/*
* Angela Lee
* Section E
* ahl2@andrew.cmu.edu
* Project 5 Wallpaper
*/
// spacing between circles
var spacing = 200;
function setup() {
createCanvas(600, 600);
var orangeBack = color(241, 94, 39); // orange color
var blueBack = color(69, 95, 172); // blue color
setGradient(orangeBack, blueBack); // background gradient
}
function draw() {
// y position for loop
for (var y = 50; y <= height - 50; y += spacing) {
// x position for loop
for (var x = 50; x <= width - 50; x += spacing) {
// calling the two functions for drawing the circles
orangeCircles(x, y);
blueCircles(x, y);
}
}
}
// Function for creating my gradient background
function setGradient(color1, color2) {
for(var a = 0; a <= height; a++) {
var amount = map(a, 0, height, 0, 1);
var back = lerpColor(color1, color2, amount);
stroke(back);
line(0, a, width, a);
}
}
// Orange circle function
function orangeCircles(x, y) {
noStroke();
//outer circle
fill(241, 94, 39);
ellipse(x, y, 100, 100);
// second-most outer circle
fill(243, 109, 66);
ellipse(x, y, 75, 75);
// third-most outer circle
fill(241, 135, 101);
ellipse(x, y, 50, 50);
// inner circle
fill(248, 161, 117);
ellipse(x, y, 25, 25);
}
// Blue circle function
function blueCircles(x, y) {
noStroke();
// blue circles are shifted right and down
x = x + 100;
y = y + 100;
//outer circle
fill(69, 95, 172);
ellipse(x, y, 100, 100);
// second-most outer circle
fill(87, 114, 183);
ellipse(x, y, 75, 75);
// third-most outer circle
fill(114, 141, 199);
ellipse(x, y, 50, 50);
// inner circle
fill(147, 173, 218);
ellipse(x, y, 25, 25);
}
I personally love playing with complementary colors, so I wanted to experiment more with complementary contrasts in the wallpaper. In my initial plan, I sketched out semicircles, but I didn’t like how they created harsh edges in the composition. I chose the colors blue and orange and created circular patterns with them. I also wanted to try playing with gradients, so for the background, I created a smooth gradient and for the circles, I created gradient intervals of colors. I alternated between orange and circle to create a balance of colors.
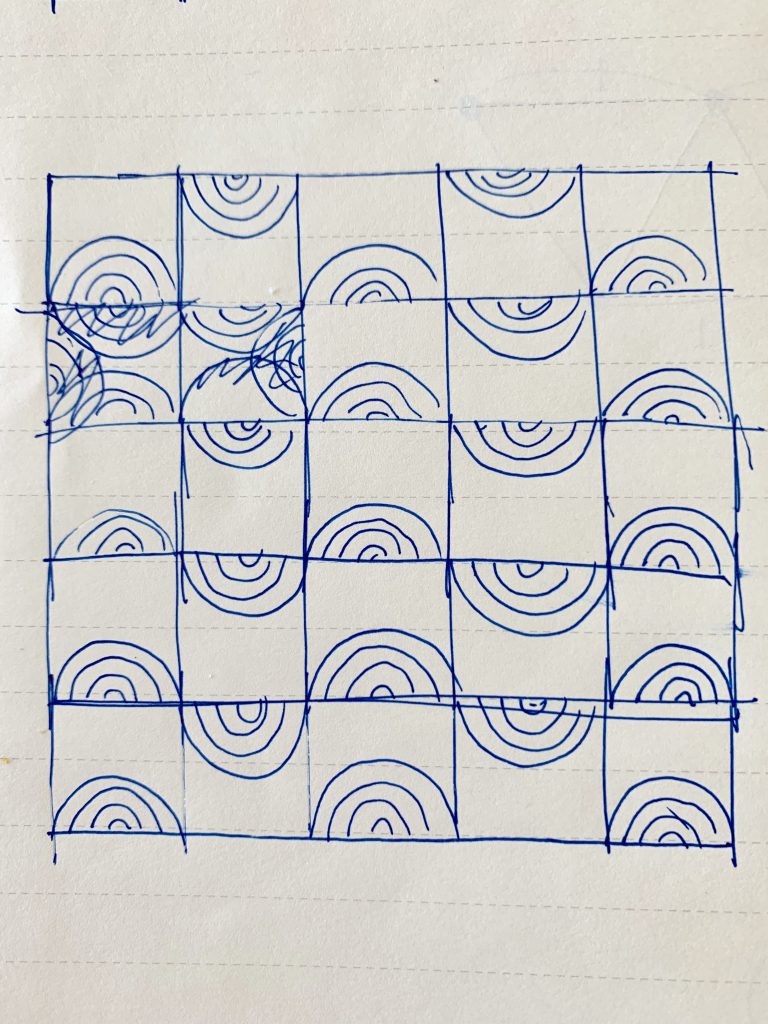