/* Ammar Hassonjee
ahassonj@andrew.cmu.edu
Section C
Project 11
*/
// global variables and arrays declared to hold objects
var traintracks = [];
var tracky = 0; //position of train tracks
var oases = []; // array for oases objects
var trees = []; // array for tree objects
function setup() {
createCanvas(480, 480);
// for loop that pushed train track objects to array
for (var i = 0; i < 480; i += 20) {
traintracks.push(makeTrainTracks(210, i));
// creates a random amount of oases to add to the oases array each time
// the program is loaded
if (i % 240 === 0) { //random iteration
// variables initialized to pass as parameters for making the oases objects
var xloc = int(random(width / 4));
var yloc = int(random(height));
var shade = int(random(200, 255));
var xsize = int(random(60, 100));
var ysize = int(random(100, 150));
oases.push(makeOasis(xloc, yloc, shade, xsize, ysize));
}
}
frameRate(20);
}
function draw() {
background(250, 245, 200);
fill(97, 74, 66);
rect(200, 0, 10, height); // train track boundaries
rect(270, 0, 10, height); // train track boundaries
strokeWeight(1);
// calling the track functions to continously move the tracks and add oases objects to canvas
updateTracks();
removeTracks();
addTracks();
// function called for drawing the train object
drawTrain();
}
// updating the position of each track and oases in each of the arrays
function updateTracks() {
// for loop that calls train track objects and moves/displays them
for (var i = 0; i < traintracks.length; i++) {
traintracks[i].move();
traintracks[i].display();
}
// for loop that calls oases objects and moves/displays them
for (var j = 0; j < oases.length; j++) {
oases[j].move();
oases[j].display();
}
// for loop that calls tree objects and moves/displays them
for (var k = 0; k < trees.length; k++) {
trees[k].move();
trees[k].display();
}
}
function addTracks() {
var position = traintracks[traintracks.length - 1].y; // initializing traintracks position
traintracks.push(makeTrainTracks(210, position + 20)); // adding a traintracks object to the end of the array
var chance = int(random(0, 40)); // making sure the chance of appending an oases object is low
if (chance === 2) {
// variables declared as parameters to pass to the oases object
var xloc = int(random(width / 4));
var yloc = height;
var shade = int(random(200, 255));
var xsize = int(random(40, 60));
var ysize = int(random(100, 400));
oases.push(makeOasis(xloc, yloc, shade, xsize, ysize));
}
// varying the chance of the trees appearing
var chance2 = int(random(0, 25));
if (chance2 === 4) { // making sure the trees appear on both sides of the tracks
var xpo = int(random(180)); // xvariable for first half of the canvas
var ypo = height;
var treeColor = int(random(100, 255));
var treeSize = int(random(20, 40));
trees.push(makeTree(xpo, ypo, treeSize, treeColor));
} else if (chance === 6) {
var xpo = int(random(300, width)); // x variable for second half of the canvas
var ypo = height;
var treeColor = int(random(100, 255));
var treeSize = int(random(20, 30));
trees.push(makeTree(xpo, ypo, treeSize, treeColor));
}
}
function removeTracks() {
// creating a duplicate array to keep track objects that are within the canvas
var trackKeep = [];
for (var i = 0; i < traintracks.length; i++) {
if (traintracks[i].y > -10) {
trackKeep.push(traintracks[i]);
}
}
traintracks = trackKeep; // reassigning the tracks array to the duplicate array
// creating a duplicate array to keep track objects that are within the canvas
var oasesKeep = [];
for (var i = 0; i < oases.length; i++) {
if (oases[i].y > -40) {
oasesKeep.push(oases[i]);
}
}
oases = oasesKeep; // reassigning the oases array to the duplicate array
// creating a duplicate array to keep track objects that are within the canvas
var treeKeep = [];
for (var i = 0; i < trees.length; i++) {
if (trees[i].y > -40) {
treeKeep.push(trees[i]);
}
}
trees = treeKeep; // reassigning the trees array to the duplicate array
}
function trackMove() { // moving the position of each track and oases object
this.y -= 10;
}
function trackDisplay() { // displaying each track object with rectangles
strokeWeight(1);
fill(97, 74, 66);
rect(this.x, this.y, 60, 10);
}
function makeTrainTracks(xpos, ypos) { //object for making traintrack
var trck = {x: xpos, y: ypos, move: trackMove,
display: trackDisplay}
return trck;
}
function makeOasis(xco, yco, color, xscale, yscale) { //object for making oases
var oasis = {x: xco, y: yco, move: trackMove,
display: oasisDisplay, tone: color,
size1: xscale, size2: yscale};
return oasis;
}
function oasisDisplay() { // displaying the oases objects as an ellipse
fill(0, this.tone, this.tone);
noStroke();
ellipse(this.x, this.y, this.size1, this.size2);
strokeWeight(1);
stroke(0);
}
function makeTree(xp, yp, xsize, colour) { //function for making tree objects
var tree1 = {x: xp, y: yp, move: trackMove, display: treeDisplay,
tone: colour, size1: xsize};
return tree1;
}
function treeDisplay() { // displaying the tree objects by drawing from parameters
noStroke();
fill(0, this.tone, 0);
ellipse(this.x, this.y, this.size1 * 1.5);
fill(0, this.tone - 50, 0);
ellipse(this.x, this.y, this.size1);
}
function drawTrain() { // drawing the train object as a static image
noStroke();
fill(200, 100, 100);
rect(207, 220, 67, 160);
rect(207, 390, 67, 160);
strokeWeight(4);
stroke(0);
noFill()
rect(230, 382, 20, 7);
strokeWeight(2);
rect(220, 230, 40, 120);
rect(220, 410, 40, 120);
}
For this project, I chose to animate an aerial view over a train moving across a desert. I’ve always been interested in Wild West type scenes, so the image of a train moving past oases and small little cacti is one that stood out to me. I attached a sketch of my thought process at the bottom.
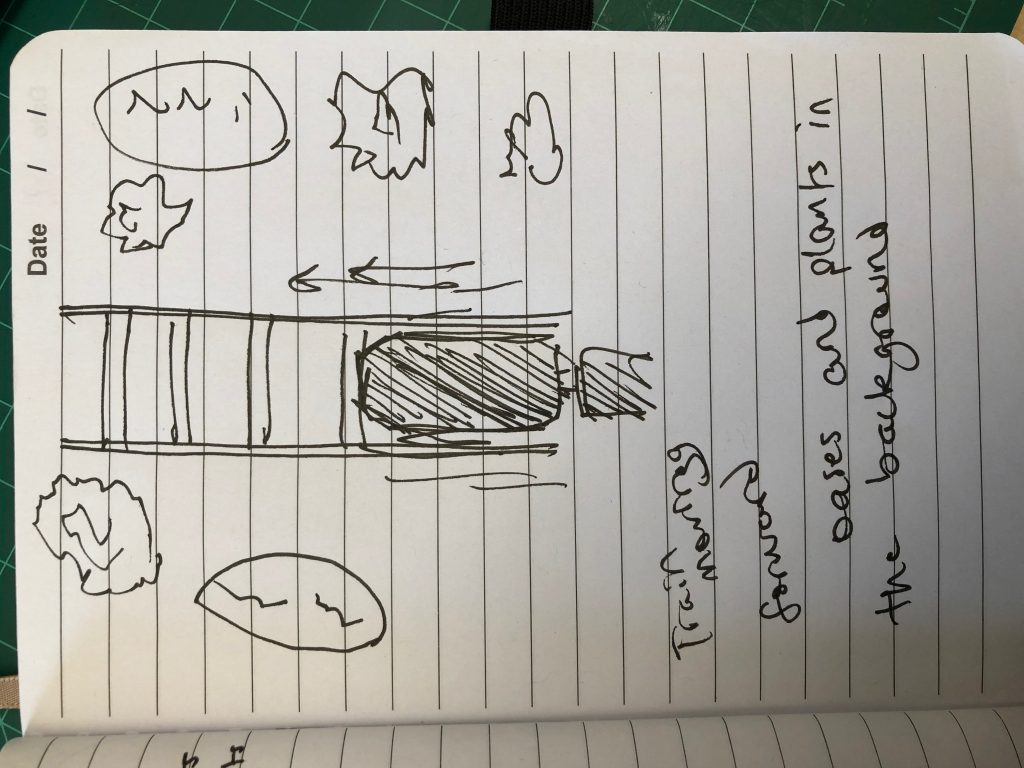