/*
Joanne Chui
Section C
Project 11
*/
var terrainSpeed = 0.0001;
var terrainDetail = 0.005;
var riverDetail = 0.0005
var birds = [];
var c1, c2;
function setup() {
createCanvas(480, 240);
frameRate(10);
for(i = 0; i < 10; i++){
var rx = random(width);
var ry = random(height);
birds[i] = makeBird(rx, ry);
}
}
function draw() {
// background(220, 220, 230);
c1 = color(170, 170, 190);
c2 = color(240, 190, 120);
setGradient(c1, c2);
//sun
noStroke();
fill(210, 100, 40);
ellipse(width/2, 160, 170, 170);
//call landscape and birds
drawMountain();
updateBird();
addBird();
removeBird();
}
//background gradient
function setGradient(c1, c2){
noFill();
for(i = 0; i < height; i++){
var inter = map(i, 0, height, 0, 1);
var c = lerpColor(c1, c2, inter);
stroke(c);
line(0, i, width, i);
}
}
function drawMountain(){
//furthest mountain
fill(55, 63, 82);
noStroke();
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0,1, 0, height);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
//middle river
fill(220, 180, 150);
noStroke();
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * riverDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0,1, height/2, height);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
//closest mountain
fill(170, 120, 40);
noStroke();
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed*4);
var y = map(noise(t), 0,2, 0, height);
vertex(x, y*3);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function makeBird(birdX, birdY){
var bird = {
x: birdX,
y: birdY,
speed: random(5, 20),
size: random(5, 20),
color: random(0, 100),
move: birdMove,
display: displayBird
}
return bird;
}
function birdMove(){
this.x += this.speed;
this.y -= this.speed / 5;
}
function displayBird(){
strokeWeight(2);
stroke(this.color);
noFill();
push();
translate(this.x, this.y);
arc(0, 0, this.size, this.size, PI + QUARTER_PI, 0);
arc(this.size, 0, this.size, this.size, PI, 0 - QUARTER_PI);
pop();
}
function updateBird(){
for(i = 0; i < birds.length; i++){
birds[i].move();
birds[i].display();
}
}
//adding birds with a random probability
function addBird(){
var newBird = random(1);
if(newBird < 0.2){
var birdX = 0;
var birdY = random(height);
birds.push(makeBird(birdX, birdY));
}
}
//removing birds from the array once they fall off the canvas
function removeBird(){
var keepBird = [];
for(i = 0; i < birds.length; i++){
if(birds[i].x + birds[i].size > 0){
keepBird.push(birds[i]);
}
}
birds = keepBird;
}
I was interested in creating a landscape with many layers to show depth. I was interested in creating birds because i felt that they would add a dynamic movement in the landscape.
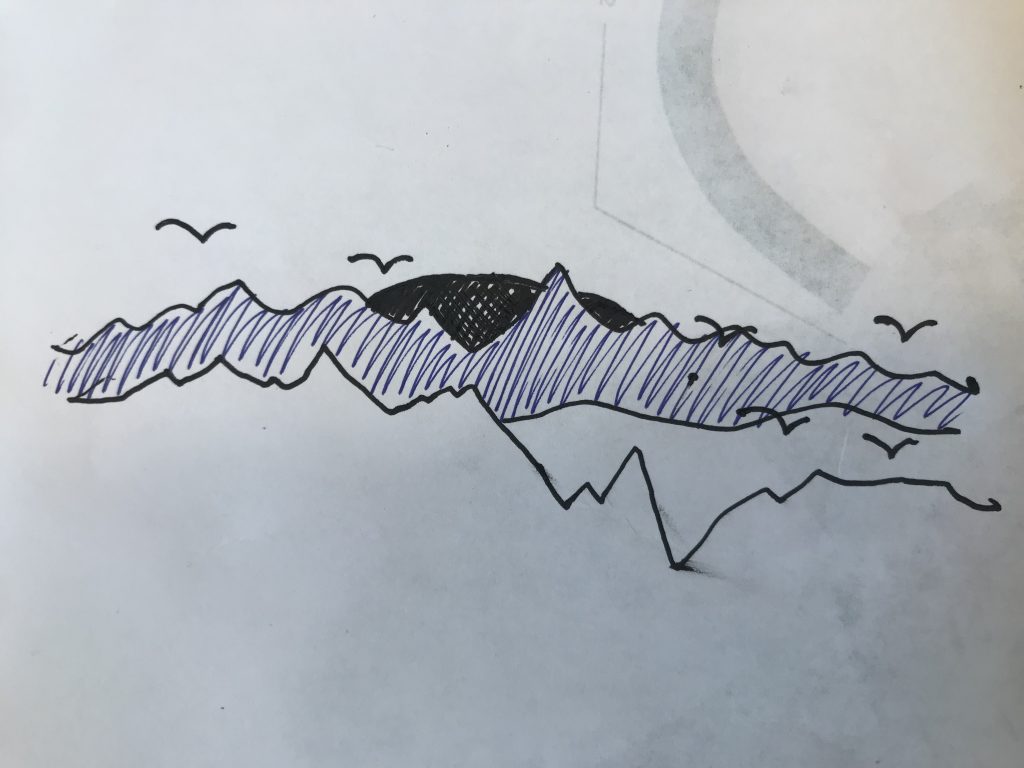