//initialize variables
var terrainSpeed = 0.0005;
var terrainDetail = 0.005;
var groundDetail= 0.005;
var groundSpeed = 0.0005;
var cactus = [];
var col1, col2;
function setup() {
createCanvas(480,480);
frameRate(10);
//start landscape out with 3 cacti
for(i = 0; i < 3; i++){
var rx = random(0,width);
cactus[i] = makeCactus(rx);
}
}
function draw (){
//create gradient colors
c1= color(255, 149, 0);
c2= color(235, 75, 147);
setGradient(c1, c2);
//call functions
drawDesertMtn();
updateCactus();
removeCactus();
addCactus();
}
function setGradient(c1, c2){
//create the gradient background
noFill();
for(i = 0; i < height; i++){
var grad = map(i, 0, height, 0, 1);
var col = lerpColor(c1, c2, grad);
stroke(col);
line(0, i, width, i);
}
}
function drawDesertMtn(){
//back mtn
fill(255, 155, 84);
noStroke();
beginShape();
//make the terrain randomize and go off screen
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0,2, 50, height);
vertex(x, y);
}
vertex(width,height);
vertex(0,height);
endShape();
//middle mtn
fill(245, 105, 98);
noStroke();
beginShape();
//make the terrain randomize and go off screen
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0,1, 100, height);
vertex(x, y);
}
vertex(width,height);
vertex(0,height);
endShape();
//closest mtn
fill(224, 54, 139);
noStroke();
beginShape();
//make the terrain randomize and go off screen
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0,1,200,height);
vertex(x, y);
}
vertex(width,height);
vertex(0,height);
endShape();
//groud
fill(165, 196, 106);
noStroke();
beginShape();
//make the ground randomize and go off screen
for (var x = 0; x < width; x++) {
var t = (x * groundDetail) + (millis() * groundSpeed);
var y = map(noise(t), 0, 1,400,480);
vertex(x, y);
}
vertex(width, height);
vertex(0,height);
endShape();
}
//change x value of cactus as cactus moves
function moveCactus(){
this.x += this.speed;
}
function makeCactus(cactusX){
//create the cactus as an object w some random parts
var cactus={
x:cactusX,
speed: -6,
breadth:311,
color: random(0,100),
length: random(50,100),
move: moveCactus,
display: displayCactus,
}
return cactus;
}
function updateCactus(){
//loop through an array of cactus to update as adding and moving
for(i = 0; i < cactus.length; i++){
cactus[i].move();
cactus[i].display();
}
}
function displayCactus(){
//create the cactus visuals with object parts
noStroke();
fill(this.color, 107, 9);
//translate to x value of new cactus
push();
translate(this.x+157, height-40);
ellipse(0,0,94,137);
fill(this.color, 107,9);
ellipse(61, -52, this.length,48);
fill(this.color, 115, 28);
ellipse(47,-83,19,27);
fill(90, 115, 22);
ellipse(81, -74, 24,24);
fill(90, 115, 22);
ellipse(-34, -86, this.length, 74);
fill(this.color, 115, 22);
ellipse(-18, -131, 41,41);
fill(252, 197, 247);
//create flower on cactus
beginShape();
curveVertex(-1, -150);
curveVertex(-7,-152);
curveVertex(-7, -147);
curveVertex(-10, -157);
curveVertex(-5, -141);
curveVertex(-4, -135);
curveVertex(1, -140);
curveVertex(8, -135);
curveVertex(8, -142);
curveVertex(8, -147);
curveVertex(3, -148);
curveVertex(3, -153);
curveVertex(-1, -150);
endShape();
pop();
}
function addCactus(){
//add a new cactus to the array
var newCactus = 0.01;
if(random(0,1)< newCactus){
cactus.push(makeCactus(width));
}
}
function removeCactus(){
//remove cactus from array as it goes off the screen
var keepCactus = [];
for(i = 0; i < cactus.length; i++){
if(cactus[i].x + cactus[i].breadth > 0){
keepCactus.push(cactus[i]);
}
}
cactus = keepCactus;
}
I was inspired by desert landscapes in which the sun is setting and there are cacti as well as communicate the vastness of a place like joshua tree.ca.
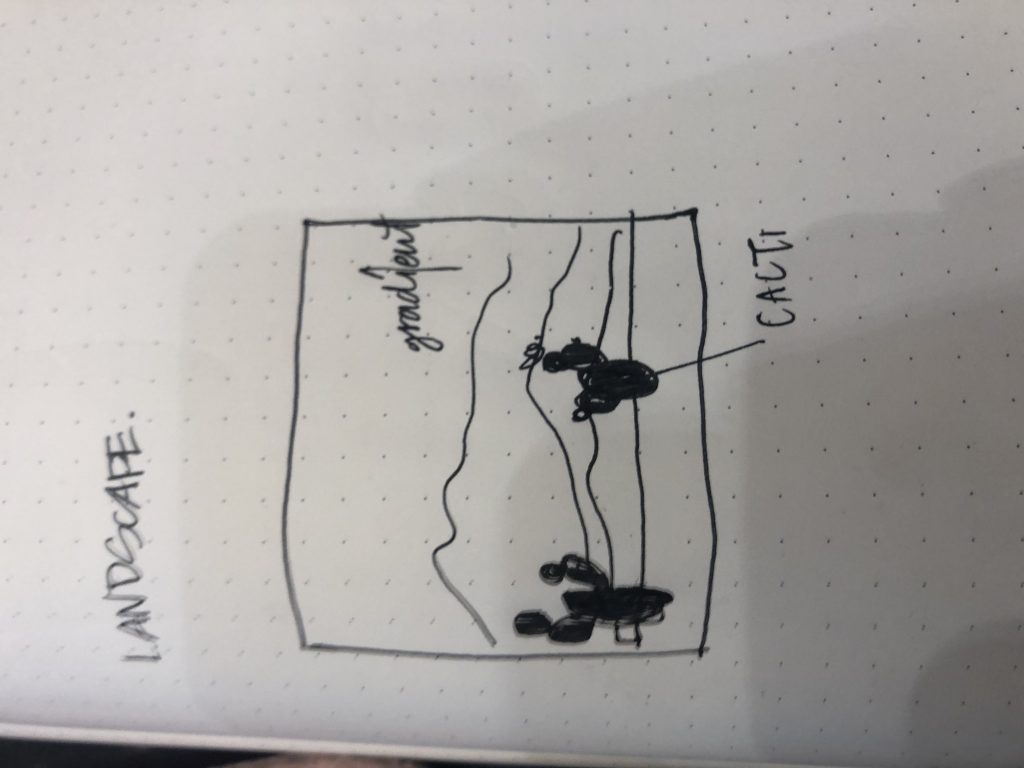