//Monica Chang
//mjchang@andrew.cmu.edu
//Section D
//Project 06 - Abstract Clock
var prevSec;
var millisRolloverTime;
var rVal = 50;
var bVal = 50;
//--------------------------
function setup() {
createCanvas(480, 480);
millisRolloverTime = 0;
frameRate(5);
r = random(255);
g = random(255);
b = random(255);
}
//--------------------------
function draw() {
background(0);
//millis is represented in drawGrid
drawGrid();
// Fetch the current time
var H = hour();
var M = minute();
var S = second();
var a = H*3600 + M*60 + S;
// Reckon the current millisecond,
// particularly if the second has rolled over.
// Note that this is more correct than using millis()%1000;
if (prevSec != S) {
frameRate(25);
millisRolloverTime = millis();
}
prevSec = S;
var mils = floor(millis() - millisRolloverTime);
fill(128,100,100);
stroke(128,100,100);
text("Hour: " + H, 10, 22);
text("Minute: " + M, 10, 42);
text("Second: " + S, 10, 62);
text("Millis: " + mils, 10, 82);
var hourCircSize = map(H, 0, 23, 0, width);
var minuteBarWidth = map(M, 0, 59, 0, width);
var blueChange = map(S, 0, 60, 0, 255); //blue changing color
var radiusChange = map(S, 0, 60, 0, 400); //increasing circle size
// hours
fill(3);
noStroke();
ellipse(width / 2, height / 2, hourCircSize / 2);
//minutes
rectMode(CORNER);
noStroke();
fill(20);
rect(50, height / 2, 380, 20);//darker background
noStroke();
fill(r, g, b);
rect(50, height / 2, minuteBarWidth, 20); //minutes passed
strokeWeight(1);
//seconds: empty, blue circles getting bigger and bigger.
noFill();
stroke(66, blueChange, 244);
ellipse(width / 2, height / 2, radiusChange);
a = a + 1;
//analog clock
fill(128,100,100);
noStroke();
textAlign(LEFT);
text(H % 12, width / 2 - 20, 460);
textAlign(CENTER);
text(M, width / 2 , 460);
textAlign(LEFT);
text(S, width / 2 + 7, 460);
}
//millis
function drawGrid() {
// noprotect
for (var y = 0; y < 490; y += 10) {
for (var x = 0; x < 650; x += 10) {
frameRate(millis());
rVal = (rVal - 1) % 200;
bVal = (bVal + 3) % 200;
//color gradient
fill(rVal, 0, bVal);
noStroke();
ellipse(x, y, 10, 10);
}
}
}
It was really interesting how much I had to think about the readability of this concept. To make something that we look at daily, making it abstract can arguably take more time for it to function regularly like it does now for it. With this idea, I concentrated on abstracting the form of the clock while also allowing it to still require not that much cognitive processing to understand.
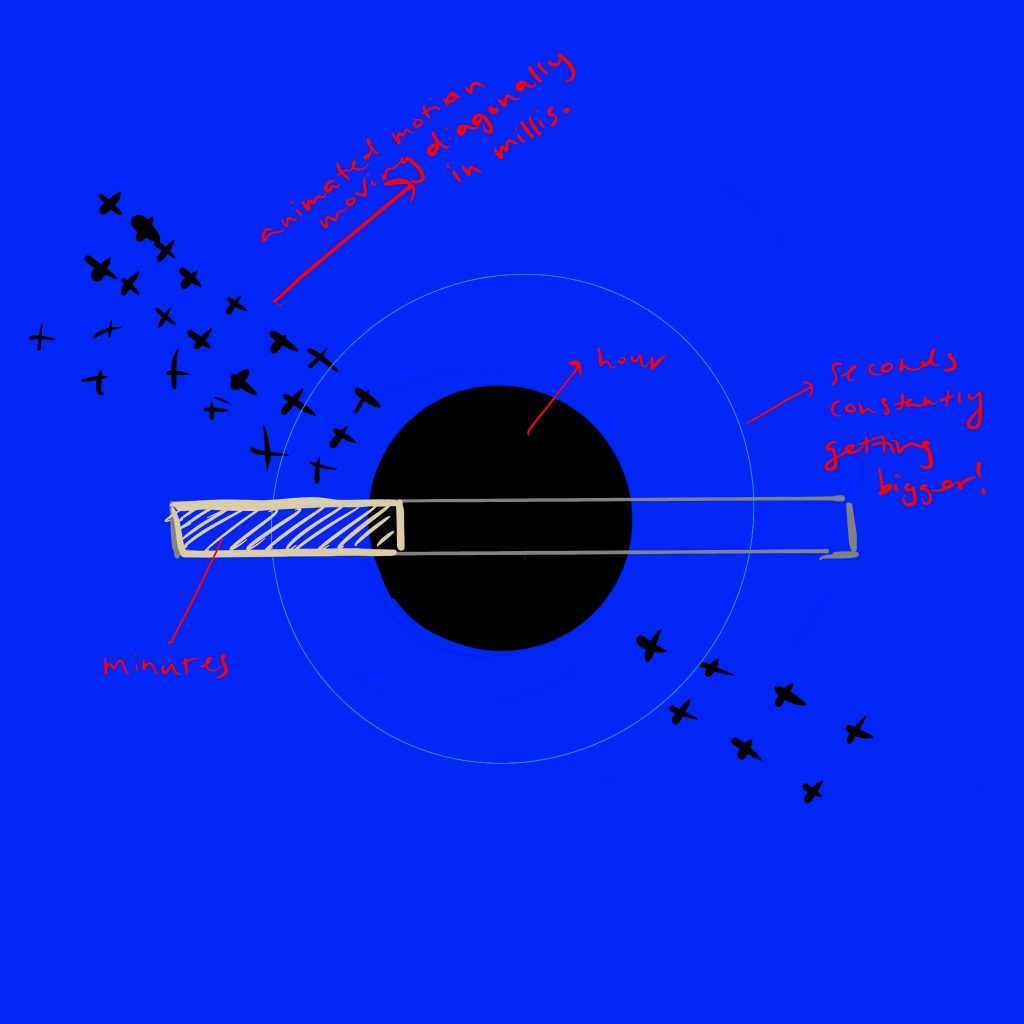