/*
* Angela Lee
* Section E
* ahl2@andrew.cmu.edu
* Project 9 Computational Portrait
*/
var underlyingImage;
// loading the image
function preload() {
var url = "https://i.imgur.com/GsniIVQ.jpg";
underlyingImage = loadImage(url);
}
function setup() {
createCanvas(300, 400);
background(145, 1, 10);
underlyingImage.loadPixels();
frameRate(60); // how fast the pixels come up
}
function draw() {
var px = random(width);
var py = random(height);
var ix = constrain(floor(px), 0, width);
var iy = constrain(floor(py), 0, height);
var theColorAtLocationXY = underlyingImage.get(ix, iy);
noStroke();
fill(theColorAtLocationXY);
ellipseW = random(1, 8); // original ellipse width
ellipseH = random(1, 8); // original ellipse height
keyPressed(); // key pressed determines ellipse width and height
// drawing the ellipse
ellipse(px, py, ellipseW, ellipseH);
}
// what happens if the key is pressed
function keyPressed() {
// ellipse will grow if g is pressed
if (key == "g") {
ellipseW = random (5, 15);
ellipseH = random(5, 15);
} else if (key == "s") {
// ellipse will shrink if s is pressed
ellipseW = random(0.5, 3);
ellipseH = random(0.5, 3);
} else if (key == "n") {
// ellipses will return to original size
ellipseW = random(1, 8);
ellipseH = random(1, 8);
}
return ellipseW;
return ellipseH;
}
For this portrait, I started out with ellipses being randomized for a width and height ranging from 1 to 5. However, I got impatient while waiting for the portrait to finish, so I decided to make the ellipse grow when you pressed the key “g.” But since I didn’t like how blurry the portrait got, I gave the user the option of returning the ellipses back to its original size or an even smaller size.
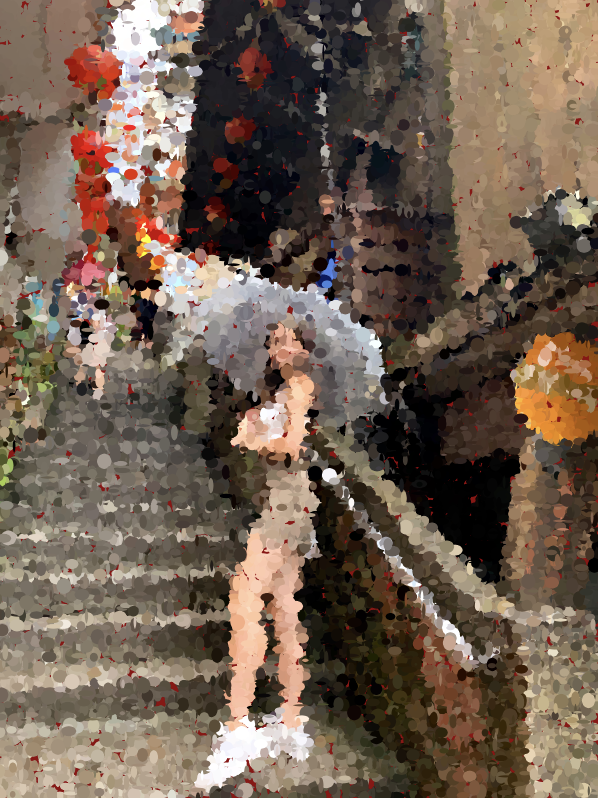
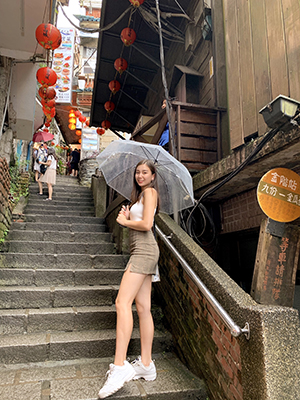