function setup() {
createCanvas(600, 450);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(0);
noStroke();
//boxes coming in from the right
fill(mouseY, 0, mouseX);
rect(0, 0, mouseX, 50);
fill(0, mouseY/2, mouseX/2);
rect(0, 100, mouseX, 50);
fill(mouseY/3, 0, mouseX/3);
rect(0, 200, mouseX, 50);
fill(0, mouseY, mouseX);
rect(0, 300, mouseX, 50);
fill(mouseY/2, 0, mouseX/2);
rect(0, 400, mouseX, 50);
//boxes coming in from the left
fill(255-mouseY, 0, 255-mouseX);
rect(600, 50, -mouseX, 50);
fill(0, 255-mouseY/2, 255-mouseX/2);
rect(600, 150, -mouseX, 50);
fill(255-mouseY/3, 0, 255-mouseX/3);
rect(600, 250, -mouseX, 50);
fill(0, 255-mouseY, 255-mouseX);
rect(600, 350, -mouseX, 50);
//boxes coming in from the top
fill(255-mouseY, 0, 255-mouseX);
rect(0, 0, 50, mouseY);
fill(0, 255-mouseY/2, 255-mouseX/2);
rect(120, 0, 50, mouseY);
fill(255-mouseY/3, 0, 255-mouseX/3);
rect(240, 0, 50, mouseY);
fill(0, 255-mouseY, 255-mouseX);
rect(360, 0, 50, mouseY);
fill(255-mouseY/2, 0, 255-mouseX/2);
rect(480, 0, 50, mouseY);
//boxes coming in from the bottom
fill(mouseY, 0, mouseX);
rect(60, 600, 50, -mouseY);
fill(0, mouseY/2, mouseX/2);
rect(180, 600, 50, -mouseY);
fill(mouseY/3, 0, mouseX/3);
rect(300, 600, 50, -mouseY);
fill(0, mouseY, mouseX);
rect(420, 600, 50, -mouseY);
fill(mouseY/2, 0, mouseX/2);
rect(540, 600, 50, -mouseY);
//moving origin point to the center
translate(300, 225);
//rect & all ellipses rotate based on mouse
rectMode(CENTER);
rotate(radians(mouseX));
rect(0, 0, 100, 100);
//ellipses change position based on mouse
ellipse(-100, mouseX, 30, 30);
ellipse(mouseX, -100, 30, 30);
//ellipses get bigger based on mouse
stroke(255);
strokeWeight(3);
noFill();
ellipse(0, 0, mouseX, mouseY);
stroke(100);
ellipse(0, 0, -mouseX, mouseY);
//rotated ellipses
rotate(radians(45));
stroke(255);
ellipse(0, 0, mouseX+20, mouseY);
stroke(100);
ellipse(0, 0, -mouseX+20, mouseY);
rotate(radians(45));
stroke(255);
ellipse(0, 0, mouseX+50, mouseY);
stroke(100);
ellipse(0, 0, -mouseX+50, mouseY);
rotate(radians(45));
stroke(255);
ellipse(0, 0, mouseX+90, mouseY);
stroke(100);
ellipse(0, 0, -mouseX+90, mouseY);
}
I was inspired by the shape of atoms. For the background, I was partly inspired by the way a tv looks when it glitches.
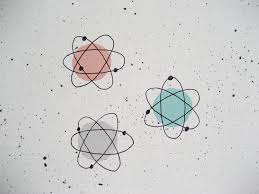
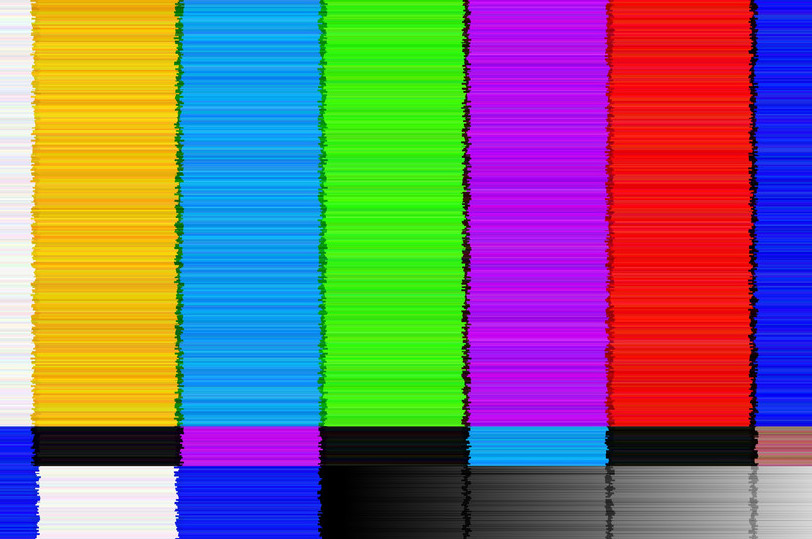