For Project 6, I created a rather simple 24 hr clock but displayed abstractly. I purposely duplicated multiple clocks into one composition to create visual intrigue and guide the eye through the mesmerizing movement of the seconds, minutes, and hours. I was inspired by the Apple Watch and its demonstration of time through simplicity and color.
function setup() {
createCanvas(400, 400);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
angleMode(DEGREES);
}
function draw() {
background(38, 84, 124);
//clock transformation
//total of nine clocks
push();
translate(80, 80);
scale(0.3);
rotate(-90);
clock();
pop();
push();
translate(320, 320);
scale(0.3);
rotate(-90);
clock();
pop();
push();
translate(80, 320);
scale(0.3);
rotate(-90);
clock();
pop();
push();
translate(320, 80);
scale(0.3);
rotate(-90);
clock();
pop();
push();
translate(200, 80);
scale(0.3);
rotate(-90);
clock();
pop();
push();
translate(200, 320);
scale(0.3);
rotate(-90);
clock();
pop();
push();
translate(200, 200);
scale(0.3);
rotate(-90);
clock();
pop();
push();
translate(80, 200);
scale(0.3);
rotate(-90);
clock();
pop();
push();
translate(320, 200);
scale(0.3);
rotate(-90);
clock();
pop();
}
function one() {
//seconds function
let s = second();
strokeCap(SQUARE);
noFill();
strokeWeight(50);
stroke(6, 214, 160);
//movement
let secondHand = map(s, 0, 60, 0, 360);
arc(0, 0, 100, 100, 0, secondHand);
}
function two() {
//minute function
let m = minute();
strokeCap(SQUARE);
noFill();
stroke(255, 209, 102);
//movement
let minuteHand = map(m, 0, 60, 0, 360);
arc(0, 0, 200, 200, 0, minuteHand);
}
function three() {
//hour function
let h = hour();
strokeCap(SQUARE);
noFill();
stroke(239, 71, 111);
//movement
let hourHand = map(h % 24, 0, 24, 0, 360);
arc(0, 0, 300, 300, 0, hourHand);
}
function clock() {
//entire clock
one();
two();
three();
//center of clock
fill(255);
noStroke();
circle(0, 0, 50);
}
Inspiration:
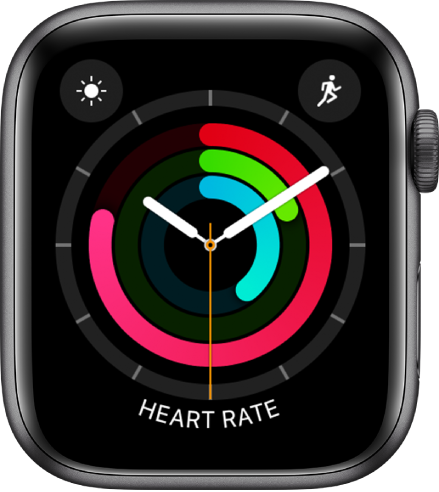