judyli: Abstract Clock Project 06
/*
Judy Li
Section A
judyli@andrew.cmu.edu
Project-06
*/
var prevSec;
var millisRolloverTime;
//--------------------------
function setup() {
createCanvas(300, 300);
millisRolloverTime = 0;
}
//--------------------------
function draw() {
background(255,255,255);
noStroke();
fill(253, 185,200);
rect(0, 65, 300, 57.5);
noStroke();
fill(252, 163, 183);
rect(0, 122.5, 300, 60);
noStroke();
fill(254, 127, 156);
rect(0, 182.5, 300, 60);
noStroke();
fill(251, 96, 127);
rect(0, 242.5, 300, 60);
for (var i = 0; i < width; i = i + 5) {
stroke(255);
strokeWeight(1);
line(i + 5, 0, i + 5, 242.5);
}
for (var i = 0; i < width; i = i + 12.5) {
stroke(255);
strokeWeight(1);
line(i + 12.5, 242.5, i + 12.5, height);
}
// Fetch the current time
var H = hour();
var M = minute();
var S = second();
// Reckon the current millisecond,
// particularly if the second has rolled over.
// Note that this is more correct than using millis()%1000;
if (prevSec != S) {
millisRolloverTime = millis();
}
prevSec = S;
var mils = floor(millis() - millisRolloverTime);
strokeWeight(1);
stroke("pink");
text("M I L L I S : " + mils, 5, 15);
text("S E C O N D : " + S, 5, 30);
text("M I N U T E : " + M, 5, 45);
text("H O U R : " + H, 5, 60);
var hourBarWidth = map(H, 0, 23, 0, width);
var minuteBarWidth = map(M, 0, 59, 0, width);
var secondBarWidth = map(S, 0, 59, 0, width);
// Make a bar which *smoothly* interpolates across 1 minute.
// We calculate a version that goes from 0...60,
// but with a fractional remainder:
var secondsWithFraction = S + (mils / 1000.0);
var secondsWithNoFraction = S;
var secondBarWidthChunky = map(secondsWithNoFraction, 0, 59, 0, width);
var secondBarWidthSmooth = map(secondsWithFraction, 0, 59, 0, width);
strokeWeight(5);
stroke(254, 220, 86);
line(300, 300, hourBarWidth, 250);
stroke(248, 228, 115);
line(300, 240, minuteBarWidth, 190);
stroke(248, 222, 126)
line(300, 180, secondBarWidthChunky, 130);
stroke(252, 244, 163)
line(300, 120, secondBarWidthSmooth, 70);
}
For this project, I think I wanted to start out with a grid system that shows the count of the milliseconds, seconds, minutes, and hours including the real time text of those times. I think that adding a darker tone as the metrics of time increased added a visual value to the graphics. I had fun with this project, but what I would do to make it better, would change the lines to ellipses because I think that it wouldn’t be as skewed as the line when it moves towards the right side.
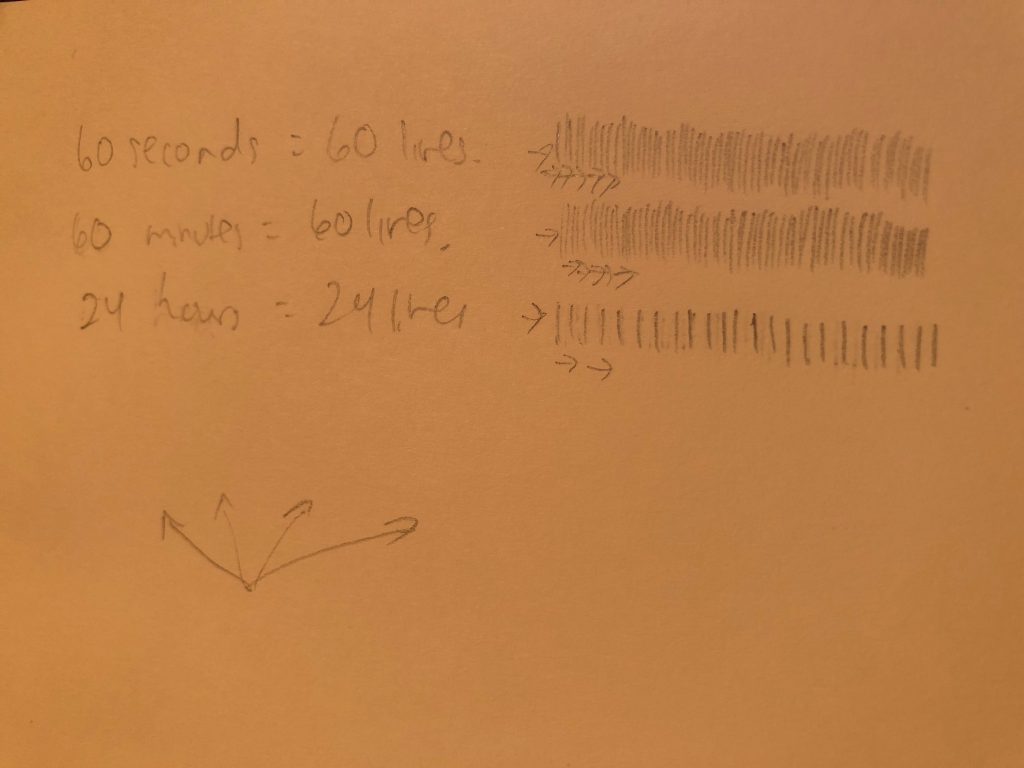