/*
Joanne Chui
Section C
Project 8
*/
var underlyingImage;
function preload() {
var myImageURL = "https://i.imgur.com/cYVj323.png";
underlyingImage = loadImage(myImageURL);
}
function setup() {
createCanvas(500, 500);
background(0);
underlyingImage.loadPixels();
frameRate(100000);
}
function draw() {
var px = random(width);
var py = random(height);
var ix = constrain(floor(px), 0, width-1);
var iy = constrain(floor(py), 0, height-1);
var theColorAtLocationXY = underlyingImage.get(ix, iy);
var value = brightness(theColorAtLocationXY);
var scaleBrush = map(value, 0, 225, 0, 50);
noStroke();
fill(theColorAtLocationXY);
ellipse(px, py, scaleBrush, scaleBrush);
}
function mouseDragged(){
var theColorAtTheMouse = underlyingImage.get(mouseX, mouseY);
noStroke();
fill(theColorAtTheMouse);
var brushSize = random(10, 50);
ellipse(mouseX, mouseY, brushSize, brushSize);
}
I varied the circles that populated the picture based on the brightness. Where the image is brighter, the size of the circle would be bigger. I also included an interactive portion where the user would be able to brush in the photo if they dragged the mouse, creating a more dynamic and abstract image.
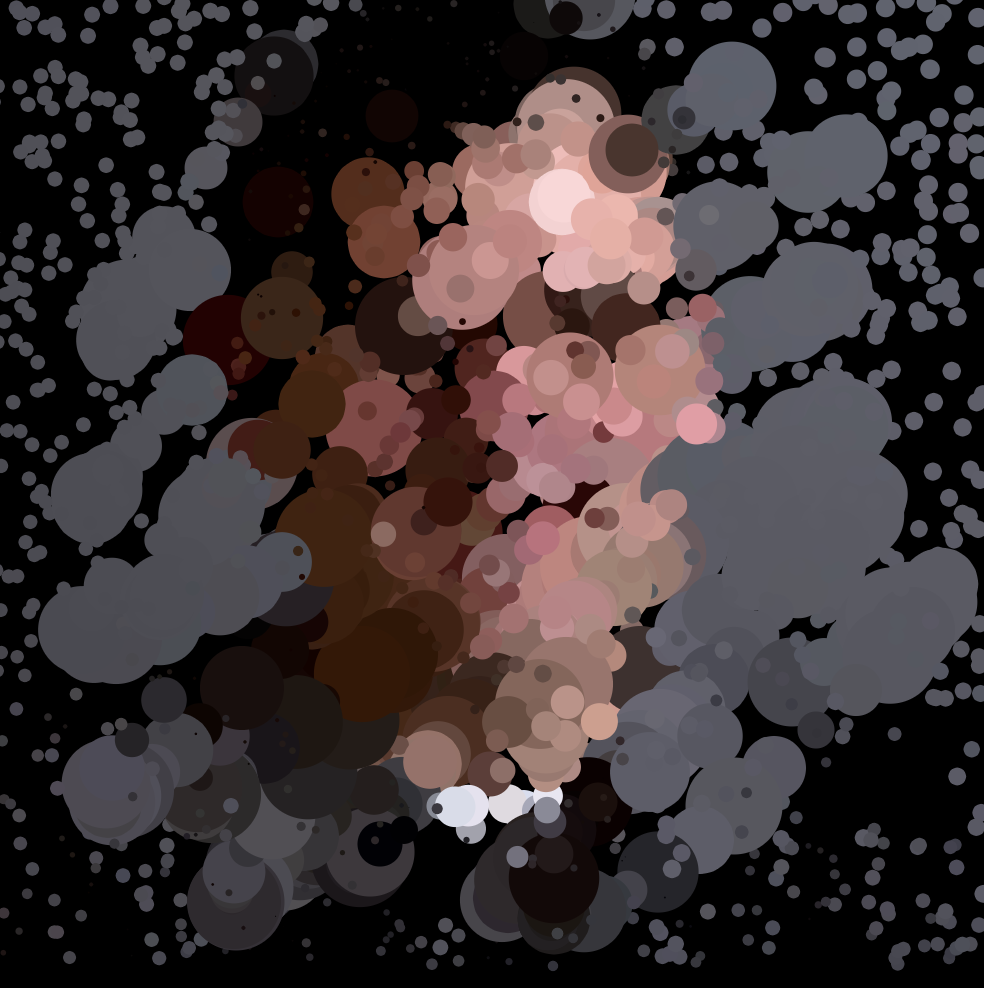
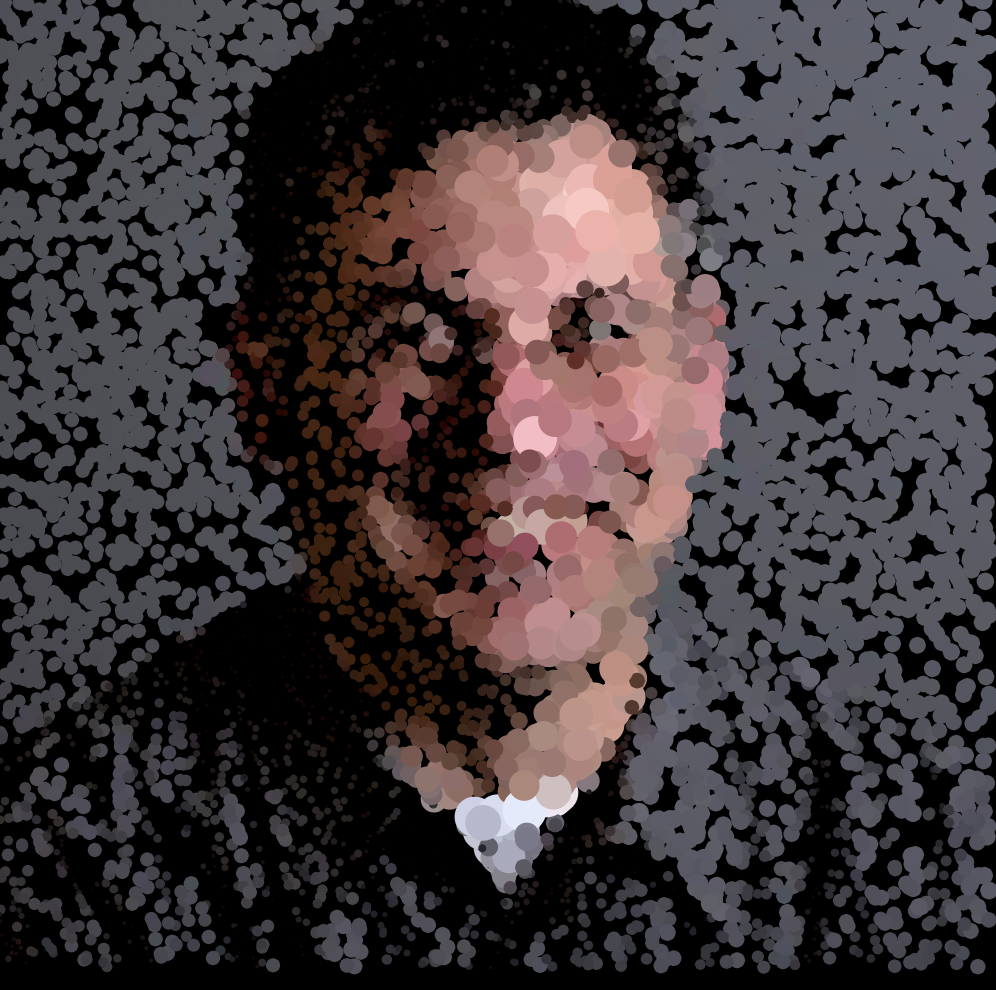
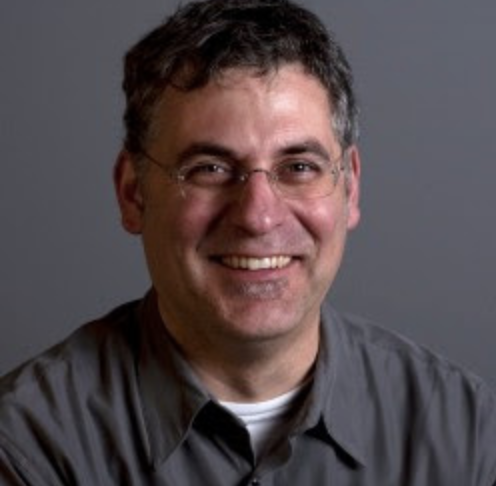