//Sammie Kim
//Section D
//sammiek@andrew.cmu.edu
//Project 06 - Abstract Clock
function setup(){
createCanvas(400,600);
}
function draw(){
background(0);
//variables for time
var s = second();
var m = minute();
var hr = hour();
//Shining stars and black night background
for (var i = 0; i < 100; i ++) {
frameRate(2);
fill(225);
var eSize = random(1, 5);
var x = random(0, width);
var y = random(0, height);
ellipse(x, y, eSize, eSize);
}
//Mapping time to shape components
var secIncrease = map(s, 0,59, 0, height);
var minIncrease = map(m,0, 59, 50, height/2);
var hrIncrease = map(hr, 0, 23, 100, height * 3/4);
//Every minute, the blue sky background will decrease, slowly revealing the space
fill(102, 153, 255);
rect(0, 0, width, height - minIncrease);
//Rocket flies upwards every second
fill(78, 78, 252);
triangle(225, 525 - secIncrease, 190, 570 - secIncrease, 260, 570 -secIncrease);
fill(252, 84, 78)
rect(190, 570 - secIncrease, 70, 100);
stroke(0);
strokeWeight(10);
fill(255);
ellipse(225, 600 - secIncrease, 30, 30);
noStroke();
fill(252, 153, 78);
triangle(190, 670 - secIncrease, 225, 730 - secIncrease, 260, 670 - secIncrease);
fill(170, 110, 196);
triangle(190, 670 - secIncrease, 190, 710 - secIncrease, 230, 670 - secIncrease);
//Pink circle is the sun, which is changing color every hour
//The sun also goes down every hour
noStroke();
fill(hrIncrease, 180, 220);
ellipse(100, hrIncrease, 60, 60);
}
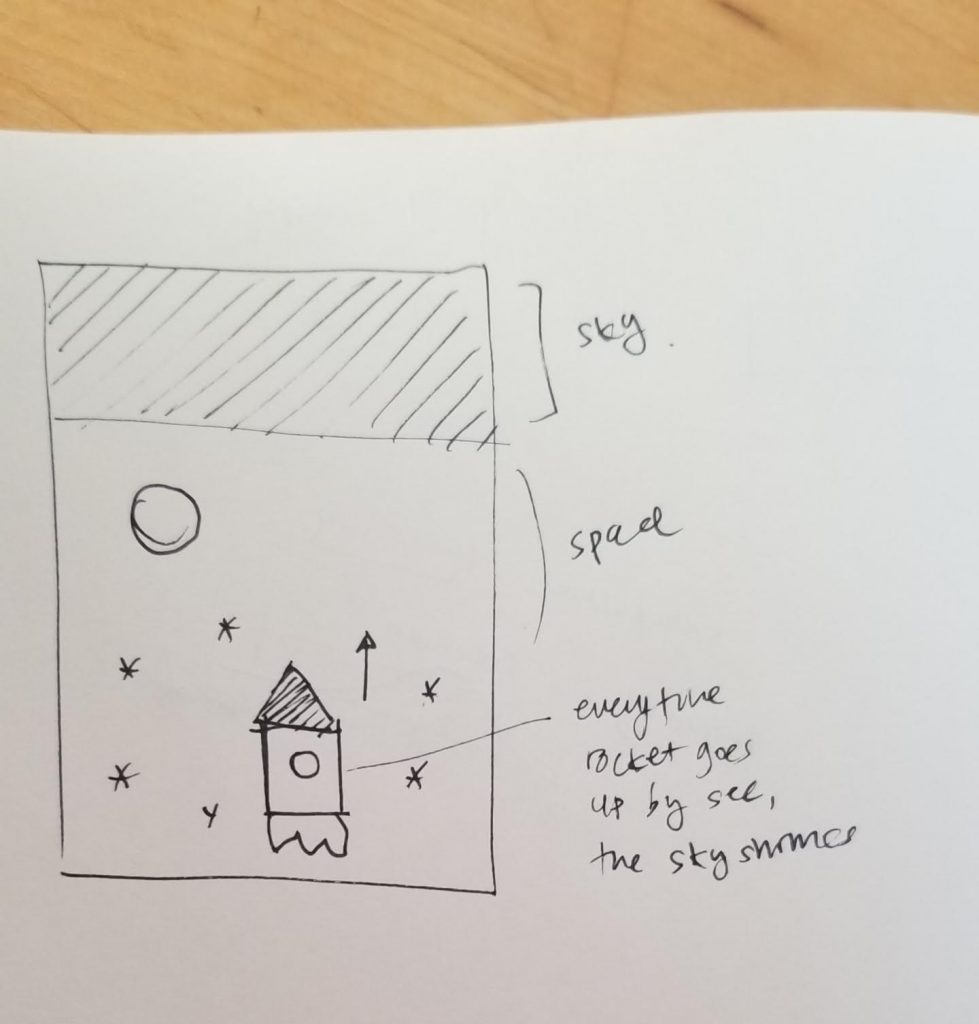
This project was challenging as I had to analyze how to incorporate the time concept into my canvas, letting each element pace by a specific rate of time. I imagined a rocket flying up by each second, and the sky slowly disappearing along as the rocket goes further up. The sun would also go down at the same time by every hour. With this, I got to review the map function once more, as I got to think about the range that is needed to limit the movement of the rocket. Also it took some time figuring out how to move the rocket in second increments up, which I eventually did by subtracting “secIncrease,” since the coordinates get smaller as it gets nearer to the top.