//Robert Rice
//Section C
var gender = 0 //"gender", determines eyelashes
var eyeSize = 10 //sets eye diameter
var eyeSquint = 1 //how squinted the eyes are
var eyebrowLength = 70 //how long the eyebrows are
var mouthLength = 90 //how long the mouth is
var mouthPosition = 0 //adjust how high up the mouth is
var noseX1 = 250 //adjusts the base of the nose
var noseY1 = 230
var noseX2 = 270 //adjust the tip of the nose
var noseY2 = 220
function setup() {
createCanvas(400, 400);
text("p5.js vers 0.9.0 test.", 10, 15);
angleMode(DEGREES); //degrees bc i'm bad at math
}
function draw() {
background(0) //black background
noFill();
stroke(255); //white lines bc the background is black
strokeWeight(3); //draws the basic face shape
strokeJoin(ROUND);
line(140,270, 127,220);
arc(193,202, 136,136, 165, 73.38);
line(212,268, 232,288);
strokeWeight(2); //stroke weight for facial features
if (gender>0.5) { //Gives the face eyelashes if it's 'female'
line(186,180, 172,180); //does nothing if the face is 'male'
line(186,180, 174,173);
line(186,180, 180,168);
line(186+97,180, 172+97,180);
line(186+97,180, 174+97,173);
line(186+97,180, 180+97,168);
}
noStroke(); //Makes the pupils
fill(255);
ellipse(186,180, eyeSize, eyeSize / eyeSquint); //pupils squint based on
ellipse(186+97,180, eyeSize, eyeSize / eyeSquint); //eyeSquit variable
stroke(255); //makes the eyebrow arcs, which move with the eyes
strokeWeight(2);
noFill();
arc(201,6-((eyeSize/eyeSquint)/2), 351,351, eyebrowLength, 94.91);
//left eyebrow arc
arc(201+97,6-((eyeSize/eyeSquint)/2), 351,351, eyebrowLength, 94.91);
//right eyebrow arc
arc(246,78 - mouthPosition, 342,342, mouthLength,111.06); //mouth
noStroke();
fill(255);
triangle(255,188, noseX1,noseY1, noseX2,noseY2);
}
function mousePressed() {
// when the user clicks, these variables are reassigned
// to random values within specified ranges.
gender = random(0,1); //variables defined at top of code
eyeSize = random(6,20);
eyeSquint = random(1,2);
eyebrowLength = random(69.42, 82.17);
mouthLength = random(79.13, 104.53);
mouthPosition = random(0,35);
noseX1 = random(247, 260);
noseY1 = random(200, 238);
noseX2 = random(270, 290);
noseY2 = random(199, 232);
}
Category: SectionC
Project 03 – Dynamic Flower
Originally, I had no design in mind for my project. After creating some elements, I realized I had the base for a flower. I used differing opacities to create an aesthetic design.
var x = -225;
var y = -300;
/*d was used as a variable for translation as the artwork was originally
created with a grid*/
var d = 50;
var r = 50;
var angle = 45;
var colorChange = 255;
function setup() {
createCanvas(450, 600);
background(255);
}
function draw() {
var colorReduce = mouseX / 50
background(255);
var extend = 3 * d; //stretches dimensions on varying shapes
translate(width / 2, height / 2); //makes the center the origin
extend -= .3 * mouseX; //modifies the stretching variable
//determines whether an RGB value should be increased or decreased
if (mouseX <= width / 2){
colorChange -= colorReduce
} else {
colorChange = 255
colorChange += colorReduce;
}
/*creates the triangle-circle combination and rotates according to
mousePressed*/
push();
fill(0, 0, colorChange, 60);
rotate(radians(45 - 2 * angle)); //rotates the shapes around the center
translate(-4.5 * d, -6 * d); //simplifies the location of each combo
circle(7 * d + extend, 6 * d, 50);
triangle(5 * d, 6.5 * d, 5 * d, 5.5 * d, 7 * d + extend, 6 * d);
circle(4.5 * d, 9 * d + extend, 50);
triangle(5 * d, 6.5 * d, 4.5 * d, 9 * d + extend, 4 * d, 6.5 * d);
circle(2 * d - extend, 6 * d, 50);
triangle(4 * d, 6.5 * d, 2 * d - extend, 6 * d, 4 * d, 5.5 * d);
circle(4.5 * d, 3 * d - extend, 50);
triangle(4 * d, 5.5 * d, 4.5 * d, 3 * d - extend, 5 * d, 5.5 * d);
pop();
/*creates the tilted petals of the flower and rotates them with the center
when the mouse is pressed*/
push();
rotate(radians(45 + angle));
fill(120, 30, 220, 35);
circle(140, 0, 280);
circle(0, 140, 280);
circle(-140, 0, 280);
circle(0, -140, 280);
pop();
//creates the non-tilted petals and rotates around the center with mouse
push();
rotate(radians(angle));
fill(255, 176, 221, 120);
circle(140, 0, 280);
circle(0, 140, 280);
circle(-140, 0, 280);
circle(0, -140, 280);
pop();
/*creates the ellipses in the center of the petals and rotates them around
the center*/
push();
fill(colorChange, 192, 176, 200);
rotate(radians(2 * angle));
ellipse(0, 100 + r, r, 2 * r);
ellipse(0, -100 - r, r, 2 * r);
ellipse(100 + r, 0, 2 * r, r);
ellipse(-100 - r, 0, 2 * r, r);
pop();
//changes the size of the ellipses based on mouse position
if (mouseY >= height / 2) {
r = 45 + .05 * mouseY;
} else {
r = 50
}
//creates the green center square and rotation for mousePressed
push();
rotate(radians(angle - 45));
rectMode(CENTER);
fill(0, 255, 0, 80);
rect(0, 0, 2 * d - 0.6 * extend, 2 * d - 0.6 * extend);
pop();
//creates the red center square and rotation for mousePressed
push();
rotate(radians(angle));
rectMode(CENTER);
fill(255, 0, 0, 80);
rect(0, 0, 2 * d - .6 * extend, 2 * d - .6 * extend);
pop();
//creates the blue gradient when the y-mouse position changes
let i=3;
while (i<15) {
noStroke(); //produces a graident effect based on ellipses
fill(168, 235, 255, 10);
ellipse(width / 3, height + i, mouseY + 50 * i, mouseY + 50 * i);
ellipse(-width / 3, -height, mouseY + 50 * i, mouseY + 50 * i);
ellipse(width / 3, -height, mouseY + 50 * i, mouseY + 50 * i);
ellipse(-width / 3, height + i, mouseY + 50 * i, mouseY + 50 * i);
i += 1; //increases the modifier as long as i<15
}
}
function mousePressed() {
//for when mouseX is less than the width, the angle of rotations increase
if (mouseX < width) {
angle += 45
}
}
Pinwheel drawing
My process for this project at first was to just mess around and see what shapes i can get to move, and then I realized I can make a moving pinwheel thats speed and direction is determined by mouse clicks and what side of the canvas the clicks occur. Everything after the pinwheel was design to make the appearance look better, the pinwheel is the main part of this piece.
//Anthony Prestigiacomo aprestig section:C
var angle=0;
var degree=5;
var x=-300;
var y=0;
var hvel=5;
var cloudx=0;
var cloudxx=0;
cloudyy=0;
var cloudy=-10;
var r=50;
var vcloud=2;
var vcloud2=2.75;
var suncolor=0;
var bcolor=0;
var mooncolor=0;
function setup() {
createCanvas(600, 450);
background(203,234,246);
}
function draw() {
background(203, 234, 246);
noStroke();
fill(bcolor);
rect(0,0,600,450); //night sky
translate(width / 2, height / 3);
push();
if(mouseX>300){ //twinkling stars
scale(2);
};
fill(203, 234, 246);
circle(50,100,1); //stars
circle(-50,100,1);
circle(57,250,1);
circle(82,-58,1);
circle(-200,133,1);
circle(-5,-124,1);
circle(-72,-230,1);
circle(100,10,1);
circle(150,45,1);
circle(-150,-67,1);
circle(50,50,1);
circle(-50,-50,1);
circle(-50,50,1);
circle(-150,125,1);
circle(-75,-30,1);
circle(-45,-75,1);
circle(-75,-75,1);
circle(-200,-125,1);
pop();
push();
noStroke();
fill(suncolor); //sun
circle(-150,-150,250);
fill(203, 234, 246); //moon
circle(150,150,250);
fill(mooncolor);
circle(105,105,25); //moon craters
circle(100,220,20);
circle(175,175,75);
circle(190,90,40);
push();
translate(-300,-height/3);
fill(212,124,47); //bird on the mouse
circle(mouseX,mouseY,50);
fill(48,46,59);
circle(mouseX+25,mouseY-13,25);
fill(105,107,102);
triangle(mouseX-35,mouseY-5,mouseX+5,mouseY-5,mouseX-25,mouseY+25);
fill(255,255,0);
triangle(mouseX+25, mouseY-13, mouseX+30,mouseY-13, mouseX+25, mouseY-8);
pop();
fill(255); //cloud white
circle(cloudx,cloudy,r); //top cloud
circle(cloudx+25,cloudy,r);
circle(cloudx+12.5,cloudy-25,r);
circle(cloudxx-100, cloudy+150,r); //bottom cloud
circle(cloudxx-75,cloudy+150,r);
circle(cloudxx-87.5,cloudy+125,r);
cloudxx+=vcloud2;
cloudx+= vcloud;
if(cloudxx>400){ //makes clouds reappear on left side of screen
cloudxx=-300;
};
if(cloudx>325){
cloudx=-300;
}
pop();
push();
strokeWeight(10);
stroke(0);
line(0,0,0,height); //pinwheel handle
noStroke();
rotate(radians(angle)); //rotate pinwheel
push();
push();
if(mouseX>width/2){ //scale white triangles
scale(.5)
};
fill(255);
triangle(0,0,100,100,60,10);
triangle(0,0,100,-100,10,-60);
triangle(0,0,-100,100,-10,60);
triangle(0,0,-100,-100,-60,-10);
pop();
if(mouseY>height/3){ //scale colored triangles
scale(1.25)
}
fill(255,0,0);
triangle(0,0,0,145,25,50); //red triangle
fill(0,255,0);
triangle(0,0,0,-145,-25,-50); //green triangle
fill(0,0,255);
triangle(0,0,145,0,50,-25); //blue triangle
fill(255,255,0);
triangle(0,0,-145,0,-50,25); //yellow triangle
pop();
pop();
fill(0);
circle(0,0,25); //black middle circle
fill(255);
circle(0,0,12.5); //small white triangle
rect(x,y-105, 100,1); //wind lines
rect(x+50,y-100, 100,1);
rect(x+25,y-95, 100,1);
x +=hvel;
if(x>=300){ //wind starts at left when passing x=300
x=-300
};
angle+=degree;
fill(0,100,0);
rect(-300, 250,600,75); //grass
}
function mousePressed() {
if(mouseX < width / 2) {
degree -= 5
} else {
degree += 5
};
if (mouseX<width/2 & mouseY>height/3){
suncolor=0;
bcolor=0;
mooncolor=color(211,211,211);
} else {
suncolor=color(255,255,0);
bcolor=color(203, 234, 246);
mooncolor=color(203, 234, 246);
}
}
LO 03: Computational Fabrication
Artwork: Thallus
Creator: Zaha Hadid Architects
Thallus is a complex 3D-printed sculpture. The form is mesmerizing as an open, expending, and curved piece. The “walls” of the sculpture are created by intricate webbings of curves resembling roots. Not only does this create an aesthetic design, the complexity of it truly impressed me. The generation of the art was done through extensive computational geometry. Additionally, more algorithms had to be created for the printing robots to create the spiraling effect and create the artwork in a single piece. A large amount of research was done by the Zaha Hadid to not only create the design with computations, but also to enable the actual printing. The artistry of the designers is apparent with the expansive, yet open spiral that creates a flow to the work. Every part of the piece perfectly coincides with each other to create a balance of elegance and abstractness. The art itself is impressive, however, the most substantial part to me is the complex mathematics and robotic algorithms that actually allowed for the design to come to life in the 3D format.
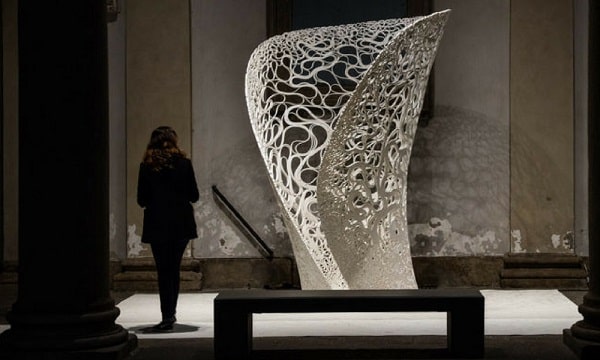
LookingOutwards-03 Section C
A project or work that I find inspirational is Probability Lattice by Marius Watz. This work is a set of 4 3D printed pieces. I admire the design of these pieces, as they are all different and unique yet very similar looking at the same time. Watz designed each parametric design with software much like his other visual abstraction pieces that he designs through generative software processes. Watz likes to design cool looking abstract pieces and this set, Probability Lattice, is an abstract piece designed with software and 3D printed, a combination of all of Watz’s artistic sensibilities. These four pieces in this set are all abstract and made with code, the base layer of what Watz believes his art should be. The 3D printed aspect makes this set a computational digital fabrication.
links: http://mariuswatz.com/2012/05/09/probability-lattice/

**3D printed probability lattices by Marius Watz, designed with software.**
Project-03-Dynamic-Drawing
My concept is creating a very elaborated and aesthetic pattern out of one kind of simple geometric shapes.
var angle=0;
var r;
function setup() {
createCanvas(600, 450);
frameRate(12);
}
function draw() {
//switch color according to mouseX position
if(mouseX<300){
background(100,165,172);
noFill();
stroke(255,196,234);
}
else{
background(255,196,234);
noFill();
stroke(100,165,172);
}
//set the new origin so that ellipses rotate around the center
translate(300,235);
push();
//rotate the first set of ellipses 30 degrees
rotate(radians(30));
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
//60
rotate(radians(30));
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
//90
rotate(radians(30));
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
//120
rotate(radians(30));
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
//150
rotate(radians(30));
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
//180
rotate(radians(30));
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
//210
rotate(radians(30));
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
rotate(radians(30));
//240
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
rotate(radians(30));
//270
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
//300
rotate(radians(30));
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
//330
rotate(radians(30));
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
//360
rotate(radians(30));
ellipseMode(CENTER);
ellipse(0,100,min(100,Math.abs(mouseX-300)),min(200,Math.abs(mouseY-225)));
ellipse(0,100,min(105,Math.abs(mouseX-255)),min(199,Math.abs(mouseY-224)));
ellipse(0,100,min(110,Math.abs(mouseX-250)),min(198,Math.abs(mouseY-223)));
ellipse(0,100,min(115,Math.abs(mouseX-195)),min(197,Math.abs(mouseY-222)));
ellipse(0,100,min(120,Math.abs(mouseX-190)),min(196,Math.abs(mouseY-221)));
pop();
}
Looking Outward-03
This project creates jewelry out of voice by mimicking the shape of the audio’s sound wave. I like this simple yet unexpected way of transforming information. On an abstract level, this project makes use of synesthesia to bridge different sensations, in this case, the hearing and the touching. I admire the connection it builds because offers artists and designers another tool for their creative expression toolset.
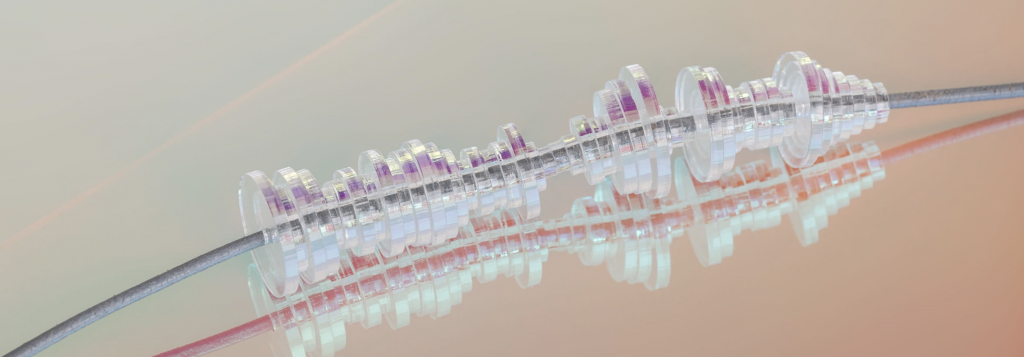
The algorithm should be quite simple. The input will be an audio file. Then, the algorithm processes the file into a sound wave, turns it into a shape, and renders a form base on the shape. Lastly, the form would be sent to the 3D printer.
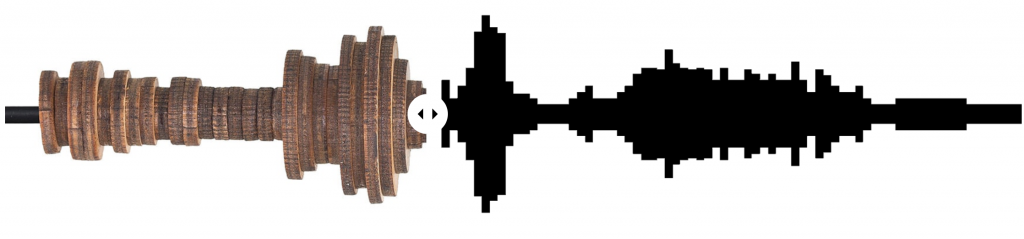
The creator’s artistic sensibilities are not directly manifest in the form but embedded in it. In the project, the role of the creator is more about creating more options for the users to play with.
Looking Outwards 03: Computational Fabrication, SectionC
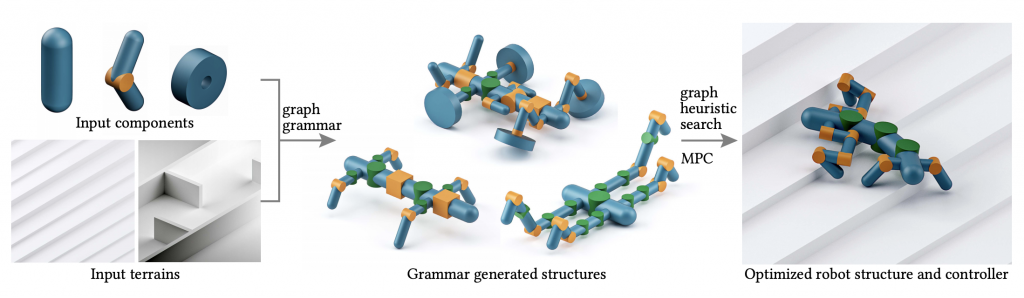
A project I found to be inspirational was a project done by ALLAN ZHAO, JIE XU, MINA KONAKOVIĆ-LUKOVIĆ, JOSEPHINE HUGHES, ANDREW SPIELBERG, DANIELA RUS, and WOJCIECH MATUSIK, called RoboGrammar. This project is an approach to generate different robot structures that is able to move along various terrains. This project is admirable because they were able to come up with a small set of rules that can describe the hundreds and thousands arrangements of physical robot assemblies. While the rules or grammar supports many types of physical arrangements, it also limits the design space to designs that can be physically produced. I thought this related to programming because through assembling a code that supports the randomness of the variations while also setting limitations, a rule is formed that the project follows.
https://cdfg.mit.edu/publications/robogrammar-graph-grammar-for-terrain-optimized-robot-designLooking Outwards 3: Computational Fabrication
The project that I chose to highlight actually belongs to another CMU student –David Perry. It was interesting being able to see his project come to fruition throughout the Lunar Gala preparation process. More recently, I was able to interview him about the details and craftsmanship behind his line. During the interview, David talked about how his background in physics was able to help him develop the algorithms to laser cut the various materials and fabrics he used to construct his clothing line.
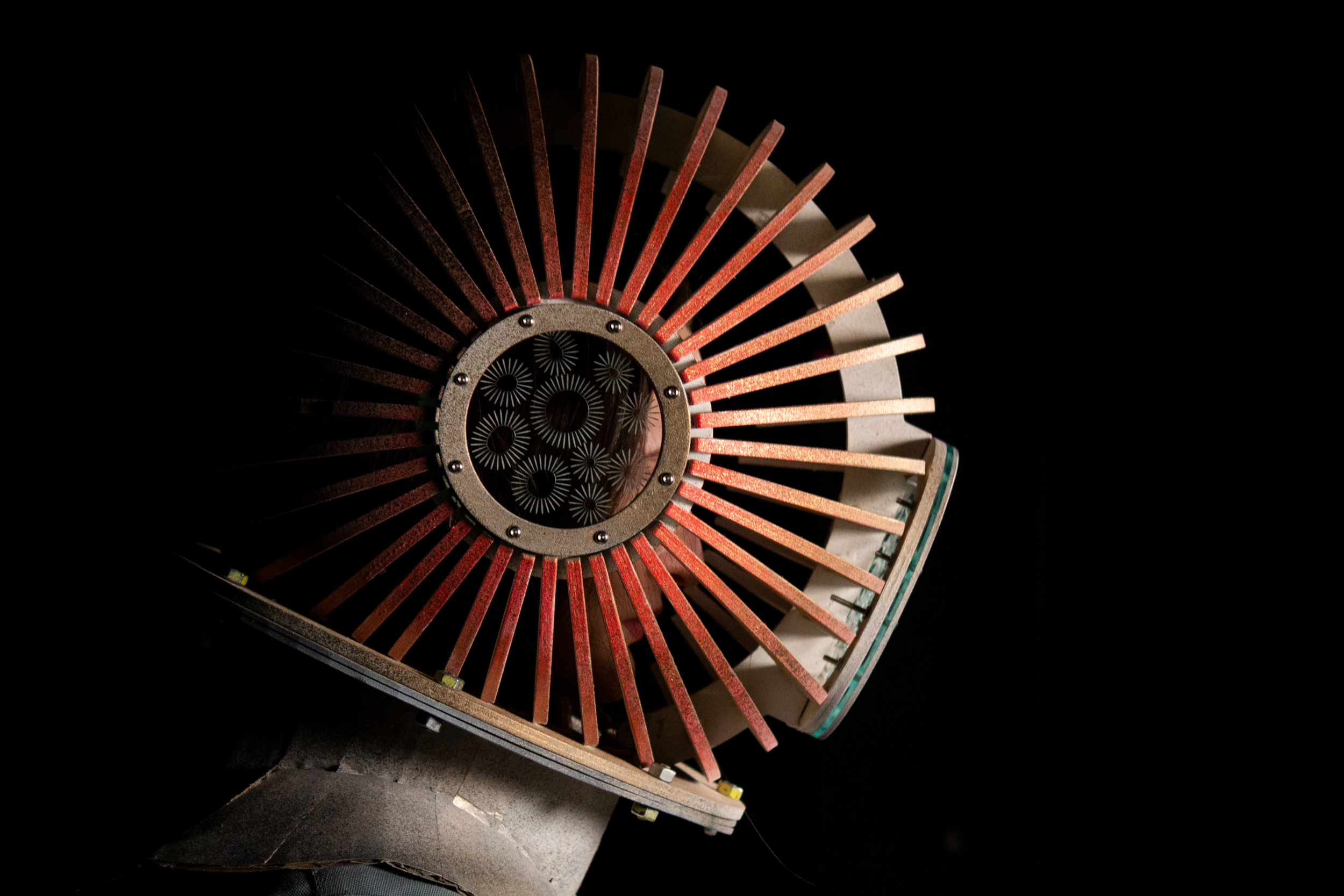
His most famous piece is this scuba diving helmet shown below. The whole product was visualized in CAD before each piece was individually laser cut and eventually put together (systematically) by hand. What I think is so amazing about this clothing line is how he draws together 2 disciplines to create wearable art inspired by the patterns of sea life.
Project 02- Variable Face
var bg1=255
var bg2=98
var bg3=109
var hairlength=200
var teethwidth=50
var teethHeight=50
var lipsWidth= 90
var lipsHeight= 90
var eyeGreen= 77
var hc1=215 // hair color
var hc2= 200
var hc3= 174
function setup() {
createCanvas(480, 640);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(204);
fill (bg1, bg2, bg3); // red dark
rect (0, 0, 480, 640)
fill (238, 208, 191) ; // skin base color
circle (240, 240, 240);
fill (181, 82, 94); // lips
arc (240, 300, lipsWidth, lipsHeight, 245, 600)
fill (255, 254, 246); // teeth
arc (240, 307, teethwidth, teethHeight, 245, 600)
fill (228, 141, 146) ; // cheek left
circle (175, 280, 28);
fill (228, 141, 146) ; // cheek right
circle (305, 280, 28);
fill (223, 190, 170) ; // nose left
circle (230, 278, 10);
fill (223, 190, 170) ; // nose right
circle (250, 278, 10);
fill (223, 190, 170) ; // nose center
circle (240, 280, 20);
fill (235, 258, 234); // eye white left
circle (200, 235, 35)
fill (235, 258, 234); // eye white right
circle (280, 235, 35)
fill (74, eyeGreen, 68); // eye color left
circle (200, 235, 25)
fill (74, eyeGreen, 68); // eye color right
circle (280, 235, 25)
fill (28, 22, 26); // eye pupil left
circle (200, 235, 12)
fill (28, 22, 26); // eye pupil right
circle (280, 235, 12);
fill (251, 255, 248); // eye hl left
circle (205, 230, 5)
fill (251, 255, 248); // eye hlright
circle (285, 230, 5);
fill (154, 114, 71); // eyebrow left thick
rect (165, 205, 50, 5)
fill (154, 114, 71); // eyebrow right thick
rect (260, 205, 50, 5)
fill (hc1, hc2, hc3); // hair left side
rect (100, 180, 60, hairlength)
fill (hc1, hc2, hc3); // hair right side
rect (320, 180, 60, hairlength);
fill (hc1, hc2, hc3); // hair across
arc (240, 200, 280, 190, 531, 710);
}
function mousePressed() {
bg1 = random(1, 254);
bg2 = random(1, 254);
bg3 = random(1, 254);
hairlength = random(200,400);
teethwidth= random(40,60);
teethHeight= random(40,60);
lipsWidth= random(80,100);
lipsHeight= random(80,100);
eyeGreen= random (30,150);
hc1= random (1,254);
hc2= random (1,254);
hc3= random (1,254);
}
I wanted to see variations in color. I changed the eyes and background colors. I also altered hair length and mouth size.