// Paul Greenway
// pgreenwa
// pgreenwa@andrew.cmu.edu
// Project-11-Landscape
var terrainSpeed = 0.0005;
var terrainDetail = 0.005;
var buildings = [];
var trees = [];
var c1;
var c2;
function setup() {
createCanvas(480, 300);
//gradient background color set
c1 = color(0, 90, 186);
c2 = color(255, 166, 0);
setGradient(c1, c2);
// create an initial collection of buildings
for (var i = 0; i < 20; i++){
var rx = random(width);
buildings[i] = makeBuilding(rx);
}
frameRate(60);
}
function draw() {
setGradient(c1, c2);
mountains1();
mountain2();
strokeWeight(1);
updateAndDisplayBuildings();
removeBuildingsThatHaveSlippedOutOfView();
addNewBuildingsWithSomeRandomProbability();
updateAndDisplayTrees();
removeTreesThatHaveSlippedOutOfView();
addNewTreesWithSomeRandomProbability();
fill(40);
rect (0,height-60, width, height-50);
noFill();
stroke(255);
strokeWeight(1);
line(0,height-40, width, height-40);
strokeWeight(3);
line(0,height-30, width, height-30);
noStroke();
fill(255);
ellipse(500,50,30,30);
}
//create background mountains
function mountains1() {
noStroke();
fill(255,100);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail*3) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, height/4, height/2);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
}
function mountain2() {
noStroke();
fill(185, 96, 0, 100);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail*3) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, height/1.5, height/2);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
}
//set background color gradient
function setGradient(c1, c2) {
noFill();
for (var y = 0; y < 300; y++) {
var inter = map(y, 0, 150, 0, 1);
var c = lerpColor(c1, c2, inter);
stroke(c);
line(0, y, width, y);
}
}
//create windy trees
function updateAndDisplayTrees(){
for (var i = 0; i < trees.length; i++){
trees[i].move();
trees[i].display();
}
}
function removeTreesThatHaveSlippedOutOfView(){
var treesToKeep = [];
for (var i = 0; i < trees.length; i++){
if (trees[i].x + trees[i].breadth > 0) {
treesToKeep.push(trees[i]);
}
}
trees = treesToKeep; // remember the surviving buildings
}
function addNewTreesWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newTreeLikelihood = 0.01;
if (random(0,1) < newTreeLikelihood) {
trees.push(makeTree(width));
}
}
function treeMove() {
this.x += this.speed;
}
function treeDisplay() {
var treeHeight = random(45,50);
var tHeight = treeHeight;
fill(0,100);
noStroke();
push();
translate(this.x, height - 40);
ellipse(0, -tHeight, this.breadth-10, tHeight);
fill('black');
rect(0-4,-tHeight, this.breadth-45, tHeight);
pop();
}
function makeTree(birthLocationX) {
var tre = {x: birthLocationX,
breadth: 50,
speed: -1.0,
nFloors: round(random(2,8)),
move: treeMove,
display: treeDisplay}
return tre;
}
//create buildings based on starter code
function updateAndDisplayBuildings(){
for (var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].display();
}
}
function removeBuildingsThatHaveSlippedOutOfView(){
var buildingsToKeep = [];
for (var i = 0; i < buildings.length; i++){
if (buildings[i].x + buildings[i].breadth > 0) {
buildingsToKeep.push(buildings[i]);
}
}
buildings = buildingsToKeep; // remember the surviving buildings
}
function addNewBuildingsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newBuildingLikelihood = 0.005;
if (random(0,1) < newBuildingLikelihood) {
buildings.push(makeBuilding(width));
}
}
function buildingMove() {
this.x += this.speed;
}
function buildingDisplay() {
var floorHeight = 20;
var bHeight = this.nFloors * floorHeight;
fill(100,200);
noStroke();
push();
translate(this.x, height - 40);
rect(0, -bHeight, this.breadth, bHeight);
noStroke();
fill(255);
for (var i = 0; i < this.nFloors; i++) {
ellipse(3+5,-10+20*-i,5,5);
}
for (var y = 0; y < this.nFloors; y++) {
ellipse(13+5,-10+20*-y,5,5);
}
for (var z = 0; z < this.nFloors; z++) {
ellipse(23+5,-10+20*-z,5,5);
}
for (var x = 0; x < this.nFloors; x++) {
ellipse(33+5,-10+20*-x,5,5);
}
pop();
}
function makeBuilding(birthLocationX) {
var bldg = {x: birthLocationX,
breadth: 50,
speed: -1.0,
nFloors: round(random(2,8)),
move: buildingMove,
display: buildingDisplay}
return bldg;
}
For this project I wanted to create the view of a landscape as seen when driving in a car. I made use of the base passing buildings object as well as adding trees, mountains, and a gradient sky color to create the overall composition.
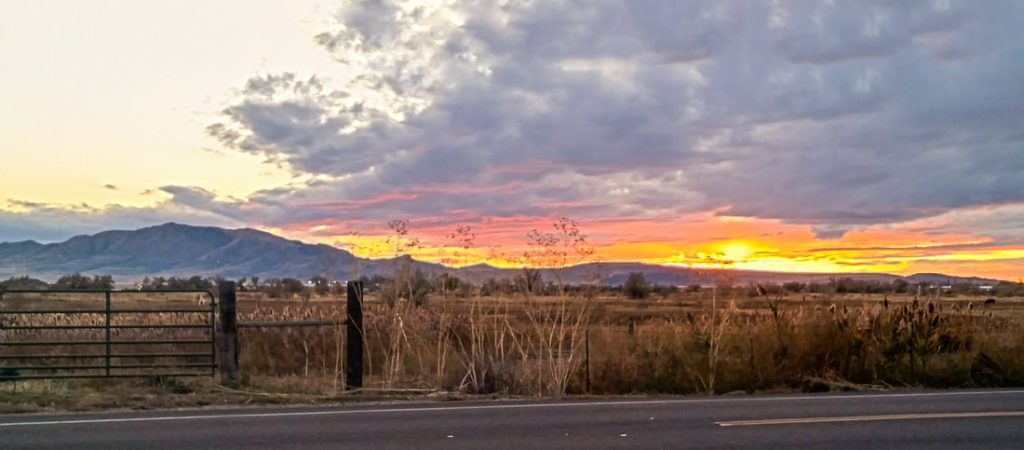