With my short-lived experience in physical computing taking an intro Arduino class last semester, I was drawn to the appearance of Ototo by Yuri Suzuki. It resembles a chip but claims to be a musical invention kit. Reading into it, Ototo does exactly as it promises. It allows the user to interact tactically with the product to experiment with possible sounds, manipulate add-ons that increase the range of sounds that can be made, and wire the product to everyday items (i.e. water, a piece of cake, a spoon) so that the musical performance experience expands to include any object you desire.
What I love about the Ototo is the accessibility of the product by the communicative means of sounds and its relationship with touch. From a young age, we are introduced to toys with buttons assigned to certain sound effects. I think this project builds on that simple concept but elevates it to something even more interesting by allowing the user to transform their own personal items into musical instruments. I’m unsure of how the machine detects changes in the connected objects (conductivity?), but I do know that a lot of thought was put into writing code that assigns sounds to different ports on the device that is further changed based on the additional input information from the connected objects. I think Suzuki’s artistry comes into this project in the consideration of human emotion and how we respond to interactivity and sound. His sound projects are highly engaging and portray sound as a viable and fun aspect of art and design.
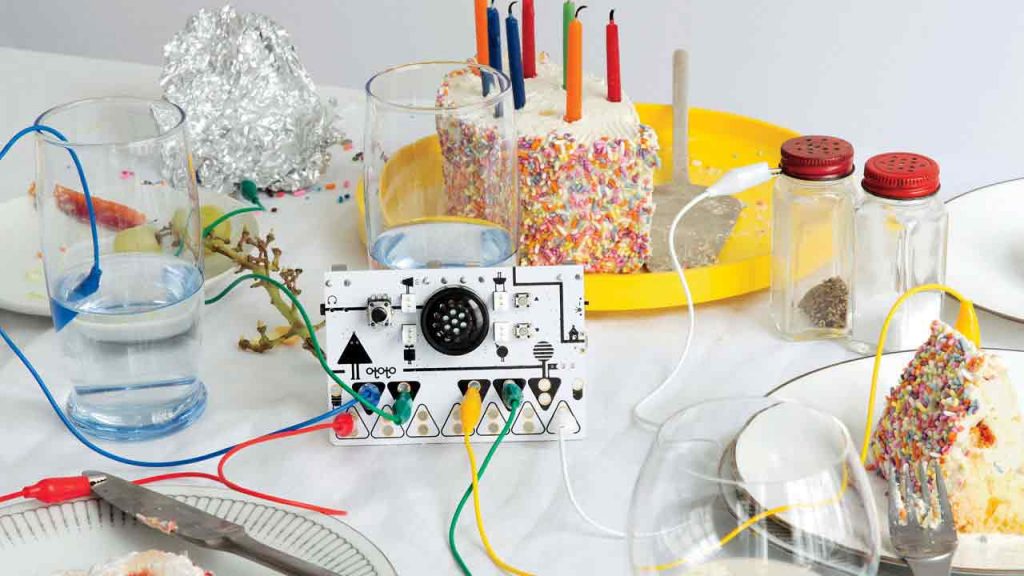