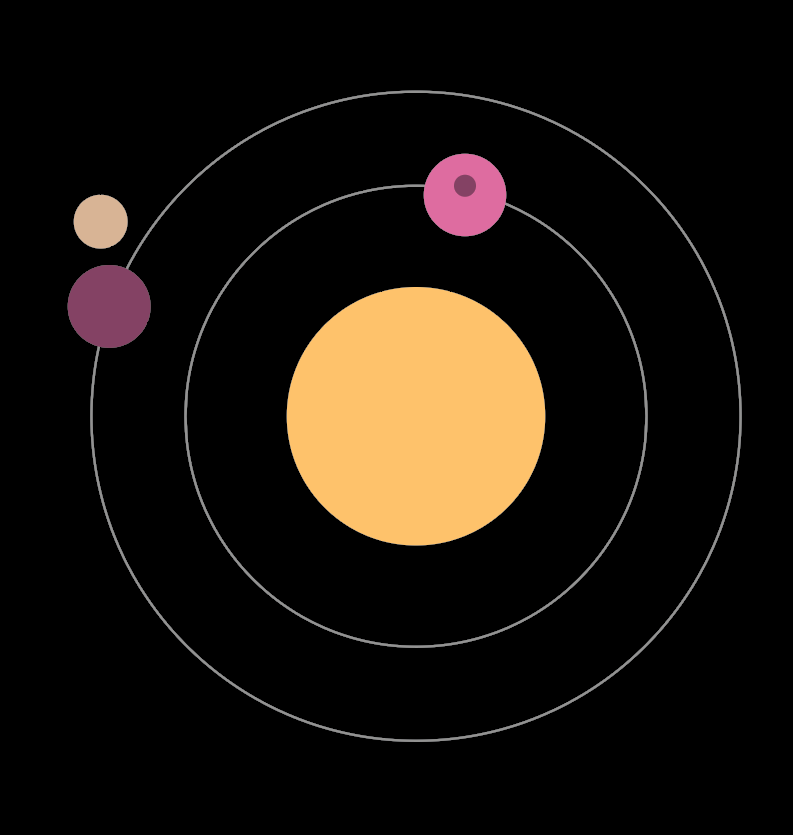