I was somewhat inspired by the overlapping tonal shapes of the art of designer Verner Panton, so I decided to use a similar concept for building tonal shades through scaling, adapting to the mouse movements. The whole structure of the program runs off of one while loop to create the intervals in spacing, location, rotation, and scale that many of the objects have.
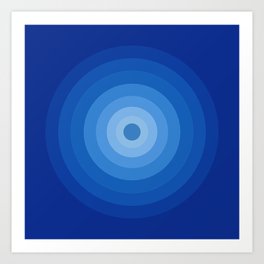
var centerX = 300;
var centerY = 225;
function setup() {
createCanvas(600, 450);
background(255);
}
function draw() {
noStroke();
background(255);
rectMode(CENTER);
let i =0;
while(i<mouseX){
noStroke();
//centered rectangle matrix
fill(0,0,mouseX/3,20);
rect(centerX,centerY,mouseX-i,mouseY-i);
rect(centerX,centerY,mouseY-i,mouseX-i);
//circles
fill(255-(mouseY/3),0,255-(mouseX/3),10);
//top left
ellipse(50,50,width-(mouseX-i),height-(mouseY-i));
ellipse(50,50,mouseY-i,mouseX-i);
//top right
ellipse(550,50,width-(mouseX-i),height-(mouseY-i));
ellipse(550,50,mouseY-i,mouseX-i);
//bottom right
ellipse(550,400,width-(mouseX-i),height-(mouseY-i));
ellipse(550,400,mouseY-i,mouseX-i);
//bottom left
ellipse(50,400,width-(mouseX-i),height-(mouseY-i));
ellipse(50,400,mouseY-i,mouseX-i);
//gray bars
fill(0,0,0,7);
//left vert
rect(150,centerY,300-(mouseY-(i/2)),height);
//right vert
rect(450,centerY,300-(mouseY-(i/2)),height);
//white bars
fill(255);
rect(centerX, centerY-i,width, i/20);
rect(centerX, centerY+i,width, i/20);
//black twisting lines, use i to determine degree
stroke(0);
line(50,50+(i/2),550,400-(i/2));
line(550-(i/2),50,50+(i/2),400);
i+=50;
}
//white ellipses
strokeWeight(10);
stroke(255);
noFill();
let j=0;
while(j<15){
strokeWeight(5/j);
ellipse(centerX, centerY+i,mouseX+(50*j), mouseX+(50*j));
ellipse(centerX, centerY-i,mouseX+(50*j), mouseX+(50*j));
j++;
}
}