//Crystal Xue
//15104-section B
//luyaox@andrew.cmu.edu
//Project-11
var terrainSpeed;
var terrainDetail;
var c1, c2;
var cacti = [];
var cWidth = 50; //cactus Width
var cLeft = - 20 - cWidth; //left point of cactus drawn
function setup() {
createCanvas(480, 240);
// create an initial collection of cacti
for (var i = 0; i < 4; i++){
var xLocation = random(width);
var yLocation = random(150, 170);
cacti[i] = makeCacti(xLocation, yLocation);
}
frameRate(20);
//two end colors of the gradient backgound
c1 = color(134, 162, 148);
c2 = color(245, 193, 140);
}
function draw() {
setGradient(c1, c2);
terrain();
//addCacti();
updateCacti();
}
function drawCacti() {
noStroke();
fill(255, 230, 238);
push();
translate(this.x2, this.y2);
scale(this.cactiSize);
stroke(61,73,49);
strokeWeight(10);
line(0, 0, 0,60);
noFill();
stroke(61,73,49);
strokeWeight(10);
beginShape();
curveVertex(-20,20);
curveVertex(-20,20);
curveVertex(-15,30);
curveVertex(0,30);
curveVertex(20,25);
curveVertex(25,-10);
curveVertex(25,-10);
endShape();
stroke(100,100,89);
strokeWeight(0.5);
line(0, -2, 0,62);
line(2, -2, 2,62);
line(-2, -2, -2,62);
//draw cacti spines
fill(0);
noStroke();
ellipse(0,2,2,2);
ellipse(5,20,3,3);
ellipse(20,30,2,2);
ellipse(30,10,2,2);
ellipse(-15,17,3,3);
ellipse(-10,27,2,2);
ellipse(5,45,2,2);
pop();
}
function makeCacti(xlocation, ylocation) {
//Cacti objects
var makeCactus = {x2: xlocation,
y2: ylocation,
cactiSize: random(0.8,1.5),
speed: -2.0,
move: moveCacti,
draw: drawCacti}
return makeCactus;
}
function moveCacti() {
//make cacti move
this.x2 += this.speed;
if (this.x2 <= cLeft) {
this.x2 += width - cLeft;
}
}
function updateCacti() {
for(i = 0; i < cacti.length; i++) {
cacti[i].move();
cacti[i].draw();
}
}
function setGradient(c1, c2) {
//gradient color background
noFill();
for (var y = 0; y < height; y++) {
var inter = map(y, 0, height, 0, 1);
var c = lerpColor(c1, c2, inter);
stroke(c);
line(0, y, width, y);
}
}
function terrain(){
terrainSpeed = 0.0002;
terrainDetail = 0.006;
//draw dessert mountain 1
fill(225, 184, 139);
noStroke();
beginShape();
vertex(0, height);
for (var x = 0; x < width; x++) {
var t1 = (x * terrainDetail) + (millis() * terrainSpeed);
var y1 = map(noise(t1), 0, 1, 80, height);
vertex(x, y1);
}
vertex(width, height);
endShape();
//draw dessert mountain 2
terrainDetail = 0.007;
fill(225, 164, 109);
noStroke();
beginShape();
vertex(0, height);
for (var x = 0; x < width; x++) {
var t1 = (x * terrainDetail) + (millis() * terrainSpeed);
var y1 = map(noise(t1), 0, 1, 100, height);
vertex(x, y1);
}
vertex(width, height);
endShape();
//draw ground
terrainDetail = 0.0005;
fill(160, 96, 69);
noStroke();
beginShape();
vertex(0, height);
for (var x = 0; x < width; x++) {
var t2 = (x * terrainDetail) + (millis() * terrainSpeed);
var y2 = map(noise(t2), 0, 1, 180, height);
vertex(x, y2);
}
vertex(width, height);
endShape();
}
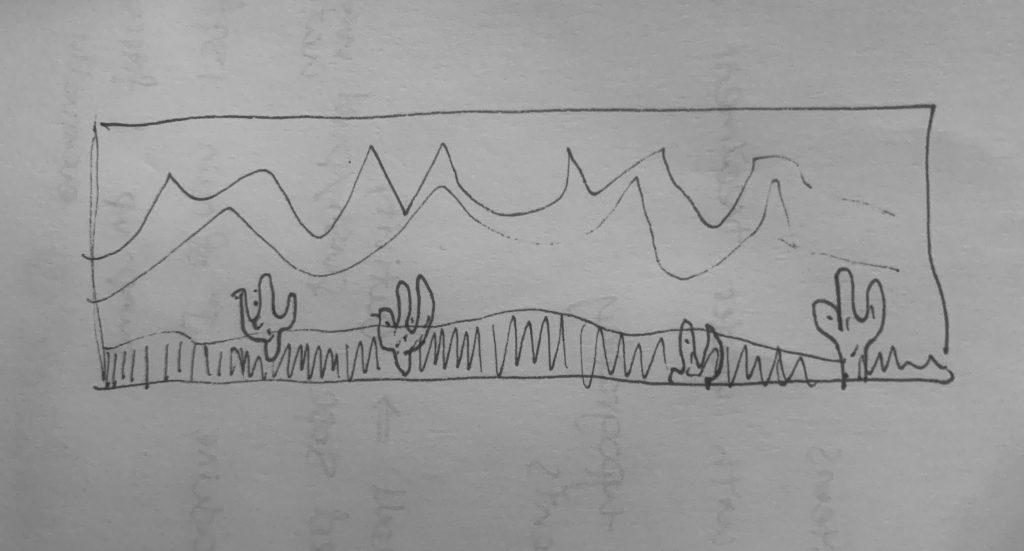
This is a landscape of a desert with different sized cactus along the road and different depth of the scenery.